http://msdn.microsoft.com/zh-cn/library/system.windows.controls.viewbox.aspx
这里我们将介绍Silverlight中ViewBox组件,这个组件的作用主要是做布局与视觉效果。并给出实例代码和最终效果图。
ViewBox组件的作用是拉伸或延展位于其中的组件,使之有更好的布局及视觉效果。本文将为大家介绍该组件的基本特性以及应用实例。
组件所在命名空间:
System.Windows.Controls
组件常用属性:
Child:获取或设置一个ViewBox元素的单一子元素。
Stretch:获取或设置拉伸模式以决定该组件中的内容以怎样的形式填充该组件的已有空间。
StretchDirection:获取或设置该组件的拉伸方向以决定该组件中的内容将以何种形式被延展。
实例:
详细的说明在代码注释中给出。
MainPage.xaml文件代码:
<Window x:Class="WpfApplication3.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid x:Name="LayoutRoot" Width="320" Height="240" Background="White">
<Slider x:Name="HSlider" Minimum="0" Maximum="100" Height="24" Margin="79,0,91,42" VerticalAlignment="Bottom" Width="150"/>
<Slider x:Name="VSlider" Minimum="0" Maximum="100" HorizontalAlignment="Right" Margin="0,24,57,66" Width="30" Orientation="Vertical" Height="150"/>
<Border Margin="79,24,91,66" BorderBrush="Black" BorderThickness="1">
<Grid x:Name="theContainer" Background="AntiqueWhite">
<Viewbox x:Name="sampleViewBox" Margin="0,0,-2,-2">
<!--放入ViewBox中的按钮对象-->
<Button Width="101" Content="Button"/>
</Viewbox>
</Grid>
</Border>
<ComboBox x:Name="cbStretch" Height="21" HorizontalAlignment="Left" Margin="8,0,0,8" VerticalAlignment="Bottom" Width="139"/>
<ComboBox x:Name="cbStretchDirection" Height="21" HorizontalAlignment="Right" Margin="0,0,8,8" VerticalAlignment="Bottom" Width="139"/>
<TextBlock Height="16" HorizontalAlignment="Left" Margin="9,0,0,33" VerticalAlignment="Bottom" Width="66" Text="拉伸模式:" TextWrapping="Wrap"/>
<TextBlock Height="16" HorizontalAlignment="Right" Margin="0,0,8,33" VerticalAlignment="Bottom" Width="56" Text="拉伸方向:" TextWrapping="Wrap"/>
</Grid>
</Window>
MainPage.xaml.cs文件代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WpfApplication3
{
//辅助类StretchHelper
public class StretchHelper
{
public string StretchModeName { get; set; }
public Stretch theStretchMode { get; set; }
}
//辅助类StretchDirectionHelper
public class StretchDirectionHelper
{
public string StretchDirectionName { get; set; }
public StretchDirection theStretchDirection { get; set; }
}
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
//定义cbStretch与cbStretchDirection的数据源
List<StretchHelper> cbStretchList = new List<StretchHelper>();
List<StretchDirectionHelper> cbStretchDirectionList = new List<StretchDirectionHelper>();
public MainWindow()
{
InitializeComponent();
//注册事件触发
this.Loaded += new RoutedEventHandler(MainPage_Loaded);
this.cbStretch.SelectionChanged += new SelectionChangedEventHandler(cbStretch_SelectionChanged);
this.cbStretchDirection.SelectionChanged += new SelectionChangedEventHandler(cbStretchDirection_SelectionChanged);
this.HSlider.ValueChanged += new RoutedPropertyChangedEventHandler<double>(HSlider_ValueChanged);
this.VSlider.ValueChanged += new RoutedPropertyChangedEventHandler<double>(VSlider_ValueChanged);
}
void VSlider_ValueChanged(object sender, RoutedPropertyChangedEventArgs<double> e)
{
sampleViewBox.Height = theContainer.ActualHeight * VSlider.Value / 100.0;
}
void HSlider_ValueChanged(object sender, RoutedPropertyChangedEventArgs<double> e)
{
sampleViewBox.Width = theContainer.ActualWidth * HSlider.Value / 100.0;
}
void cbStretchDirection_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
if (cbStretchDirection.SelectedItem != null)
{
sampleViewBox.StretchDirection = (cbStretchDirection.SelectedItem as StretchDirectionHelper).theStretchDirection;
}
}
void cbStretch_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
if (cbStretch.SelectedItem != null)
{
sampleViewBox.Stretch = (cbStretch.SelectedItem as StretchHelper).theStretchMode;
}
}
void MainPage_Loaded(object sender, RoutedEventArgs e)
{
//填充各ComboBox内容
cbStretchList.Add(new StretchHelper() { StretchModeName = "Fill", theStretchMode = Stretch.Fill });
cbStretchList.Add(new StretchHelper() { StretchModeName = "None", theStretchMode = Stretch.None });
cbStretchList.Add(new StretchHelper() { StretchModeName = "Uniform", theStretchMode = Stretch.Uniform });
cbStretchList.Add(new StretchHelper() { StretchModeName = "UniformToFill", theStretchMode = Stretch.UniformToFill });
cbStretch.ItemsSource = cbStretchList;
cbStretch.DisplayMemberPath = "StretchModeName";
cbStretchDirectionList.Add(new StretchDirectionHelper() { StretchDirectionName = "DownOnly", theStretchDirection = StretchDirection.DownOnly });
cbStretchDirectionList.Add(new StretchDirectionHelper() { StretchDirectionName = "UpOnly", theStretchDirection = StretchDirection.UpOnly });
cbStretchDirectionList.Add(new StretchDirectionHelper() { StretchDirectionName = "Both", theStretchDirection = StretchDirection.Both });
cbStretchDirection.ItemsSource = cbStretchDirectionList;
cbStretchDirection.DisplayMemberPath = "StretchDirectionName";
}
}
}
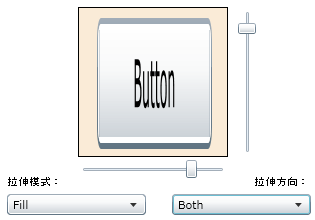