1 WebSocketServer
@Slf4j
public class WebSocketServer {
public static void main(String[] args) {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup(2);
try {
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new WSServerInitializer());
ChannelFuture future = serverBootstrap.bind(8088).sync();
log.info("WebSocketServer starting ...");
future.channel().closeFuture().sync();
} catch (InterruptedException e) {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
e.printStackTrace();
}
}
}
2 WSServerInitializer
public class WSServerInitializer extends ChannelInitializer<NioSocketChannel> {
@Override
protected void initChannel(NioSocketChannel channel) throws Exception {
ChannelPipeline pipeline = channel.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new ChunkedWriteHandler());
pipeline.addLast(new HttpObjectAggregator(1024 * 64));
pipeline.addLast(new WebSocketServerProtocolHandler("/ws"));
pipeline.addLast(new CustomerHandler());
}
}
3 CustomerHandler
@Slf4j
public class CustomerHandler extends SimpleChannelInboundHandler<TextWebSocketFrame> {
public CustomerHandler() {
log.info("CustomerHandler invoke CustomerHandler");
}
private ChannelGroup clients = new DefaultChannelGroup(GlobalEventExecutor.INSTANCE);
@Override
protected void channelRead0(ChannelHandlerContext ctx, TextWebSocketFrame msg) throws Exception {
String text = msg.text();
log.info("接收到的数据:{}", text);
String responseMessage = "服务端接收到消息:" + text;
clients.writeAndFlush(new TextWebSocketFrame(responseMessage));
}
@Override
public void handlerAdded(ChannelHandlerContext ctx) throws Exception {
clients.add(ctx.channel());
}
@Override
public void handlerRemoved(ChannelHandlerContext ctx) throws Exception {
clients.remove(ctx.channel());
log.info("客户端:{} 已经断开", ctx.channel().id().asLongText());
}
}
4 index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Netty 实时通信</title>
</head>
<body>
发送消息:<input type="text" id="msgContent" />
<input type="button" value="发送消息" onclick="CHAT.chat()" />
<hr />
接收消息:
<div id="receiveMsg"></div>
<script type="text/javascript">
window.CHAT = {
socket: null,
init:function(){
if(window.WebSocket){
CHAT.socket = new WebSocket("ws://127.0.0.1:8088/ws");
CHAT.socket.onopen = function(){
console.log("链接建立成功");
},
CHAT.socket.close=function(){
console.log("链接关闭");
},
CHAT.socket.onerror = function(){
console.log("发生异常");
},
CHAT.socket.onmessage = function(e){
console.log("接受消息:"+e.data);
var receiveMsg = document.getElementById("receiveMsg");
var html= receiveMsg.innerHTML;
receiveMsg.innerHTML= html + "<br/>"+e.data;
}
}else{
console.log("您的浏览器不支持websocket协议");
}
},
chat:function(){
var msgContent = document.getElementById("msgContent").value;
CHAT.socket.send(msgContent);
}
};
CHAT.init();
</script>
</body>
</html>
5 运行
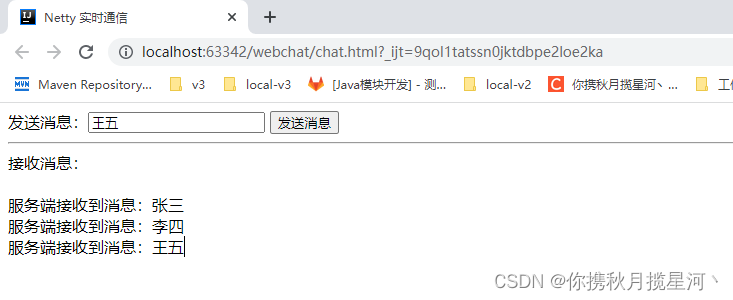