链表设计
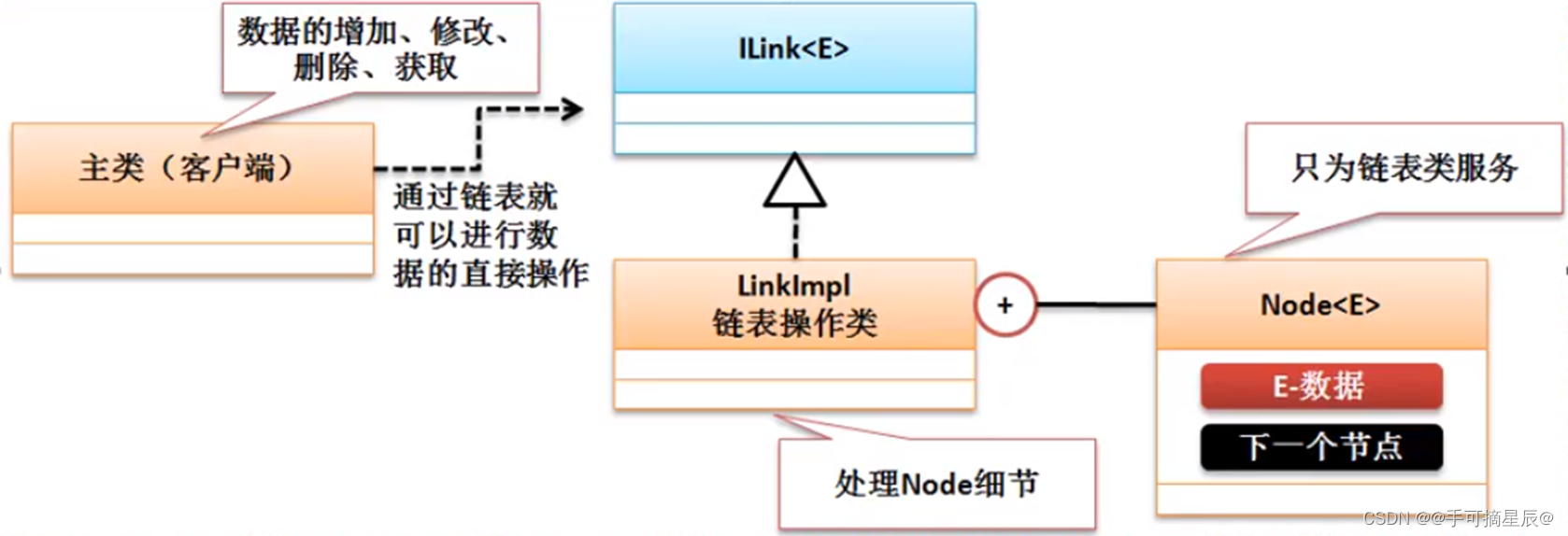
package test;
interface ILink<E> {
public void add(E e);
public int size();
public boolean isEmpty();
public Object[] toArray();
public E get(int index);
public void set(int index ,E data);
public boolean contains(E data);
public void remove(E data);
public void clean();
}
class LinkImpl<E> implements ILink<E>{
private class Node{
private E data;
private Node next;
public Node (E data) {
this.data=data;
}
public void addNode(Node newNode) {
if(this.next==null) {
this.next=newNode;
}else {
this.next.addNode(newNode);
}
}
public void toArrayNode() {
LinkImpl.this.returnData[LinkImpl.this.foot++]=this.data;
if (this.next !=null) {
this.next.toArrayNode();
}
}
public E getNode(int index) {
if(LinkImpl.this.foot++==index) {
return this.data;
}else {
return this.next.getNode(index);
}
}
public void setNode(int index ,E data) {
if(LinkImpl.this.foot++==index) {
this.data=data;
}else {
this.next.setNode(index,data);
}
}
public boolean containsNode(E data) {
if (this.data.equals(data)) {
return true;
}else {
if(this.next==null) {
return false;
}else {
return this.next.containsNode(data);
}
}
}
public void removeNode(Node previous,E data) {
if(this.data.equals(data)) {
previous.next=this.next;
}else {
if(this.next!=null) {
this.next.removeNode(this, data);
}
}
}
}
private Node root;
private int count;
private int foot;
private Object[] returnData;
public void add(E e)
{
if(e==null)
{
return;
}
Node newNode=new Node(e);
if (this.root==null)
{
this.root=newNode;
}
else
{
this.root.addNode(newNode);
}
this.count++;
}
public int size() {
return this.count;
}
public boolean isEmpty()
{
return this.root==null;
}
public Object[] toArray() {
if(this.isEmpty()) {
return null;
}
this.foot=0;
this.returnData=new Object [this.count];
this.root.toArrayNode();
return this.returnData;
}
public E get(int index) {
if(index>=this.count) {
return null;
}
this.foot=0;
return this.root.getNode(index);
}
public void set(int index ,E data) {
if(index>=this.count) {
return ;
}
this.foot=0;
this.root.setNode(index,data);
}
public boolean contains(E data) {
if(data==null) {
return false;
}
return this.root.containsNode(data);
}
public void remove(E data) {
if(this.contains(data)) {
if(this.root.data.equals(data)) {
this.root=this.root.next;
}else {
this.root.next.removeNode(this.root, data);
}
this.count--;
}
}
public void clean() {
this.root=null;
this.count=0;
}
}
interface Pet{//定义宠物标准
public String getName();
public String getColor();
}
class PetShop{//定义宠物商店功能
private ILink<Pet> allPets=new LinkImpl<Pet>();
public void add(Pet pet) {
this.allPets.add(pet);
}
public void delete(Pet pet) {
this.allPets.remove(pet);
}
public ILink<Pet> search(String keyword){
ILink<Pet> searchResult=new LinkImpl<Pet>();
Object result []=this.allPets.toArray();
if(result !=null) {
for(Object obj:result) {
Pet pet=(Pet) obj;
if(pet.getName().contains(keyword)||pet.getColor().contains(keyword))
{
searchResult.add(pet);
}
}
}
return searchResult;
}
}
class Fish implements Pet{
private String name;
private String color;
public Fish(String name,String color)
{
this.name=name;
this.color=color;
}
public String getName() {
return this.name;
}
public String getColor()
{
return this.color;
}
public boolean equals(Object obj) {
if(obj==null){
return false;
}
if(!(obj instanceof Fish)) {
return false;
}
if(this==obj) {
return true;
}
Fish fish=(Fish)obj;
return this.name.equals(fish.name) && this.color.equals(fish.color);
}
public String toString()
{
return "宠物鱼的名字:"+this.name+" 颜色:"+this.color;
}
}
class Cat implements Pet{
private String name;
private String color;
public Cat(String name,String color)
{
this.name=name;
this.color=color;
}
public String getName() {
return this.name;
}
public String getColor()
{
return this.color;
}
public boolean equals(Object obj) {
if(obj==null){
return false;
}
if(!(obj instanceof Cat)) {
return false;
}
if(this==obj) {
return true;
}
Cat cat=(Cat)obj;
return this.name.equals(cat.name) && this.color.equals(cat.color);
}
public String toString()
{
return "宠物猫的名字:"+this.name+" 颜色:"+this.color;
}
}
public class tty {
public static void main(String[] args) {
// TODO Auto-generated method stub
PetShop shop=new PetShop();
System.out.println("*********上架商品:\"小鱼\",\"黄色\"\"淡黄猫\",\"淡黄色\"\"黑猫\",\"黑色\"深黄猫\",\"深黄色\"\"草鱼\",\"褐色\"*******");
Fish fishyd=new Fish("小鱼","黄色");
shop.add(new Cat("淡黄猫","淡黄色"));//上架
shop.add(new Cat("黑猫","黑色"));
shop.add(new Cat("深黄猫","深黄色"));
shop.add(new Fish("草鱼","褐色"));
shop.add(fishyd);
System.out.println("*********根据黄色查询*******");
Object result []=shop.search("黄").toArray();//查询
for (Object obj:result) {
System.out.println(obj);
}
System.out.println("***开始下架商品:黄色小鱼*******");
shop.delete(fishyd);//下架
System.out.println("***********再次查询********");
Object resultonce []=shop.search("黄").toArray();//查询
for (Object obj:resultonce) {
System.out.println(obj);
}
}
}