### 14. 列表扁平
下面的方法使用**递归**将一个潜在的深层列表偏平化。
def spread(arg):
ret = []
for i in arg:
if isinstance(i, list):
ret.extend(i)
else:
ret.append(i)
return ret
def deep_flatten(xs):
flat_list = []
[flat_list.extend(deep_flatten(x)) for x in xs] if isinstance(xs, list) else flat_list.append(xs)
return flat_list
print(deep_flatten([1, [2], [[3], 4], 5]))
[1,2,3,4,5]
### 15. 列表求差
下面方法通过对列表模拟集合,快速求差。
def difference(a, b):
set_a = set(a)
set_b = set(b)
comparison = set_a.difference(set_b)
return list(comparison)
difference([1,2,3], [1,2,4]) # [3]
### 16. 差值函数
下面方法在对两个列表的每个元素应用给定函数后,返回两个列表的差值。
def difference_by(a, b, fn):
b = set(map(fn, b))
return [item for item in a if fn(item) not in b]
from math import floor
difference_by([2.1, 1.2], [2.3, 3.4], floor) # [1.2]
difference_by([{ ‘x’: 2 }, { ‘x’: 1 }], [{ ‘x’: 1 }], lambda v : v[‘x’]) # [ { x: 2 } ]
### 17. 链式函数
可以在一行里面调用多个函数.
def add(a, b):
return a + b
def subtract(a, b):
return a - b
a, b = 4, 5
print((subtract if a > b else add)(a, b)) # 9
### 18. 重复值
利用`set()`只包含唯一的元素特点来判断列表是否有重复的值。
def has_duplicates(lst):
return len(lst) != len(set(lst))
x = [1,2,3,4,5,5]
y = [1,2,3,4,5]
print(has_duplicates(x)) # True
print(has_duplicates(y)) # False
### 19. 合并字典
下面方法利用`update()`合并两个字典。
def merge_two_dicts(a, b):
c = a.copy() # make a copy of a
c.update(b) # modify keys and values of a with the ones from b
return c
a = { ‘x’: 1, ‘y’: 2}
b = { ‘y’: 3, ‘z’: 4}
print(merge_two_dicts(a, b)) # {‘y’: 3, ‘x’: 1, ‘z’: 4}
在Python 3.5及以上版本中,可以更优雅:
def merge_dictionaries(a, b):
return {**a, **b}
a = { ‘x’: 1, ‘y’: 2}
b = { ‘y’: 3, ‘z’: 4}
print(merge_dictionaries(a, b)) # {‘y’: 3, ‘x’: 1, ‘z’: 4}
### 20. 两列表转为字典
下面的方法用`zip()`及`dict()`函数将两个列表转换为一个字典。
def to_dictionary(keys, values):
return dict(zip(keys, values))
keys = [“a”, “b”, “c”]
values = [2, 3, 4]
print(to_dictionary(keys, values)) # {‘a’: 2, ‘c’: 4, ‘b’: 3}
### 21. 巧用`enumerate`
使用`enumerate()`来获取列表的值和索引。
list = [“a”, “b”, “c”, “d”]
for index, element in enumerate(list):
print(“Value”, element, "Index ", index, )
(‘Value’, ‘a’, 'Index ', 0)
(‘Value’, ‘b’, 'Index ', 1)
#(‘Value’, ‘c’, 'Index ', 2)
(‘Value’, ‘d’, 'Index ', 3)
### 22. 耗时统计
用`time.time()`计算执行某段代码所需的时间。
import time
start_time = time.time()
a = 1
b = 2
c = a + b
print© #3
end_time = time.time()
total_time = end_time - start_time
print("Time: ", total_time)
('Time: ', 1.1205673217773438e-05)
### 23. `try-else`
`else`子句作为`try/except`块的一部分, 如果没有抛出异常, 则执行该子句。
try:
2*3
except TypeError:
print(“An exception was raised”)
else:
print(“Thank God, no exceptions were raised.”)
#Thank God, no exceptions were raised.
### 24. 高频发现
下面方法返回列表中出现频率最高的元素。
def most_frequent(list):
return max(set(list), key = list.count)
numbers = [1,2,1,2,3,2,1,4,2]
print(most_frequent(numbers))
2
### 25. 回文判断
检查给定的字符串是否是一个回文。
def palindrome(a):
return a == a[::-1]
print(palindrome(‘mom’)) # True
print(palindrome(‘pythontip’)) # False
### 26. 简单计算器
编写一个简单的计算器,而不需要使用`if-else`条件。
import operator
action = {
“+”: operator.add,
“-”: operator.sub,
“/”: operator.truediv,
“*”: operator.mul,
“**”: pow
}
print(action[‘-’](50, 25)) # 25
### 27. 随机洗牌
用`random.shuffle()`随机化一个列表中的元素顺序。注意`shuffle`在原地工作, 并返回`None`。
from random import shuffle
foo = [1, 2, 3, 4]
shuffle(foo)
print(foo) # [1, 4, 3, 2] , foo = [1, 2, 3, 4]
### 28. 列表展开
类似于JavaScript中的`[].concat(...arr)`,将一个列表扁平化,注意下面代码**不能对深层嵌套列表**扁平化。
def spread(arg):
ret = []
for i in arg:
if isinstance(i, list):
ret.extend(i)
else:
ret.append(i)
return ret
print(spread([1,2,3,[4,5,6],[7],8,9]))
[1,2,3,4,5,6,7,8,9]
print(spread([1,2,3,[4,5,6],[7],8,9,[10,[11]]]))
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, [11]]
### 29. 变量交换
一个非常快速的交换两个变量的方法,不需要使用额外的变量。
a, b = -1, 14
a, b = b, a
print(a) # 14
print(b) # -1
### 30. 字典默认值
使用`dict.get(key,default)`在要找的键不存在字典中的时返回默认值。
d = {‘a’: 1, ‘b’: 2}
print(d.get(‘a’, 3)) # 1
print(d.get(‘c’, 3)) # 3
### 小节
上面分享的30个python有趣代码,希望对大家有用。
如果觉得还可以,点赞收藏,好人一生平安 。
[pythontip](https://bbs.csdn.net/topics/618317507) 出品,Happy Coding!
公众号: 夸克编程
>
> 加vx: **pythontip**,谈天说地,聊Python
>
>
>
文末有福利领取哦~
---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
👉**一、Python所有方向的学习路线**
Python所有方向的技术点做的整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。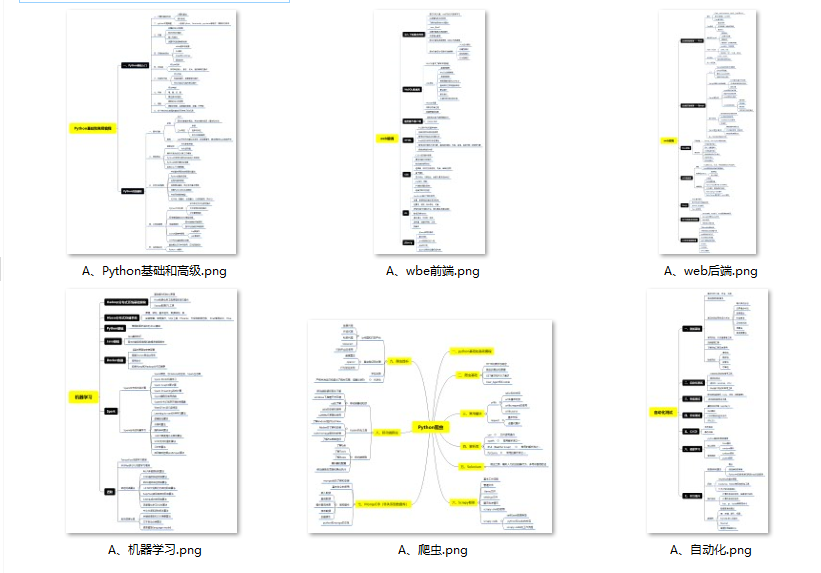
👉**二、Python必备开发工具**
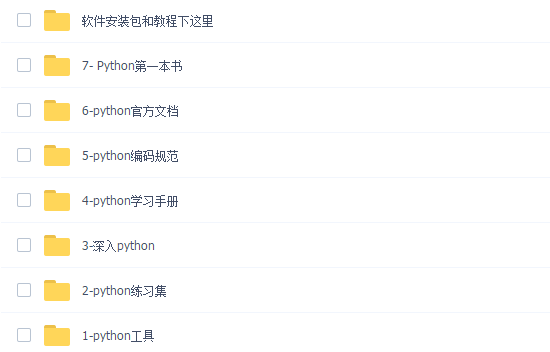
👉**三、Python视频合集**
观看零基础学习视频,看视频学习是最快捷也是最有效果的方式,跟着视频中老师的思路,从基础到深入,还是很容易入门的。
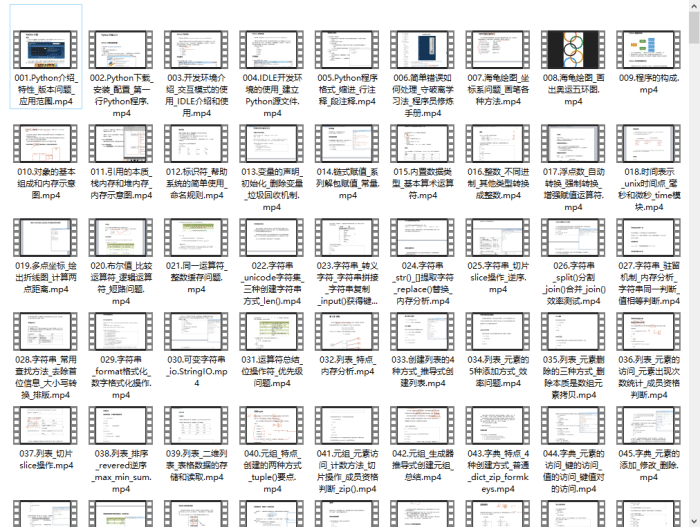
👉 **四、实战案例**
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。**(文末领读者福利)**
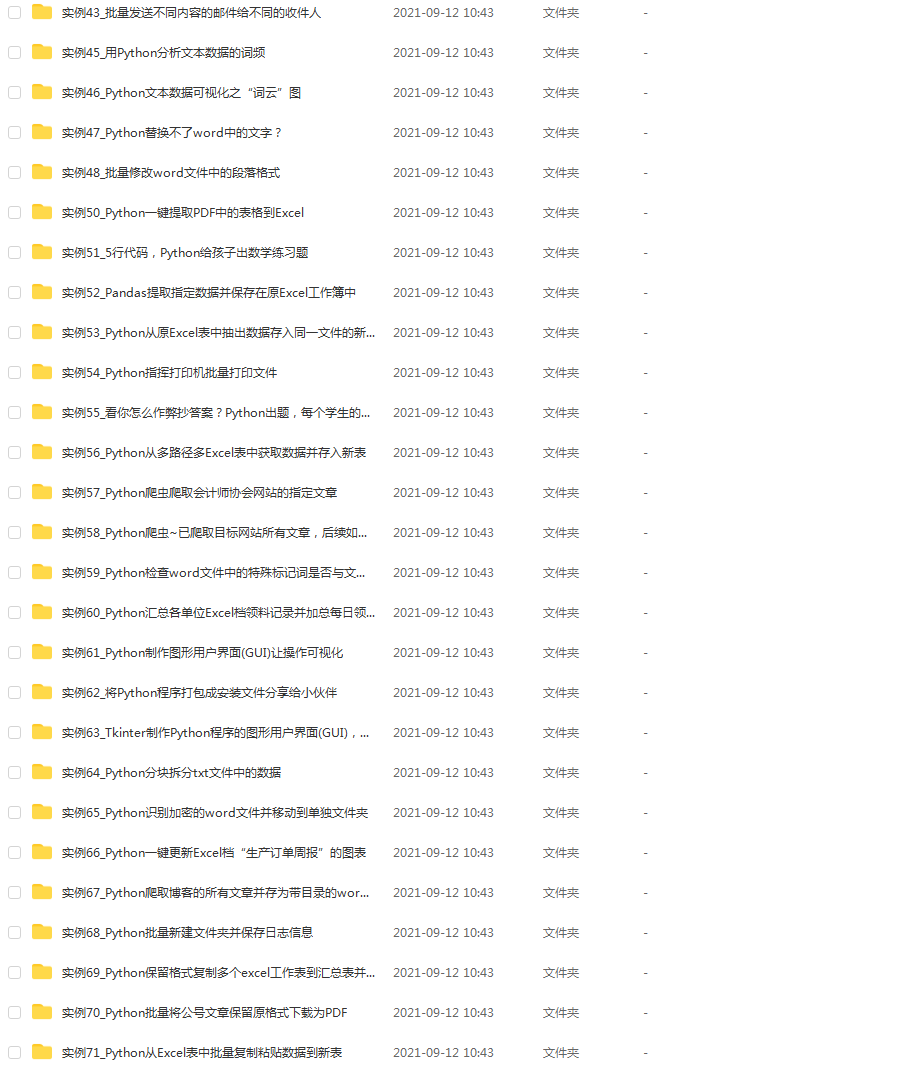
👉**五、Python练习题**
检查学习结果。
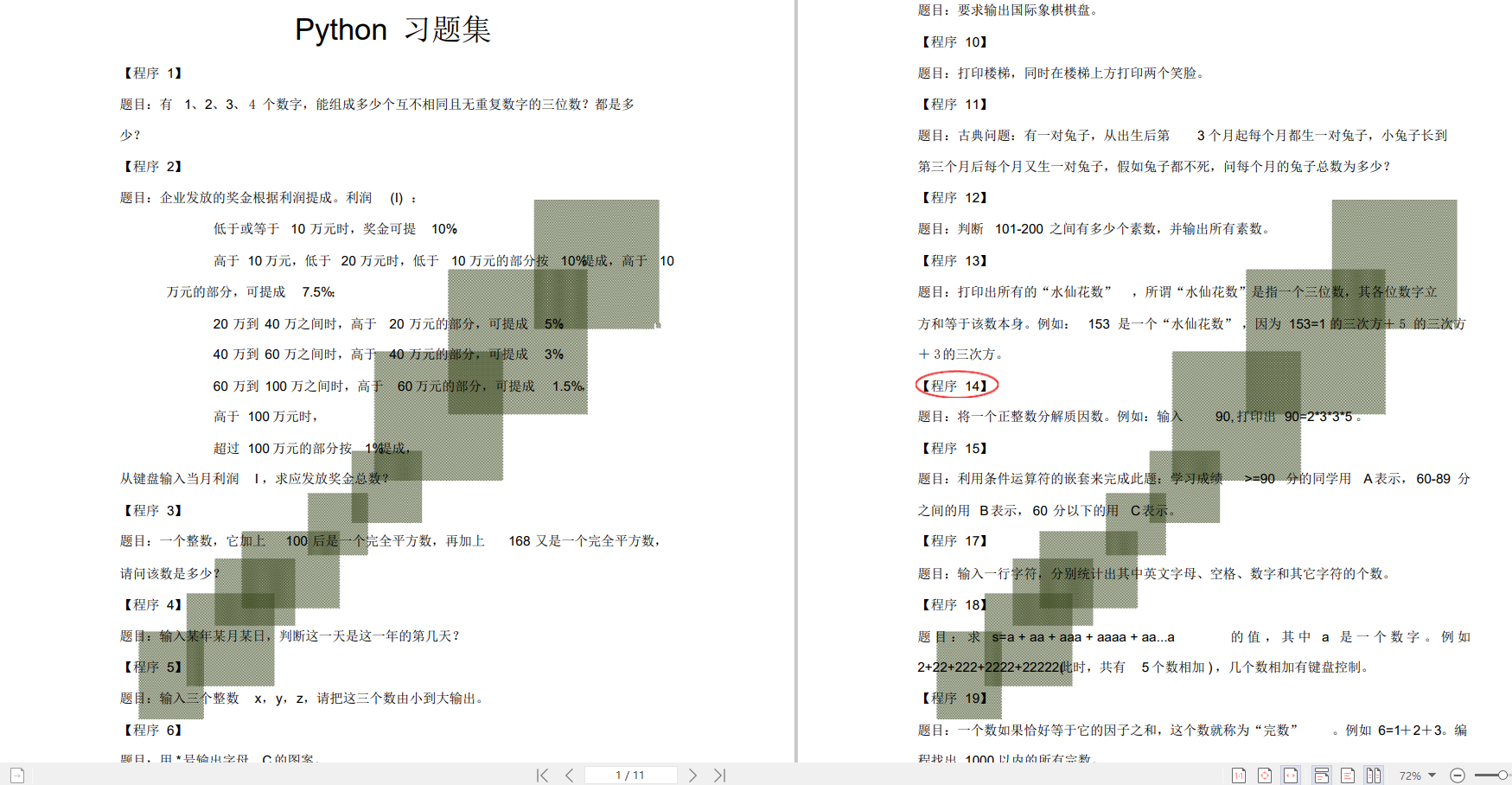
👉**六、面试资料**
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
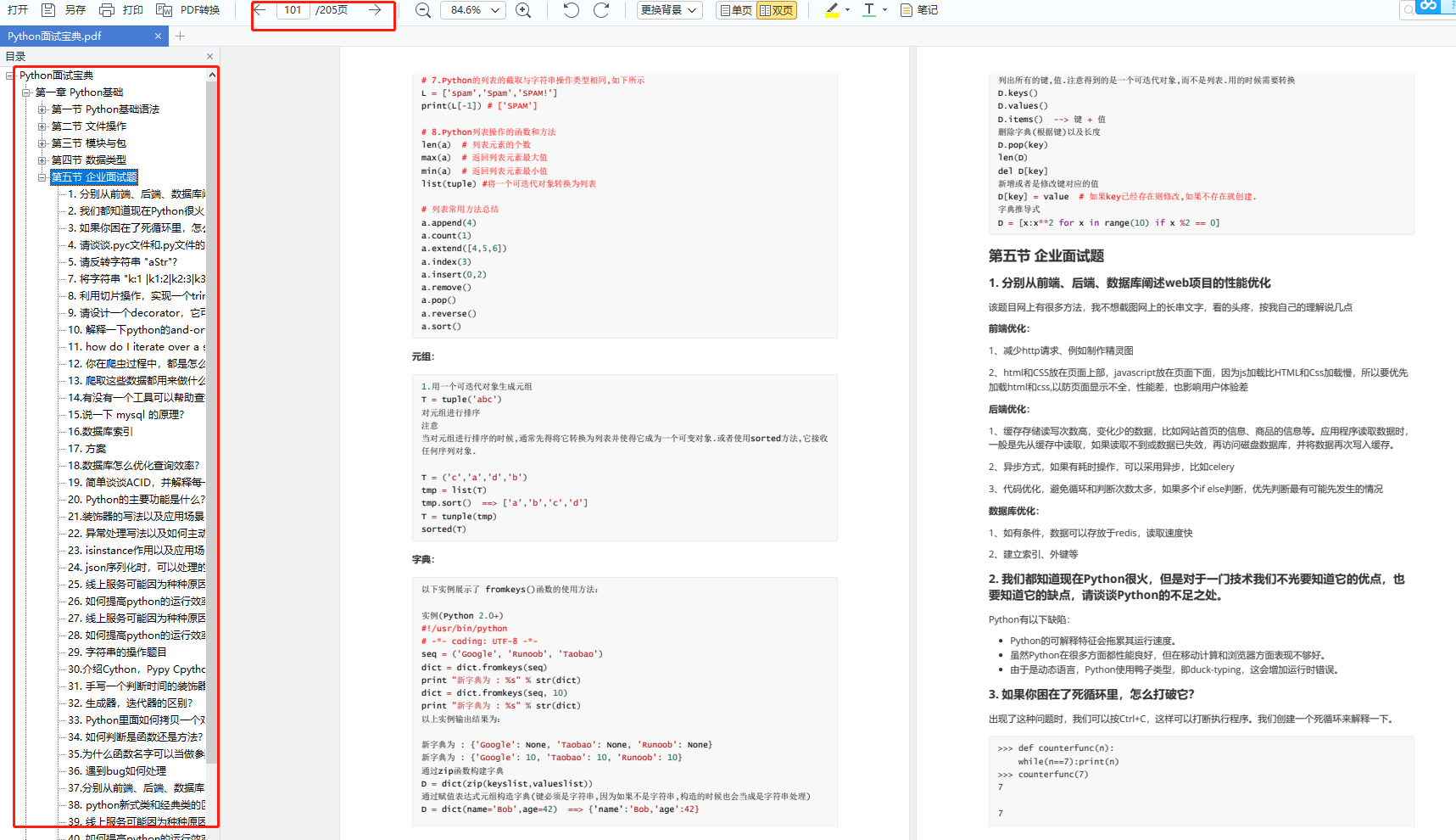
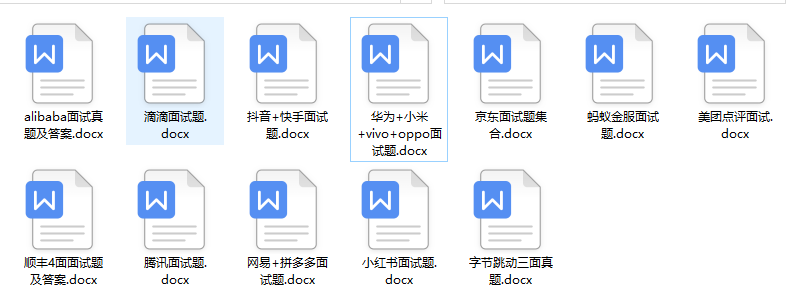
👉因篇幅有限,仅展示部分资料,这份完整版的Python全套学习资料已经上传
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**