3.1、编写一个程序,利用球体半径作为输入,计算体积和表面积。
# File: 3.1.py
# -*- coding: utf-8 -*-
# Calculation of the surface area and volume of a sphere
import math
def calA_V():
print("This program calculates the surface area and volume of a sphere.")
r = eval(input("The radius is: "))
PI = math.pi
v = 4/3*PI*r**3 #**操作符进行指数运算
s = 4*PI*r**2
print("The surface area is: %.2f\nThe volume is: %.2f"%(s, v))
calA_V()

3.2、给定披萨饼的直径和价格,计算每平方英寸成本。
# File: 3.2.py
# -*- coding: utf-8 -*-
# unit cost of pizza
import math
def unitCost():
print("This program calculates the unit cost of\nthe pizza with given diameter and price.")
print("*"*20)
d = eval(input("The diameter of the pizza(inches): "))
price, currency = input("The price of the pizza: ").split() #从空格处分割字符串
price = eval(price)
Ucost = price / (math.pi*d/4)
print("This pizza cost %.2f %s per suqare inch."%(Ucost, currency))
unitCost()

3.3、编写程序,计算碳水化合物分子量。
# File:3.3.py
# -*-coding:utf-8 -*-
#
def Counting():
print("This program caculates the formula weight of carbohydrate.")
h, o, c = eval(input("The number of hydrogens, oxygens and carbons (separate by comma): "))
carb= 1.00794*h + 12.1017*o + 15.9994*c
print("The formula weight of carbohydrate is: ", carb)
Counting()
3.4、编写程序,根据闪光和雷声之间的时间差来确定雷击距离。声速1100英尺/秒,1英里=5280英尺。
3.5、Konditorei咖啡店售卖咖啡,每磅10.5美元加上运费。每份订单运费每磅0.86美元+固定成本1.5美元。编写计算订单费用的程序。
# File: 3.4.py
# -*- coding : utf-8 -*-
def thunderDis():
print("This program cauculates the distance from a thunder.")
t = eval(input("Enter the time interval between the lighting and thunder: "))
print("The distance from the thunder is: %.5f"%(t*1100/5280), "miles.")
thunderDis()
def coffeePrice():
w = eval(input("The weight of coffee (rating in pounds): "))
price = w*(10.5+1.86) + 1.5
print("The price of this order is %.3f dollars"%price)
coffeePrice()
3.6~3.7、使用坐标(x1, y1)和(x2, y2)指定平面中的两个点,编写程序,计算通过用户输入的两个点的(非垂直)的直线的斜率。编写程序,计算两点间距离。
# Flie: 3.6.py
# -*- coding: utf-8 -*-
def rateNdis(a, b):
import math
x1, y1 = a[0], a[1]
x2, y2 = b[0], b[1]
d = math.sqrt((x1 - x2)**2 + (y1 - y2)**2) #计算两点间距离
if x1 == x2:
r = "infinity" #直线垂直于x轴,斜率无穷大
elif y1 == y2:
r = 0 #直线平行于x轴,斜率为0
else:
r = (y1 - y2)/(x1 - x2)
return r, d
p1 = eval(input("The coordinates of the first point: "))
p2 = eval(input("The coordinates of the second point: "))
r, d = rateNdis(p1, p2) #将函数的返回值赋给r, d
print("The rate of this line is: ",r, "\nThe distance of this segment is: ",d)
运行结果:

3.8、格里高利润余是从1月1日到前一个新月的天数。此值用于确定复活节的日期,它由下列公式计算(使用整型算术):
C = year // 100
epact = (8 + (C // 4) - C + ((8C + 13)//25) + 11(year % 19))%30
编写程序,提示用户输入4位年份,然后输出润余的值。
# File:3.8.py
# -*- coding:utf-8 -*-
def main():
year = eval(input("Enter the year: "))
c = year//100
epact = (8 + (c//4) - c +((8*c + 13)//25) + 11*(year%19))%30
print("Epact: ",epact)
main()
3.9、使用以下公式编写程序以计算三角形的面积,其三边长度为a,b,c。
s = (a + b + c)/2
A = √s(s - a)(s - b)(s - c)
# Flie:3.9.py
# -*- coding: utf-8 -*-
import math #
def TriArea():
"This program calculates the area of a triangle according the length of its sides."
sides = eval(input("Enter the length of three sides of a triangle: ")) #此时sides为字典类型
s = sum(sides)/2
A = math.sqrt(s * (s - sides[0]) * (s - sides[1]) * (s - sides[2]))
print("The area of the triangle is: %.2f"%A)
TriArea()

3.10、编写程序,确定梯子斜靠在房子上时,达到给定高度所需的长度,并使用公式进行转换。
# File: 3.10.py
# -*- coding: utf-8 -*-
import math #
def main():
h = eval(input("The height of the ladder: "))
a = eval(input("The angle between the latter and the wall: "))
PI = math.pi
print("The length of the ladder should be %.2f"%(h/math.sin(a/180*PI)))
#计算时应将输入的角度转为弧度
main()
3.11~3.12、编写程序计算前n个自然数的和,n由用户提供。计算前n个自然数的立方和。
# File 3.11.py
# -*- coding: utf-8 -*-
import math #
def main():
n = int(input("The number of the nature number is: "))
a, b = 0, 0
for i in range(n):
a = a + i
b = b + i**2
print("The sum is: ", a)
print("The sum of quadratic sum is: ", b)
main()
3.13~3.14、编写程序对用户输入的一系列数字求和。程序应首先提示用户有多少数字要求和,然后依次提示用户输入每个数字,并在输入所有数字后打印出总和。对用户输入的一系列数字求平均值。
# File: 3.14.py
# -*- coding: utf-8 -*-
# average
def main():
n = eval(input("How many figures are there?\n"))
s = 0
for i in range(n):
a = eval(input("Enter the figures: "))
s = s + a
print("The input figures added up to be: ", s)
print("The average value of the input numbers is:", float(s/n))
main()
运行结果:
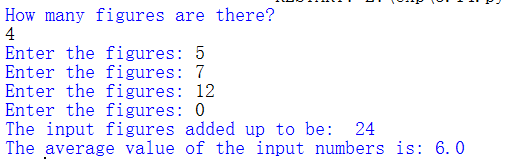
3.15、编写程序,通过对级数的项进行求和来求近似π值。
# File 3.15.py
# -*- coding: utf-8 -*-
import math #
def pi():
p = 0
n = eval(input("The length of the series is: "))
for i in range(n):
p =p + 4/(2*i+1)*(-1)**i
print("The Approximate value of π is: ",p, "\nThe deviation is: ", (math.pi-p))
pi()
运行结果:
3.16、计算第n个斐波那契数
# File: 3.16.py
# -*- coding: utf-8 -*-
def main():
"This program calculates the n-th Fibonacci number."
n = int(input("Enter the index: "))
if n > 2:
f = [1, 1]
for i in range(n-2):
f.append(f[-1] + f[-2]) #append方法在列表末尾增添一项,增添项为当前数列最后两项之和
fi = f[-1]
else:
fi = 1
print("The %d-th Fibonacci number is: %d"%(n,fi))
main()
运行结果:
3.17、你已经看到math库包含了一个计算数字平方根的函数。在本练习中,你讲编写自己的算法来计算平方根。解决这个问题的一种方法是使用猜测和检查。你首先猜测平方根可能是什么,然后看看你的猜测是多么接近。一个好的猜测法是使用牛顿法。猜测x是我们希望的根,guess是当前猜测的答案。猜测可以通过使用下一个猜测来改进:(guess+x/guess)/2。编程实现牛顿方法。程序应提示用户找到值的平方根(x)和改进猜测的次数。从猜测值x/2开始,程序应指定猜测的次数,并报告猜测的终值。
# File:3.17.py
# -*- coding: utf-8 -*-
import math #
def main():
x = float(input("Enter a positive number: "))
n = int(input("Enter the number of iterations: "))
guess = x/2
for i in range(n):
guess = (guess+x/guess)/2 #迭代计算平方根
e = abs(math.sqrt(x) - guess) #计算猜测值与真实值间误差
print("The guess value is: %f\nThe error is: %f"%(guess, e))
if e > 0.001: #如果误差过大,则增加迭代次数
print("You may want to increase the number of iterations to get a more accurate value.")