一、飞机票折扣
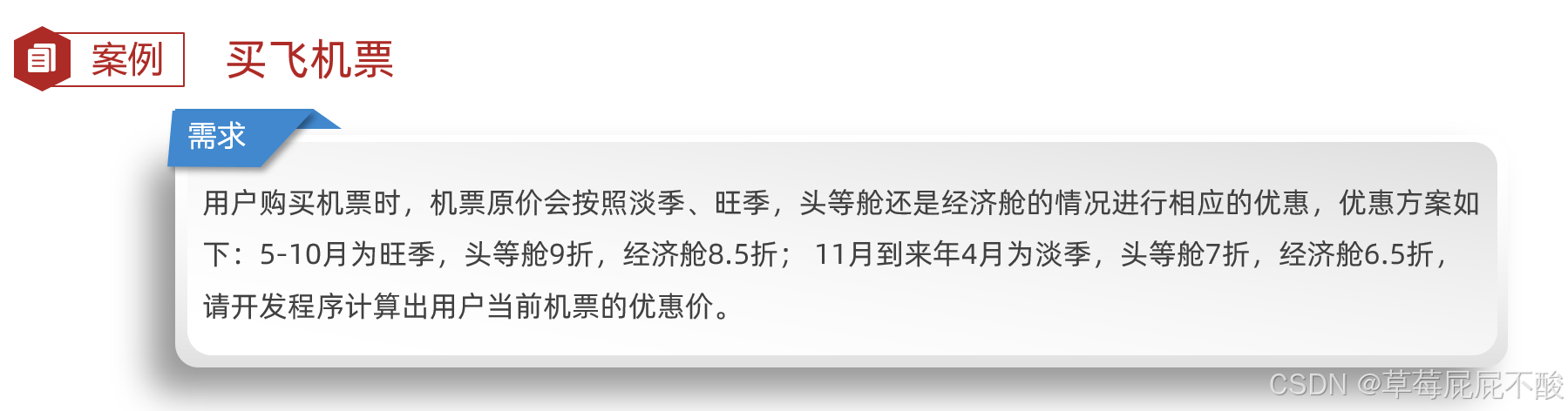
参数:
int month : 月份
String type : 舱位类型
double price : 机票原始价格
返回值:
double : 优惠之后的机票价格
public class Case1 {
public static void main(String[] args) {
double price = culate(1000, 11, "头等舱");
System.out.println("优惠价是:" + price);
}
public static double culate(int price, int month, String type){
//优惠后的价格
double money = 0;
//1.通过if判断淡季或旺季
if(month >= 5 && month <= 10){
//2.旺季中舱位类型,计算不同票价
switch (type){
case "头等舱":
money = price * 0.9;
break;
case "经济舱":
money = price * 0.85;
break;
default:
money = price * 1.0;
}
}else {
// 3.淡季中舱位类型,计算不同票价
switch (type){
case "头等舱":
money = price * 0.7;
break;
case "经济舱":
money = price * 0.65;
break;
default:
money = price * 1.0;
}
}
return money;
}
}
二、开发验证码
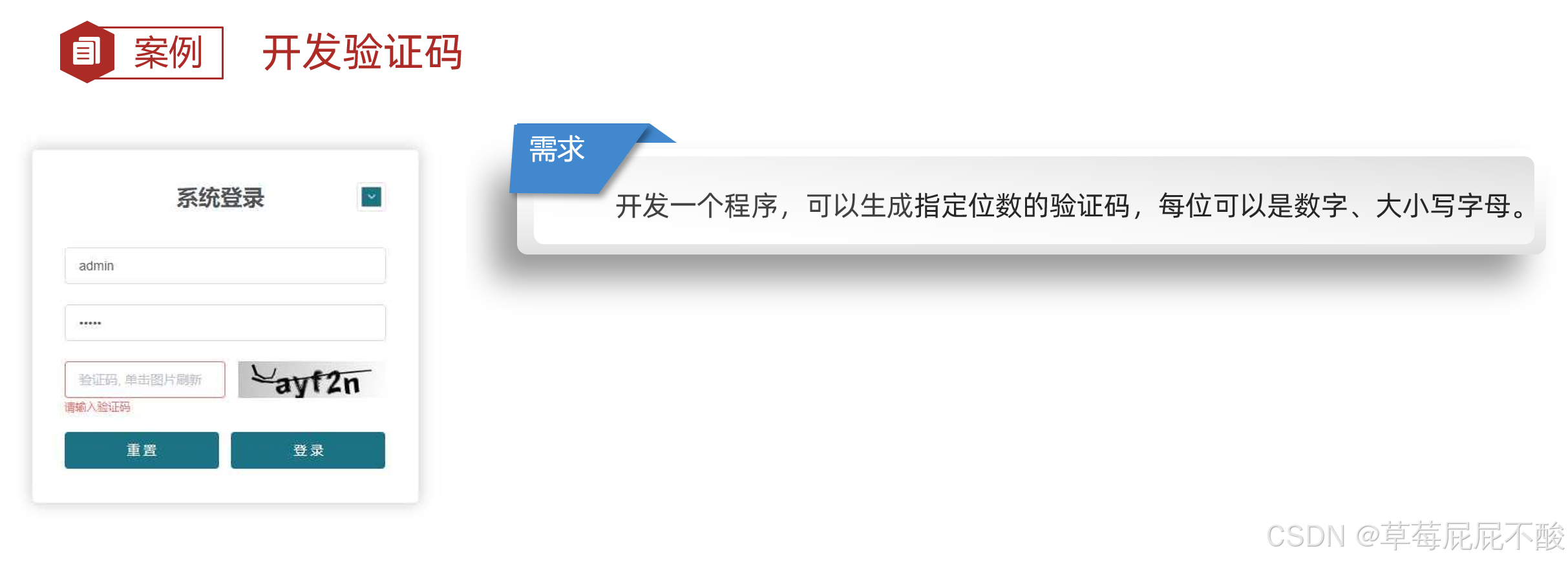
定义方法,接受一个数字,生成指定位数的验证码字符串,并返回
参数: int num(验证码的位数)
返回: String (验证码)
//方法1:
public class Case2 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入想生成验证码的位数:");
int n = sc.nextInt();
System.out.println("生成的" + n + "位验证码为:" + getCode(n));
}
public static String getCode (int num){
String code = "";
//1.定义一个字符串数组,每一位验证码的可选数据
String[] codes = {"0","1","2","3","4","5","6","7","8","9","a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z","A","B","C","D","E","F","G","H","I","J","J","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z"};
//2.创建随机工具
Random r = new Random();
//3.通过for循环生成验证码
for (int i = 0; i < num; i++) {
//4.生成一个字符串数组的索引值,本位的数据索引
int index = r.nextInt(62);
//5.获取本次随机的字符
code += codes[index];
}
return code;
}
}
//方法2:
public class Case2 {
public static void main(String[] args) {
System.out.println("验证码为:" + getCode2(8));
}
public static String getCode2(int n){
//1)先按照方法接收的验证码位数n,循环n次
Random r = new Random();
//3)定义一个String类型的变量用于记住产生的每位随机字符
String code = "";
for (int i = 0; i < n; i++) {
// 2)每次循环,产生一个字符,可以是数字字符、或者大小写字母字符
// 思路:随机一个0 1 2之间的数字出来,
// 0代表随机一个数字字符,1、2代表随机大写字母,小写字母。
int type = r.nextInt(3);//0,1,2
switch (type){
case 0:
//随机一个数字字符 0~9
code += r.nextInt(10);
break;
case 1:
//随机一个大写字符 A 65 ~ Z 65+25
char ch1 = (char)(r.nextInt(25+1)+65);
code += ch1;
break;
case 2:
//随机一个小写字符 a 97 ~ z 97+25
char ch2 = (char)(r.nextInt(25+1)+97);
code += ch2;
break;
}
}
return code;
}
}
三、评委打分
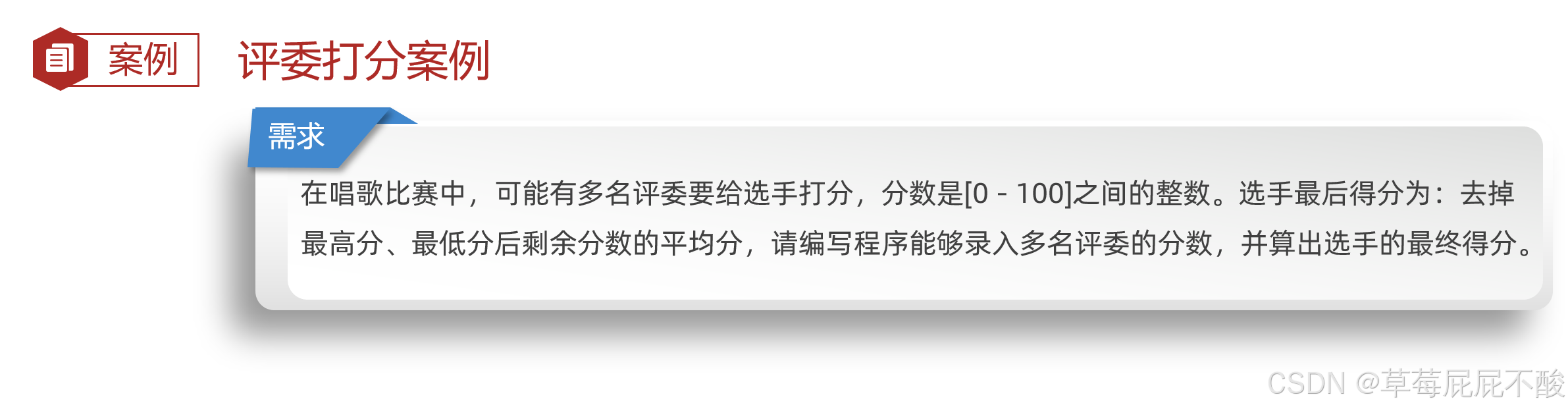
传入一个评委人数的参数,获取选手的最终平均分
参数:评委人数 int num
返回值:选手最终的平均分 int
public class Case3 {
public static void main(String[] args) {
System.out.println("当前选手的得分是:" + getAvgScore(10));
}
public static double getAvgScore(int n){
Scanner sc = new Scanner(System.in);
int[] scores = new int[n];
//循环n次,使用Scanner键盘录入n个1~100范围内的整数,
// 并把整数存储到数组中
for (int i = 1; i <= n; i++) {
System.out.print("请输入第" + i + "个评委的分数:");
scores[i-1] = sc.nextInt();
}
//求数组元素的总和
int sum = 0;
for (int i = 0; i < scores.length; i++) {
sum += scores[i];
}
//求数组元素的最大值
int max = scores[0];
for (int i = 1; i < scores.length; i++) {
if (scores[i] > max){
max = scores[i];
}
}
//求数组元素的最小值
int min = scores[0];
for (int i = 1; i < scores.length; i++) {
if(scores[i] < min){
min = scores[i];
}
}
double avg = 1.0*(sum - min - max)/(scores.length-2);
return avg;
}
}
四、数字加密
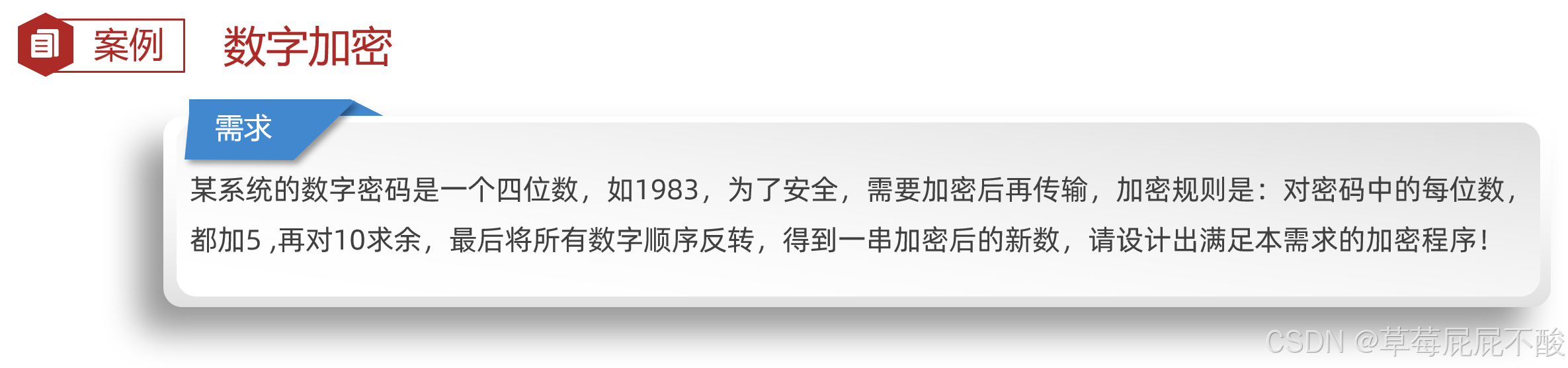
参数:int 1983
返回值:String
代码(加密)
1.获取代码的每一位 1,9,8,3
2.加密:(x + 5)% 10 6,4,3,8
3.反转 8346
public class Case4 {
public static void main(String[] args) {
System.out.println("加密后的结果是:" + newNum(8346));
}
//先要把4位数整数拆分为,4个数字,用一个数组保存起来
public static int[] split(int num){
int[] number = new int[4];
number[0] = num / 1000;
number[1] = num / 100 % 10;
number[2] = num / 10 % 10;
number[3] = num % 10;
return number;
}
//写一个方法,实现数组元素的反转
public static void reverse(int[] a){
for (int i = 0, j = a.length-1; i < j; i++, j--){
int temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
//实现数字加密
public static String newNum(int num){
//1、把这个密码拆分成一个一个的数字,才可以对其进行加密
int[] number = split(num);
for (int i = 0; i < number.length; i++) {
number[i] = (number[i] + 5) % 10;
}
reverse(number);
String newnumber = "";
for (int i = 0; i < number.length; i++) {
newnumber += number[i];
}
return newnumber;
}
}
五、数组拷贝

参数: int[] a
返回: int[] newa
业务:
1.创建一个新数组,长度和a的长度一致
2.循环a数组,获取每一位数据,赋值到新数组的对应位置上
public class Case5 {
public static void main(String[] args) {
int[] arr = {11,22,33,44,55,66};
int[] brr = copy(arr);
System.out.println("拷贝的新数组为:" );
for (int i = 0; i < brr.length; i++) {
System.out.print(brr[i] + " ");
}
}
public static int[] copy(int[] a){
//1、创建一个新数组,长度和a一样
int[] newa = new int[a.length];
//2、循环a,获取a的每个元素,存到新数组对应的索引位置上
for (int i = 0; i < a.length; i++) {
newa[i] = a[i];
}
//3、返回新数组
return newa;
}
}
六、抢红包
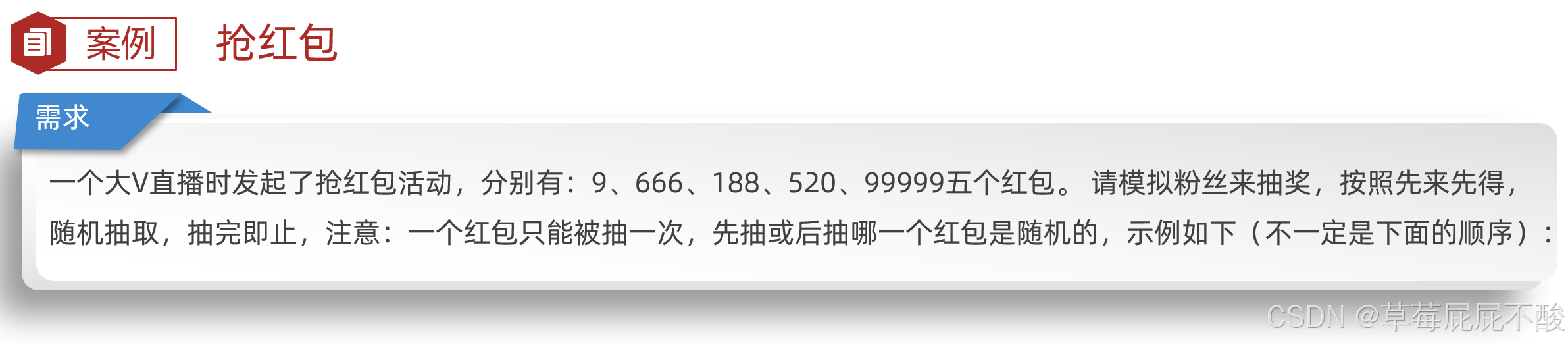
参数: int[] a
无返回值
方法1:
每次抽奖都从数组中,随机找出一个金额,如果该金额不是0,就输出该金额,
然后用0替换该位置处的金额;
如果该位置就是0,则重复上一步操作!
public class Case6 {
public static void main(String[] args) {
int[] arr = {9,666,180,520,99999};
qhb(arr);
}
public static void qhb(int[] a){
Random r = new Random();
Scanner sc = new Scanner(System.in);
int i = 0;
while (i < a.length){
System.out.println("请按任意键完成抽奖:");
String s = sc.next();
int index = r.nextInt(a.length);
if(a[index] != 0){
System.out.println("恭喜您!抽中了,"+ a[index]);
a[index] = 0;
i++;
}
}
System.out.println("活动结束!");
}
}
方法2:
先把数组里面的5个金额打乱顺序,打乱后的顺序就认为是中奖顺序;
接着,写个for循环,执行5次,每次都提示抽奖;
每次抽奖,都依次取出数组中的每个位置处的金额作为中奖金额即可。
public class Case62 {
public static void main(String[] args) {
int[] arr = {9,666,180,520,99999};
qhb2(arr);
}
public static void qhb2(int[] a){
Scanner sc = new Scanner(System.in);
Random r = new Random();
//1.先把数组里面的5个金额打乱顺序,打乱后的顺序就认为是中奖顺序
for (int i = 0; i < a.length; i++) {
int index = r.nextInt(a.length);
int temp = a[i];
a[i] = a[index];
a[index] = temp;
}
//写个for循环,执行5次,每次都提示抽奖;
for (int i = 0; i < a.length; i++) {
System.out.println("请按任意键完成抽奖:");
String s = sc.next();
System.out.println("恭喜您!抽中了,"+ a[i]);
}
System.out.println("活动结束!");
}
}
七、找素数
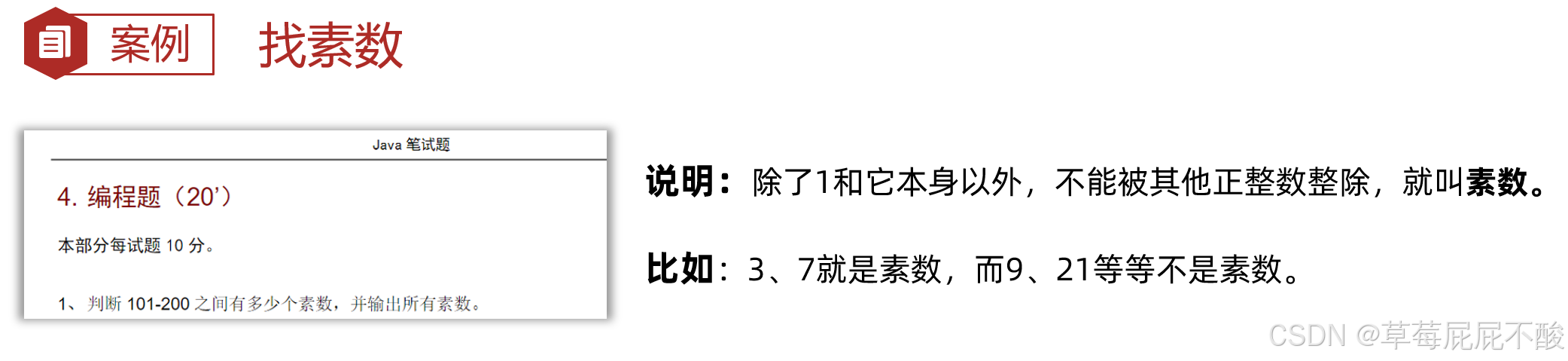
参数: int num1 , int num2
返回值: int count 素数个数
public class Case7 {
public static void main(String[] args) {
int a = 101;
int b = 200;
int count = getSu(a,b);
int count1 = getHe(a, b);
System.out.println("101~200素数有" + count + "个");
System.out.println("101~200合数有" + count1 + "个");
}
//求num1~num2之间的素数(除了1和它本身以外,不能被其他正整数整除,就叫素数)
public static int getSu(int num1, int num2){
int count = 0;
for (int i = num1; i <= num2; i++) {
int j;
//从2开始遍历到该数的数据,看是否有数据可以整除它,
// 有则不是素数,没有则是素数
for (j = 2; j < i; j++) {
if(i % j == 0){
//不是素数
break;
}
}
//内层循环执行结束,j++=数本身i,说明i一直未被j整除,i是素数
if(j == i){
System.out.println(i+ "是素数");
count++;
}
}
return count;
}
//求num1~num2之间的合数
public static int getHe(int num1, int num2){
int count = 0;
for (int i = num1; i <= num2; i++) {
int j;
for (j = 2; j < i; j++) {
if(i % j == 0){
//System.out.println(i+ "是合数");
count++;
break;
}
}
}
return count;
}
}