效果图
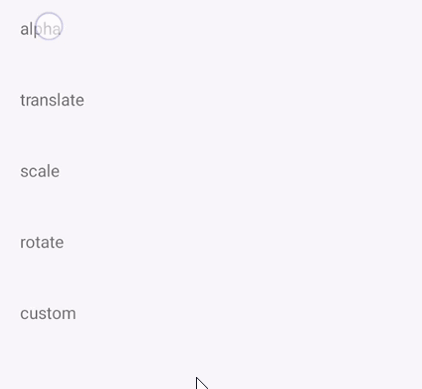
MyAnimTextView
public class MyAnimTextView extends AppCompatTextView {
private Animation mAlpha;
private Animation mTranslate;
private Animation mScale;
private Animation mRotate;
private MyAnimListener myAnimListener;
public MyAnimTextView(@NonNull Context context) {
super(context);
}
public MyAnimTextView(@NonNull Context context, @Nullable AttributeSet attrs) {
super(context, attrs, android.R.attr.textViewStyle);
mAlpha = AnimationUtils.loadAnimation(context, R.anim.alpha);
mTranslate = AnimationUtils.loadAnimation(context, R.anim.translate);
mScale = AnimationUtils.loadAnimation(context, R.anim.scale);
mRotate = AnimationUtils.loadAnimation(context, R.anim.rotate);
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.MyAnimTextView);
final int anInt = typedArray.getInt(R.styleable.MyAnimTextView_animType, 0);
typedArray.recycle();
mAlpha.setInterpolator(new CycleInterpolator(0.5f));
mTranslate.setInterpolator(new BounceInterpolator());
mTranslate.setFillAfter(true);
mScale.setInterpolator(new BounceInterpolator());
mRotate.setInterpolator(new BounceInterpolator());
mRotate.setFillAfter(true);
setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
switch (anInt) {
case 0:
startAnimation(mAlpha);
break;
case 1:
startAnimation(mTranslate);
break;
case 2:
startAnimation(mScale);
break;
case 3:
startAnimation(mRotate);
break;
}
}
});
mAlpha.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
setClickable(false);
setFocusable(false);
}
@Override
public void onAnimationEnd(Animation animation) {
setClickable(true);
setFocusable(true);
if (myAnimListener != null) {
myAnimListener.onClick(MyAnimTextView.this);
}
}
@Override
public void onAnimationRepeat(Animation animation) {
}
});
mTranslate.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
setClickable(false);
setFocusable(false);
}
@Override
public void onAnimationEnd(Animation animation) {
setClickable(true);
setFocusable(true);
if (myAnimListener != null) {
myAnimListener.onClick(MyAnimTextView.this);
}
}
@Override
public void onAnimationRepeat(Animation animation) {
}
});
mScale.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
setClickable(false);
setFocusable(false);
}
@Override
public void onAnimationEnd(Animation animation) {
setClickable(true);
setFocusable(true);
if (myAnimListener != null) {
myAnimListener.onClick(MyAnimTextView.this);
}
}
@Override
public void onAnimationRepeat(Animation animation) {
}
});
mRotate.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
setClickable(false);
setFocusable(false);
}
@Override
public void onAnimationEnd(Animation animation) {
setClickable(true);
setFocusable(true);
if (myAnimListener != null) {
myAnimListener.onClick(MyAnimTextView.this);
}
}
@Override
public void onAnimationRepeat(Animation animation) {
}
});
}
public void setMyAnimListener(MyAnimListener myAnimListener) {
this.myAnimListener = myAnimListener;
}
public MyAnimTextView(@NonNull Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
public void setMyAnim(final Animation animation) {
setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
startAnimation(animation);
}
});
}
public interface MyAnimListener {
void onClick(MyAnimTextView view);
}
}
新建declare-styleable
<declare-styleable name="MyAnimTextView">
<attr name="animType">
<enum name="alpha" value="0" />
<enum name="translate" value="1" />
<enum name="scale" value="2" />
<enum name="rotate" value="3" />
</attr>
</declare-styleable>
使用
<com.weiou.mydemo.anim.MyAnimTextView
android:id="@+id/tv4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:text="rotate"
app:animType="rotate" />
MyAnimTextView tv4 = findViewById(R.id.tv4);
tv4.setMyAnimListener(new MyAnimTextView.MyAnimListener() {
@Override
public void onClick(MyAnimTextView view) {
LogUtil.e("动画播放结束后会执行");
}
});
属性说明
- animType
- alpha 透明度动画
- translate 平移动画
- scale 缩放动画
- rotate 选装动画
动画代码贴出
<?xml version="1.0" encoding="utf-8"?>
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="500"
android:fromAlpha="1.0"
android:toAlpha="0.5">
</alpha>
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="500"
android:pivotX="50%"
android:pivotY="50%"
android:fromDegrees="0"
android:toDegrees="-270"
>
</rotate>
<?xml version="1.0" encoding="utf-8"?>
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="500"
android:fromXScale="1"
android:fromYScale="1"
android:toXScale="1.5"
android:toYScale="1.5"
android:pivotX="50%"
android:pivotY="50%"
android:fillAfter="true"
>
</scale>
<?xml version="1.0" encoding="utf-8"?>
<translate xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="500"
android:fromXDelta="0"
android:fromYDelta="0"
android:toXDelta="100%"
android:toYDelta="0"
>
</translate>