作者 群号 C语言交流中心 240137450 微信 15013593099
bzs0低清音---------------------------------------------------------------------------------------------------------------------------------------------------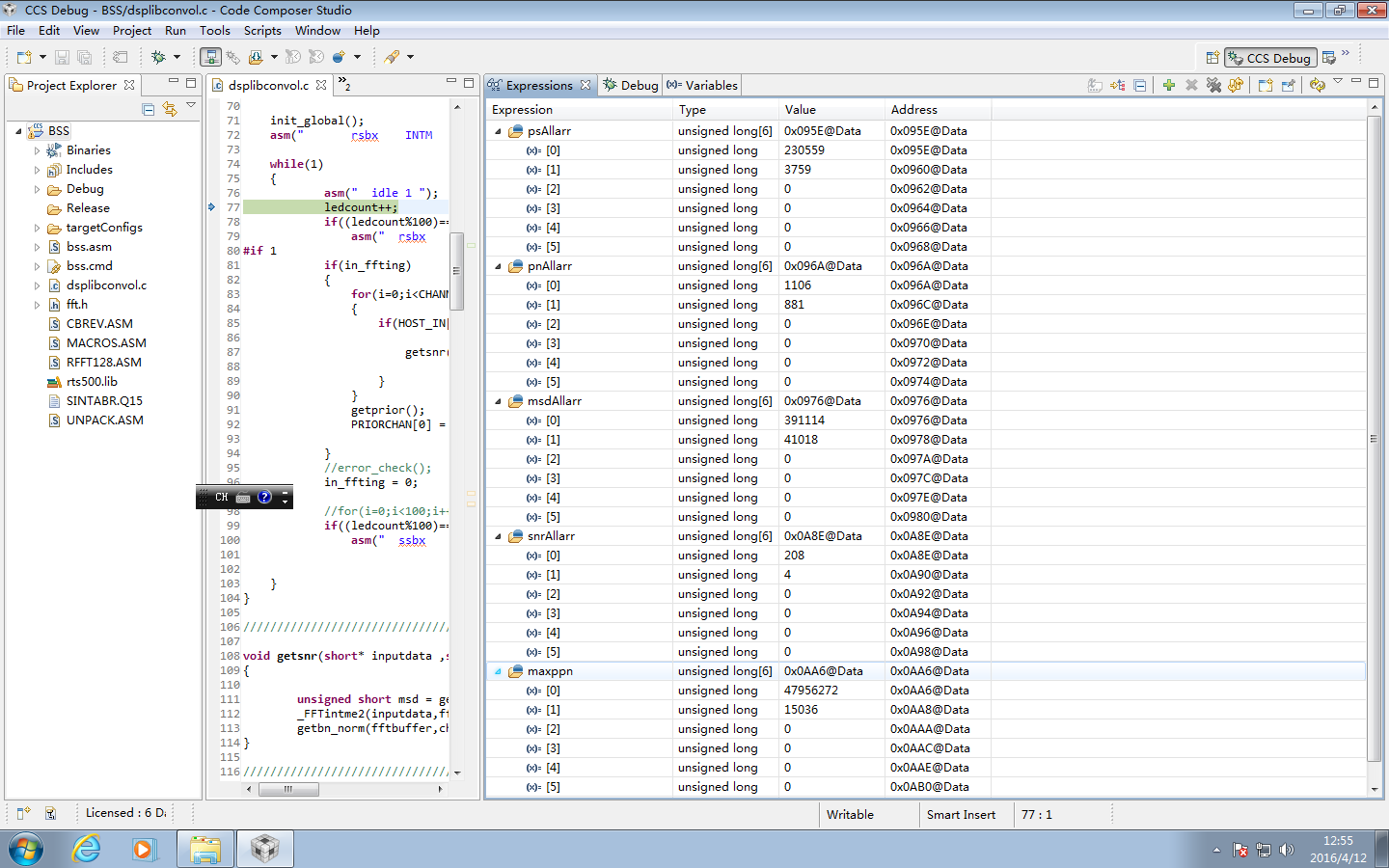
bzs0高清音
-------------------------------------------------------------------------------------------------------------
bzs3轻
----------------------------------------------------------------------------------------------
bzs3高
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
z0
-----------------------------------------------------------------------------------------------------------------------------------------
z2音
----------------------------------------------------------------------------------------------------------------------------------------------------------------------
z3音
------------------------------------------------------------------------------------------------------------------------------------------------
for(i=0;i<SAMPLEPOINTS;i=i+2)
{
x[i]=INPUT[i/2];
}
for(i=1;i<SAMPLEPOINTS;i=i+2){
x[i]=0;
}
cbrev(x,x,SAMPLEPOINTS/2);
cfft(x,128, 0);
for ( i=0;i<SAMPLEPOINTS/2;i++ ) {
OUTPUT[i]=x[i];//qrt(x[2*i]*x[2*i]+x[2*i+1]*x[2*i+1]);
}
DMAC0 bd DMAC0_ISR ; 0xFFD8
pshm ST0 ; Save status registers on stack
pshm ST1
DMAC0_ISR:
pshm AL
pshm AH
stm #0, AR2
nop
nop
ld *AR2(DMA2_IN), B ;ld #0,B
nop
nop
stl B, *AR2(DMA0_OUT)
DMAC0 bd DMAC0_ISR ; 0xFFD8
pshm ST0 ; Save status registers on stack
pshm ST1
DMAC0_ISR:
pshm AL
pshm AH
stm #0, AR2
nop
nop
ld *AR2(DMA2_IN), B ;ld #0,B
nop
nop
stl B, *AR2(DMA0_OUT)
fft例子1
#include "test.h"
short i;
short eflagf= PASS; short eflagi= PASS;
short scale = 1; short noscale = 0;
short x1[2*NX];
void main(void) {
cbrev(x,x,NX); //倒置,使x满足FFT输入的格式要求
cfft(x,NX,scale);//FFT运算
cbrev(x,x,NX);//倒置
cifft(x,NX,noscale);//IFFT运算
return;
}
在test.h中x[2*NX]存放样本函数的样点值
fft 例子2
#include "stdio.h"
#include "usbstk5515.h"
#include "usbstk5515_led.h"
#include "aic3204.h"
#include "PLL.h"
#include "bargraph.h"
#include "oled.h"
#include "pushbuttons.h"
#include "stereo.h"
#include "dsplib.h"
Int16 left_input;
Int16 right_input;
Int16 left_output;
Int16 right_output;
Int16 mono_input;
#define SAMPLES_PER_SECOND 8000
/* Use 20 for guitar */
#define GAIN_IN_dB 0
unsigned long int i = 0;
unsigned int Step = 0;
unsigned int LastStep = 99;
int j = 0;
int k = 0;
int m = 0;
int display_counter = 0;
int waveform_counter;
long delay;
DATA input_buffer[1024]; /* Must be declared as DATA for dsplib compatibility */
DATA buffer2[1024];
int display_buffer[96];
int buffer1[96];
/*****************************************************************************/
/* calculate_power() /
/---------------------------------------------------------------------------*/
/* /
/ Parameter 1: Real term a. /
/ Parameter 2: Immaginary term jb. /
/ /
/ RETURNS: a*a + b*b. Result will always be positive. /
/ /
/****************************************************************************/
int calculate_power (int a, int b)
{
return ( (int) ( ( (long)a * a + (long) b * b) >> 14) );
}
/*****************************************************************************/
/* calculate_FFT() /
/---------------------------------------------------------------------------*/
/* /
/ Parameter 1: Latest audio input (real value). /
/ Parameter 2: size of FFT e.g. 128, 512 and 1024 elements. /
/ /
/ RETURNS: None. /
/ /
/****************************************************************************/
void calculate_FFT(int input, int size)
{
static int i = 0;
static int counter = 0;
buffer2[i] = input; /* Store as a real value /
i++;
buffer2[i] = 0; / Store with an imaginary value of 0 */
i++;
if ( i >= size-1)
{
i = 0;
/* Perform complex FFT using N real and N imaginary values */
cfft (&buffer2[0], size/2, SCALE);
cbrev(&buffer2[0], &buffer2[0], size/2);
for ( j = 0 ; j < 96 ; j ++)
{
display_buffer[j] = calculate_power((int) buffer2[2*j], (int)buffer2[2*j+1]);
}
counter++;
if ( counter >= 1)
{
/* Slow down the number of updates to make display easier to see */
counter = 0;
oled_display_bargraph( &display_buffer[0]);
}
}
}
/* ------------------------------------------------------------------------ *
*
main( ) *
*
------------------------------------------------------------------------ /
void main( void )
{
/ Initialize BSL */
USBSTK5515_init( );
/* Initialize PLL */
pll_frequency_setup(120);
/* Initialise hardware interface and I2C for code */
aic3204_hardware_init();
/* Initialise the AIC3204 codec */
aic3204_init();
/* Turn off the 4 coloured LEDs */
USBSTK5515_ULED_init();
/* Initialise the OLED LCD display /
oled_init();
SAR_init();
/ Flush display buffer */
oled_display_message(" ", " ");
printf("\n\nRunning Project Spectrum Analyser\n");
printf( "<-> Audio Loopback from Microphone In --> to Headphones/Lineout\n\n" );
/* Setup sampling frequency and 30dB gain for microphone */
set_sampling_frequency_and_gain(SAMPLES_PER_SECOND, GAIN_IN_dB);
oled_display_message("Application 20 ", "Spectrum Analyser ");
/* New. Add descriptive text */
puts("\n Bargraph at 6dB intervals");
puts("\n Press SW1 for DOWN, SW2 for UP, SW1 + SW2 for reset\n");
puts(" Step 1 = Straight through, no signal processing. Set levels");
puts(" Step 2 = Waveform view");
puts(" Step 3 = FFT 1024 Display. Calculate power and display as bargraph");
puts(" Step 4 = FFT 512 Display. Calculate power and display as bargraph");
/* Default to XF LED off */
asm(" bclr XF");
for ( i = 0 ; i < SAMPLES_PER_SECOND * 600L ;i++ )
{
aic3204_codec_read(&left_input, &right_input); // Configured for one interrupt per two channels.
mono_input = stereo_to_mono(left_input, right_input);
Step = pushbuttons_read(4);
if ( Step == 1 )
{
if ( Step != LastStep )
{
oled_display_message("STEP1 No Processing", "Set Levels ");
LastStep = Step;
}
left_output = left_input; // Straight trough. No processing.
right_output = right_input;
}
else if ( Step == 2)
{
if ( Step != LastStep)
{
oled_display_message("STEP2 ", " Waveform View");
LastStep = Step;
display_counter = 0;
waveform_counter = 0;
}
if ( display_counter < 8000)
{
display_counter++;
}
if (display_counter >= 8000)
{
buffer1[k] = mono_input;
k++;
if ( k >= 96)
{
k = 0;
waveform_counter++;
if ( waveform_counter >= 6)
{
waveform_counter = 0;
oled_display_waveform(&buffer1[0]);
delay = 0xFFFFFF;
while ( delay--)
{
/* Wait */
}
}
}
}
}
else if ( Step == 3)
{
if ( Step != LastStep)
{
oled_display_message("STEP3 ", " FFT 1024 Display");
LastStep = Step;
display_counter = 0;
}
if ( display_counter < 8000)
{
display_counter++;
}
if (display_counter >= 8000)
{
calculate_FFT(mono_input, 1024);
}
}
else if ( Step == 4)
{
if ( Step != LastStep)
{
oled_display_message("STEP4 ", " FFT 512 Display");
LastStep = Step;
display_counter = 0;
}
if ( display_counter < 8000)
{
display_counter++;
}
if (display_counter >= 8000)
{
calculate_FFT(mono_input, 512);
}
}
aic3204_codec_write(left_output, right_output);
if ( Step == 1)
{
/* Only display bargraph when setting up. Distracting otherwise */
bargraph_6dB(left_output, right_output);
}
}
/* Disable I2S and put codec into reset */
aic3204_disable();
printf( "\n***Program has Terminated***\n" );
oled_display_message("PROGRAM HAS ", "TERMINATED ");
SW_BREAKPOINT;
}
/* ------------------------------------------------------------------------ *
*
End of main.c *
*
------------------------------------------------------------------------ */
定点fft
#include "math.h"
#include "fft.h"
typedef struct COMPLEX3{
long real,imag;
}COMPLEX3;
void fft2(int n,COMPLEX3 f[]);
static COMPLEX3 _inputpoint[SAMPLEPOINTS];
void FFTme(short * inputtimrs,unsigned short * freqz,int size)
{
// 计算加权系数
int i;
for(i=0;i<size;i++){
_inputpoint[i].real=inputtimrs[i];
_inputpoint[i].imag=0;
}
fft2(size,_inputpoint);
for(i=0;i<size;i++){
freqz[i]=sqrt((_inputpoint[i].real*_inputpoint[i].real)+(_inputpoint[i].imag*_inputpoint[i].imag));
}
}
//精度0.0001弧度
void conjugate_complex(int n,COMPLEX3 in[],COMPLEX3 out[])
{
int i = 0;
for(i=0;i<n;i++)
{
out[i].imag = -in[i].imag;
out[i].real = in[i].real;
}
}
void c_add(COMPLEX3 a,COMPLEX3 b,COMPLEX3 *c)
{
c->real = a.real + b.real;
c->imag = a.imag + b.imag;
}
void c_sub(COMPLEX3 a,COMPLEX3 b,COMPLEX3 *c)
{
c->real = a.real - b.real;
c->imag = a.imag - b.imag;
}
#define FFTSHIFT 14
void c_mul_sin(COMPLEX3 a,COMPLEX3 b_sin,COMPLEX3 *c)
{
long ma;
long mb;
ma=a.real * b_sin.real;
ma=ma>>FFTSHIFT;
mb=a.imag * b_sin.imag;
mb=mb>>FFTSHIFT;
c->real = ma - mb;
ma=a.real * b_sin.imag;
ma=ma>>FFTSHIFT;
mb=a.imag * b_sin.real;
mb=mb>>FFTSHIFT;
c->imag =ma + mb;
}
/*
void c_div(COMPLEX3 a,COMPLEX3 b,COMPLEX3 *c)
{
c->real = (a.real * b.real + a.imag * b.imag)/(b.real * b.real +b.imag * b.imag);
c->imag = (a.imag * b.real - a.real * b.imag)/(b.real * b.real +b.imag * b.imag);
}
*/
#define SWAP(a,b) tempr=(a);(a)=(b);(b)=tempr
//
short cosin[128]={
16384,
16364,
16305,
16206,
16069,
15892,
15678,
15426,
15136,
14810,
14449,
14053,
13622,
13159,
12665,
12139,
11585,
11002,
10393,
9759,
9102,
8423,
7723,
7005,
6269,
5519,
4756,
3981,
3196,
2404,
1605,
803,
0,
-803,
-1605,
-2404,
-3196,
-3980,
-4755,
-5519,
-6269,
-7005,
-7723,
-8423,
-9102,
-9759,
-10393,
-11002,
-11585,
-12139,
-12664,
-13159,
-13622,
-14053,
-14449,
-14810,
-15136,
-15426,
-15678,
-15892,
-16069,
-16206,
-16305,
-16364,
-16383,
-16364,
-16305,
-16206,
-16069,
-15893,
-15678,
-15426,
-15136,
-14810,
-14449,
-14053,
-13622,
-13159,
-12665,
-12139,
-11585,
-11002,
-10393,
-9759,
-9102,
-8423,
-7723,
-7005,
-6269,
-5519,
-4756,
-3981,
-3196,
-2404,
-1605,
-803,
0,
803,
1605,
2403,
3196,
3980,
4755,
5519,
6269,
7004,
7723,
8422,
9102,
9759,
10393,
11002,
11585,
12139,
12664,
13159,
13622,
14052,
14449,
14810,
15136,
15426,
15678,
15892,
16069,
16206,
16305,
16364,
};
short sine[128]={
0,
803,
1605,
2404,
3196,
3980,
4756,
5519,
6269,
7005,
7723,
8423,
9102,
9759,
10393,
11002,
11585,
12139,
12664,
13159,
13622,
14053,
14449,
14810,
15136,
15426,
15678,
15892,
16069,
16206,
16305,
16364,
16383,
16364,
16305,
16206,
16069,
15892,
15678,
15426,
15136,
14810,
14449,
14053,
13622,
13159,
12665,
12139,
11585,
11002,
10393,
9759,
9102,
8423,
7723,
7005,
6269,
5519,
4756,
3981,
3196,
2404,
1605,
803,
0,
-803,
-1605,
-2403,
-3196,
-3980,
-4755,
-5519,
-6269,
-7005,
-7723,
-8423,
-9102,
-9759,
-10393,
-11002,
-11585,
-12139,
-12664,
-13159,
-13622,
-14052,
-14449,
-14810,
-15136,
-15426,
-15678,
-15892,
-16069,
-16206,
-16305,
-16364,
-16383,
-16364,
-16305,
-16206,
-16069,
-15893,
-15678,
-15426,
-15136,
-14810,
-14449,
-14053,
-13622,
-13159,
-12665,
-12139,
-11585,
-11002,
-10393,
-9760,
-9102,
-8423,
-7723,
-7005,
-6269,
-5519,
-4756,
-3981,
-3196,
-2404,
-1605,
-804,
};
//
void Wn_i(int n,int i,COMPLEX3 *Wn,char flag)
{
Wn->real =cosin[i];// cos(2*PI*i/n);
if(flag == 1)
Wn->imag =-sine[i];// -sin(2*PI*i/n);
else if(flag == 0)
Wn->imag =sine[i];// -sin(2*PI*i/n);
}
//
int my_pow(int a,int b)
{
int c=1;
int i;
for(i=0;i<b;i++){
c =c*a;
}
return c;
}
//傅里叶变化
void fft2(int N,COMPLEX3 f[])
{
COMPLEX3 t,wn;//中间变量
short i,j,k,m,n,l,r,M;
long la,lb,lc;
/*----计算分解的级数M=log2(N)----*/
for(i=N,M=1;(i=i/2)!=1;M++);
/*----按照倒位序重新排列原信号----*/
for(i=1,j=N/2;i<=N-2;i++)
{
if(i<j)
{
t=f[j];
f[j]=f[i];
f[i]=t;
}
k=N/2;
while(k<=j)
{
j=j-k;
k=k/2;
}
j=j+k;
}
/*----FFT算法----*/
for(m=1;m<=M;m++)
{
la=my_pow(2,m); //la=2^m代表第m级每个分组所含节点数
lb=la/2; //lb代表第m级每个分组所含碟形单元数
//同时它也表示每个碟形单元上下节点之间的距离
/*----碟形运算----*/
for(l=1;l<=lb;l++)
{
r=(l-1)*my_pow(2,M-m);
for(n=l-1;n<N-1;n=n+la) //遍历每个分组,分组总数为N/la
{
lc=n+lb; //n,lc分别代表一个碟形单元的上、下节点编号
Wn_i(N,r,&wn,1);//wn=Wnr
c_mul_sin(f[lc],wn,&t);//t = f[lc] * wn复数运算
c_sub(f[n],t,&(f[lc]));//f[lc] = f[n] - f[lc] * Wnr
c_add(f[n],t,&(f[n]));//f[n] = f[n] + f[lc] * Wnr
}
}
}
return ;
}
void MakeWave()
{
int i;
int j;
int f1a=29;
int f1=400;
int f2a=30;
int f2=600;
int f3=3000;
int f3a=88;
float val;
for(i=0;i<SAMPLEPOINTS+BL;i++){
val=sin((2*PI*f1*i)/8000)*f1a+sin((2*PI*f2*i)/8000)*f2a+sin((2*PI*f3*i)/8000)*f3a;
INPUT[i]=val;
}
}
extern unsigned short fftbuffer[];
/
void main()
{
short i;
short j;
short index=0;
MakeWave();
FFTme(INPUT,fftbuffer,SAMPLEPOINTS);
while(1);
红色是定点Fir后的信号以及fft
黑色是原始信号以及fft
dsplib用法例子
跟大家交流一下FFT函数库的用法。相信很多人都有用
函数用的是TI的,之前参考了他的文档,不太清楚,说起教科书?我真想揍那些wbd!说得跟实际关系太少,靠,什么破书!
然后我就在论坛搜索了一下,发现 computer00 和 iceage 说的特有启发性,呵呵,先感谢他们。
废话少讲:
cpu=6713
原始信号:rand()/32767.0+(sin(2 * PI * F1 * i / N) + sin(2 * PI * F2 * i / N)+ sin(2 * PI * F3 * i / N) );
用到ti的函数库:dsp67x.lib
我把程序贴一下:
#define N 512
#define F1 20
#define F2 100000
#define F3 1000
gen_w_r2(wd, N); // 产生旋转因子
bit_rev(wd, N>>1); // 把系数倒置,为下面函数输入准备
DSPF_sp_cfftr2_dit(xd, wd, N); //计算该FFT,需要先调用上面两个函数
//输出是倒置的,所以重新倒置为正常
bit_rev(xd,N);
//画频谱图
for (i=0;i<(N);i++)
{
mo[i] = sqrt(xd[2*i]*xd[2*i]+xd[2*i+1]*xd[2*i+1])/( i==0 ? N:(N>>1) );
} //求输出模值
//画相位图
for(i=0;i<N;i++)
{
wd[i]=atan(xd[2*i+1]/xd[2*i]);
}
、、、、、、、
其中,wd[N]为存放旋转因子用的,xd[2*N]是用来计算FFT的输入矩阵,因为FFT是复数运算,但我们基本用的是实数,所以xd是
连续实部虚部存放的:re[0],im[0],re[1],im[1],......,
所以我们AD采样数据后把实际数据存入xd[2*i],虚部xd[2*i+1]全部置为零,就可以用DSPF_sp_cfftr2_dit(xd, wd, N)了。
这个DSPF_sp_cfftr2_dit(xd, wd, N)输出有点奇怪,是位倒置的,具体如何倒,就要看看教科书了,哈哈,这时候还是有参考价
值得。所以输入xd变成了输出xd,但是还需要调用bit_rev才能转换成自然顺序。
有兄弟要问?FFt后出来的是啥东东?
我以前搞过,这两天也是才想起来。
computer00说得很清楚了:
““
如果我没理解错的话,FFT后的第1个点应该就是直流分量,第128个点就是采样频率,中间按线性划分。
每个点对应的值,就是该频率下的幅度。
””
所以,出来的数组xd[0],xd[1]就分别是直流分量的实部跟虚部!!!!!!,xd[2*i],xd[2*i+1]就是第i个频率的实部跟虚部,
这下大家清楚了吧???
好了,有了这些东东我们就可以做其他的分析了,比如 iceage 讲的:
“
FFT 的结果为 N/2 + 1 复数序列:R(k) + j I(k), k=0 为 DC, 第 k 次谐波的幅度为:sqrt(R^2 + I^2), 相位: arctan(I,
R). 基波(k = 1) frequency 为 sampling_frequency / window_size .
"比如噪声在低频的话 是不是把信号FFT了 然后把前面的数据变成0的话就可以减小噪声了" --- 没错, 不过很少有人做这么奢
侈的滤波器,上述的频域表达式为:
F'(K) = F(K) * H(K) ---- F(K) 是原始时域信号 f(k) 频谱序列,H(K) = [0 0 0 ... 1 1 1 ... ], 把前面的数据变成0
”
没错!我们只要把不要的频率成分变成0,然后反FFT变换就OK了!其实FFT就是滤波器,哈哈。
还有个问题,我要求幅值跟相位怎么办?这里也有个问题,估计教科书没怎么讲的。幅值就是复数的模呗,但是用公式
mo=sqrt(Re*Re + Im*Im)计算出来的并不是实际频率对应的模,他还需要除于一个系数,这个系数也不能瞎除,教科书有没有
讲,我也不清楚了!
computer00是这样讲的:
“取它的模,然后应该在第1点有一个非0值,这个值除以64,就是它的这个10Hz信号的峰值了。
(注意频率为0时,是除以128).”
注意,这里128是采样128点,我这里是512点。也就是说,我采样N点,直流分量的幅值就是第一个复数的模除于N,第二个开始就
处于N/2,所以程序会出现“/( i==0 ? N:(N>>1) )”这个东东。
相位也简单了,就是arctan(Im/Re)。说道这里,大家清楚这个FFT函数怎么用了吧?
汇编的fft算法实现例子
fft算法
extern short fftcalcbuffer[];
short rfft128 (short *x, short scale);
short cbrev (short *x, short *y, unsigned short n);
void _FFTintme2(short * input,unsigned short * freqz,int size)
{
int i;
long temp1;
long temp2;
for(i=0;i<size;i++){
fftcalcbuffer[i]=input[i];
//calcbuffer[i+i+1]=0;
}
cbrev(fftcalcbuffer,fftcalcbuffer,SAMPLEPOINTS/2);
rfft128(fftcalcbuffer,1);
//cbrev(calcbuffer,cfftbuffer,SAMPLEPOINTS/2);
for(i=0;i<SAMPLEPOINTS/2;i++){
short n=2*i;
temp1 = fftcalcbuffer[n]/2;
temp1 *=temp1;
temp2 = fftcalcbuffer[n+1]/2;
temp2 *=temp2;
temp1+=temp2;
freqz[i]=sqrt(temp1);
}
}
cmd文件
SECTIONS
{
.text > PROG PAGE 0
.cinit > PROG PAGE 0
vectors > VECT PAGE 0
.data > DATA PAGE 1
.bss > DATA PAGE 1
.const > DATA PAGE 1
.sysmem > DATA PAGE 1
.cio > DATA PAGE 1
.sintab : align(512)> DATA PAGE 1
stack > asmSTACK PAGE 1
dma_buf_in > DMAIN PAGE 1
dma_buf_out > DMAOUT PAGE 1
sample_in > INPUT PAGE 1
fir_before > BEFORE PAGE 1
fftcalcbuffer : align(512) {}>DATA PAGE 1
.stack > CSTCK PAGE 1
host_buf > HBUF PAGE 1
}
asm文件
_DMA2_IN .usect "dma_buf_in",10h
_INPUT .usect "sample_in",256*8
_BEFORE .usect "fir_before",33*8
_fftcalcbuffer .usect "fftcalcbuffer",128*2
void MakeWave()
{
int i;
int j;
int f1a=10; int f1=400;
int f2a=100; int f2=800;
int f3a=1000; int f3=1200;
float val;
for(i=nextsampleI;i<SAMPLEPOINTS+nextsampleI;i++)
{
val=sin((2*PI*f1*i)/8000)*f1a/*+sin((2*PI*f2*i)/8000)*f2a+sin((2*PI*f3*i)/8000)*f3a*/;
INPUT[i-nextsampleI]=val;
}
nextsampleI+=SAMPLEPOINTS;
}
extern unsigned short fftbuffer[SAMPLEPOINTS];
void main()
{
short i;
short j;
short index=0;
short * pDb;
pDb = &BEFORE[0];
while(1){
MakeWave();
_FFTintme2(INPUT,fftbuffer,SAMPLEPOINTS);
//getpriorchennel(INPUT,SAMPLEPOINTS,&pDb,0);
}
//while(1);
dsp out文件转换为bin 文件
The Windows batch file tiobj2bin.bat converts a TI executable file, COFF
or ELF, to a "binary" file. This binary format is the one defined by the
"objcopy" Unix command. Here is a description of the format from the
objcopy man page ...
When objcopy generates a raw binary file, it will essentially produce
a memory dump of the contents of the input object file. All symbols
and relocation information will be discarded. The memory dump will
start at the load address of the lowest section copied into the output
file.
This batch file makes use of a new script mkhex4bin.pl to create a hex
utility command file. The hex utility does the heavy lifting. Documentation
on how to use tiobj2bin.bat is contained in comments at the top of the
file. To see a command invocation summary, run tiobj2bin.bat with no
parameters.
rem ==========================================================================
rem tiobj2bin.bat - Converts TI object file from COFF or ELF to binary
rem dump format. Intended for use as a post-build step in CCS.
rem
rem This code released under a license detailed at the end of this file.
rem
rem Invoke: tiobj2bin file.out file.bin [ofd] [hex] [mkhex]
rem
rem file.out - The TI .out file to convert to binary. Can be COFF or ELF.
rem file.bin - Name the binary output file
rem ofd - The object file display (ofd) utility command to invoke, with
rem path info as needed. If not given, defaults to ofd470.
rem hex - The hex utility command to invoke, with path info as needed.
rem If not given, defaults to hex470.
rem mkhex - A custom utility which takes XML from OFD and outputs the hex
rem command file needed for the hex utility. Path info is given
rem as needed. If not given, defaults to mkhex4bin.
我在CCSV5.3+RM48L950开发时,在工程属性页面:build->steps->Post-build steps->command下,输入命令:
"${CCS_INSTALL_ROOT}/utils/tiobj2bin/tiobj2bin.bat" "${BuildArtifactFileName}" "${BuildArtifactFileBaseName}.bin" "${CG_TOOL_ROOT}/bin/armofd.exe" "${CG_TOOL_ROOT}/bin/armhex.exe" "${CCS_INSTALL_ROOT}/utils/tiobj2bin/mkhex4bin.exe"
生成的bin文件过大,有131M,我的out文件才255KB。
工程中sys_link.cmd文件的内容:
/*----------------------------------------------------------------------------*/
/* sys_link.cmd */
/* */
/* (c) Texas Instruments 2009-2013, All rights reserved. */
/* */
/* USER CODE BEGIN (0) */
/* USER CODE END */
/*----------------------------------------------------------------------------*/
/* Linker Settings */
--retain="*(.intvecs)"
/* USER CODE BEGIN (1) */
/* USER CODE END */
/*----------------------------------------------------------------------------*/
/* Memory Map */
MEMORY
{
VECTORS (X) : origin=0x00000000 length=0x00000020
CODE (RX) : origin=0x00000020 length=0x03FFE0
STACKS (RW) : origin=0x08000000 length=0x00001500
RAM (RW) : origin=0x08001500 length=0x0003EB00
/* USER CODE BEGIN (2) */
/* USER CODE END */
}
/* USER CODE BEGIN (3) */
/* USER CODE END */
/*----------------------------------------------------------------------------*/
/* Section Configuration */
SECTIONS
{
.intvecs : {} > VECTORS
.text : {} > CODE
.const : {} > CODE
.cinit : {} > CODE
.pinit : {} > CODE
.LOGIC : {} > CODE
.bss : {} > RAM
.data : {} > RAM
.sysmem : {} > RAM
/* USER CODE BEGIN (4) */
/* USER CODE END */
}
/* USER CODE BEGIN (5) */
/* USER CODE END */
/*----------------------------------------------------------------------------*/
/* Misc */
/* USER CODE BEGIN (6) */
/* USER CODE END */
/*----------------------------------------------------------------------------*/
tiobj2bin.bat bss.out bss.out.bin E:\ti\ccsv5\tools\compiler\c5400_4.2.0\bin\ofd500.exe E:\ti\ccsv5\tools\compiler\c5400_4.2.0\bin\hex500.exe mkhex4bin.exe
2016/04/07 18:45 <DIR> .
2016/04/07 18:45 <DIR> ..
2016/04/07 17:23 40,215 bss.out
2016/04/07 18:45 29,012 bss.out.bin
2011/06/02 15:29 2,336,899 mkhex4bin.exe
2013/03/14 14:44 10,663 tiobj2bin.bat