#include <fstream>
#include <iostream>
#include <string>
#define FILENAME "empFile.txet"
using namespace std;
//--------------------------------------
class Worker
{
public:
virtual void ShowInfo() = 0;
virtual string getDeptName() = 0;
int m_id; //职工编号
string m_name; //职工姓名
int m_Deptid; //职工所在部门编号
};
//--------------------------------------
class Boss : public Worker
{
public:
Boss(int id, string name, int dId);
void ShowInfo(); //显示个人信息
string getDeptName();
};
//--------------------------------------
class Employee : public Worker
{
public:
Employee(int id, string name, int dId);
void ShowInfo(); //显示个人信息
string getDeptName();
};
//--------------------------------------
class Manager : public Worker
{
public:
Manager(int id, string name, int dId);
void ShowInfo(); //显示个人信息
string getDeptName();
};
//--------------------------------------
class Bosswife : public Worker
{
public:
Bosswife(int id, string name, int dId);
void ShowInfo(); //显示个人信息
string getDeptName();
};
//--------------------------------------
class WorkManager
{
public:
WorkManager(); //构造函数
~WorkManager(); //析构函数
void ShowMenu(); //展示菜单
void ExitSystem(); //退出管理系统
int m_EmpNum; //记录职工人数
Worker **m_EmpArray; //职工数组指针
void Add_Emp(); //添加员工
void save(); //保存数据
int m_FileIsEmpty;
int get_EmpNum(); //获取职工人数
void init_Emp(); //初始化
void Show_Emp(); //显示职工
int IsExist(string name); //判断是否存在
void Del_Emp(); //删除员工
void Mod_Emp(); //修改员工
void Find_Emp(); //查找员工
void Sort_Emp(); //按编号排序
void Clean_File(); //清空
};
//--------------------------------------
//--------------------------------------
//--------------------------------------
//--------------------------------------
//--------------------------------------
//--------------------------------------
//--------------------------------------
void WorkManager::Clean_File()
{
cout << "确认清空吗? \n"
<< "1.确认 \n"
<< "2.取消 \n";
int select;
cin >> select;
if (select == 1)
{
ofstream ofs(FILENAME, ios::trunc);
ofs.close();
if (this->m_EmpArray != NULL)
{
for (int i = 0; i < m_EmpNum; i++)
{
if (this->m_EmpArray[i] != NULL)
{
delete this->m_EmpArray[i];
}
}
this->m_EmpNum = 0;
delete[] this->m_EmpArray;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = 0;
}
cout << "清空成功\n";
this->save();
system("pause");
system("cls");
}
else
{
cout << "您取消了操作\n";
system("pause");
system("cls");
}
}
void WorkManager::Sort_Emp()
{
if (this->m_FileIsEmpty == 0)
{
cout << "文件为空或不存在\n";
system("pause");
system("cls");
}
else
{
cout << "请选择排序方式: \n"
<< "1.按职工号升序 \n"
<< "2.按职工号降序 \n";
int select = 0;
cin >> select;
for (int i = 0; i < m_EmpNum; i++)
{
int min = i;
if (select == 1)
{
for (int j = i; j < m_EmpNum; j++)
{
if (m_EmpArray[min]->m_id > m_EmpArray[j]->m_id)
{
min = j;
}
}
}
else
{
if (select == 2)
{
for (int j = i; j < m_EmpNum; j++)
{
if (m_EmpArray[min]->m_id < m_EmpArray[j]->m_id)
{
min = j;
}
}
}
}
if (i != min)
{
Worker *temp = m_EmpArray[i];
m_EmpArray[i] = m_EmpArray[min];
m_EmpArray[min] = temp;
}
}
cout << "排序后的结果为:\n";
for (int i = 0; i < this->m_EmpNum; i++)
{
this->m_EmpArray[i]->ShowInfo();
}
int choice = 0;
cout << "是否要对排序后的结果保存?是的话输入1否则输入0\n";
cin >> choice;
if (choice == 1)
{
this->save();
cout << "保存成功\n";
system("pause");
system("cls");
}
else
{
cout << "您取消了保存";
system("pause");
system("cls");
}
}
}
void WorkManager::Find_Emp()
{
if (this->m_FileIsEmpty == 0)
{
cout << "文件为空或不存在\n";
system("pause");
system("cls");
}
else
{
string name;
cout << "请输入要查找的职工姓名: ";
cin >> name;
int ret = this->IsExist(name);
if (ret != -1)
{
this->m_EmpArray[ret]->ShowInfo();
}
else
{
cout << "查无此人\n";
}
}
system("pause");
system("cls");
}
void WorkManager::Mod_Emp()
{
if (this->m_FileIsEmpty == 0)
{
cout << "文件为空或不存在\n";
system("pause");
system("cls");
}
else
{
cout << "请输入要修改的职工姓名: ";
string name;
cin >> name;
int ret = this->IsExist(name);
if (ret != -1)
{
int newid;
int newselect;
int newdid;
cout << "请输入新的职工编号:\n";
cin >> newid;
cout << "请选择员工岗位" << endl;
cout << "1.普通职工" << endl;
cout << "2.经理" << endl;
cout << "3.老板" << endl;
cout << "4.老板娘" << endl;
cin >> newselect;
Worker *worker = NULL;
switch (newselect)
{
case 1:
delete this->m_EmpArray[ret];
worker = new Employee(newid, name, 1);
this->m_EmpArray[ret] = worker;
cout << "修改成功\n";
break;
case 2:
delete this->m_EmpArray[ret];
worker = new Manager(newid, name, 2);
this->m_EmpArray[ret] = worker;
cout << "修改成功\n";
break;
case 3:
cout << "想取代我当老板?做梦吧你";
break;
case 4:
cout << "想修改老板娘?傻鸟玩意";
break;
default:
break;
}
this->save();
}
else
{
cout << "修改失败,未找到该员工\n";
}
}
system("pause");
system("cls");
}
void WorkManager::Del_Emp()
{
cout << "请输入想要删除的职工姓名: ";
string name;
cin >> name;
int index = this->IsExist(name);
if (index != -1)
{
if (this->m_EmpArray[index]->m_Deptid == 3)
{
cout << "傻鸟把老板删了还开你妈的公司\n";
}
if (this->m_EmpArray[index]->m_Deptid == 4)
{
cout << "傻鸟把老板娘删了还开你妈的公司\n";
}
else if (index != -1 && index != 0 && index != 1)
{
for (int i = index; i < this->m_EmpNum - 1; i++)
{
this->m_EmpArray[i] = this->m_EmpArray[i + 1];
}
this->m_EmpNum--;
this->save();
cout << "删除成功\n";
}
}
else
{
cout << "删除失败,未找到该员工\n";
}
system("pause");
system("cls");
}
int WorkManager::IsExist(string name)
{
int index = -1;
for (int i = 0; i < this->m_EmpNum; i++)
{
if (this->m_EmpArray[i]->m_name == name)
{
index = i;
break;
}
}
return index;
}
void WorkManager::Show_Emp()
{
if (this->m_FileIsEmpty == 0)
{
cout << "文件为空或不存在\n";
system("pause");
system("cls");
}
else
{
for (int i = 0; i < this->m_EmpNum; i++)
{
this->m_EmpArray[i]->ShowInfo();
}
system("pause");
system("cls");
}
}
void WorkManager::init_Emp()
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id;
string name;
int dId;
int index = 0;
while (ifs >> id && ifs >> name && ifs >> dId)
{
Worker *worker = NULL;
if (dId == 1)
{
worker = new Employee(id, name, dId);
}
else if (dId == 2)
{
worker = new Manager(id, name, dId);
}
else if (dId == 3)
{
worker = new Boss(id, name, dId);
}
else if (dId == 4)
{
worker = new Bosswife(id, name, dId);
}
this->m_EmpArray[index] = worker;
index++;
}
ifs.close();
}
int WorkManager::get_EmpNum()
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id;
string name;
int dId;
int num = 0;
while (ifs >> id && ifs >> name && ifs >> dId)
{
num++;
}
ifs.close();
return num;
}
//--------------------------------------
void WorkManager::save()
{
ofstream ofs;
ofs.open(FILENAME, ios::out);
for (int i = 0; i < this->m_EmpNum; i++)
{
ofs << this->m_EmpArray[i]->m_id << " "
<< this->m_EmpArray[i]->m_name << " "
<< this->m_EmpArray[i]->m_Deptid << " ";
}
ofs.close();
}
//--------------------------------------
WorkManager::WorkManager()
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
if (!ifs.is_open())
{
// cout << "文件不存在\n";
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = 0;
ifs.close();
return;
}
char ch;
ifs >> ch;
if (ifs.eof())
{
// cout << "文件为空\n";
this->m_EmpNum = 0;
this->m_EmpArray = NULL;
this->m_FileIsEmpty = 0;
ifs.close();
return;
}
this->m_FileIsEmpty = 1;
this->m_EmpNum = this->get_EmpNum();
// cout << "职工人数为" << this->get_EmpNum() << endl;
this->m_EmpArray = new Worker *[this->m_EmpNum];
this->init_Emp();
return;
/* for (int i = 0; i < this->m_EmpNum; i++)
{
cout << "职工编号: " << this->m_EmpArray[i]->m_id << " "
<< "职工姓名: " << this->m_EmpArray[i]->m_name << " "
<< "职工部门: " << this->m_EmpArray[i]->m_Deptid << endl;
}*/
}
//--------------------------------------
void WorkManager::Add_Emp()
{
cout << "请输入添加职工数量" << endl;
int addNum = 0; //保存用户的输入数量
cin >> addNum;
if (addNum > 0)
{
//计算新添加空间大小
int newSize = this->m_EmpNum + addNum;
Worker **newspace = new Worker *[newSize];
if (this->m_EmpArray != NULL)
{
for (int i = 0; i < this->m_EmpNum; i++)
{
newspace[i] = this->m_EmpArray[i];
}
}
for (int i = 0; i < addNum; i++)
{
int id;
string name;
int dslect;
cout << "请输入第 " << i + 1 << "个新员工编号" << endl;
cin >> id;
cout << "请输入第 " << i + 1 << "个新员工名字" << endl;
cin >> name;
cout << "请选择员工岗位" << endl;
cout << "1.普通职工" << endl;
cout << "2.经理" << endl;
cout << "3.老板" << endl;
cout << "4.老板娘" << endl;
cin >> dslect;
Worker *worker = NULL;
switch (dslect)
{
case 1:
worker = new Employee(id, name, 1);
break;
case 2:
worker = new Manager(id, name, 2);
break;
case 3:
worker = new Boss(id, name, 3);
break;
case 4:
worker = new Bosswife(id, name, 4);
break;
default:
break;
}
newspace[this->m_EmpNum + i] = worker;
}
delete[] this->m_EmpArray;
this->m_EmpArray = newspace;
this->m_EmpNum = newSize;
cout << "成功添加" << addNum << "名员工" << endl;
this->save();
m_FileIsEmpty = 1;
system("pause");
system("cls");
}
else
{
cout << "输入数据有误" << endl;
}
}
//--------------------------------------
WorkManager::~WorkManager()
{
if (this->m_EmpArray != NULL)
{
for (int i = 0; i < m_EmpNum; i++)
{
if (this->m_EmpArray[i] != NULL)
{
delete this->m_EmpArray[i];
}
}
delete[] this->m_EmpArray;
this->m_EmpArray = NULL;
}
}
//--------------------------------------
void WorkManager::ShowMenu()
{
cout << "*************************************" << endl;
cout << "*******欢迎使用职工管理系统!********" << endl;
cout << "********** 0.退出管理程序************" << endl;
cout << "********** 1.增加职工信息************" << endl;
cout << "********** 2.显示职工信息************" << endl;
cout << "********** 3.删除离职员工************" << endl;
cout << "********** 4.修改职工信息************" << endl;
cout << "********** 5.查找职工信息************" << endl;
cout << "********** 6.按照编号排序************" << endl;
cout << "********** 7.清空所有文档************" << endl;
cout << "*************************************" << endl;
cout << endl;
}
//--------------------------------------
void WorkManager::ExitSystem()
{
cout << "欢迎下次使用!" << endl;
exit(0);
}
//--------------------------------------
Employee::Employee(int id, string name, int dId)
{
this->m_id = id;
this->m_name = name;
this->m_Deptid = dId;
}
//--------------------------------------
void Employee::ShowInfo()
{
cout << "职工编号: " << this->m_id;
cout << " \t职工姓名: " << this->m_name;
cout << " \t职工岗位: " << this->getDeptName();
cout << " 岗位职责:完成经理交给的任务" << endl;
}
//--------------------------------------
string Employee::getDeptName()
{
return "员工";
}
//--------------------------------------
Manager ::Manager(int id, string name, int dId)
{
this->m_id = id;
this->m_name = name;
this->m_Deptid = dId;
}
//--------------------------------------
void Manager ::ShowInfo()
{
cout << "职工编号: " << this->m_id;
cout << " \t职工姓名: " << this->m_name;
cout << " \t职工岗位: " << this->getDeptName();
cout << " 岗位职责:完成老板交给的任务,下发任务给员工" << endl;
}
//--------------------------------------
string Manager ::getDeptName()
{
return "经理";
}
//--------------------------------------
Boss ::Boss(int id, string name, int dId)
{
this->m_id = id;
this->m_name = name;
this->m_Deptid = dId;
}
//--------------------------------------
void Boss ::ShowInfo()
{
cout << "职工编号: " << this->m_id;
cout << " \t职工姓名: " << this->m_name;
cout << " \t职工岗位: " << this->getDeptName();
cout << " 岗位职责:管理公司所有事务" << endl;
}
//--------------------------------------
string Boss ::getDeptName()
{
return "老板";
}
//--------------------------------------
Bosswife ::Bosswife(int id, string name, int dId)
{
this->m_id = id;
this->m_name = name;
this->m_Deptid = dId;
}
//--------------------------------------
void Bosswife ::ShowInfo()
{
cout << "职工编号: " << this->m_id;
cout << " \t职工姓名: " << this->m_name;
cout << " \t职工岗位: " << this->getDeptName();
cout << " 岗位职责:整天吃喝玩乐" << endl;
}
//--------------------------------------
string Bosswife ::getDeptName()
{
return "老板娘";
}
//--------------------------------------
int main()
{
WorkManager wm;
int choice;
while (1)
{
wm.ShowMenu();
cout << "请输入你的选择: " << endl;
cin >> choice;
switch (choice)
{
case 0:
wm.ExitSystem();
break;
case 1:
wm.Add_Emp();
break;
case 2:
wm.Show_Emp();
break;
case 3:
wm.Del_Emp();
break;
case 4:
wm.Mod_Emp();
break;
case 5:
wm.Find_Emp();
break;
case 6:
wm.Sort_Emp();
break;
case 7:
wm.Clean_File();
break;
default:
cout << "输入错误\n";
system("cls");
break;
}
}
return 0;
}
自己之前写的一个职工管理系统
最新推荐文章于 2022-07-13 10:19:55 发布
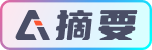