C++ STL算法基础与迭代器 day16
C++迭代器
- 迭代器是一个类中类,通过实现运算符重载实现对象模仿指针的行为对容器进行遍历
- 迭代器类型分为:
- 正向迭代器:容器名::iterator iter
- 反向迭代器:容器名::reverse_iterator iter
- 常正向迭代器:容器名::const_iterator iter
- 常反向迭代器:容器名::const_reverse_iteraor iter
- 迭代器功能分为:
容器 | 迭代器类型 |
---|
array,vector,deque | 随机 |
list,set,mutiset,map,multiset | 双向 |
stack,queue,priority_queue | 不支持 |
void advance(_InIt& _Where, _Diff _Off);
_Iter_diff_t<_InIt> distance(_InIt _First, _InIt _Last);
void iter_swap(_FwdIt1 _Left, _FwdIt2 _Right)
#include <iostream>
#include <vector>
#include <list>
int main()
{
std::list<int> myList = { 1,2,3,4,5,7 };
std::vector<int> vec = { 1,2,3,4,5,6 };
auto iterList = myList.begin();
std::advance(iterList, 3);
std::cout << *iterList << std::endl;
auto iterVec = vec.begin() + 3;
std::cout << *iterVec << std::endl;
std::cout << std::distance(vec.begin(), vec.end()) << std::endl;
iter_swap(vec.begin(), vec.begin() + 3);
for (auto v : vec)
{
std::cout << v << " ";
}
return 0;
}
- 运行结果
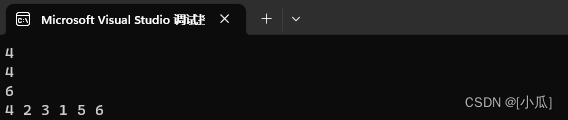
- 流型迭代器
- 输入流迭代器
- istream_iteraot<type> object EOF流
- istream_iteraot<type> object(istream& in);
- *object:等效于cin>>
- 输出流迭代器
- ostream_iteraot<type> object(ostream& out)
- ostream_iteraot<type> object(ostream& out,const char* str);
- object=value; 等效于把数据打印到屏幕上 cout<<valure
#include <iostream>
#include <vector>
#include <list>
#include <algorithm>
#include <functional>
int main()
{
std::vector<int> vec = { 1,2,3,4,5 };
std::ostream_iterator<int> object(std::cout);
object = 123;
std::cout << std::endl;
for (auto v : vec)
{
object = v;
}
std::cout << std::endl;
std::ostream_iterator<int> object2(std::cout, " ");
for (auto v : vec)
{
object2 = v;
}
std::cout << std::endl;
std::copy(vec.begin(), vec.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::cout << "不输入数字就会结束输入:" << std::endl;
std::istream_iterator<int> Eof;
std::istream_iterator<int> in(std::cin);
std::vector<int> input;
while (in != Eof)
{
input.push_back(*in);
in++;
}
for (auto v : input)
{
std::cout << v << " ";
}
std::cout << std::endl;
return 0;
}
- 运行结果
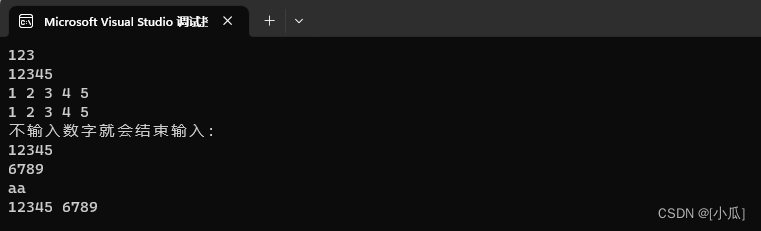
C++仿函数
- 仿函数就是类模仿函数的行为
- 仿函数重点在于重载()运算符
- 通常仿函数大部分情况都是比较准则
#include <iostream>
#include <vector>
#include <list>
#include <algorithm>
#include <functional>
struct Sum
{
int operator()(const int& a, const int& b)
{
return a + b;
}
};
void testFun()
{
Sum object;
std::cout << object.operator()(1, 2) << std::endl;
std::cout << object(1, 2) << std::endl;
std::cout << Sum{}(1, 2) << std::endl;
}
namespace mySort
{
template<typename _Ty,typename _Pr>
void sort(_Ty arr[], int size)
{
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size - i - 1; j++)
{
if (_Pr{}(arr[j], arr[j + 1]) == false)
{
_Ty temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
template<typename _Ty>
struct less
{
bool operator()(const _Ty& a, const _Ty& b) const
{
return a < b;
}
};
template<typename _Ty>
struct greater
{
bool operator()(const _Ty& a, const _Ty& b) const
{
return a > b;
}
};
}
int main()
{
testFun();
int arr[6] = { 1,3,5,6,2,4 };
mySort::sort<int, mySort::less<int>>(arr, 6);
for (auto v : arr)
{
std::cout << v << " ";
}
std::cout << std::endl;
mySort::sort<int, mySort::greater<int>>(arr, 6);
for (auto v : arr)
{
std::cout << v << " ";
}
std::cout << std::endl;
std::sort(arr, arr + 6, std::less<int>());
for (auto v : arr)
{
std::cout << v << " ";
}
std::cout << std::endl;
std::sort(arr, arr + 6, std::greater<int>());
for (auto v : arr)
{
std::cout << v << " ";
}
std::cout << std::endl;
return 0;
}
- 运行结果
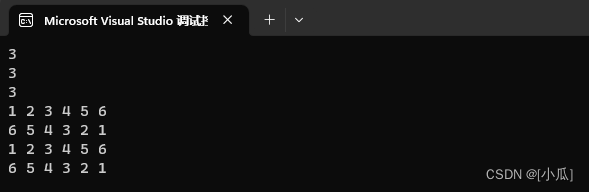
- 标准库中的仿函数特别多,这里就少例举一些
#include <iostream>
#include <set>
#include <functional>
#include <algorithm>
void stdFunctional()
{
std::cout << std::plus<int>{}(1, 2) << std::endl;
std::cout << std::greater_equal<int>{}(1, 2) << std::endl;
std::cout << std::logical_and<int>{}(1, 0) << std::endl;
std::cout << std::bit_and<int>{}(1, 1) << std::endl;
}
int main()
{
stdFunctional();
return 0;
}
- 运行结果
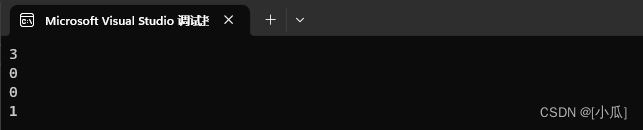
C++函数包装器
- 函数包装器就是把函数指针包装成为一个对象,通过运算符重载实现对象去调用函数
- 包装方法
- function <函数返回值类型(参数类型)> object(函数指针);
- 使用方法
#include <iostream>
#include <algorithm>
#include <functional>
int sum(int a, int b)
{
return a + b;
}
class Fun
{
public:
int operator()(int a,int b)
{
return a + b;
}
static void product(int a, int b, int c)
{
std::cout << a * b * c << std::endl;
}
using PFUNC = void(*)(int, int, int);
operator PFUNC()
{
return product;
}
};
void testFunction()
{
std::function<int(int, int)> pSum(sum);
std::cout << pSum(1, 2) << std::endl;
Fun fun;
std::function<int(int, int)> func = fun;
std::cout << func(1, 2) << std::endl;
int(Fun:: * pFunc)(int, int) = &Fun::operator();
std::cout << (fun.*pFunc)(1, 2) << std::endl;
std::function<void(int, int, int)> func2 = fun;
func2(1, 2, 3);
}
int main()
{
testFunction();
return 0;
}
- 运行结果
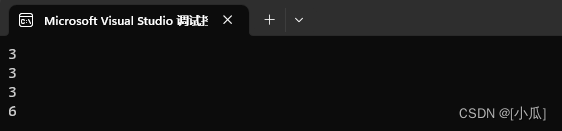
C++函数适配器
- 函数适配器就是绑定默认参数,实现函数指针特定调用方法,一般是为了适应别人提供的接口
#include <iostream>
#include <functional>
void print(int a, int b, int c)
{
std::cout << a << " " << b << " " << c << std::endl;
}
template <typename _Pr>
void printData(_Pr pr, int a, int b)
{
pr(a, b);
}
void Bind()
{
auto pFunc = std::bind(print, std::placeholders::_1, std::placeholders::_2, 0);
print(1, 2, 3);
pFunc(1, 2);
pFunc(1,2,3);
printData(pFunc, 1, 2);
}
int main()
{
Bind();
return 0;
}
- 运行结果
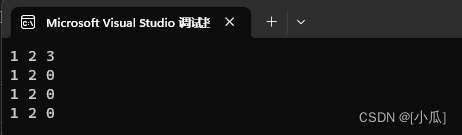
#include <iostream>
#include <functional>
#include <vector>
#include <algorithm>
template <typename _Pr>
void printTest(_Pr pr, int a, int b)
{
pr(a, b);
}
class student
{
public:
void printData(int a, int b, int c)
{
std::cout << a << " " << b << " " << c << std::endl;
}
};
void testBind()
{
student xiaogua;
void(student:: *p1)(int, int, int) = &student::printData;
(xiaogua.*p1)(1, 2, 3);
auto p2 = std::bind(&student::printData, &xiaogua,std::placeholders::_1,2,0);
p2(1, 2, 3);
auto p3 = std::bind(&student::printData, &xiaogua, std::placeholders::_1,
std::placeholders::_2, 0);
printTest(p3, 2, 3);
}
void testCount()
{
std::vector<int> score{ 99,98,97,96,59 };
std::cout << "及格人数:" << std::count_if(score.begin(), score.end(),
[](int& data) {return data >= 60; }) << std::endl;
std::cout << "及格人数:" << std::count_if(score.begin(), score.end(),
std::bind(std::greater_equal<int>(), std::placeholders::_1, 60)) << std::endl;
}
int main()
{
testBind();
testCount();
return 0;
}
- 运行结果
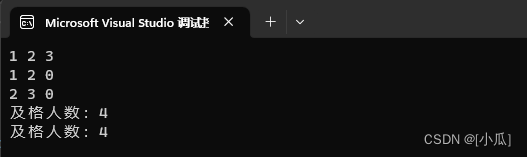
适配器与包装器混合使用
#include <iostream>
#include <functional>
#include <vector>
#include <algorithm>
class student
{
public:
};
void print(int a, std::string b, student object)
{
std::cout << __FUNCDNAME__ << std::endl;
}
void test()
{
auto p1 = std::bind(print,
std::placeholders::_2,
std::placeholders::_3,
std::placeholders::_1);
student xiaogua;
p1(xiaogua, 21, "小瓜");
std::function<void(student, int, std::string)> pFunc = p1;
pFunc(xiaogua, 21, "大瓜");
}
int main()
{
test();
return 0;
}