# -*- coding: utf-8 -*-
import os
import fitz
from PIL import Image
import datetime
# 遍历文件夹,不包括子文件夹
def ergodic(imagePath, type):
#print('文件夹' + imagePath + '的' + type + '文件将进行转换')
file_page = []
for fi in os.listdir(imagePath):
if fi.endswith(type):
file_page.append(fi)
return file_page
# 检查图片方向并旋转
def img2rotate(png_r):
#print('\n检查图片方向并旋转')
im_1 = Image.open(png_r)
a, b = im_1.size
if a < b:
im_1 = im_1.rotate(270, expand=1) # 旋转角度为270,压缩率为1
im_1.save(png_r)
# im_1.close()
conver_png(png_r) # 修改尺寸
# 修改图片尺寸
def conver_png(jpgfile):
#print('修改' + jpgfile + '宽度为2480')
width_new = 2480 # 确定宽度
im = Image.open(jpgfile)
width, height = im.size
height_new = round(width_new * height / width) # 修改高度为整数
new_im = im.resize((width_new, height_new))
new_im.save(jpgfile, quality=80) # 替换原文件
cheek_size(jpgfile)
# im.close()
# new_im.save(os.getcwd() + '\\' + jpgfile) # 替换原文件
def cheek_size(png):
im = Image.open(png)
width, height = im.size
if height > 1755:
height_new = 1755
width_new = round(height_new * width / height) # 修改高度为整数
new_im = im.resize((width_new, height_new))
new_im.save(png, quality=100)
# pdf改为jpg输入文件
def img2png(path):
os.chdir(path) # 修改文件路径
format_pdf = '.pdf'
file = ergodic(path, format_pdf)
if len(file) > 0:
#print('\n开始转换')
for fname in file:
doc = fitz.open(fname) # open document
for page in doc: # iterate through the pages
pix = page.get_pixmap(dpi=300)
pix.save(fname + "%i.png" % page.number) # store image as a PNG
#print(fname + "%i.png" % page.number)
doc.close()
# os.remove(fname)#移除PDF文件
png2jpg(path)
else:
png2jpg(path)
def png2jpg(path):
os.chdir(path) # 修改文件路径
format_png = '.png'
file = ergodic(path, format_png)
if len(file) > 0:
#print('\n开始将PNG转换为JPG')
for fname in file:
im = Image.open(fname)
im = im.convert('RGB')
im.save(fname[:-4] + '.jpg')
os.remove(fname) #移除PNG文件
jpg2jpg(path)
else:
jpg2jpg(path)
def jpg2jpg(path):
os.chdir(path) # 修改文件路径
format_jpg = '.jpg'
file = ergodic(path, format_jpg)
if len(file) > 0:
#print('\n开始将JPG进行压缩')
for fname in file:
img2rotate(fname)
else:
return
# 重命名
def jpgrename(path):
os.chdir(path) # 修改文件路径
format_jpg = '.jpg'
file = ergodic(path, format_jpg)
#print(file)
i = file[0][0:-5]
for ra in range(0, 10):
try: #跳过报错
a = '{}{}.jpg'.format(i, ra)
#print(a)
b = '{}0{}.jpg'.format(i, ra)
#print(b)
os.rename('{}'.format(a), '{}'.format(b))
except:
continue
# pdf文件的def
def img2to1pdf(path): # 将N张JPG合成一张PNF
os.chdir(path) # 修改文件路径
format_jpg = '.jpg'
file = ergodic(path, format_jpg)
if len(file) > 0:
#print('\n开始合并PDF')
step = 2 # 设定张数为2
im_group = [file[i:i + step] for i in range(0, len(file), step)] # N张一组
# print(im_group)
img_list = [] # 一张PDF包含的值
for i in im_group:
img_a4 = Image.new(mode="RGB", size=(2480, 3509), color="white") # 纵向空白png,SIZE尺寸
x = 0
for ea_1 in i: # 读取图片信息
op = Image.open(ea_1)
img_a4.paste(op, (0, x))
x = x + 1755
img_list.append(img_a4)
# print(img_list)
img_pdf = img_list[0]
img_list = img_list[1:]
today = datetime.date.today()
img_pdf.save('{} 合并的PDF.pdf'.format(today), "PDF", resolution=300.0, save_all=True, append_images=img_list)
print('\n任务结束')
# for fname in file:
# os.remove(fname) #移除JPG文件
else:
return
if __name__ == "__main__":
imagePath = input("输入目标路径:(若为当前路径:" + os.getcwd() + ",请直接回车)\n")
if (imagePath == ""):
imagePath = os.getcwd()
print('格式装换')
img2png(imagePath)
print('修改完成')
print('对编号10以下的文件进行重命名')
jpgrename(imagePath)
print('已重命名')
print('开始合并')
img2to1pdf(imagePath)
print('合并完成')
将多张PDF,每张PDF缩小一半,并合成一张
于 2023-02-28 09:40:52 首次发布
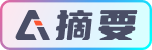