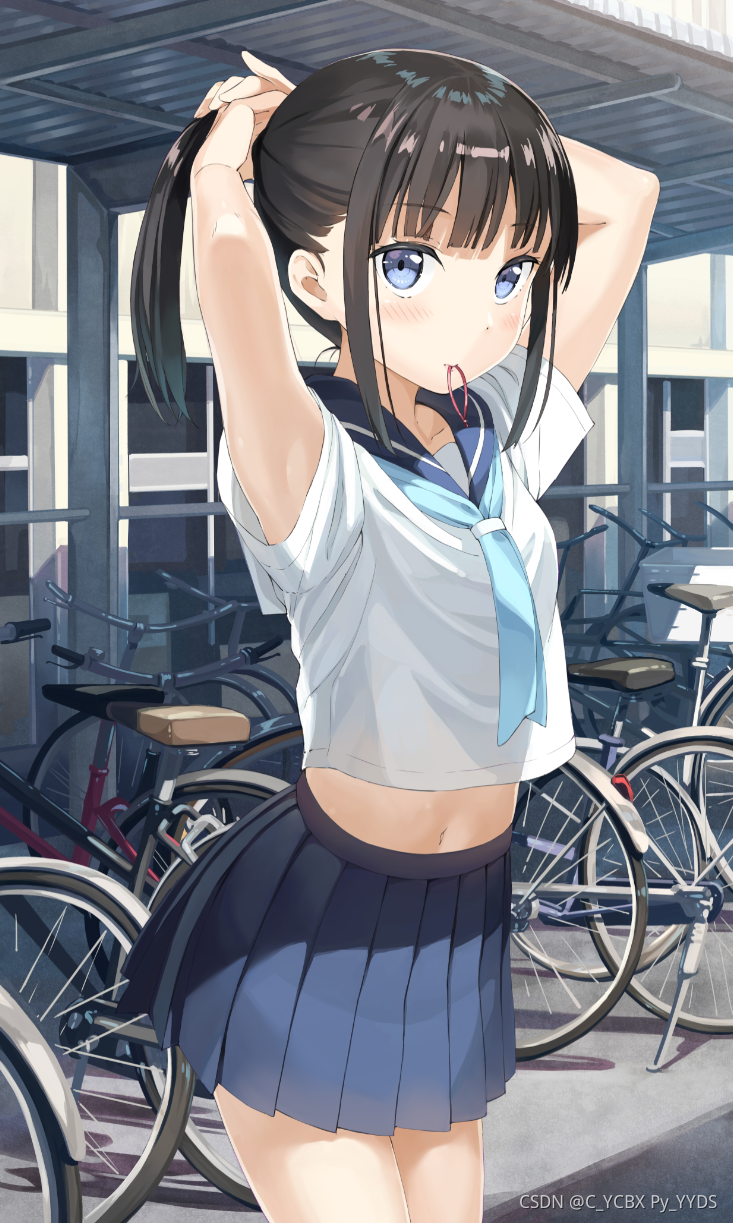
题目
题目翻译
单词积累
sign in 登入
sign out 登出
题目描述
At the beginning of every day,
the first person who signs in the computer room will unlock the door,
and the last one who signs out will lock the door.
Given the records of signing in's and out's,
you are supposed to find the ones who have unlocked and locked the door on that day.
# 在每一天开始后,登录计算机机房的第一个人将会打开这个门,
# 然后登出的最后一个人将会锁上这个门,
# 给定登入和登出的秒的记录,
# 你一应该找出这一天中谁打开和关闭了这个门。
题目整体翻译
Input Specification:
每个输入文件包含一个测试用例。每个用例里都有一天的记录。这种情况以正整数M开始,M是记录的总数,后面是M行,每一行都是这种格式:ID_number Sign_in_time Sign_out_time
其中时间以 HH:MM:SS 格式给出,ID_number 是一个不超过 15 个字符的字符串。
Output Specification:
对于每个测试用例,在一行中输出当天锁门和开锁人员的ID号。两个ID号之间必须用一个空格隔开。
注:保证记录一致。也就是说,每个人的登入时间必须早于登出时间,并且不能有两个人同时登入或登出。
解题思路
我算是发现了,PAT甲级的题目就是用来练输入输出模拟题熟练度的,挺简单的。就是存储一对映射对其进行排序就行。
解题代码
效率还行
- 对仿函数的定义(用于定义堆的排序方式)
struct tmp1
{
bool operator()(const pair<string,int>& a,const pair<string,int>& b) const
{
return a.second < b.second; //大顶堆
}
};
struct tmp2
{
bool operator()(const pair<string,int>& a,const pair<string,int>& b) const
{
return a.second > b.second; //小顶堆
}
};
- solve函数将时间统一转为秒钟计算
int solve(string& s){
int sz = s.size();
int state = 0;
int sum = 0;
for(int i=0;i<sz;i++){
string t = "";
while(isdigit(s[i])&&i<sz){
t += s[i++];
}
if(state==0){
sum += atoi(t.c_str())*3600;
}else if(state==1){
sum += atoi(t.c_str())*60;
}else if(state==2){
sum += atoi(t.c_str());
}
state++;
}
return sum;
}
- Input函数的实现
void Input(){
ios::sync_with_stdio(false);
int n;cin>>n;
while(n--){
string s,st,ed;
cin>>s>>st>>ed;
int start = solve(st),end = solve(ed);
Q1.push(make_pair(s,end));
Q2.push(make_pair(s,start));
}
}
- Output函数的实现
void Output(){
pair<string,int> lock = Q1.top();
pair<string,int> unlock = Q2.top();
cout<<unlock.first<<' '<<lock.first;
}
整体实现
#include<bits/stdc++.h>
using namespace std;
struct tmp1
{
bool operator()(const pair<string,int>& a,const pair<string,int>& b) const
{
return a.second < b.second; //大顶堆
}
};
struct tmp2
{
bool operator()(const pair<string,int>& a,const pair<string,int>& b) const
{
return a.second > b.second; //小顶堆
}
};
priority_queue<pair<string,int>,vector<pair<string,int> >,tmp1>Q1;
priority_queue<pair<string,int>,vector<pair<string,int> >,tmp2>Q2;
int solve(string& s){
int sz = s.size();
int state = 0;
int sum = 0;
for(int i=0;i<sz;i++){
string t = "";
while(isdigit(s[i])&&i<sz){
t += s[i++];
}
if(state==0){
sum += atoi(t.c_str())*3600;
}else if(state==1){
sum += atoi(t.c_str())*60;
}else if(state==2){
sum += atoi(t.c_str());
}
state++;
}
return sum;
}
void Input(){
ios::sync_with_stdio(false);
int n;cin>>n;
while(n--){
string s,st,ed;
cin>>s>>st>>ed;
int start = solve(st),end = solve(ed);
Q1.push(make_pair(s,end));
Q2.push(make_pair(s,start));
}
}
void Output(){
pair<string,int> lock = Q1.top();
pair<string,int> unlock = Q2.top();
cout<<unlock.first<<' '<<lock.first;
}
int main(){
Input();
Output();
return 0;
}