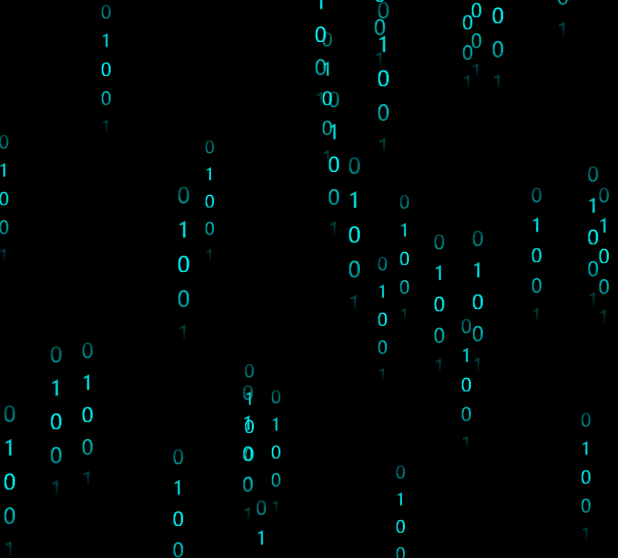
var texture_up;
this.planeUp = []
var flyShader = {
vertexshader: `
varying vec2 vUv;
varying float u_opacity;
uniform sampler2D texture;
uniform float time;
uniform float vsize;
uniform float vheight;
void main() {
vUv = vec2(uv.x,uv.y - time);
float op = (position.y + vheight / 2.0) / vheight;
u_opacity = sin( op * 3.1415);
vec4 mvPosition = modelViewMatrix * vec4(position, 1.0);
gl_Position = projectionMatrix * mvPosition;
gl_PointSize = vsize * 300.0 / (-mvPosition.z);
}`,
fragmentshader: `
varying vec2 vUv;
varying float u_opacity;
uniform sampler2D texture;
uniform vec3 vcolor;
void main() {
gl_FragColor = vec4(vcolor,u_opacity)*texture2D( texture, vUv );
}
`
}
function initContent() {
var w = 16, h = 64;
texture_up = createCanvas(w, h, '101010', 4);
texture_up.wrapS = THREE.RepeatWrapping;
texture_up.wrapT = THREE.RepeatWrapping;
for (let index = 0; index < 40; index++) {
var p = addUpPlane(w, h, texture_up, new THREE.Vector3(random(), random(), random()));
thm.scene.add(p);
thm.planeUp.push(p);
}
}
function addUpPlane(width, height, textur, position) {
textur.repeat.x = 1;
textur.repeat.y = 1;
var planeGeometry = new THREE.PlaneBufferGeometry(width, height, 1, 32);
var material = new THREE.ShaderMaterial({
uniforms: {
time: { value: 0 },
texture: { value: textur },
vsize: { value: 400 },
vcolor: { value: new THREE.Color(0.0, 1.0, 1.0) },
vheight: { value: height }
},
transparent: true,
depthTest: false,
fragmentShader: flyShader.fragmentshader,
vertexShader: flyShader.vertexshader
})
var plane_up = new THREE.Mesh(planeGeometry, material);
plane_up.rotation.x -= Math.PI / 2;
plane_up.position.copy(position);
return plane_up;
}
function createCanvas(w, h, text, dpi) {
dpi = dpi || 4;
text = String(text);
var width = w * dpi;
var height = h * dpi;
let canvas = document.createElement('canvas');
canvas.width = width;
canvas.height = height;
var ctx = canvas.getContext("2d");
ctx.fillStyle = "rgba(0,0,0,0)";
var size = width / 2;
ctx.font = size + "px 微软雅黑"
ctx.textAlign = 'left'
ctx.textBaseline = 'left'
ctx.fillStyle = '#ffffff';
for (let i = 0; i < text.length; i++) {
ctx.fillText(text[i], (width - size) / 2, i * (height / (text.length - 1)));
}
var textur = new THREE.Texture(canvas);
textur.needsUpdate = true;
return textur;
}
if (texture_up) {
texture_up.offset.y -= dt / 4;
}
thm.planeUp.forEach(function (elem) {
if (elem.material) {
elem.material.uniforms.time.value += dt / 5;
}
})