#include <iostream>
#include <string>
#include <map>
std::map<std::string, int> findRepeatedSequences(const std::string& str, int minSeqLength) {
std::map<std::string, int> repeatedSequences;
for (size_t i = 0; i < str.length(); ++i) {
for (int length = minSeqLength; length <= str.length() - i; ++length) {
std::string subSequence = str.substr(i, length);
++repeatedSequences[subSequence];
}
}
// 去除重复的子序列
for (auto it = repeatedSequences.begin(); it != repeatedSequences.end();) {
if (it->second == 1) {
it = repeatedSequences.erase(it);
} else {
++it;
}
}
return repeatedSequences;
}
int main() {
std::string sequence = "ATCGATCGATCGATCG";
int minSeqLength = 2;
std::map<std::string, int> repeatedSequences = findRepeatedSequences(sequence, minSeqLength);
// 输出重复序列和重复次数
std::cout << "重复序列及其重复次数:" << std::endl;
for (const auto& pair : repeatedSequences) {
std::cout << "重复序列:" << pair.first << ", 重复次数:" << pair.second << std::endl;
}
return 0;
}
c++查找DNA重复序列和重复次数
最新推荐文章于 2024-11-14 19:11:37 发布
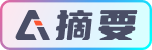