谷歌提供了搜索控件SearchView,在v7包中,我们可以在Toolbar的menu中定义它
<?xml version ="1.0" encoding ="utf-8"?><!-- Learn More about how to use App Actions: https://developer.android.com/guide/actions/index.html -->
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/searchview"
android:orderInCategory="100"
app:actionViewClass="android.support.v7.widget.SearchView"
android:title="搜索"
app:showAsAction="always" />
<item
android:title="其他"
app:showAsAction="never" />
<item
android:title="设置"
app:showAsAction="never" />
</menu>
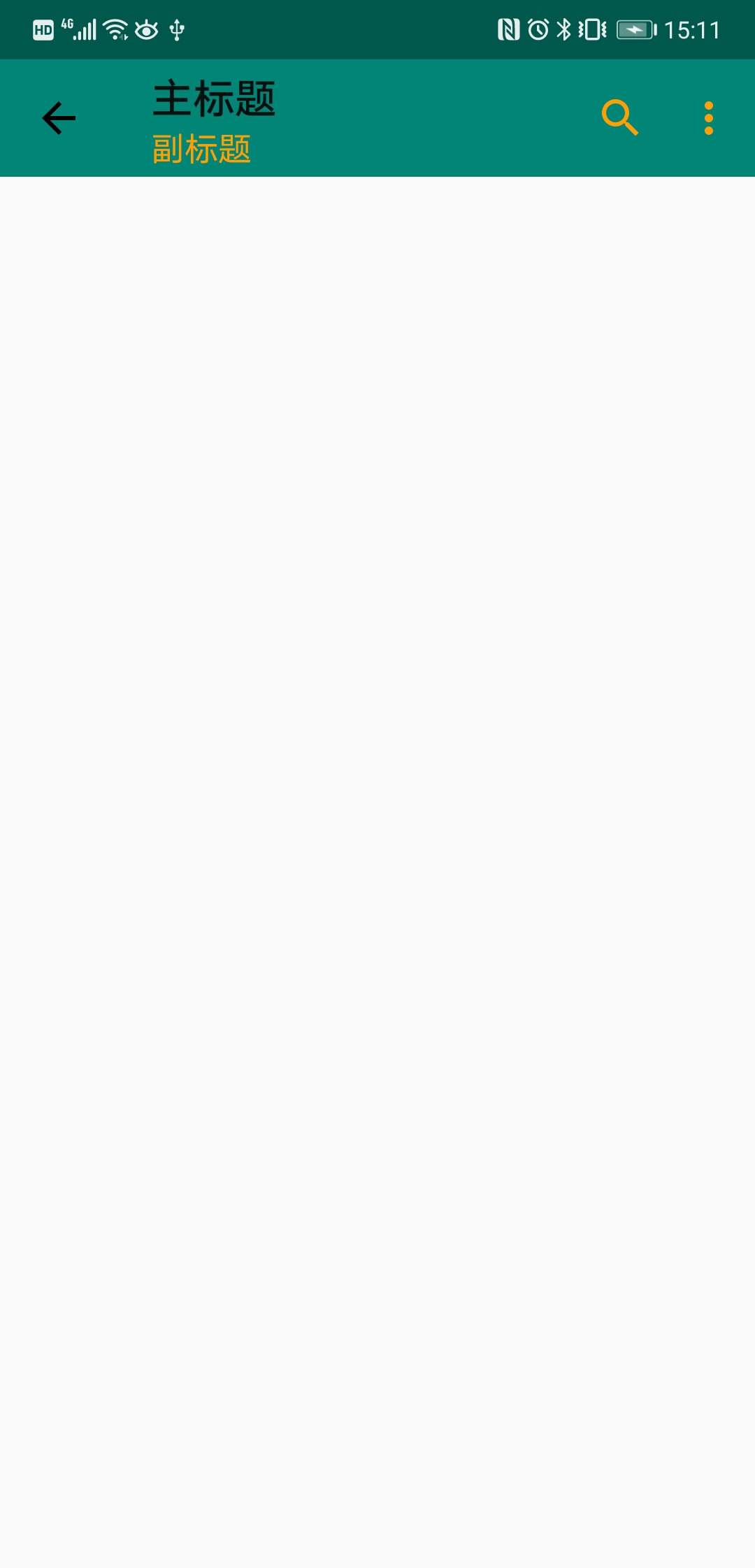
SearchView.jpg
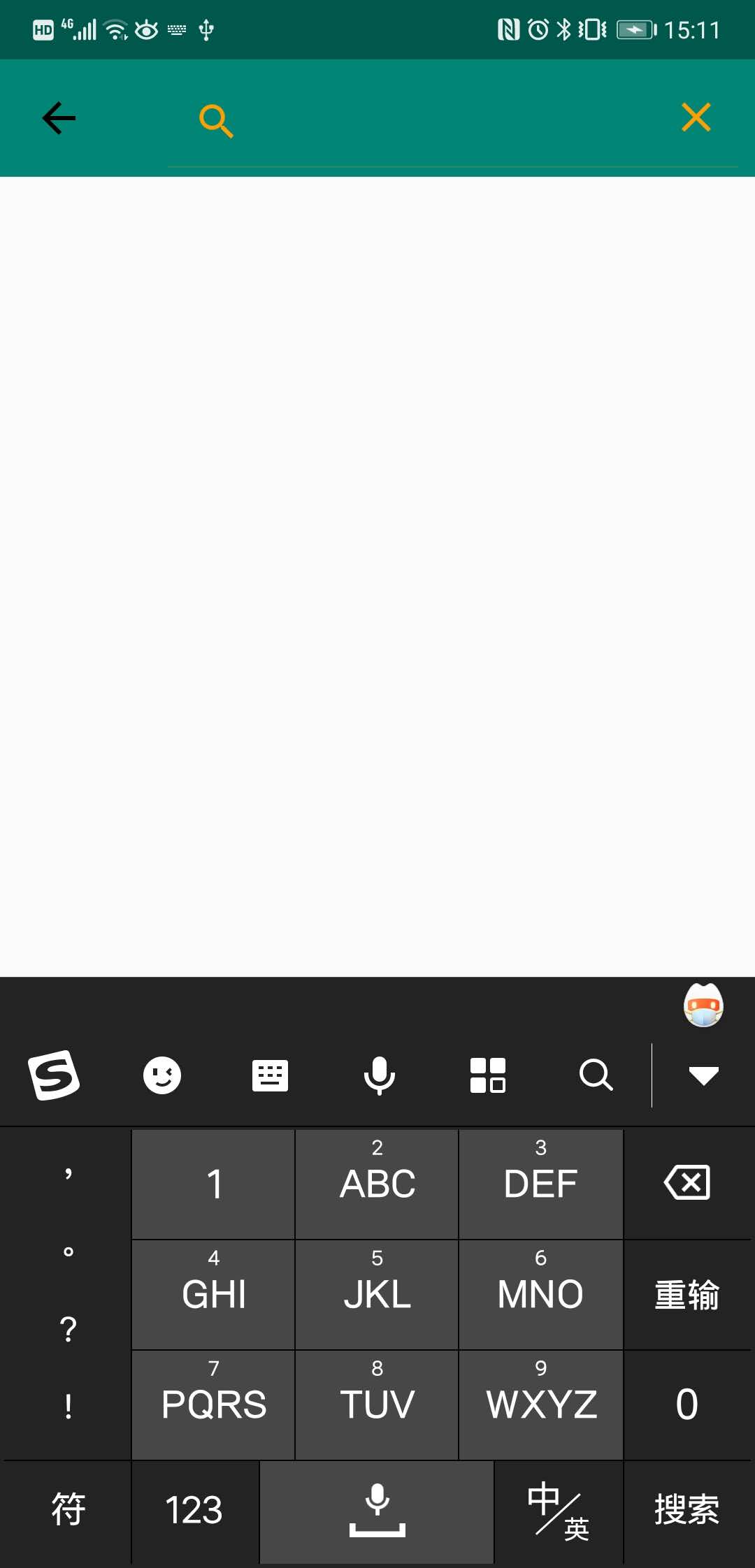
SearchView.jpg
在Activity的onCreateOptionsMenu方法中获取SearchView
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu, menu);
MenuItem menuItem = menu.findItem(R.id.searchview);
SearchView searchView;
if (Build.VERSION.SDK_INT >= 14) {//大于 v14
searchView = (SearchView) menuItem.getActionView();
} else {//小于 v14
searchView = (SearchView) MenuItemCompat.getActionView(menuItem);
}
//默认就是搜索框展开
searchView.setIconified(false);
//一直都是搜索框,搜索图标在输入框左侧(默认是内嵌的)
searchView.setIconifiedByDefault(false);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
//文字输入完成,提交的回调
@Override
public boolean onQueryTextSubmit(String s) {
return false;
}
//输入文字发生改变
@Override
public boolean onQueryTextChange(String s) {
return false;
}
});
//点击搜索图标,搜索框展开时的回调
searchView.setOnSearchClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
}
});
return super.onCreateOptionsMenu(menu);
}
如果想要修改它的控件,可以通过id查找,我们先看下SearchView的布局文件
<?xml version="1.0" encoding="utf-8"?>
<!--
/*
* Copyright (C) 2014 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
-->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/search_bar"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<!-- This is actually used for the badge icon *or* the badge label (or neither) -->
<TextView
android:id="@+id/search_badge"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center_vertical"
android:layout_marginBottom="2dip"
android:drawablePadding="0dip"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textColor="?android:attr/textColorPrimary"
android:visibility="gone" />
<ImageView
android:id="@+id/search_button"
style="?attr/actionButtonStyle"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:focusable="true"
android:contentDescription="@string/abc_searchview_description_search" />
<LinearLayout
android:id="@+id/search_edit_frame"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_marginLeft="8dip"
android:layout_marginRight="8dip"
android:orientation="horizontal"
android:layoutDirection="locale">
<ImageView
android:id="@+id/search_mag_icon"
android:layout_width="@dimen/abc_dropdownitem_icon_width"
android:layout_height="wrap_content"
android:scaleType="centerInside"
android:layout_gravity="center_vertical"
android:visibility="gone"
style="@style/RtlOverlay.Widget.AppCompat.SearchView.MagIcon" />
<!-- Inner layout contains the app icon, button(s) and EditText -->
<LinearLayout
android:id="@+id/search_plate"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:layout_gravity="center_vertical"
android:orientation="horizontal">
<view class="android.support.v7.widget.SearchView$SearchAutoComplete"
android:id="@+id/search_src_text"
android:layout_height="36dip"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_gravity="center_vertical"
android:paddingLeft="@dimen/abc_dropdownitem_text_padding_left"
android:paddingRight="@dimen/abc_dropdownitem_text_padding_right"
android:singleLine="true"
android:ellipsize="end"
android:background="@null"
android:inputType="text|textAutoComplete|textNoSuggestions"
android:imeOptions="actionSearch"
android:dropDownHeight="wrap_content"
android:dropDownAnchor="@id/search_edit_frame"
android:dropDownVerticalOffset="0dip"
android:dropDownHorizontalOffset="0dip" />
<ImageView
android:id="@+id/search_close_btn"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:paddingLeft="8dip"
android:paddingRight="8dip"
android:layout_gravity="center_vertical"
android:background="?attr/selectableItemBackgroundBorderless"
android:focusable="true"
android:contentDescription="@string/abc_searchview_description_clear" />
</LinearLayout>
<LinearLayout
android:id="@+id/submit_area"
android:orientation="horizontal"
android:layout_width="wrap_content"
android:layout_height="match_parent">
<ImageView
android:id="@+id/search_go_btn"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:paddingLeft="16dip"
android:paddingRight="16dip"
android:background="?attr/selectableItemBackgroundBorderless"
android:visibility="gone"
android:focusable="true"
android:contentDescription="@string/abc_searchview_description_submit" />
<ImageView
android:id="@+id/search_voice_btn"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:paddingLeft="16dip"
android:paddingRight="16dip"
android:background="?attr/selectableItemBackgroundBorderless"
android:visibility="gone"
android:focusable="true"
android:contentDescription="@string/abc_searchview_description_voice" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
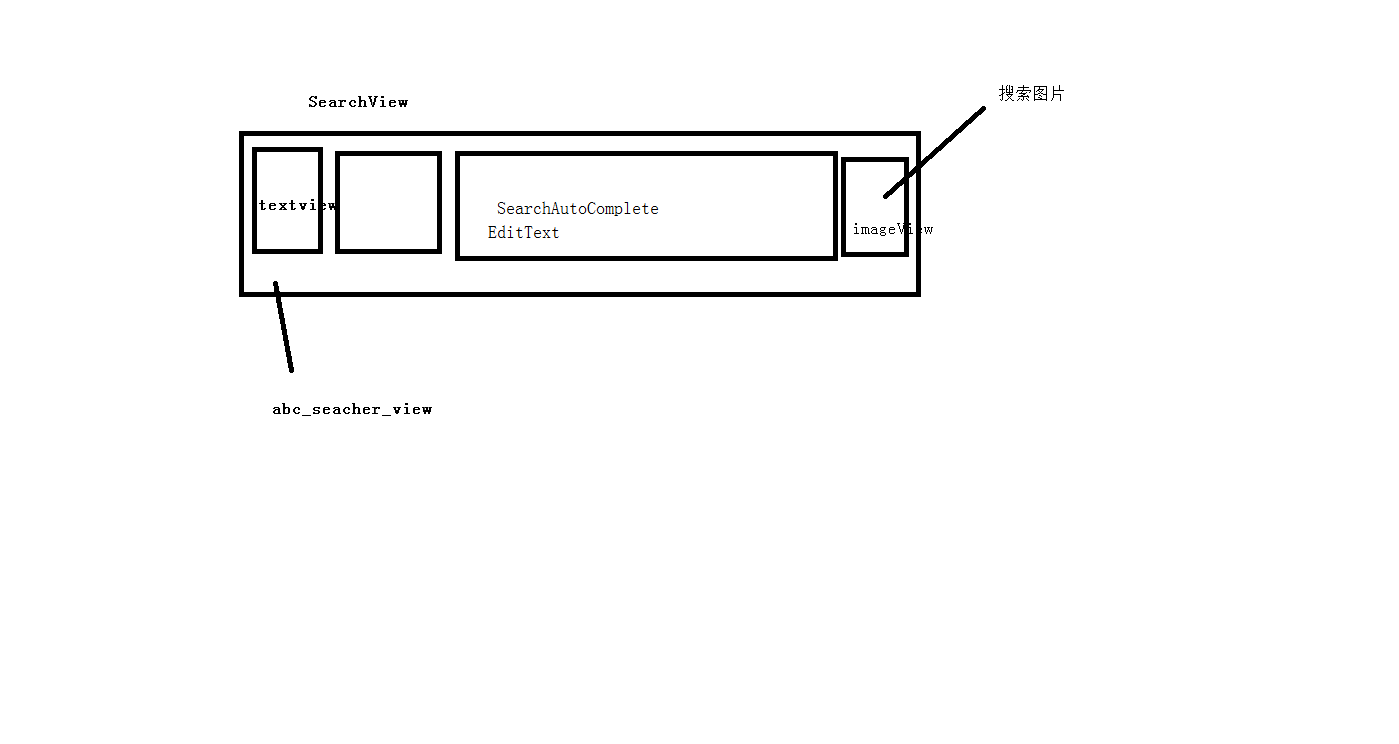
SearchView.png
通过findViewById方法,我们可以随意的修改它的控件样式,最后我们还要调用searchView.setSubmitButtonEnabled(true)才能起作用
ImageView imageView = searchView.findViewById(R.id.search_go_btn);
imageView.setVisibility(View.VISIBLE);
imageView.setImageResource(R.drawable.ic_arrow_back_black_24dp);
searchView.setSubmitButtonEnabled(true);
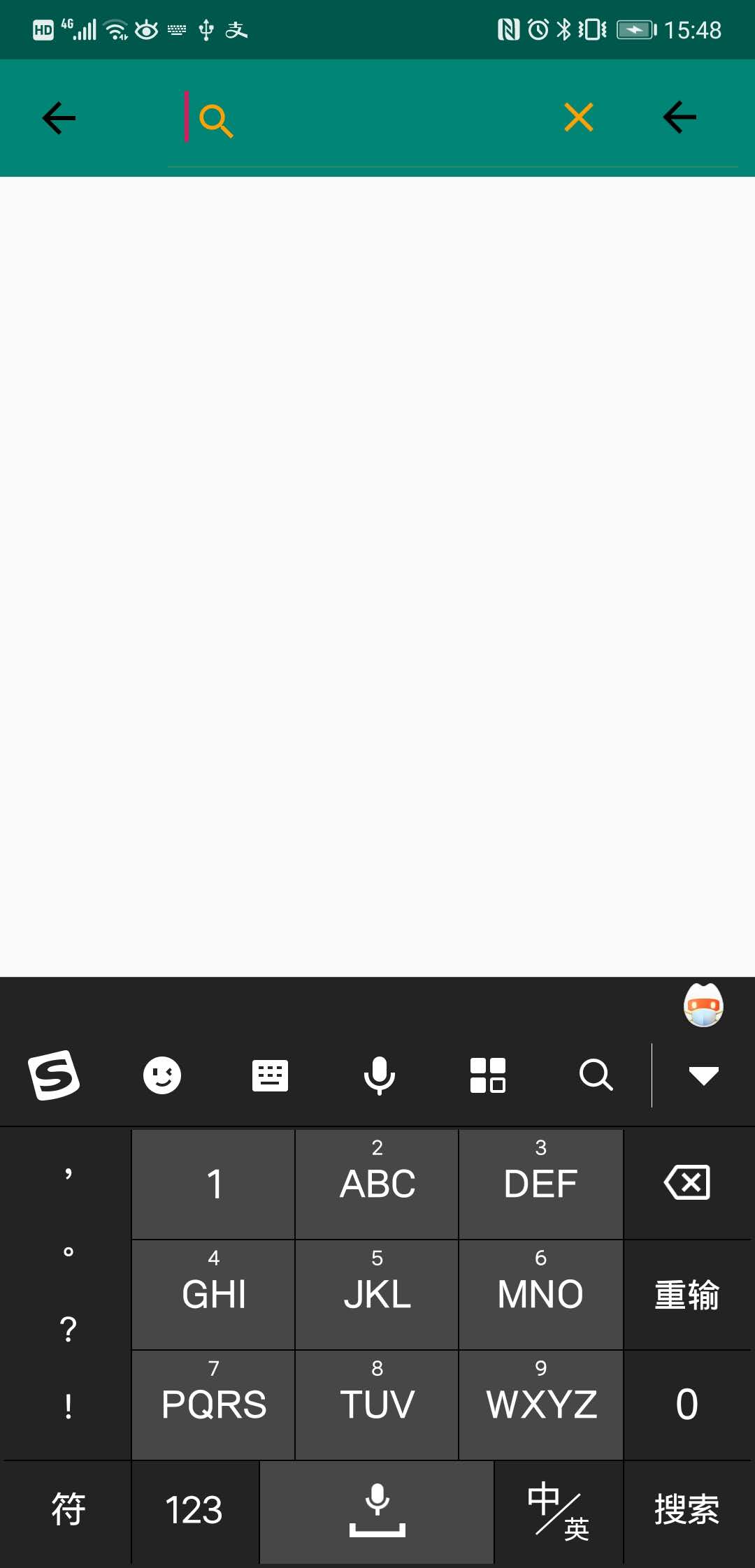
SearchView带有右侧图标.jpg
此外SearchView还带有模糊搜索的功能,它的内部有一个Adapter
CursorAdapter mSuggestionsAdapter;