石头剪刀布游戏——Java
package com.lhy.oop3;
import java.util.Scanner;
import static com.bjsxt.oop3.TestCycles.getRandomNumber;
import static com.bjsxt.oop3.TestCycles.judgeGesture;
public class TestCycles {
public static void main(String[] args) {
test();
}
public static void test(){
Mygame mygame = new Mygame();
mygame.initGame();
mygame.startGame();
}
public static int getRandomNumber(int min,int max){
return (int)(Math.random() * (max-min)+min);
}
public static String judgeGesture(int num){
String gesture = "" ;
if(num == Constant.ROCK){
gesture = Constant.getRock(Constant.ROCK);
}
if(num == Constant.SCISSORS){
gesture = Constant.getScissors(Constant.SCISSORS);
}
if(num ==Constant.CLOTH){
gesture =Constant.getCloth(Constant.CLOTH);
}
return gesture;
}
}
class Constant{
public static final int ROCK = 0;
public static final int SCISSORS = 1;
public static final int CLOTH = 2;
int[] arr = {ROCK,SCISSORS,CLOTH};
public static final int GANADOS = 3 ;
public Constant() {
}
public static String getRock(int rock) {
String gesture = " ";
if(rock == Constant.ROCK){
gesture = "石头";
}
return gesture;
}
public static String getScissors(int scissors) {
String gesture = " ";
if(scissors == Constant.SCISSORS){
gesture = "剪刀";
}
return gesture;
}
public static String getCloth(int cloth) {
String gesture = " ";
if(cloth == Constant.CLOTH){
gesture = "布";
}
return gesture;
}
public int getGanados() {
return GANADOS;
}
}
abstract class GamePlayer{
String name;
int value;
int wincount;
abstract int getInputValue();
}
class Player extends GamePlayer{
int playerWincount = 0;
public Player() {
}
public Player(String name) {
this.name = name;
}
@Override
int getInputValue() {
Scanner scanner = new Scanner(System.in);
int playervalue = scanner.nextInt();
return playervalue;
}
@Override
public String toString() {
return "Player{" +
"name='" + name + '\'' +
'}';
}
}
class Computer extends GamePlayer{
int computerWincount = 0;
public Computer() {
}
public Computer(String name) {
this.name = name;
}
@Override
int getInputValue() {
int computervalue = getRandomNumber(0,3);
return computervalue;
}
@Override
public String toString() {
return "Computer{" +
"name='" + name + '\'' +
'}';
}
}
interface GameComparator{
int compare(int playerValue, int computerValue);
}
class GameComparatorImpl implements GameComparator{
@Override
public int compare(int playerValue, int computerValue) {
int result = 3;
if((playerValue == Constant.ROCK&&computerValue == Constant.SCISSORS) ||(playerValue == Constant.SCISSORS &&computerValue == Constant.CLOTH) ||(playerValue == Constant.CLOTH &&computerValue == Constant.ROCK)){
result = 1;
}
if((playerValue == Constant.ROCK&&computerValue == Constant.CLOTH) ||(playerValue == Constant.SCISSORS &&computerValue == Constant.ROCK) ||(playerValue == Constant.CLOTH &&computerValue == Constant.SCISSORS)){
result = -1;
}
if((playerValue == Constant.ROCK&&computerValue == Constant.ROCK) ||(playerValue == Constant.SCISSORS &&computerValue == Constant.SCISSORS) ||(playerValue == Constant.CLOTH &&computerValue == Constant.CLOTH)){
result = 0;
}
return result;
}
}
interface GameInterface{
public abstract void initGame();
public abstract void startGame();
public abstract void endGame();
}
class Mygame implements GameInterface{
int playerWincount = 0;
int computerWincount = 0;
int time = 0;
int judgeWincout( int result) {
if (result == 1) {
System.out.println("您获胜了");
playerWincount++;
}
if (result == -1) {
System.out.println("您输了");
computerWincount++;
}
if(result == 0){
System.out.println("平局");
time++;
}
System.out.println(toString());
return result;
}
@Override
public String toString() {
return "共计玩"+(playerWincount+computerWincount+time)+"场"+
"\n您的胜利场数为"+playerWincount+
"\n电脑玩家的胜利场数为:"+computerWincount+
"\n平局场数为:"+time;
}
@Override
public void initGame() {
System.out.println("---欢迎使用猜拳游戏----\n---(5局3胜制)---");
GamePlayer player = new Player("小明");
GamePlayer computer = new Computer("电脑玩家");
System.out.println( player.toString());
System.out.println(computer.toString());
}
@Override
public void startGame() {
Player player = new Player();
Computer computer = new Computer();
Scanner scanner = new Scanner(System.in);
GameComparatorImpl gameComparator = new GameComparatorImpl();
int a =0;
int b = 0;
while(true){
System.out.println("请输入您要出的手势:0代表石头 1代表剪刀 2代表布");
int playervalue= player.getInputValue();
int computervalue = computer.getInputValue();
System.out.println("您选择的手势是"+judgeGesture(playervalue)+
"\n电脑玩家选择的手势是"+judgeGesture(computervalue));
a = gameComparator.compare(playervalue,computervalue);
judgeWincout(a);
if(playerWincount < Constant.GANADOS&&computerWincount < Constant.GANADOS){
}else {
endGame();
break;
}
System.out.println("您是否继续游戏:按3结束,按其他任意数字继续");
b = scanner.nextInt();
if(b == 3){
endGame();
break;
}
}
}
@Override
public void endGame() {
System.out.println("游戏结束");
return;
}
}
```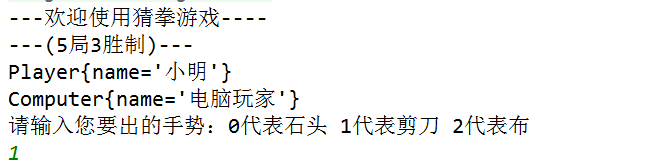
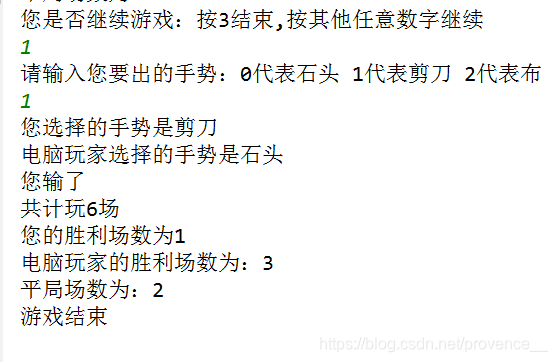