import time
import os
from PyQt5.QtCore import Qt
from PyQt5 import QtWidgets, QtCore, QtGui
from PyQt5.QtGui import *
import cv2
import sys
from ultralytics import YOLO
from PyQt5.QtWidgets import *
import csv
class picture(QWidget):
def __init__(self,username=''):
super(picture, self).__init__()
self.str_name = '0'
self.v8save=False
self.running=True
self.yolo = YOLO('runs/detect/train23/weights/best.pt')#换权重
#定义窗口大小
self.resize(1000, 550)
self.setWindowIcon(QIcon(os.getcwd() + '\\data\\source_image\\Detective.ico'))
self.setWindowTitle("基于改进YOLOv8的谷穗识别系统")#系统标题名字
#定义背景窗口
palette2 = QPalette()
# 加载背景图片
background_image = QPixmap("beijing2.jpg") # 请将"bb.png"替换为你的背景图片文件路径
#设置背景尺寸
background_image = background_image.scaled(1000, 550)
# 将背景图片设置到 QBrush 中
brush = QBrush(background_image)
# 设置背景色为背景图片
palette2.setBrush(self.backgroundRole(), brush)
# 将 QPalette 应用到窗口
self.setPalette(palette2)
self.label1 = QLabel(self)
self.label1.setText("原图")
self.label1.setFixedSize(400, 400)
self.label1.move(165, 60)
self.label1.setStyleSheet("QLabel{background:rgba(255, 255, 255, 40);}"
"QLabel{color:black;font-size:20px;font-weight:bold;font-family:宋体;}"
)
self.label2 = QLabel(self)
self.label2.setText("识别之后的图")
self.label2.setFixedSize(400, 400)
self.label2.move(580, 60)
self.label2.setStyleSheet("QLabel{background:rgba(255, 255, 255, 40);}"
"QLabel{color:black;font-size:20px;font-weight:bold;font-family:宋体;}"
)
#设置按钮
btn = QPushButton(self)
btn.setText("上传图片")
btn.move(20, 100)
btn.setFixedSize(130, 40)
btn.setStyleSheet("background-color: #ADD8E6;")
#connect是连接下面的函数实现功能
btn.clicked.connect(self.openimage)
btn3 = QPushButton(self)
btn3.setText("开始检测")
btn3.setFixedSize(130, 40)
btn3.move(20, 200)
btn3.setStyleSheet("background-color: #9370DB;")
btn3.clicked.connect(self.button1_test1)
self.label = QLabel('识别结果统计:', self)
self.label.move(10, 300)
self.label.setStyleSheet("color: black")
self.label3 = QLabel('谷子穗数:', self)
self.label3.move(10, 330)
self.label3.setFixedSize(130, 40)
self.label3.setStyleSheet("background-color: #90EE90;")
def openimage(self):#打开文件并将图片展示到前端
imgName, imgType = QFileDialog.getOpenFileName(self, "打开图片", "D://", "Image files (*.jpg *.gif *.png *.jpeg)")#"*.jpg;;*.png;;All Files(*)"
if imgName!='':
self.imgname1=imgName
self.im0=cv2.imread(imgName)
# 设置新的图片分辨率框架
width_new = 400
height_new = 400
show = cv2.resize(self.im0, (width_new , height_new))#设置新的尺寸
im0 = cv2.cvtColor(show, cv2.COLOR_RGB2BGR)
showImage = QtGui.QImage(im0, im0.shape[1], im0.shape[0], 3 * im0.shape[1], QtGui.QImage.Format_RGB888)
#将图片映射到前端
self.label1.setPixmap(QtGui.QPixmap.fromImage(showImage))
def button1_test1(self):
if self.imgname1!='0':
#将从前端获取到的值进行入参
t1=time.time()
results = self.yolo(self.imgname1,conf=0.25,iou=0.45,save=False,device='cpu')
res = results[0].boxes.cls.tolist()
endtime = time.time() - t1
im0 = results[0].plot()
im0 = cv2.cvtColor(im0, cv2.COLOR_BGR2RGB)
cv2.imwrite(f'{os.path.join("results_images/", self.imgname1.split("/")[-1])}', cv2.cvtColor(im0, cv2.COLOR_BGR2RGB))
# 设置新的图片分辨率框架
width_new = 400
height_new = 400
im0 = cv2.resize(im0, (width_new, height_new))
image_name = QtGui.QImage(im0, im0.shape[1], im0.shape[0], 3 * im0.shape[1], QtGui.QImage.Format_RGB888)
self.label2.setPixmap(QtGui.QPixmap.fromImage(image_name))
self.label3.setText(f'谷子穗数:{len(res)}')
else:
QMessageBox.information(self, '错误', '请先选择一个图片文件', QMessageBox.Yes, QMessageBox.Yes)
class bg(QMainWindow):
def __init__(self):
super(bg, self).__init__()
self.str_name = '0'
self.resize(1000, 550)
self.setWindowIcon(QIcon(os.getcwd() + '\\data\\source_image\\Detective.ico'))
self.setWindowTitle("基于改进YOLOv8的谷穗识别系统")
label = QLabel(self)
# 创建一个QPixmap对象,加载背景图片
pixmap = QPixmap('beijing3.png') # 请替换为你自己的背景图片文件路径
pixmap = pixmap.scaled(self.size(), Qt.IgnoreAspectRatio)
# 将图片设置到QLabel中
label.setPixmap(pixmap)
# 设置QLabel的位置和大小
label.setGeometry(0, 0, pixmap.width(), pixmap.height())
self.ui_p = picture()
btn = QPushButton(self)
btn.setText("进入系统")
btn.move(420, 350)
btn.setFixedSize(180, 80)
# connect是连接下面的函数实现功能
btn.clicked.connect(self.login)
def login(self):
self.ui_p.show() # 显示你的设计好的界面
self.close()
from time import sleep
if __name__ == '__main__':
app = QApplication(sys.argv)
splash = QSplashScreen(QPixmap(".\\data\\source_image\\logo.png"))
# 设置画面中的文字的字体
splash.setFont(QFont('Microsoft YaHei UI', 12))
# 显示画面
splash.show()
# 显示信息
splash.showMessage("程序初始化中... 0%", QtCore.Qt.AlignLeft | QtCore.Qt.AlignBottom, QtCore.Qt.black)
time.sleep(0.3)
splash.showMessage("正在加载模型配置文件...60%", QtCore.Qt.AlignLeft | QtCore.Qt.AlignBottom, QtCore.Qt.black)
splash.showMessage("正在加载模型配置文件...100%", QtCore.Qt.AlignLeft | QtCore.Qt.AlignBottom, QtCore.Qt.black)
# cam_t=Ui_MainWindow()
ui_p1=bg()
ui_p1.show()
splash.close()
sys.exit(app.exec_())
我的自己的25412736541876
最新推荐文章于 2024-09-12 12:49:01 发布
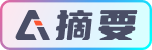