#include <cstdio>
#include <iostream>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <deque>
#include <vector>
#include <set>
#include <algorithm>
#include <math.h>
#include <cmath>
#include <stack>
#include <iomanip>
#define mem0(a) memset(a,0,sizeof(a))
#define meminf(a) memset(a,0x3f,sizeof(a))
using namespace std;
typedef long long ll;
typedef long double ld;
typedef double db;
const int maxn=2505,inf=0x3f3f3f3f;
const ll llinf=0x3f3f3f3f3f3f3f3f;
const ld pi=acos(-1.0L);
int main() {
int i,j,k,n,ans=0;
cin >> n;
ans=0;
for (i=1;i<=n;i++) {
for (j=i;j<=n;j++) {
k=(i^j);
if (k<i+j&&k>=j&&k<=n)
ans++;
}
}
cout << ans << endl;
return 0;
}
我们对n比较小的情况打表之后,发现满足条件的k不会太大,于是可以大胆猜想对于给定的n,只要穷举最大的k就好了~
以下为打表程序,发现甚至连大于23的都没有
#include <cstdio>
#include <iostream>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <deque>
#include <vector>
#include <set>
#include <algorithm>
#include <math.h>
#include <cmath>
#include <stack>
#include <iomanip>
#define mem0(a) memset(a,0,sizeof(a))
#define meminf(a) memset(a,0x3f,sizeof(a))
using namespace std;
typedef long long ll;
typedef long double ld;
typedef double db;
const int inf=0x3f3f3f3f;
const ll llinf=0x3f3f3f3f3f3f3f3f;
const ld pi=acos(-1.0L);
bool a[100005];
int main() {
ll n,k,i;
// cin >> n >> k;
// if (k>=n) cout << "No";
for (ll n=1;n<=100000000;n++) {
mem0(a);
for (i=1;i<=n;i++) {
if (a[n%i]) break; else a[n%i]=1;
}
if (i>=23) cout << n << endl;
// cout << n << " " << i << endl;
}
return 0;
}
AC程序
#include <cstdio>
#include <iostream>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <deque>
#include <vector>
#include <set>
#include <algorithm>
#include <math.h>
#include <cmath>
#include <stack>
#include <iomanip>
#define mem0(a) memset(a,0,sizeof(a))
#define meminf(a) memset(a,0x3f,sizeof(a))
using namespace std;
typedef long long ll;
typedef long double ld;
typedef double db;
const int inf=0x3f3f3f3f;
const ll llinf=0x3f3f3f3f3f3f3f3f;
const ld pi=acos(-1.0L);
bool a[10000005];
int main() {
ll n,k,i;
cin >> n >> k;
mem0(a);
for (i=1;;i++) {
if (a[n%i]) break;
a[n%i]=1;
}
if (k>=i) cout << "No"; else cout << "Yes";
return 0;
}
Pushok the dog has been chasing Imp for a few hours already.
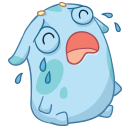
Fortunately, Imp knows that Pushok is afraid of a robot vacuum cleaner.
While moving, the robot generates a string t consisting of letters 's' and 'h', that produces a lot of noise. We define noise of string t as the number of occurrences of string "sh" as a subsequence in it, in other words, the number of such pairs (i, j), that i < j and and
.
The robot is off at the moment. Imp knows that it has a sequence of strings ti in its memory, and he can arbitrary change their order. When the robot is started, it generates the string t as a concatenation of these strings in the given order. The noise of the resulting string equals the noise of this concatenation.
Help Imp to find the maximum noise he can achieve by changing the order of the strings.
The first line contains a single integer n (1 ≤ n ≤ 105) — the number of strings in robot's memory.
Next n lines contain the strings t1, t2, ..., tn, one per line. It is guaranteed that the strings are non-empty, contain only English letters 's' and 'h' and their total length does not exceed 105.
Print a single integer — the maxumum possible noise Imp can achieve by changing the order of the strings.
4 ssh hs s hhhs
18
2 h s
1
The optimal concatenation in the first sample is ssshhshhhs.
结论题,按照s和h的比例贪心,把s比例高的排在前面就可以了~
#include <cstdio>
#include <iostream>
#include <string.h>
#include <string>
#include <map>
#include <queue>
#include <deque>
#include <vector>
#include <set>
#include <algorithm>
#include <math.h>
#include <cmath>
#include <stack>
#include <iomanip>
#define mem0(a) memset(a,0,sizeof(a))
#define meminf(a) memset(a,0x3f,sizeof(a))
using namespace std;
typedef long long ll;
typedef long double ld;
typedef double db;
const int maxn=1000005,inf=0x3f3f3f3f;
const ll llinf=0x3f3f3f3f3f3f3f3f;
const ld pi=acos(-1.0L);
char s[maxn];
struct qwe{
ll ns,nh;
};
qwe a[maxn];
bool cmp(qwe x,qwe y) {
return x.nh*y.ns<y.nh*x.ns;
}
int main() {
ll n,i,j,ta,tb,ans=0;
scanf("%I64d",&n);
for (i=1;i<=n;i++) {
scanf("%s",s);
int len=strlen(s);
a[i].ns=a[i].nh=0;
ll cnt=0;
for (j=0;j<len;j++) {
if (s[j]=='s') a[i].ns++,cnt++; else a[i].nh++,ans+=cnt;
}
}
sort(a+1,a+n+1,cmp);
ll cnt=0;
for (i=1;i<=n;i++) {
ans+=cnt*a[i].nh;
cnt+=a[i].ns;
}
printf("%I64d\n",ans);
return 0;
}
Apart from plush toys, Imp is a huge fan of little yellow birds!
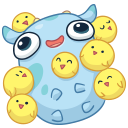
To summon birds, Imp needs strong magic. There are n trees in a row on an alley in a park, there is a nest on each of the trees. In the i-th nest there are ci birds; to summon one bird from this nest Imp needs to stay under this tree and it costs him costi points of mana. However, for each bird summoned, Imp increases his mana capacity by B points. Imp summons birds one by one, he can summon any number from 0 to ci birds from the i-th nest.
Initially Imp stands under the first tree and has W points of mana, and his mana capacity equals W as well. He can only go forward, and each time he moves from a tree to the next one, he restores X points of mana (but it can't exceed his current mana capacity). Moving only forward, what is the maximum number of birds Imp can summon?
The first line contains four integers n, W, B, X (1 ≤ n ≤ 103, 0 ≤ W, B, X ≤ 109) — the number of trees, the initial points of mana, the number of points the mana capacity increases after a bird is summoned, and the number of points restored when Imp moves from a tree to the next one.
The second line contains n integers c1, c2, ..., cn (0 ≤ ci ≤ 104) — where ci is the number of birds living in the i-th nest. It is guaranteed that .
The third line contains n integers cost1, cost2, ..., costn (0 ≤ costi ≤ 109), where costi is the mana cost to summon a bird from the i-th nest.
Print a single integer — the maximum number of birds Imp can summon.
2 12 0 4 3 4 4 2
6
4 1000 10 35 1 2 4 5 1000 500 250 200
5
2 10 7 11 2 10 6 1
11
In the first sample base amount of Imp's mana is equal to 12 (with maximum capacity also equal to 12). After he summons two birds from the first nest, he loses 8 mana points, although his maximum capacity will not increase (since B = 0). After this step his mana will be 4 of 12; during the move you will replenish 4 mana points, and hence own 8 mana out of 12 possible. Now it's optimal to take 4 birds from the second nest and spend 8 mana. The final answer will be — 6.
In the second sample the base amount of mana is equal to 1000. The right choice will be to simply pick all birds from the last nest. Note that Imp's mana doesn't restore while moving because it's initially full.
一个简单DP,真心觉得这题比D简单,D的结论不好猜