功能说明:
用户有信用卡和储蓄卡,储蓄卡有查询余额和取款功能,信用卡能够查看账单金额、查看还款日和查看余额三个功能;
使用委托与事件实现下列功能:用户可自由设置信用卡还款关联的储蓄卡,当还款日到期时进行账单金额的自动划扣;
(PS)本代码为了专注于实现关键功能,固定了信用卡有三张,储蓄卡有两张,如果需要拓展,更全面的模拟业务可以写些增加卡片的逻辑
设计流程:
根据题目要求,我们知道此题关键点是利用事件与委托的概念来实现信用卡扣款功能
结合我所了解的信用卡还款流程,主要执行还款流程逻辑结构如下:
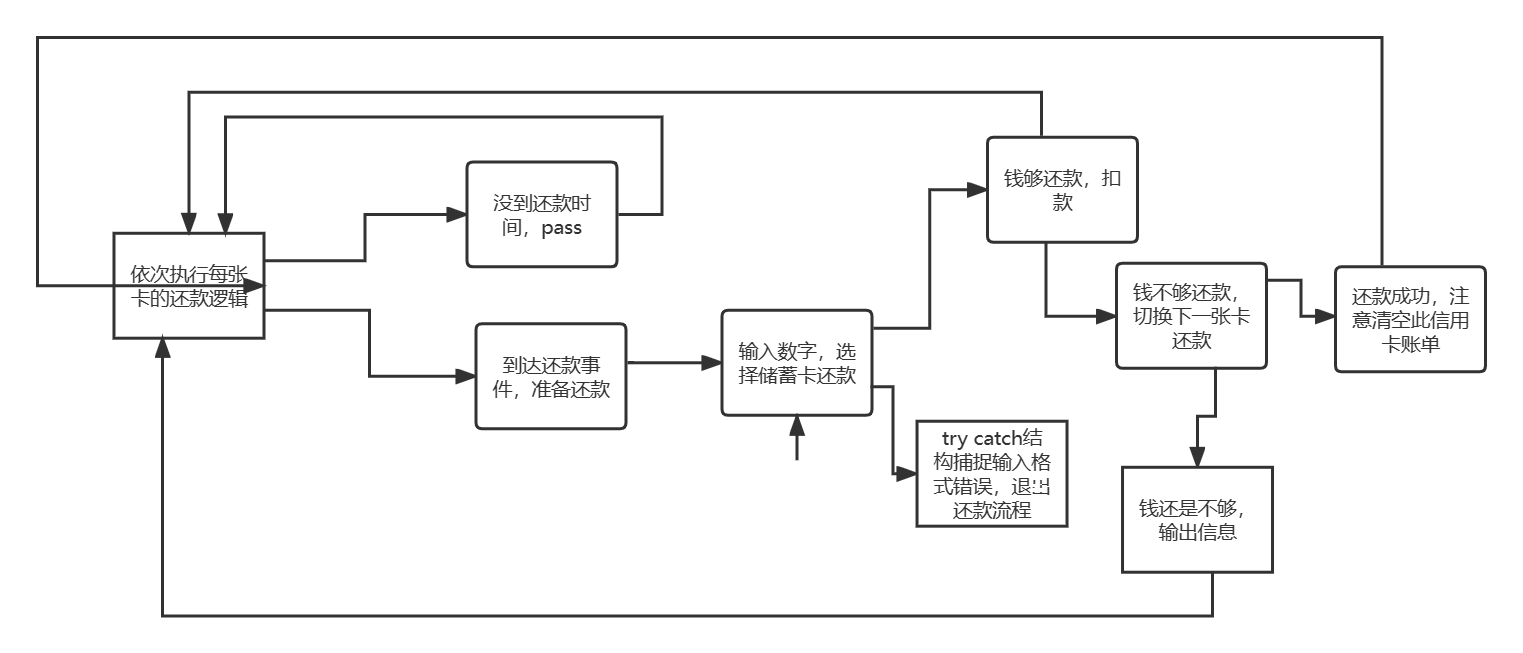
关键源码展示:
储蓄卡类:
class DepositCard
{
public int amount;
public void Display()
{
Console.WriteLine("储蓄卡余额为:{0}", amount);
}
public void Account(int balance, int payday)
{
amount += balance;
Console.WriteLine("今天是本月的{0}号,取款{1},储蓄卡余额为:{2}。",DateTime.Today.Day, balance, amount);
}
}
信用卡类:
class CreditCard
{
private int billamount;
private int repaymentday;
public CreditCard(int billamount, int repaymentday)
{
this.billamount = billamount;
this.repaymentday = repaymentday;
}
public int getbillamount() { return billamount; }
public int getrepaymentday() { return repaymentday; }
public void Display() { Console.WriteLine("信用卡余额为:{0}", billamount); }
public void havePayed()
{
billamount = 0;
}
}
委托类(事件也定义在此类内部)
class CreditCardDelegate
{
public int billamount;
public int repaymentday;
//请在此处添加自己的代码
public CreditCardDelegate(int billamount, int repaymentday)
{
this.billamount = billamount;
this.repaymentday = repaymentday;
}
public delegate void cardDelegate(int billmount,int repaymentday);
public event cardDelegate notifyRepayment = delegate { };
public void notify()
{
if(notifyRepayment != null)
{
notifyRepayment(billamount,repaymentday);
}
}
}
主函数(相关逻辑代码实现关键部分):
static void Main(string[] args)
{
DepositCard depositCard = new DepositCard();
depositCard.amount = 10000;
DepositCard depositCard1 = new DepositCard();
depositCard1.amount = 2000;
List<DepositCard> depositCards = new List<DepositCard>();
depositCards.Add(depositCard); depositCards.Add(depositCard1);
CreditCard creditCard1 = new CreditCard(-3000, 5);
CreditCard creditCard2 = new CreditCard(-3000, 13);
CreditCard creditCard3 = new CreditCard(-5000, 29);
depositCard.Display();
depositCard1.Display(); Console.WriteLine("");
List<CreditCard> Cards = new List<CreditCard>();
Cards.Add(creditCard1); Cards.Add(creditCard2); Cards.Add(creditCard3);
Console.WriteLine("在您的账户下找到了{0}张储蓄卡,{1}张信用卡", depositCards.Count, Cards.Count);
foreach (CreditCard card in Cards)
{
CreditCardDelegate creditCardDelegate = new CreditCardDelegate(card.getbillamount(), card.getrepaymentday());
Console.WriteLine("");
Console.WriteLine("信用卡开始执行委托还款。。。。。。");
//请在此处添加自己的代码
if (DateTime.Today.Day == card.getrepaymentday())
{
Console.WriteLine("此张信用卡今天已需还款,还款金额为{0},请按‘1’或者‘2’在您的两张储蓄卡中选择一张卡还款", card.getbillamount());
try
{
int number = Convert.ToInt32(Console.ReadLine());
if (number == 1)
{
if (depositCard.amount + card.getbillamount() >= 0)
{
creditCardDelegate.notifyRepayment += new CreditCardDelegate.cardDelegate(depositCard.Account);
creditCardDelegate.notify();
card.havePayed();
Console.WriteLine("付款成功,欢迎下次光临~");
}
else
{
Console.WriteLine("这张卡钱不够您还款,你怎么这么能花,自动选择另外一张卡为您还款");
if (depositCard1.amount + card.getbillamount() >= 0)
{
creditCardDelegate.notifyRepayment += new CreditCardDelegate.cardDelegate(depositCard1.Account);
creditCardDelegate.notify();
card.havePayed();
Console.WriteLine("付款成功,欢迎下次光临~");
}
else
{
Console.WriteLine("这张卡钱也不够您还款,您的信息已被银行记录");
}
}
}
else if (number == 2)
{
if (depositCard1.amount + card.getbillamount() >= 0)
{
creditCardDelegate.notifyRepayment += new CreditCardDelegate.cardDelegate(depositCard1.Account);
creditCardDelegate.notify();
card.havePayed();
Console.WriteLine("付款成功,欢迎下次光临~");
}
else
{
Console.WriteLine("这张卡钱不够您还款,你怎么这么能花,自动选择另外一张卡为您还款");
if (depositCard.amount + card.getbillamount() >= 0)
{
creditCardDelegate.notifyRepayment += new CreditCardDelegate.cardDelegate(depositCard.Account);
creditCardDelegate.notify();
card.havePayed();
Console.WriteLine("付款成功,欢迎下次光临~");
}
else
{
Console.WriteLine("这张卡钱也不够您还款,您的信息已被银行记录");
}
}
}
}
catch (Exception e) { Console.WriteLine(e + "输入错误,请配合程序工作,不要瞎输"); }
}
else
{
Console.WriteLine("yes!,此卡未到您的还款日,下面为您显示此卡账单:");
card.Display();
}
}
Console.WriteLine("");
Console.WriteLine("信用卡还款流程结束,下面是您的{0}张卡现在余额,祝您生活愉快~", depositCards.Count);
depositCard.Display();
depositCard1.Display(); Console.WriteLine("");
}
总结:
关键代码讲解:
creditCardDelegate.notifyRepayment += new CreditCardDelegate.cardDelegate(depositCard.Account);
creditCardDelegate.notify();
如上两行代码,实现了委托类对象的事件notifyRepayment绑定委托类中定义的委托cardDelegate,同时传递了储蓄卡类的Account方法,然后调用了委托类对象的notify()函数
public void notify()
{
if(notifyRepayment != null)
{
notifyRepayment(billamount,repaymentday);
}
}
what it mean?
一种理解方式:
事件绑定了委托,委托绑定了函数,委托可以切换绑定多个形参与返回值一样的函数,
事件是拥有可以注册和解绑方法(函数)的功能
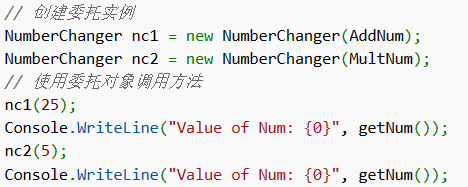
一旦满足条件,咱们可以启动事件,启动相关函数,此题我只不过把启动事件又封装在了notify()函数
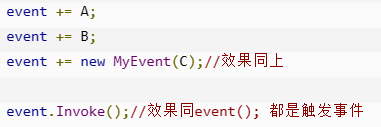
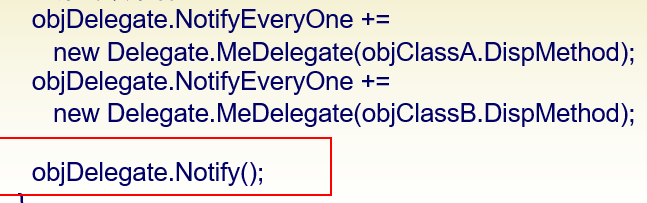
其他的话:
其实委托和事件还是很绕的,初学者面临的最大问题就是不知道这个要干嘛,这么复杂;不熟练实现相关代码的细节,写不出来函数。针对此问题的建议就是看看别人是如何实现的,认真分析每一行要干嘛,这个代码在干嘛,享受学不会到学会的乐趣。
To exist is to be reasonable
实现效果截图:
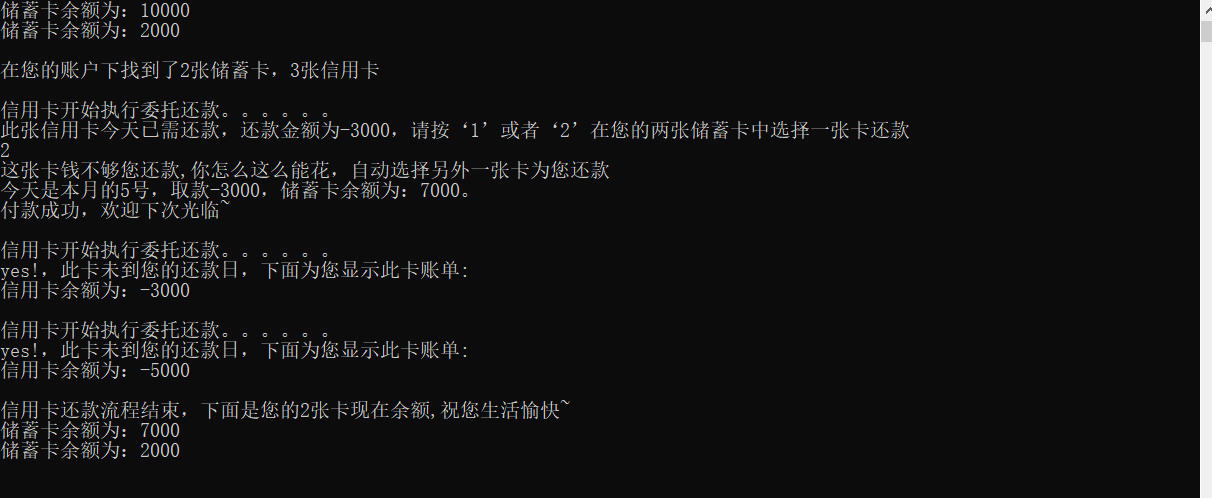
源码开源地址:
部分图源:
runnoob.com以及老师的ppt
希望此篇博客可以帮助到你