题目
题目描述】
编程计算由“*”号围成的下列图形的面积。面积计算方法是统计*号所围成的闭合曲线中水平线和垂直线交点的数目。如下图所示,在10×10的二维数组中,有“*”围住了15个点,因此面积为15。
【输入】
10×10的图形。
【输出】
输出面积。
【输入样例】
0 0 0 0 0 0 0 0 0 0
0 0 0 0 1 1 1 0 0 0
0 0 0 0 1 0 0 1 0 0
0 0 0 0 0 1 0 0 1 0
0 0 1 0 0 0 1 0 1 0
0 1 0 1 0 1 0 0 1 0
0 1 0 0 1 1 0 1 1 0
0 0 1 0 0 0 0 1 0 0
0 0 0 1 1 1 1 1 0 0
0 0 0 0 0 0 0 0 0 0
【输出样例】
15
思路&分析
这道题,直接看不容易分析,我们不妨用图形表示。
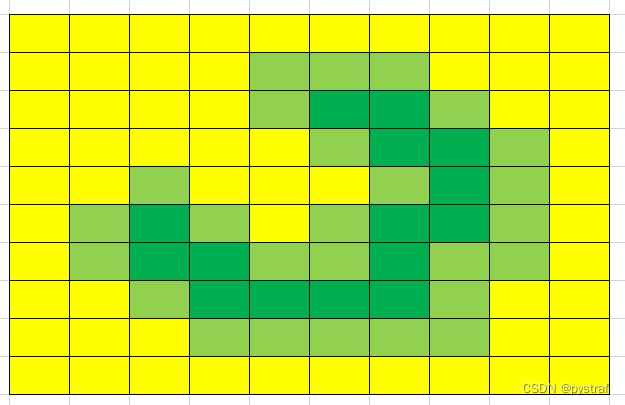
根据图形分析,我们可以想到一种思路:在1围成的图形外面全部填入2,最后统计0的数量即可。
但是,该从哪里开始呢?一定是(1,1)吗?
不一定,比如下面:
因此,只能这么处理:遍历最外圈,对于圈上的每一个点,若不为1,就进行染色,将该点,直接和间接可达点全部涂上2
(一个点的的直接可达点是它的上下左右四个方向的相邻的数不为1的格子)
那怎么做到呢?这就需要Flood Fill算法了
(flood fill可以参见:Flood Fill 算法)
代码
#include<iostream>
#include<queue>
using namespace std;
struct point {
int x, y;
};
int a[10][10];
int dx[4] = { -1,1,0,0 };
int dy[4] = { 0,0,-1,1 };
// 检查(x,y)是否可达
bool check(int x, int y) {
return x >= 0 && x < 10 && y >= 0 && y < 10 && a[x][y] == 0;
}
// (x,y)入队,染色
void push(queue<point> &q, int x, int y) {
point p = { x,y };
q.push(p);
a[x][y] = 2;
}
// 洪水填充
void flood_fill(int x, int y) {
// (x,y)本身是1
if (!check(x, y)) return;
queue<point> q;
push(q, x, y);
while (q.size()) {
point t = q.front();
q.pop();
for (int i = 0; i < 4; i++) {
int nx = t.x + dx[i], ny = t.y + dy[i];
if (check(nx, ny)) push(q, nx, ny);
}
}
}
int main() {
int ans = 0;
for (int i = 0; i < 10; i++)
for (int j = 0; j < 10; j++) cin >> a[i][j];
for (int i = 0; i < 10; i++) flood_fill(0, i); // 上面
for (int i = 0; i < 10; i++) flood_fill(9, i); // 下面
for (int i = 0; i < 10; i++) flood_fill(i, 0); // 左面
for (int i = 0; i < 10; i++) flood_fill(i, 9); // 右面
for (int i = 0; i < 10; i++)
for (int j = 0; j < 10; j++)
if (a[i][j] == 0) ans++;
cout << ans << endl;
return 0;
}
评测结果:
另一种思路
我们可以在输入序列的外面留出一圈,就是a数组中,只有a[1...10][1...10]会存储实际的值,外面都是0,这样就可以直接从(0,0)开始了
例:
实现
#include<iostream>
#include<queue>
using namespace std;
struct point {
int x, y;
};
int a[12][12]; // 外面留一圈空间
int dx[4] = { -1,1,0,0 };
int dy[4] = { 0,0,-1,1 };
// 检查(x,y)是否可达
bool check(int x, int y) {
return x >= 0 && x <= 11 && y >= 0 && y <= 11 && a[x][y] == 0;
}
// (x,y)入队,染色
void push(queue<point> &q, int x, int y) {
point p = { x,y };
q.push(p);
a[x][y] = 2;
}
// 洪水填充
void flood_fill(int x, int y) {
queue<point> q;
push(q, x, y);
while (q.size()) {
point t = q.front();
q.pop();
for (int i = 0; i < 4; i++) {
int nx = t.x + dx[i], ny = t.y + dy[i];
if (check(nx, ny)) push(q, nx, ny);
}
}
}
int main() {
int ans = 0;
for (int i = 1; i <= 10; i++)
for (int j = 1; j <= 10; j++) cin >> a[i][j];
flood_fill(0, 0);
for (int i = 1; i <= 10; i++)
for (int j = 1; j <= 10; j++)
if (a[i][j] == 0) ans++;
cout << ans << endl;
return 0;
}
测试结果: