文章目录
【一】 Array(数组)
- 连续 的内存空间
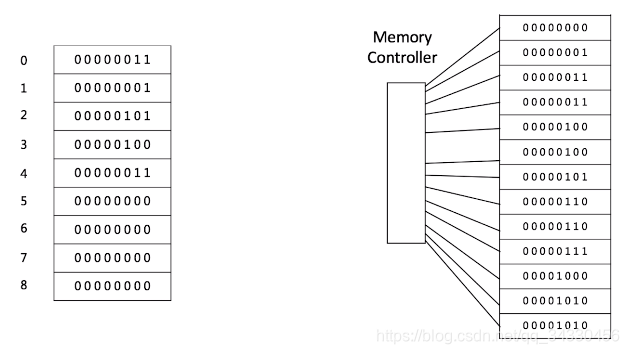
- Inserting(插入)和 Deleting(删除)
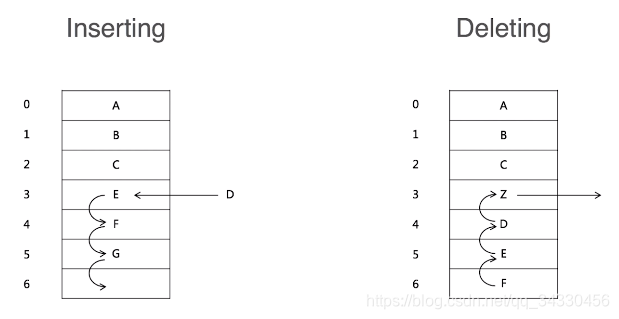
【二】 Linked List(链表)
- 单链表
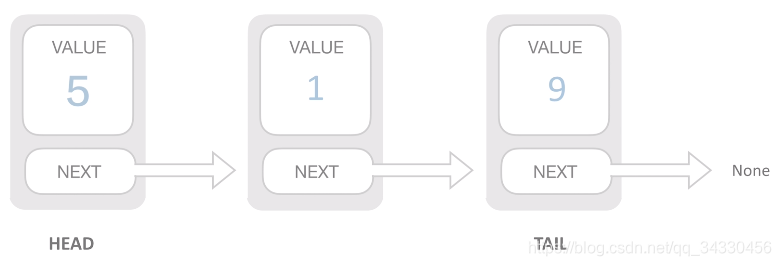
- 双链表
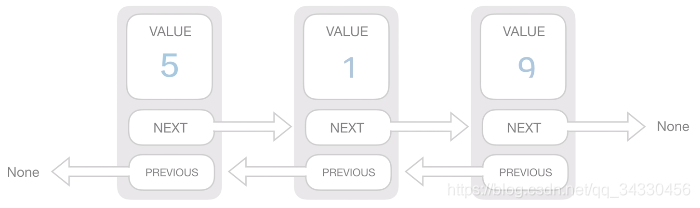
- Inserting(插入)
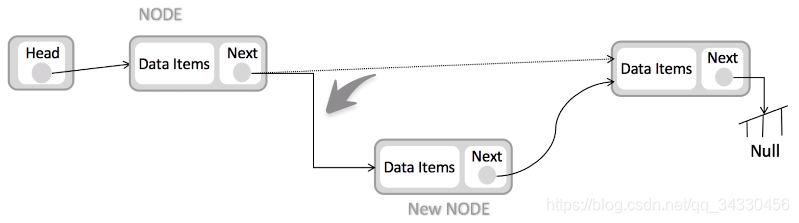
Deleting(删除)
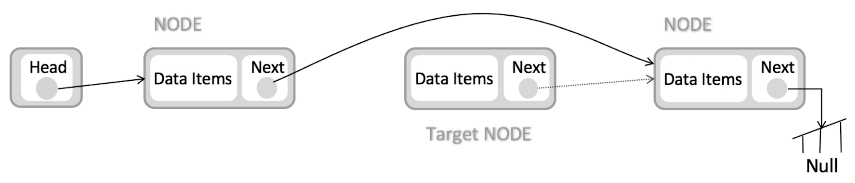
【三】 Interview(面试题)
【3.1】 LeetCode 206:Reverse Linked List(反转链表)
Input: 1->2->3->4->5->NULL
Output: 5->4->3->2->1->NULL
# Python(迭代)
class Solution:
def reverseList(self, head: ListNode) -> ListNode:
# 定义两个指针,不断后移
cur, newHead = head, None
while cur:
# 先改变指向,再向后移动指针
cur.next, newHead , cur = newHead , cur, cur.next
return newHead
# Python(递归)
class Solution:
def reverseList(self, head: ListNode) -> ListNode:
# head 指向空 或者 指向最后一个结点 都直接返回
if not head or not head.next:
return head
# 这里递归要用到 self
newHead = self.reverseList(head.next)
head.next.next = head
head.next = None
return newHead
// c++(迭代)
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* newHead = NULL;
while(head){
ListNode* t = head->next;
head->next = newHead; // 改变指向
newHead = head; head = t; // 向后移动指针
}
return newHead;
}
};
// c++(递归)
class Solution {
public:
ListNode* reverseList(ListNode* head) {
// head 指向空 或者 指向最后一个结点 都直接返回
if(!head || !head->next) return head;
// 递归调用
ListNode* newHead = reverseList(head->next);
// 参考 https://blog.csdn.net/qq_37117521/article/details/80808631 图解
head->next->next = head;
head->next = NULL;
return newHead;
}
};
【3.2】 LeetCode 24:Swap Nodes in Pairs(两两交换链表中的节点)
Input: 1->2->3->4
Output: 2->1->4->3
# Python(迭代)
class Solution:
def swapPairs(self, head: ListNode) -> ListNode:
pre, pre.next = self, head
while pre.next and pre.next.next:
a = pre.next;
b = a.next;
pre.next, b.next, a.next = b, a, b.next
pre = a
return self.next
# Python(递归)
class Solution:
def swapPairs(self, head: ListNode) -> ListNode:
if not head or not head.next:
return head
newHead = head.next
head.next = self.swapPairs(head.next.next)
newHead.next = head
return newHead
// c++(迭代)
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
ListNode* dummy = new ListNode(-1), *pre = dummy;
dummy->next = head; // dummy 相当于 python 中的 self
while(pre->next && pre->next->next){
ListNode* t = pre->next->next; // t 标志第二个位置 B
pre->next->next = t->next; // A -> C
t->next = pre->next; // B -> A
pre->next = t; // pre -> B
pre = t->next; // 更新 pre = A
}
return dummy->next;
}
};
// c++(递归)
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
if(!head || !head->next) return head;
ListNode* t = head->next;
head->next = swapPairs(head->next->next);
t->next = head;
return t;
}
};
【3.3】 LeetCode 141:Linked List Cycle(单链表中的环)
① 硬做,设置一个时间阈值,判断在规定的时间内是否能到达NULL节点(性能差)
② set 数据结构存储节点的 地址,每次遍历链表时判断当前节点的地址是否存储在 set 内(O(n))
③ 快慢指针,龟兔赛跑,设置两个指针,快的每次走两步,慢的每次走一步,若最终快慢指针相遇,则有环(O(n))
# Python(快慢指针)
class Solution(object):
def hasCycle(self, head):
slow = fast = head
while slow and fast and fast.next:
slow = slow.next;
fast = fast.next.next;
if slow is fast:
return True
return False
// c++(快慢指针)
class Solution {
public:
bool hasCycle(ListNode *head) {
// 快慢指针
ListNode* slow = head, *fast = head;
while(fast && fast->next){
slow = slow->next;
fast = fast->next->next;
if(slow == fast) return true;
}
return false;
}
};
【3.4】 LeetCode 148:单链表排序( O ( N l o g N ) O(NlogN) O(NlogN))
Input: 4->2->1->3
Output: 1->2->3->4
Input: -1->5->3->4->0
Output: -1->0->3->4->5
# Python(归并法)
class Solution:
def sortList(self, head):
# 判断头结点并返回
if head is None or head.next is None:
return head
# 快慢指针实现将原链表分半
pre, slow, fast = head
while fast is not None and fast.next is not None:
pre = slow
slow = slow.next
fast = fast.next.next
pre.next = None
# 合并
return self.merge(self.sortList(head), self.sortList(slow))
# 合并过程
def merge(self, left, right):
# 判空
if left is None:
return right
if right is None:
return left
# 判断大小
if left.val <= right.val:
left.next = self.merge(left.next, right)
return left
else:
right.next = self.merge(left, right.next)
return right