this指针:
是一个特殊的指针,放回这个对象本身,this指针是属于实例对象,不能访问静态方法(不属于某一个实例对象,属于共有的,大众的,由类直接调用)
第一种用法:
void Human::setName(string name1) {
this->name = name1;
}
void Human::setAge(int age1) {
this->age = age1;
}
void Human::setSalary(int salary1) {
this->salary = salary1;
}
第二三种用法:引用和指针
//this指针的用法两种
const Human* comBetterMan(const Human*);
const Human& comBetterMan1(const Human&);
/*
//当返回对象是指针时
const Human *Human::comBetterMan(const Human *other) {
if (this->getSalary() >other->getSalary()) {
return this;
}
else {
return other;
}
}
//当返回对象是引用时
const Human& Human::comBetterMan1(const Human&other) {
if (this->getSalary() > other.getSalary()) {
return *this;
}
else {
return other;
}
}
*/
int main(void) {
//自定义一个带参的构造函数
Human h1("张三", 24, 25000);
Human h2("李四", 40, 50000);
cout << "用指针返回的是:" << h1.comBetterMan(&h2) << endl;
cout << "用引用返回的是:" << &h1.comBetterMan1(h2) << endl;
return 0;
}
类文件的分离
分为定义(.h的文件中)和实现(.cpp文件中)两个部分和一个主函数(main)文件.cpp
.h中
#pragma once
#include <iostream>
#include <string>
using namespace std;
class Human {
public://公有的,对外的
void eat();//方法,成员函数
void sleep();
void play();
void work();
void descript();
//自定义的默认构造函数
Human();
~Human();
//手动定义带参的(重载)构造函数
Human(string name, int age, int salary);
//手动定义拷贝构造函
Human(const Human& other);
//手动定义赋值构造函数,
// 运算符重载"operator ="
Human& operator= (const Human& other1);
void setName(string name);//设置对内的数据
void setAge(int age);
void setSalary(int salary);
void setAddr(char* addr);
//this指针的用法两种
const Human* comBetterMan(const Human*);
const Human& comBetterMan1(const Human&);
string getName()const;//获取对内的数据
int getAge()const;
int getSalary()const;
char* getAddr() const;
private://对内的,私有的
string name;
int age;//设置类内初始值
int salary;
char* addr;
};
实现 .cpp中代码
#include "Human.h"
#define size 16
string Human::getName() const {
return name;
}
int Human::getAge() const {
return age;
}
int Human::getSalary() const {
return salary;
}
char* Human::getAddr() const
{
return addr;
}
//手动定义的默认构造函数
Human::Human() {
cout << "手动定义的默认构造函数" << this << endl;
name = "张三";
age = 23;
salary = 25000;
addr = new char[32];
strcpy_s(addr, size, "Amecrican");
}
//自定义重载构造函数
Human::Human(string name, int age, int salary) {
cout << "调用自定义的带参构造函数" << this << endl;
this->age = age;
this->name = name;
this->salary = salary;
addr = new char[32];
strcpy_s(addr, size, "China");
}
//相当于 Human h2 = h1; //const Human &other = h1;
Human::Human(const Human& other) {
cout << "调用拷贝构造函数" << endl;
this->age = other.age;
this->name = other.name;
this->salary = other.salary;
//为addr分配一块独立的内存空间,不进行浅拷贝
addr = new char[32];
strcpy_s(addr, size, other.addr);//设置值为深拷贝
}
//如果时引用的话,是不会创建新的对象
//void type2(const Human& h2) {//相当于别名
// cout << "拷贝构造函数作为函数的参数,是引用类型" << endl;
// //h2.setAddr("芜湖");//可以修改值,定义为const就不能修改了
// cout << "name: " << h2.getName()
// << "age: " << h2.getAge()
// << "salary: " << h2.getSalary()
// << "addr: " << h2.getAddr() << endl;
//}
//函数的返回类型是类,而非引用类型,,参数是对象,而非引用类型
//这个函数会返回一个临时的对象,这个对象会调用拷贝构造函数,加上参数的两个,这里一共会调用三次拷贝构造函数
//这里可以将参数设置为引用类型,避免浪费资源(其他成员函数最好设置为const的类型,避免值无意间自己给修改了)
//返回的类型也可以设置为引用类型,避免创建临时对象
const Human& getBetterMan(const Human& h1, const Human& h2) {
if (h1.getSalary() > h2.getSalary()) {
return h1;
}
else {
return h2;
}
}
//赋值构造函数,&Human等同于h1
//const型的 h2
Human& Human::operator= (const Human& other1) {
cout << "调用赋值构造函数" << this << endl;
//防止对象给自己赋值
if (this == &other1) {
//如果对象给自己赋值,就返回对象本身的指针
return *this;
}
//当执行h2 = h1时
//就相当于调用h2.operator =(h1);
this->name = other1.name;
this->age = other1.age;
this->salary = other1.salary;
//如果有必要可以先释放原本对象的资源
//看情况,因为对象生成的时候本身就有一块内存
delete addr;
addr = new char[64];
strcpy_s(this->addr, size, other1.addr);//深拷贝
//返回对象本身的引用,以方便做链式处理,h3=h2=h1;
return *this;//return this 这种只是返回对象的指针,这个对象如果继续给下一个对象赋值的,就是空的
}
void Human::setName(string name1) {
this->name = name1;
}
void Human::setAge(int age1) {
this->age = age1;
}
void Human::setSalary(int salary1) {
this->salary = salary1;
}
void Human::setAddr(char* addr)
{
if (!addr) {
return;
}
strcpy_s(this->addr, size, addr);
}
//当返回对象是指针时
const Human* Human::comBetterMan(const Human* other) {
if (this->getSalary() > other->getSalary()) {
return this;
}
else {
return other;
}
}
//当返回对象是引用时
const Human& Human::comBetterMan1(const Human& other) {
if (this->getSalary() > other.getSalary()) {
return *this;
}
else {
return other;
}
}
Human::~Human() {
//这里加个this,谁来调用就返回这个对象的指针,在其他对象中也加入就可以判断调用的先后顺序
cout << "调用析构函数" << this << endl;
delete addr;
}
主函数.cpp
#include <iostream>
#include <string>
#include "Human.h"
using namespace std;
#define size 16
//成员描述信息
void Human::descript() {
cout << "name: " << getName()
<< "age: " << getAge()
<< "salary: " << getSalary()
<< "addr: " << addr << endl;//可以直接访问,只是不安全
}
//拷贝构造函数作为函数的参数,而非引用类型
void type1(Human h2) {
cout << "拷贝构造函数作为函数的参数,而非引用类型" << endl;
cout << "name: " << h2.getName()
<< "age: " << h2.getAge()
<< "salary: " << h2.getSalary()
<< "addr: " << h2.getAddr() << endl;
}
int main(void) {
//自定义一个带参的构造函数
Human h1("张三", 24, 25000);
Human h2("李四", 40, 50000);
cout << "用指针返回的是:" << h1.comBetterMan(&h2) << endl;
cout << "用引用返回的是:" << &h1.comBetterMan1(h2) << endl;
return 0;
}
static关键字
弥补使用全局变量不方便,破坏程序的封装性,就是用静态
对于非 const 的类静态成员
只能在类的实现文件中初始化。所有成员函数都可读可写
头文件.h
class Human{
public:
......
private:
....
static int count;
....
};
实现文件.cpp
//初始化静态成员
//对于非 const 的类静态成员,只能在类的实现文件中初始化。
int Human::count = 0;
const 类静态成员
可以在类内设置初始值,也可以在类的实现文件中设置初始值。(但是 不要同时在这两个地方初始化,只能初始化 1 次)
类的静态方法:别人可以访问他,但是他不能访问别人
优点:可以节省创建对象来调用成员函数,因为静态的成员函数可以用类来调用
1. 可以直接通过类来访问【更常用】,也可以通过对象(实例)来访问。
/ 直接通过类名来访问静态方法!
// 用法:类名::静态方法2. 在类的静态方法中,不能访问普通数据成员和普通成员函数(对象的数据成员和成员函
数)
静态方法中,只能访问静态数据成员
静态方法的实现,不能加 static
#pragma once
......
class Human {
public:
......
static int getCount();
......
};
实现文件中
//静态方法的实现,不能加 static
int Human::getCount() {
// 静态方法中,不能访问实例成员(普通的数据成员)
// cout << age;
// 静态方法中,不能访问 this 指针,因为 this 指针是属于实例对象的
// cout << this;
//静态方法中,只能访问静态数据成员
return count;
}
//静态方法:别人可以访问他,但是他不能访问别人
void test() {
cout << "总人数: ";
// ??? 没有可用的对象来访问 getCount()
// 直接通过类名来访问静态方法!
// 用法:类名::静态方法
cout << Human::getCount();
}
int main(void) {
//自定义一个带参的构造函数
Human h1("张三", 24, 25000);
Human h2("李四", 40, 50000);
//可以通过类名访问静态成员方法,也可以通过实例对象
cout << Human::getCount() << endl;
cout << h1.getCount() << endl;
return 0;
}
所有的成员函数,都可以访问静态数据成员。
类可以直接访问public静态数据成员(Human::count 非法),但是不能访问私有的静态数据成员
对象可以直接访问静态成员函数类可以直接访问静态成员函数(但是类不能访问非静态成员函数)在类的静态成员函数(类的静态方法)内部, 不能直接访问 this 指针和对象的数据成员 !
const关键字
const成员数据
只能读不能写
初始化:
设置类内初始值,(C11以上版本支持)
const char BloodType = 'B';
使用构造函数的初始化列表:一个冒号+const成员('初始值')
Human::Human(string name, int age, int salary):BloodType('A')
如何做到灵活初始化,用带参的构造函数的参数列表里定义一个形参,去表示初始化列表里的值
/*
Human(string name, int age, int salary,string BloodType);
const string BloodType = "B";
*/
实现文件
//在定义的对象的时候就可以灵活的设置初始值了
Human::Human(string name, int age, int salary,string BloodType):BloodType(BloodType) {
}
int main(void) {
//自定义一个带参的构造函数
Human h1("张三", 24, 25000,"AB");
Human h2("李四", 40, 50000,"A");
h1.descript();
h2.descript();
return 0;
}
const成员函数
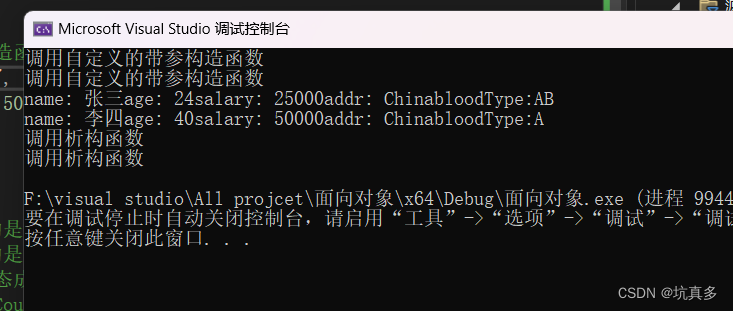
void descript() const;
//成员描述信息
void Human::descript() const{//这个函数里面就不能修改数据
cout << "name: " << getName()
<< "age: " << getAge()
<< "salary: " << getSalary()
<< "addr: " << addr
<< "bloodType:"<<BloodType << endl;//可以直接访问,只是不安全
}
int main(void) {
//自定义一个带参的构造函数
const Human h1("张三", 24, 25000,"AB");//const对象可以访问const的成员函数
Human h2("李四", 40, 50000,"A");//也可以访问const的成员函数
h1.descript();
h2.descript();
return 0;
}