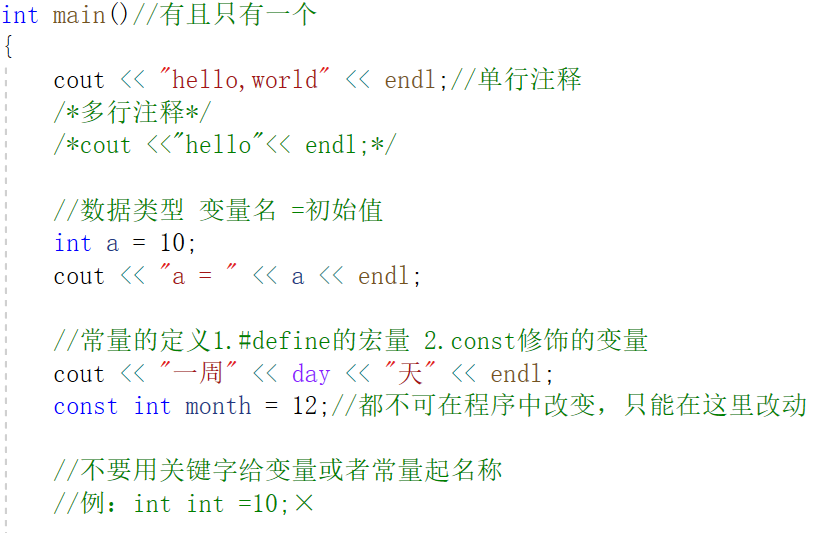
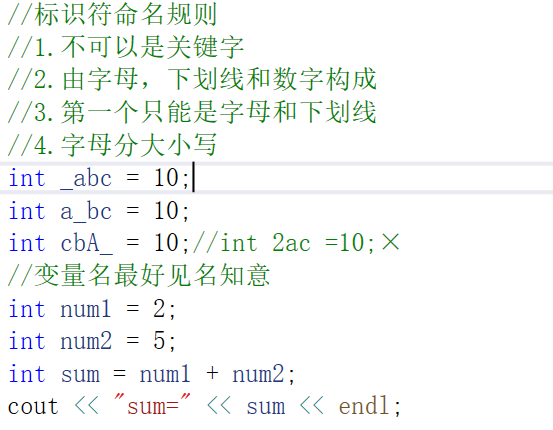
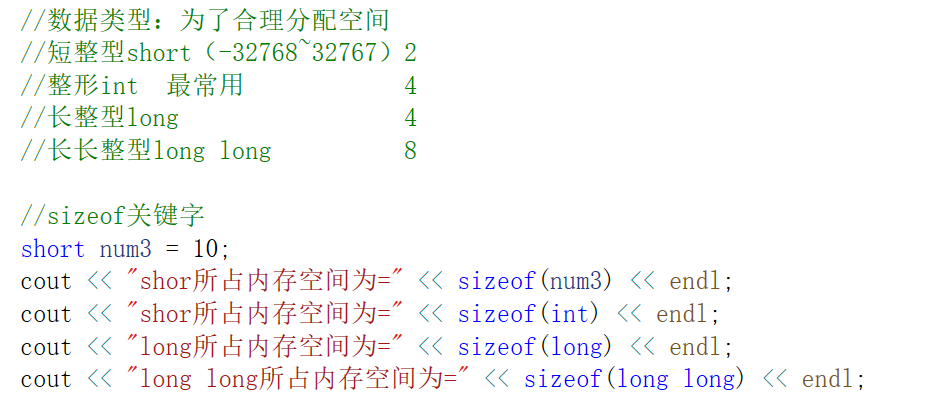
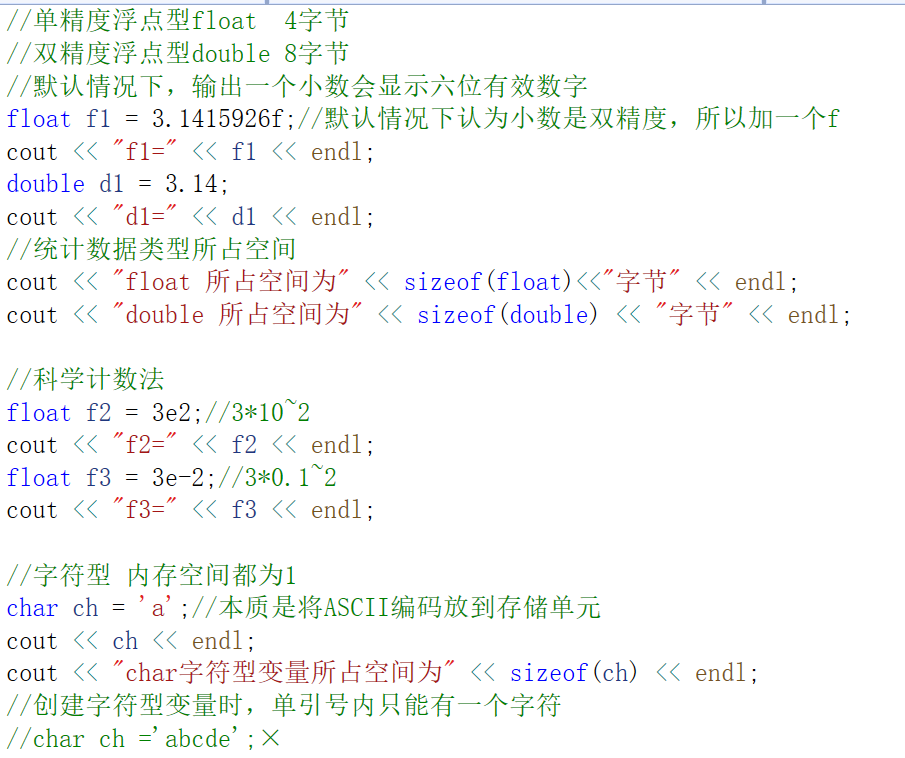
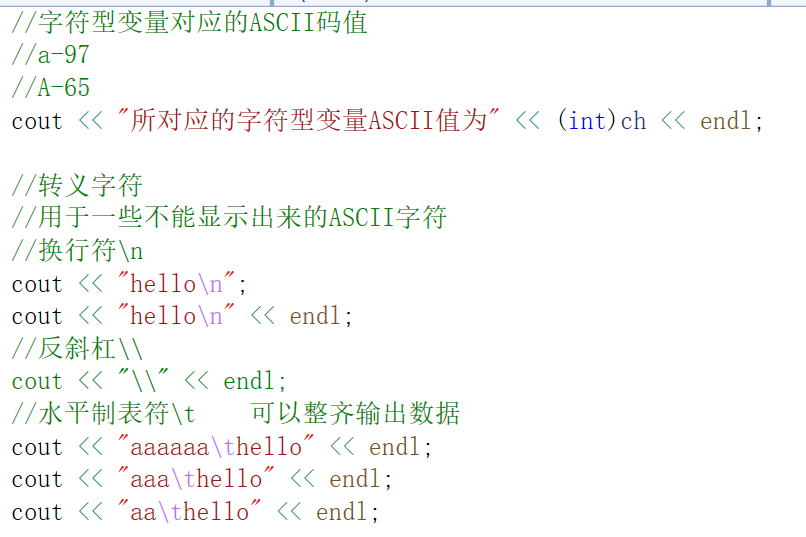
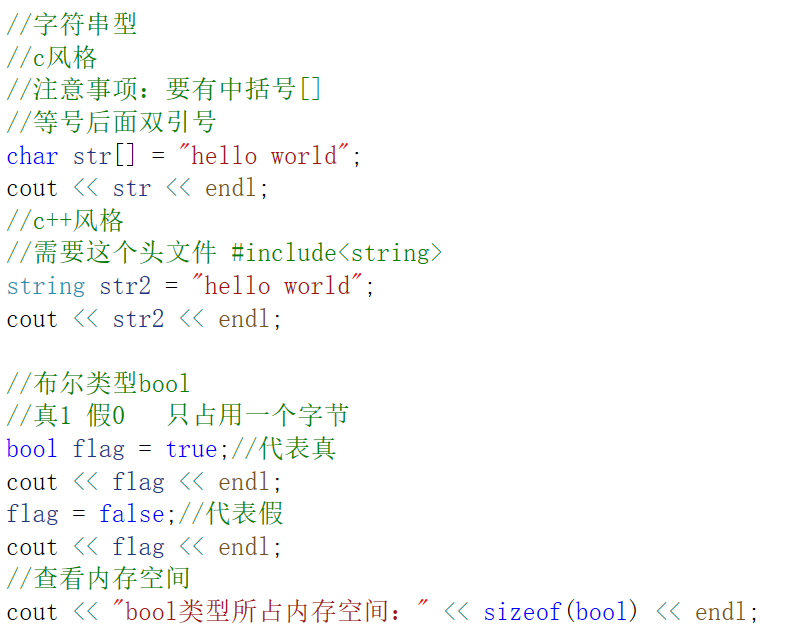
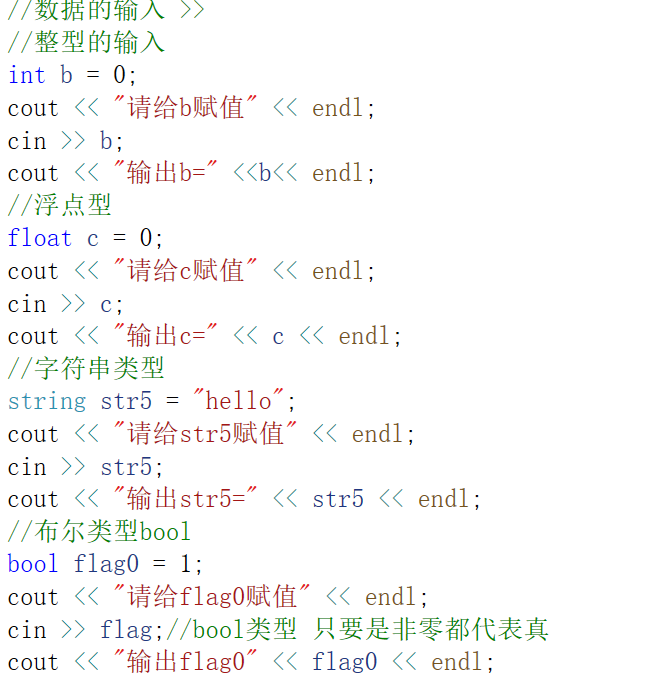
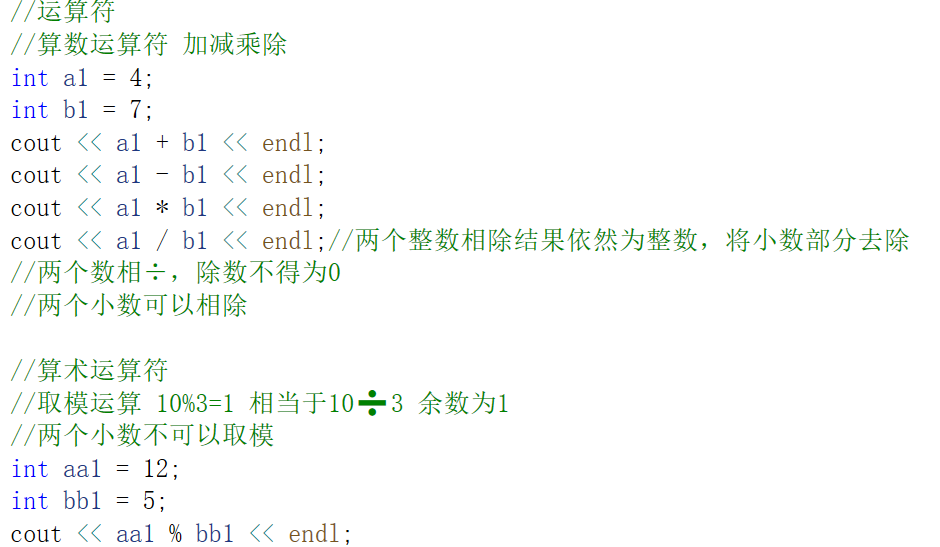
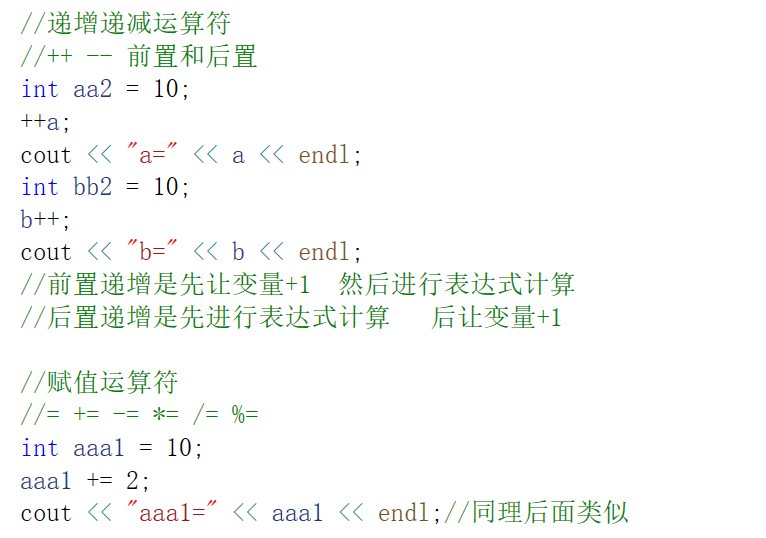
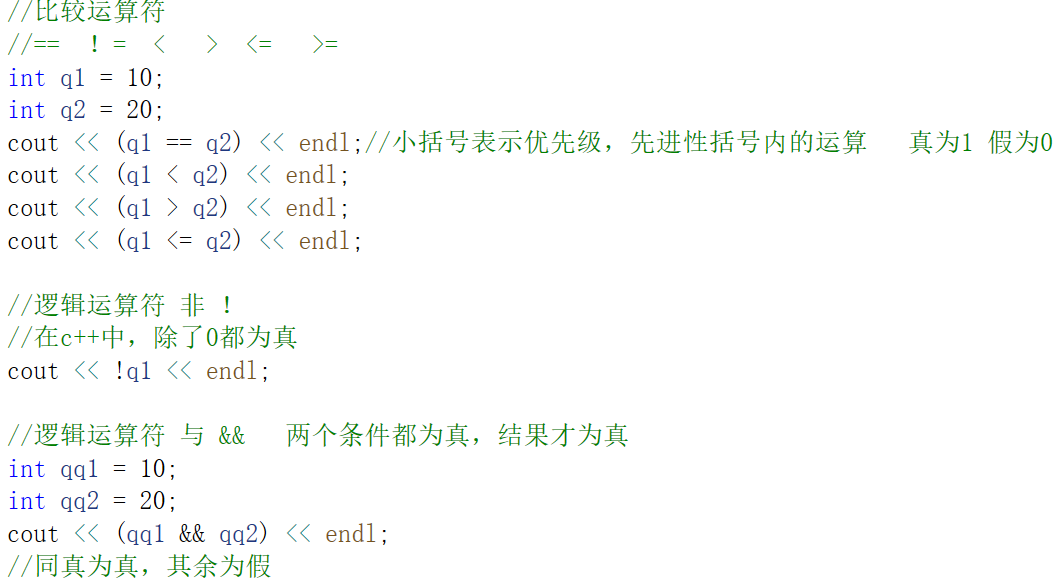
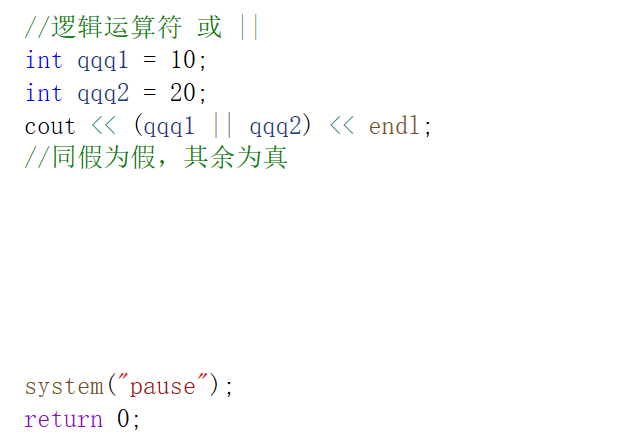
#define _CRT_SECURE_NO_WARNINGS
#include<iostream>
#include<string>
using namespace std;
#define day 7
int main()//有且只有一个
{
cout << "hello,world" << endl;//单行注释
/*多行注释*/
/*cout <<"hello"<< endl;*/
//数据类型 变量名 =初始值
int a = 10;
cout << "a = " << a << endl;
//常量的定义1.#define的宏量 2.const修饰的变量
cout << "一周" << day << "天" << endl;
const int month = 12;//都不可在程序中改变,只能在这里改动
//不要用关键字给变量或者常量起名称
//例:int int =10;×
//标识符命名规则
//1.不可以是关键字
//2.由字母,下划线和数字构成
//3.第一个只能是字母和下划线
//4.字母分大小写
int _abc = 10;
int a_bc = 10;
int cbA_ = 10;//int 2ac =10;×
//变量名最好见名知意
int num1 = 2;
int num2 = 5;
int sum = num1 + num2;
cout << "sum=" << sum << endl;
//数据类型:为了合理分配空间
//短整型short(-32768~32767)2
//整形int 最常用 4
//长整型long 4
//长长整型long long 8
//sizeof关键字
short num3 = 10;
cout << "shor所占内存空间为=" << sizeof(num3) << endl;
cout << "shor所占内存空间为=" << sizeof(int) << endl;
cout << "long所占内存空间为=" << sizeof(long) << endl;
cout << "long long所占内存空间为=" << sizeof(long long) << endl;
//单精度浮点型float 4字节
//双精度浮点型double 8字节
//默认情况下,输出一个小数会显示六位有效数字
float f1 = 3.1415926f;//默认情况下认为小数是双精度,所以加一个f
cout << "f1=" << f1 << endl;
double d1 = 3.14;
cout << "d1=" << d1 << endl;
//统计数据类型所占空间
cout << "float 所占空间为" << sizeof(float)<<"字节" << endl;
cout << "double 所占空间为" << sizeof(double) << "字节" << endl;
//科学计数法
float f2 = 3e2;//3*10~2
cout << "f2=" << f2 << endl;
float f3 = 3e-2;//3*0.1~2
cout << "f3=" << f3 << endl;
//字符型 内存空间都为1
char ch = 'a';//本质是将ASCII编码放到存储单元
cout << ch << endl;
cout << "char字符型变量所占空间为" << sizeof(ch) << endl;
//创建字符型变量时,单引号内只能有一个字符
//char ch ='abcde';×
//字符型变量对应的ASCII码值
//a-97
//A-65
cout << "所对应的字符型变量ASCII值为" << (int)ch << endl;
//转义字符
//用于一些不能显示出来的ASCII字符
//换行符\n
cout << "hello\n";
cout << "hello\n" << endl;
//反斜杠\\
cout << "\\" << endl;
//水平制表符\t 可以整齐输出数据
cout << "aaaaaa\thello" << endl;
cout << "aaa\thello" << endl;
cout << "aa\thello" << endl;
//字符串型
//c风格
//注意事项:要有中括号[]
//等号后面双引号
char str[] = "hello world";
cout << str << endl;
//c++风格
//需要这个头文件 #include<string>
string str2 = "hello world";
cout << str2 << endl;
//布尔类型bool
//真1 假0 只占用一个字节
bool flag = true;//代表真
cout << flag << endl;
flag = false;//代表假
cout << flag << endl;
//查看内存空间
cout << "bool类型所占内存空间:" << sizeof(bool) << endl;
//数据的输入 >>
//整型的输入
int b = 0;
cout << "请给b赋值" << endl;
cin >> b;
cout << "输出b=" <<b<< endl;
//浮点型
float c = 0;
cout << "请给c赋值" << endl;
cin >> c;
cout << "输出c=" << c << endl;
//字符串类型
string str5 = "hello";
cout << "请给str5赋值" << endl;
cin >> str5;
cout << "输出str5=" << str5 << endl;
//布尔类型bool
bool flag0 = 1;
cout << "请给flag0赋值" << endl;
cin >> flag;//bool类型 只要是非零都代表真
cout << "输出flag0" << flag0 << endl;
//运算符
//算数运算符 加减乘除
int a1 = 4;
int b1 = 7;
cout << a1 + b1 << endl;
cout << a1 - b1 << endl;
cout << a1 * b1 << endl;
cout << a1 / b1 << endl;//两个整数相除结果依然为整数,将小数部分去除
//两个数相÷,除数不得为0
//两个小数可以相除
//算术运算符
//取模运算 10%3=1 相当于10➗3 余数为1
//两个小数不可以取模
int aa1 = 12;
int bb1 = 5;
cout << aa1 % bb1 << endl;
//递增递减运算符
//++ -- 前置和后置
int aa2 = 10;
++a;
cout << "a=" << a << endl;
int bb2 = 10;
b++;
cout << "b=" << b << endl;
//前置递增是先让变量+1 然后进行表达式计算
//后置递增是先进行表达式计算 后让变量+1
//赋值运算符
//= += -= *= /= %=
int aaa1 = 10;
aaa1 += 2;
cout << "aaa1=" << aaa1 << endl;//同理后面类似
//比较运算符
//== != < > <= >=
int q1 = 10;
int q2 = 20;
cout << (q1 == q2) << endl;//小括号表示优先级,先进性括号内的运算 真为1 假为0
cout << (q1 < q2) << endl;
cout << (q1 > q2) << endl;
cout << (q1 <= q2) << endl;
//逻辑运算符 非 !
//在c++中,除了0都为真
cout << !q1 << endl;
//逻辑运算符 与 && 两个条件都为真,结果才为真
int qq1 = 10;
int qq2 = 20;
cout << (qq1 && qq2) << endl;
//同真为真,其余为假
//逻辑运算符 或 ||
int qqq1 = 10;
int qqq2 = 20;
cout << (qqq1 || qqq2) << endl;
//同假为假,其余为真
system("pause");
return 0;
}