- 实现了一个栈式的导航
- 常用属性
busy : 指示StackView是否正在应用过渡动画,为true时表示正在应用动画
currentItem : 保存堆栈中当前最顶层的项目
depth : 保存当前推送到堆栈上的项目数
empty : 保存堆栈是否为空
initialItem : 保存创建StackView时应显示的初始项目,初始项目可以是Item,Component或url - 附加属性
index : 保存附加的项目的堆栈索引,-1代表该项目不在堆栈中
status : 保留其附加到的物品的堆叠状态中;1)StackView.Inactive 该项目无效(或不在堆栈中) 2)StackView.Deactivating 该项目被停用(弹出) 3)StackView.Activating 该项目正在被激活(成为当前项目) 4)StackView.Active 该项目是活动的,即当前项目
view : 保留其附加到的项目的堆栈视图,null代表该项目不在堆栈中
visible : 保留其附加到的项目的可见性 - 方法
void clear(transition):从堆栈中删除所有页面
Item find(callback, behavior):查找StackView管理的某个页面
Item get(index, behavior):返回位于堆栈中位置index处的项目
Item pop(item, operation):从堆栈中弹出一个或多个页面,出栈操作
Item push(item, properties, operation):使用指定的operation操作将页面推入堆栈,入栈操作
Item replace(target, item, properties, operation):用指定的项目item和操作operation替换堆栈中的一个或多个页面,并有选择地在该项目上应用一组属性properties - 附加信号
activated():当附件中的项目在堆栈中被激活时,将发出此附件信号
activating():当附件中的项目正在堆栈中激活时,会发出此附件信号
deactivated():当堆叠中的项目被取消激活时,将发出此附件信号
deactivating():当堆叠中的项目正在被取消激活时,将发出此附件信号
removed():当已经将推入堆栈的项目从堆栈中移出时,将发出此附着的信号
例1
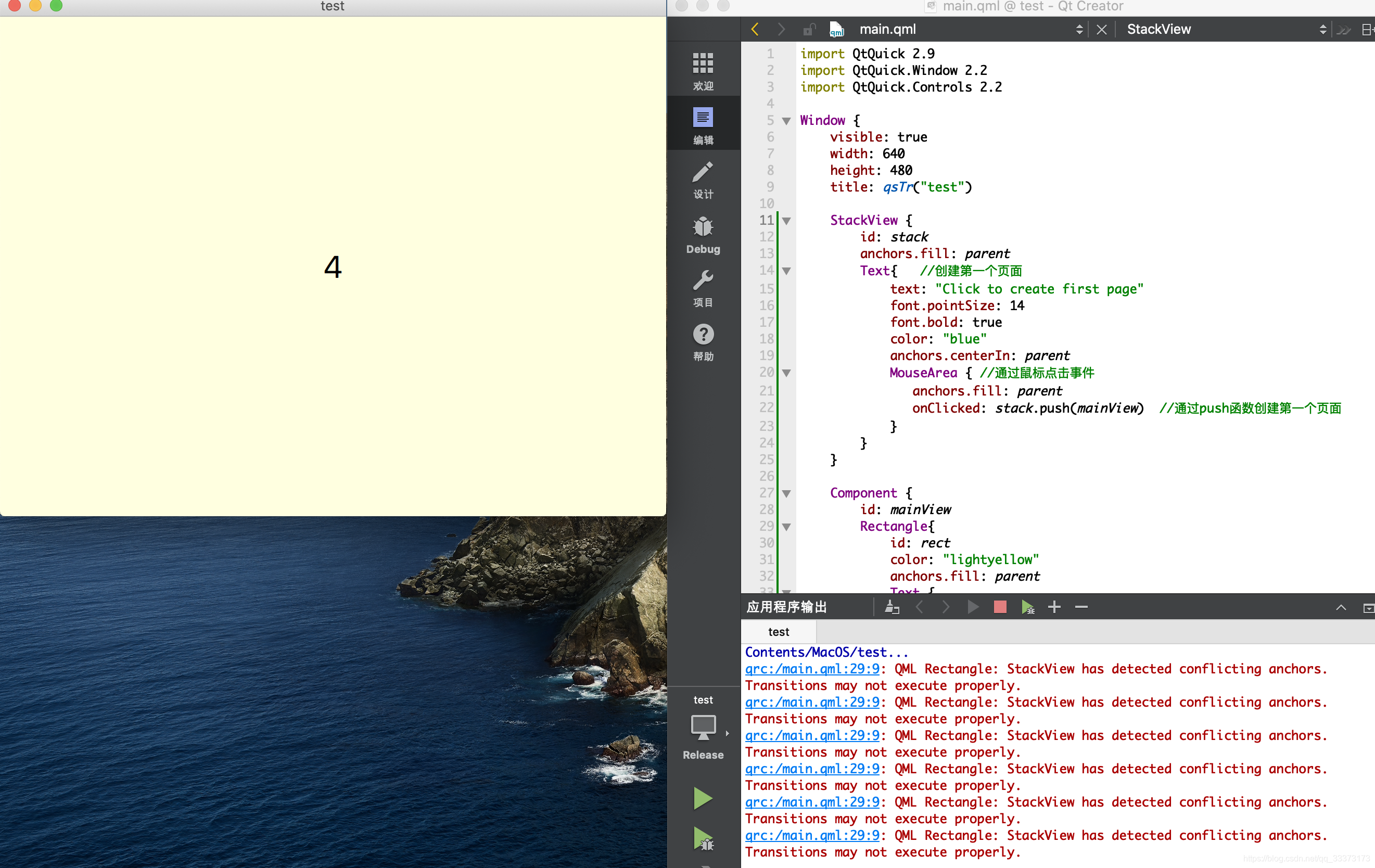
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
StackView {
id: stack
anchors.fill: parent
Text{ //创建第一个页面
text: "Click to create first page"
font.pointSize: 14
font.bold: true
color: "blue"
anchors.centerIn: parent
MouseArea { //通过鼠标点击事件
anchors.fill: parent
onClicked: stack.push(mainView) //通过push函数创建第一个页面
}
}
}
Component {
id: mainView
Rectangle{
id: rect
color: "lightyellow"
anchors.fill: parent
Text {
id: text
anchors.centerIn: parent
text: stack.depth //显示当前推送到堆栈上到项目数
font.pixelSize: 30
}
}
}
}
例2
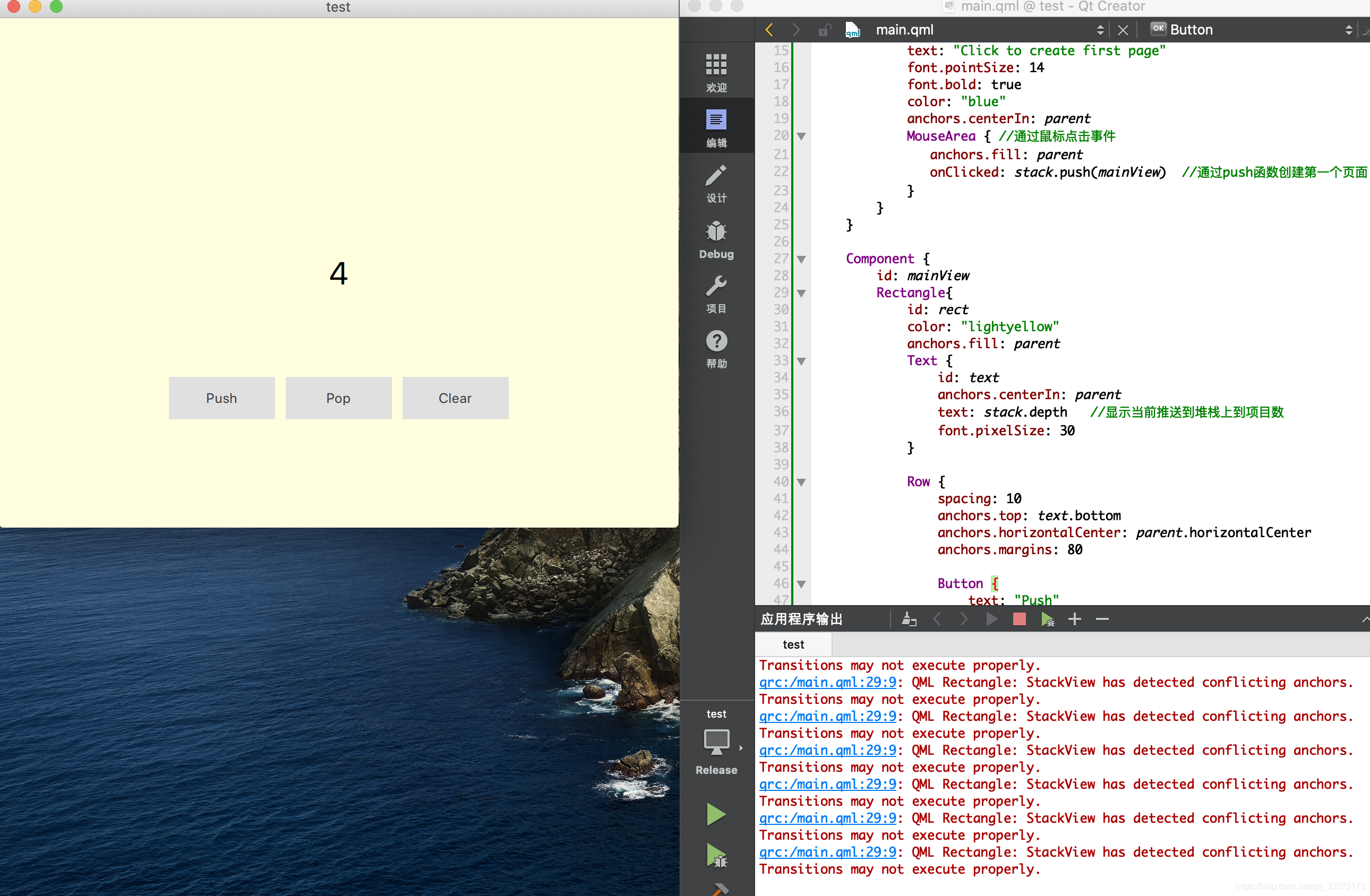
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
StackView {
id: stack
anchors.fill: parent
Text{ //创建第一个页面
text: "Click to create first page"
font.pointSize: 14
font.bold: true
color: "blue"
anchors.centerIn: parent
MouseArea { //通过鼠标点击事件
anchors.fill: parent
onClicked: stack.push(mainView) //通过push函数创建第一个页面
}
}
}
Component {
id: mainView
Rectangle{
id: rect
color: "lightyellow"
anchors.fill: parent
Text {
id: text
anchors.centerIn: parent
text: stack.depth //显示当前推送到堆栈上到项目数
font.pixelSize: 30
}
Row {
spacing: 10
anchors.top: text.bottom
anchors.horizontalCenter: parent.horizontalCenter
anchors.margins: 80
Button {
text: "Push"
onClicked: stack.push(mainView) //创建新的页面
}
Button {
text: "Pop"
enabled: stack.depth > 1
onClicked: stack.pop() //弹出当前页面 即回退到上一个页面
}
Button {
text: "Clear"
onClicked: stack.clear() //删除所以创建到页面
}
}
}
}
}
StackView扩展
- 使用stackView时不需要担心这个问题,因为stackView会接管它维护的页面对象(View)的生命周期。当我们调用pop时,那些出栈的Item,会被销毁掉
- 属性
popEnter : 此属性保存当一个页面弹出堆栈时,另一个页面进入堆栈的页面的过渡
popExit : 此属性保存当页面从堆栈中弹出时的过渡
pushEnter : 此属性保存当一个页面进入堆栈时,另一个页面弹出堆栈的页面的过渡
pushExit : 此属性保存当页面从进入堆栈时的过渡
replaceEnter : 此属性保存在替换另一个项目时进入堆栈的页面的过渡
replaceExit : 此属性保存另一个项目被替换时退出堆栈的页面的过渡。
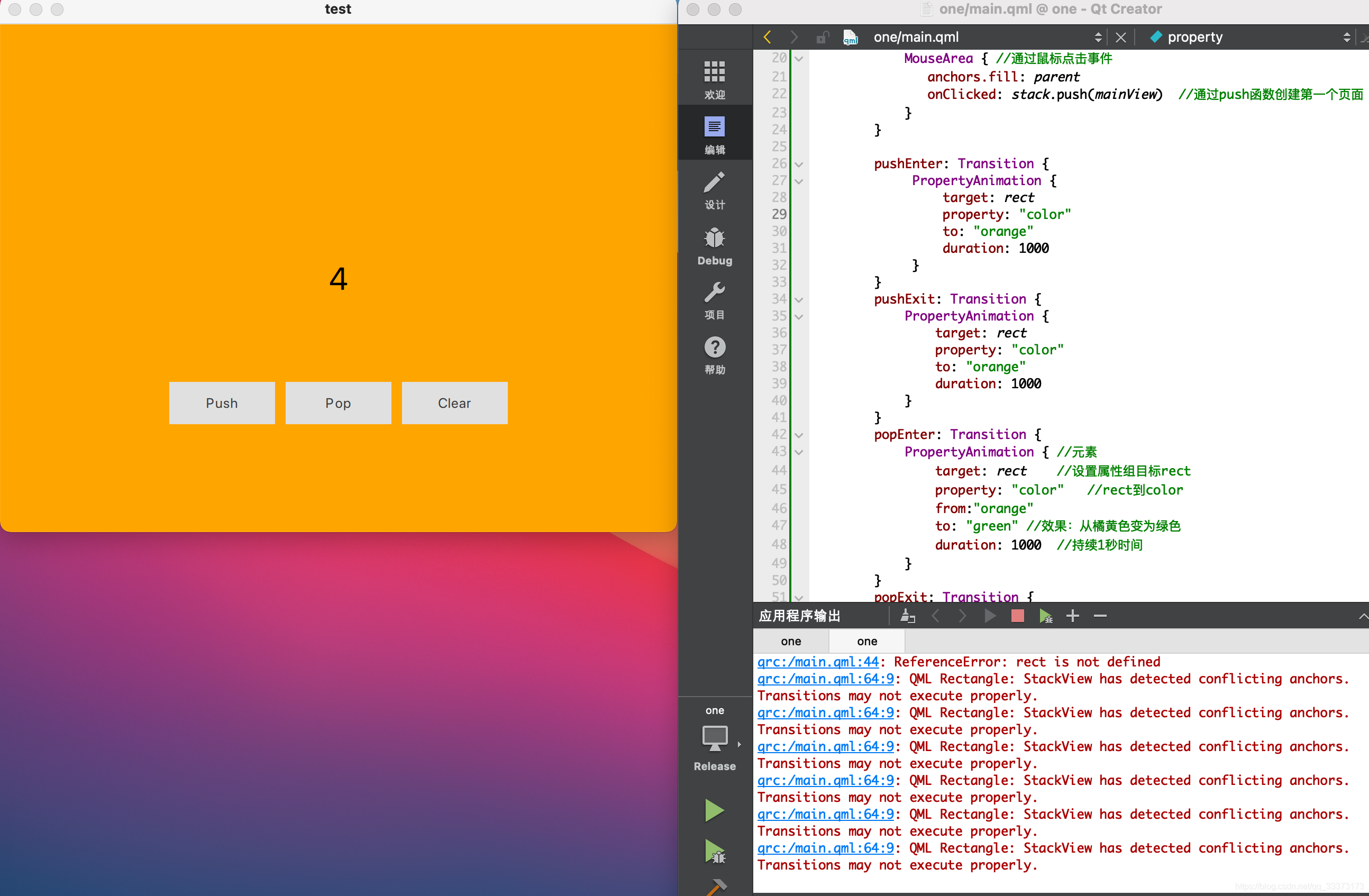
import QtQuick 2.9
import QtQuick.Window 2.2
import QtQuick.Controls 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("test")
StackView {
id: stack
anchors.fill: parent
Text{ //创建第一个页面
text: "Click to create first page"
font.pointSize: 14
font.bold: true
color: "blue"
anchors.centerIn: parent
MouseArea { //通过鼠标点击事件
anchors.fill: parent
onClicked: stack.push(mainView) //通过push函数创建第一个页面
}
}
pushEnter: Transition {
PropertyAnimation {
target: rect
property: "color"
to: "orange"
duration: 1000
}
}
pushExit: Transition {
PropertyAnimation {
target: rect
property: "color"
to: "orange"
duration: 1000
}
}
popEnter: Transition {
PropertyAnimation { //元素
target: rect //设置属性组目标rect
property: "color" //rect到color
from:"orange"
to: "green" //效果:从橘黄色变为绿色
duration: 1000 //持续1秒时间
}
}
popExit: Transition {
PropertyAnimation {
target: rect
property: "color"
from:"orange"
to: "green"
duration: 1000
}
}
}
Component {
id: mainView
Rectangle{
id: rect
color: "lightyellow"
anchors.fill: parent
Text {
id: text
anchors.centerIn: parent
text: stack.depth //显示当前推送到堆栈上到项目数
font.pixelSize: 30
}
Row {
spacing: 10
anchors.top: text.bottom
anchors.horizontalCenter: parent.horizontalCenter
anchors.margins: 80
Button {
text: "Push"
onClicked: stack.push(mainView) //创建新的页面
}
Button {
text: "Pop"
enabled: stack.depth > 1
onClicked: stack.pop() //弹出当前页面 即回退到上一个页面
}
Button {
text: "Clear"
onClicked: stack.clear() //删除所以创建到页面
}
}
}
}
}