一、Xmind整理:
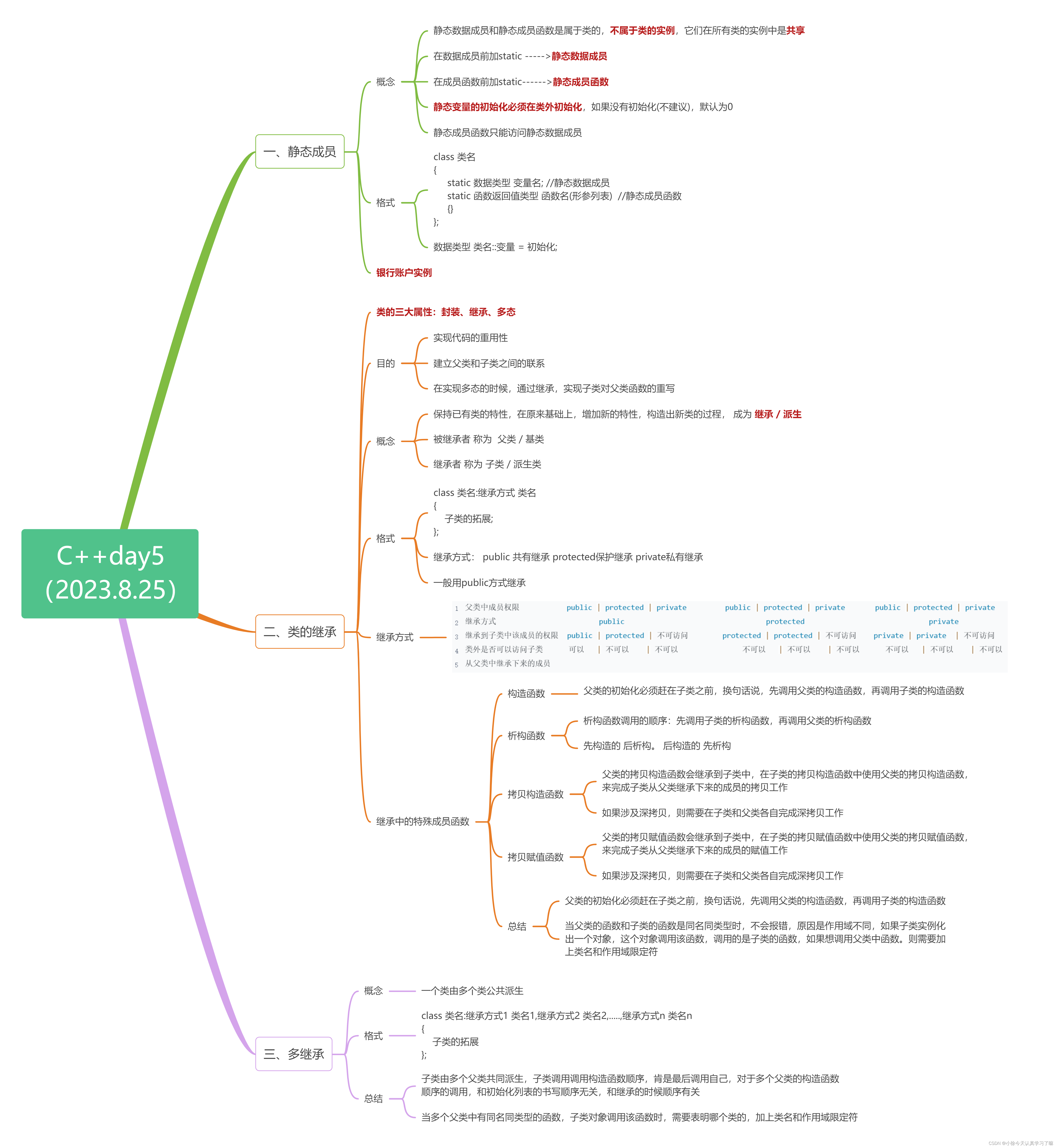
二、上课笔记整理:
1.静态数据成员+静态成员函数(银行账户实例)
#include <iostream>
using namespace std;
class BankAccount
{
private:
double balance; //余额
static double interest_rate; //利率
public:
//无参构造函数
BankAccount ()
{
}
//有参构造函数
BankAccount(double balance):balance(balance)
{
}
//静态成员函数,获取当前利率
static double getInterestRate()
{
return interest_rate;
}
//静态成员函数,设置当前利率、
static double setInterestRate(double rate)
{
interest_rate = rate;
}
//静态成员函数,获取连本带利的全额
static double getLastMoney(BankAccount &account)
{
return account.balance*(1+interest_rate);
}
};
double BankAccount::interest_rate = 0.05;
int main()
{
cout << "当前利率:" << BankAccount::getInterestRate() << endl;
BankAccount::setInterestRate(0.03);
cout << "当前利率:" << BankAccount::getInterestRate() << endl;
BankAccount account1(2000.0);
BankAccount account2(3000.0);
cout << "第一个人连本带利的余额为:" << BankAccount::getLastMoney(account1) << endl;
return 0;
}
2.类的继承
#include <iostream>
using namespace std;
//封装 人 类 父类/基类
class Person
{
private:
string name;
protected:
int age;
public:
int h;
public:
//无参构造函数
Person()
{
cout << "父类的无参构造函数" << endl;
}
//有参构造
Person(string name, int age, int h):name(name),age(age),h(h)
{
cout << "父类的有参构造函数" << endl;
}
//拷贝构造函数
Person(const Person & other):name(other.name),age(other.age),h(other.h)
{
cout << "父类的拷贝构造函数" << endl;
}
//拷贝赋值函数
Person & operator=(const Person &p)
{
name = p.name;
age = p.age;
h = p.h;
cout << "父类的拷贝赋值函数" << endl;
return *this;
}
void show()
{
cout << "父类的show" << endl;
}
};
//封装 学生 类 共有继承人 类
class Stu:public Person //子类 、派生类
{
private:
int id;
int math;
public:
//无参构造函数
Stu()
{
cout << "子类的无参构造函数" << endl;
}
//有参构造函数
Stu(string name, int age, int h, int id, int math):Person(name,age,h),id(id),math(math)
{
cout << "子类的有参构造函数" << endl;
}
//拷贝构造函数
Stu(const Stu & s):id(s.id),math(s.math),Person(s)
{
cout << "子类的拷贝构造函数" << endl;
}
//拷贝赋值函数
Stu & operator=(const Stu & s)
{
Person::operator=(s);
id = s.id;
math = s.math;
cout << "子类的拷贝赋值函数" << endl;
return *this;
}
void show()
{
cout << "子类的show" << endl;
cout << h << endl; //通过共有继承,类外、子类可以访问父类共有成员
cout << age << endl; //通过共有继承,子类可以访问父类保护成员,类外不可以访问
//cout << name << endl;//通过共有继承,子类不可访问父类私有成员,类外不可以访问
}
};
int main()
{
Stu s("zhangsan",12,190,1001,78);
Stu s2=s;
Stu s3;
s3 = s2;
// s.show();
// s.Person::show();
return 0;
}
3.多继承
#include <iostream>
using namespace std;
class Sofa
{
private:
string sitting;
public:
//无参构造函数
Sofa()
{
cout << "沙发的无参构造" << endl;
}
//有参构造函数
Sofa(string s):sitting(s)
{
cout << "沙发的有参构造" << endl;
}
void display()
{
cout << sitting << endl;
}
};
class Bed
{
private:
string sleep;
public:
//无参构造函数
Bed()
{
cout << "床的无参构造" << endl;
}
//有参构造函数
Bed(string(s)):sleep(s)
{
cout << "床的有参构造" << endl;
}
void display()
{
cout << sleep << endl;
}
};
class Sofa_Bed:public Bed,public Sofa
{
private:
int w;
public:
//无参构造函数
Sofa_Bed()
{
cout << "沙发床的无参构造" << endl;
}
//有参构造函数
Sofa_Bed(string sit, string s, int w):Bed(s),Sofa(sit),w(w)
{
cout << "沙发床的有参构造" << endl;
}
};
int main()
{
Sofa_Bed(s);
Sofa_Bed s1("可坐","可躺",123);
s1.Sofa::display();
s1.Bed::display();
return 0;
}