网络API可以帮助开发者实现网络URL访问调用、文件的上传与下载、网络套接字的使用等功能处理。微信开发团队提供了10个网络API接口:
- wx.request(object)接口:用于发起HTTPS请求。
- wx.uploadFile(object)接口:用于将本地资源上传到后台服务器。
- wx.downloadFile(object)接口:用于下载文件资源到本地。
- wx.connectSocket(object)接口:用于创建一个WebSocket连接。
- wx.sendSocketMessage(object)接口:用于实现通过WebSocket连接发送数据。
- wx.closeSocket(object)接口:用于关闭WebSocket连接。
- wx.onSocketOpen(CallBack)接口:用于监听WebSocket连接打开事件。
- wx.onSocketError(CallBack)接口:用于监听WebSocket错误。
- wx.onSocketMessage(CallBack)接口:用于实现监听WebSocket接收到服务器的消息事件。
- wx.onSocketClose(CallBack)接口:用于实现监听WebSocket关闭。
发起网络请求
wx.request(object)实现向服务器发送请求、获取数据等各种网络交互操作,其相关参数如表所示,一个微信小程序同时只能有5个网络请求连接,并且是HTTPS请求。
获取百度首页的数据代码如下:
<button type="primary" bindtap="getbaidutap">获取HTML数据</button>
<textarea value="{{html}}" auto-height="true" maxlength="0"></textarea>
Page({
/**
* 页面的初始数据
*/
data: {
html:''
},
getbaidutap:function(){
var that = this;
wx.request({
url: 'https:wwww.baidu.com',
data: {},
header:{'Content-Type':'application/json'},
success:function(res){
console.log(res);
that.setData({
html:res.data
})
}
})
},
/**
* 生命周期函数--监听页面加载
*/
onLoad(options) {
},
/**
* 生命周期函数--监听页面初次渲染完成
*/
onReady() {
},
/**
* 生命周期函数--监听页面显示
*/
onShow() {
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide() {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload() {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
onPullDownRefresh() {
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom() {
},
/**
* 用户点击右上角分享
*/
onShareAppMessage() {
}
})
通过网络请求的get方法获取邮政编码对应的地址信息,示例代码如下:
<view>邮政编码:</view>
<input type="text" bindinput="input" placeholder="6位邮政编码"/>
<button type="primary" bindtap="find">查询</button>
<block wx:for="{{address}}">
<block wx:for="{{item}}">
<text>{{item}}</text>
</block>
</block>
Page({
/**
* 页面的初始数据
*/
data: {
postcode:'',
address:[],
errMsg:'',
error_code:-1
},
input:function(e){
this.setData({
postcode:e.detail.value
}),
console.log(e.detail.value)
},
find:function(){
var postcode = this.data.postcode;
if(postcode != null && postcode != ''){
var self = this;
wx.showToast({
title: '正在查询,请稍候......',
icon:'loading',
duration:10000
});
wx.request({
url: 'https://v.juhe.cn/postcode/query',
data:{
'postcode':postcode,
'key':'0ff9bfccdf147476e067de994eb5496e'
},
header:{
'Content-Type':'application/x-www-form-urlencoded'
},
methos:'post',
success:function(res){
wx.hideToast();
if(res.data.error_code == 0){
console.log(res);
self.setData({
errMsg:'',
error_code:res.data.error_code,
address:res.data.result.list
})
} else {
self.setData({
errMsg:res.data.reason || res.data.reson,
error_code:res.data.error_code
})
}
}
})
}
}
})
上传文件:
wx.uploadFile(Object)接口用于将本地资源上传到开发者服务器,并在客户端发起一个HTTPS POST请求,其相关参数如表所示:
通过wx.uploadFile(Object),可以将图片上传到服务器并显示,示例代码如下:
<button type="primary" bindtap="uploadimage">上传图片</button>
<image src="{{img}}" mode="widthFix"/>
Page({
data:{
img:null,
},
uploadimage:function(){
var that=this;
wx.chooseImage({
success:function(res){
var tempFilePaths=res.tempFilePaths
upload(that,tempFilePaths);
}
})
function upload(page,path){
wx.showToast({
icon:"loading",
title: '正在上传',
}),
wx.uploadFile({
filePath: 'Path[0]',
name: 'file',
url: 'http://localhost',
success:function(res){
console.log(res);
if(res.statusCode!=200){
wx.showModal({
title: '提示',
content: '上传失败',
showCancel:false
})
return;
}
var data=res.data
page.setData({
img:path[0]
})
},
fail:function(e){
console.log(e);
wx.showModal({
title: '提示',
content: '上传失败',
showCancel:false
})
},
complete:function(){
wx.hideToast();
}
})
}
}
})
下载文件
wx.downloadFile(Object) 相关参数
通过wx.downloadFile(Object)实现下载图片
<button type="primary" bindtap="downloadimage">下载图像</button>
<image src="{{img}}" mode="widthFix" style="width: 90%;height: 500px"></image>
Page({
data:{
img:null,
},
downloadimage:function(){
var that=this;
wx.downloadFile({
url: 'http://localhost/1.jpg',
success:function(res){
console.log(res)
that.setData({
img:res.tempFilePath
})
}
})
}
})
多媒体APl
图片APl:
主要包括四个接口:
wx.chooselmage (Object) 接口 用于从本地相册选择图片或使用相机拍照。
wx.prrviewImage (Objct) 接口 用于预览图片。
wx.getImageInfo (Objct) 接口 用于获取图片信息。
wx.savelmageToPhotosAIbum (Object) 接口 用于保存图片到系统相册。
选择图片或拍照
wx.chooselmage (Object)相关参数
wx.chooseImage({
count:2,
sizeType:['album','compressed'],
sourceType:['album','camera'],
success:function(res){
var tempFilePaths=res.tempFilePaths
var tempFiles=res.tempFiles;
console.log(tempFilePaths)
console.log(tempFiles)
}
})
预览图片
wx.prrviewImage (Objct)相关参数
wx.previewImage({
current:"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/2.png",
urls: ["http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/1.png",
"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/2.png",
"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/3.png",
]
})
获取图片信息
wx.getImageInfo (Objct)相关参数
wx.chooseImage({
success:function(res){
wx.getImageInfo({
src: res.tempFilePaths[0],
success:function(e){
console.log(e.width)
console.log(height)
}
})
},
})
wx.savelmageToPhotosAIbum (Object)相关参数
wx.chooseImage({
success:function(res){
wx.saveImageToPhotosAlbum({
filePath: res.tempFilePaths[0],
success:function(e){
console.log(e)
}
})
},
})
录音APl
主要包括两个APl接口:
wx.startRecord (Object) 接口 用于实现开始录音。
wx.stopRecord (Object) 接口 用于实现主动调用停止录音。
wx.startRecord (Object)相关参数
停止录音
wx.startRecord({
success:function(res){
var tempFilePath=res.tempFilePath
},
fail:function(res){
}
})
setTimeout(function(){
wx.stopRecord()
},10000)
音频播放控制API
1.播放音乐
2.获取音乐播放状态
3.控制音乐播放进度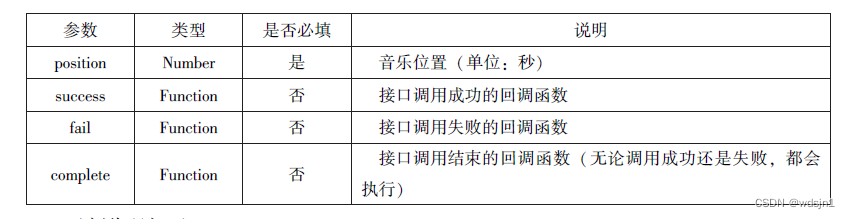
案例展示
<view class="container">
<image class="bgaudio" src="{{changedImg?music.coverImg:'../../images/tp.jpg'}}"/>
<view class="control-view">
<image src="../../images/1 .png" bindtap="onPositionTap" data-how="0"/>
<image src="../../images/{{isplaying?'pause':'play'}}.png" bindtap="onAudioTap"/>
<image src="../../images/2.jpg" bindtap="onStopTap"/>
<image src="../../images/2.jpg" bindtap="onPositionTap" data-how="1"/>
</view>
</view>
.bgaudio{
height: 350rpx;
width: 350rpx;
margin-bottom: 100rpx;
}
.control-viewimage{
height: 64rpx;
width: 64rpx;
margin: 30rpx;
}
{
"navigationBarTitleText":"music"
}
Page({
data:{
isplaying:false,
coverImg:"",
changedImg:false,
music:{
"url":"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/09/117e4a1b405195b18061299e2de89597.mp3",
"title":"盛晓玫-有一天",
"coverImg":"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/09/f604297140c9681880cc3d3e581f7724.jpg"
},
},
onLoad:function(){
this.onAudioState();
},
onAudioTap:function(event){
if(this.data.isplaying){
wx.pauseBackgroundAudio();
}else{
let music=this.data.music;
wx.playBackgroundAudio({
dataUrl: music.url,
title:music.title,
coverImgUrl:music.coverImg
})
}
},
onStopTap:function(){
let that=this;
wx.stopBackgroundAudio({
success:function(){
that.setData({
isplaying:false,changedImg:false
});
}
})
},
onPositionTap:function(event){
let how=event.target.dataset.how;
wx.getBackgroundAudioPlayerState({
success:function(res){
let status=res.status;
if(status===1){
let duration=res.duration;
let currentPosition=res.currentPosition;
if(how==="0"){
let position=currentPosition-10;
if(position<0){
position=1;
}
wx.setBackgroundAudio({
position:position
});
wx.showToast({
title: '快退10s',
duration:500
});
}
if(how==="1"){
let position=currentPosition+10;
if(position>duration){
position=duration-1;
}
wx.seekBackgroundAudio({
position: position
});
wx.showToast({
title: '快进10s',
duration:500
});
}
}else{
wx.showToast({
title: '音乐未播放',
duration:800
});
}
}
})
},
onAudioState:function(){
let that=this;
wx.onBackgroundAudioPlay(function(){
that.setData({
isplaying:true,
changedImg:true
});
console.log("on play");
});
wx.onBackgroundAudioPause(function(){
that.setData({
isplaying:false
});
console.log("on pause");
});
wx.onBackgroundAudioStop(function(){
that.setData({
isplaying:false,
changedImg:false
});
console.log("on stop")
});
}
})
文件API:
主要包括以下5个API接口:
- wx.saveFile(Object)接口:用于保存文件到本地,主要包括以下5个API接口
- wx.getSavedFileList(Object)接口:用于获取本地已保存的文件列表
- wx.getSaveFileInfo(Object)接口:用于获取本地文件的文件信息
- wx.removeSaveFile(Object)接口:用于删除本地存储的文件
- wx.openDocument(Object)接口:用于新开页面打开文档,支持格式:doc、xls、 2. 获取ppt、pdf、docx、xlsx、ppts
保存文件:
wx.saveFile(Object)用于保存文件到本地,其相关参数如表所示:
Page({
saveImg:function(){
wx.chooseImage({
count: 1,
sizeType:['original','compressed'],
sourceType:['album','camera'],
success:function(res){
var tempFilePaths = res.tempFilePaths[0]
wx.saveFile({
tempFilePath: tempFilePaths,
success:function(res){
var saveFilePath = res.savedFilePath;
console.log(saveFilePath)
}
})
}
})
}
})
获取本地文件的文件信息:
wx.getSaveFileInfo(Object)接口用于获取本地文件的文件信息,此接口只能用于获取已保存到本地的文件,若需要获取临时文件信息,则使用wx.getFileInfo(Object)接口,其相关参数如表所示:
Page({
getSaveFileInfo:function(){
wx.chooseImage({
count: 1,
sizeType:['compressed','original'],
sourceType:['album','camera'],
success:function(res){
var tempFilePaths = res.tempFilePaths[0]
wx.saveFile({
tempFilePath: tempFilePaths,
success:function(res){
var saveFilePath = res.savedFilePath;
wx.getSavedFileInfo({
filePath: saveFilePath,
success:function(res){
console.log(res.size);
}
})
}
})
}
})
}
})
获取本地文件的文件信息:
wx.getSaveFileInfo(Object)接口用于获取本地文件的文件信息,此接口只能用于获取已保存到本地的文件,若需要获取临时文件信息,则使用wx.getFileInfo(Object)接口,其相关参数如表所示:
Page({
getSaveFileInfo:function(){
wx.chooseImage({
count: 1,
sizeType:['compressed','original'],
sourceType:['album','camera'],
success:function(res){
var tempFilePaths = res.tempFilePaths[0]
wx.saveFile({
tempFilePath: tempFilePaths,
success:function(res){
var saveFilePath = res.savedFilePath;
wx.getSavedFileInfo({
filePath: saveFilePath,
success:function(res){
console.log(res.size);
}
})
}
})
}
})
}
})
删除本地文件:
wx.removeSaveFile(Object)接口用于删除本地存储的文件,其相关参数如表所示
Page({
removeFile:function(){
wx.getSavedFileList({
success:function(res){
if(res.fileList.length > 0){
wx.removeSavedFile({
filePath: res.fileList[0].filePath,
complete:function(res){
console.log(res)
}
})
}
}
})
}
})
打开文档:
wx.openDocument(Object)接口用于新开页面打开文档,支持格式有doc、xls、ppt、pdf、docx、xlsx、pptx,其相关参数如表所示:
Page({
openDocument:function(){
wx.downloadFile({
url: "http://localhost/fm2.pdf",
success:function(res){
var tempFilePath = res.tempFilePath;
wx.openDocument({
filePath: tempFilePath,
success:function(res){
console.log("打开成功")
}
})
}
})
}
})
6.4 本地数据及缓存 APl
保存数据
wx.seSyorage(Object) 相关参数
wx.setStorage({
key:'name',
data:'sdy',
success:function(res){
console.log(res)
}
})
wx.setStorageSync(key,data)是同步接口,其参数只有key和data。
示例代码如下:
wx.setStorageSync('age','25')
6.4.2获取数据
wx.setStorage(Object)相关参数
wx.getStorage({
key:'name',
success:function(res){
console.log(res.data)
},
})
wx.setStorageSync(key) 示例代码如下:
try{
var value =wx.getStorageSync('age')
if(value){
console.log("获取成功"+value)
}
}catch(e){
console.log("获取失败")
}
删除数据
wx.removeStorage(Object)相关参数
wx.removeStorage({
key: 'name',
success:function(res){
console.log("删除成功")
},
fail:function(){
console.log("删除失败")
}
})
wx.removeStorageSync(key)示例代码如下:
try{
wx.removeStorageSync('name')
}catch(e){
}
6.4.4清空数据
1.wx.clearStorage()
示例代码如下:
wx.getStorage({
key: 'name',
success:function(res){
wx.clearStorage()
},
})
wx.clearStorageSync() 示例代码如下:
try{
wx.clearStorageSync()
}catch(e){
}
位置信息APl
获取位置信息
wx.getLocation(Object) 相关参数
wx.getLocation(Object) 成功返回相关信息
示例代码如下:
wx.getLocation({
type:'wgs84',
success:function(res){
console.log("经度"+res.longitude);
console.log("纬度度"+res.latitude);
console.log("速度"+res.longitude);
console.log("位置的精确度"+res.accuracy);
console.log("水平精确度"+res.horizontalAccuracy);
console.log("垂直精确度"+res.verticalAccuracy);
}
})
选择位置信息
wx.chooseLocation(Object)相关参数
wx.chooseLocation(Object)成功返回相关参数
示例代码如下:
wx.chooseLocation({
success:function(res){
console.log("位置的名称"+res.longitude)
console.log("位置的地址"+res.accuracy)
console.log("位置的纬度"+res.horizontalAccuracy)
console.log("位置的纬度"+res.verticalAccuracy)
}
})
显示位置信息
wx.openLocation(Object)相关参数
示例代码如下:
wx.getLocation({
type:'gcj02',
success:function(res){
var latitude=res.latitude
var longitude=res.longitude
wx.openLocation({
latitude: latitude,
longitude: longitude,
scale:10,
name:'智慧国际酒店',
address:'西安市长安区西长安区300号'
})
}
})
设备相关APl
获取系统信息
wx.getSystemInfo(Object)接口、wx.getSystemInfoSync()相关参数
wx.getSystemInfo(Object)接口、wx.getSystemInfoSync()成功返回相关信息
示例代码如下:
wx.getSystemInfo({
success:function(res){
console.log("手机型号:"+res.model);
console.log("设备像素比:"+res.pixelRatio);
console.log("窗口的高度:"+res.windowHeight);
console.log("窗口的宽度:"+res.windowWidth);
console.log("微信的版本号:"+res.version);
console.log("操作系统版本:"+res.system);
console.log("客户端平台:"+res.platform);
},
})
网络状态
获取网络状态
wx.getNetworkType(Object)相关参数
wx.getNetworkType({
success:function(res){
console.log(res.networkType)
},
})
监听网络状态变化
wx.onNetworkStatusChange({function(res){
console.log("网络是否链接"+res.isConnected)
console.log("变化后的网络类型"+res.networkType)
}
})
拨打电话
wx.makePhoneCall(Object) 相关参数
wx.makePhoneCall({
phoneNumber: '18092585093',
})
扫描二维码
wx.scanCode(Object) 相关参数
wx.scanCode(Object) 成功返回相关信息
示例代码如下:
允许从相机和相册扫码
wx.scanCode({
success:(res)=>{
console.log(res.result)
console.log(res.scanType)
console.log(res.charSet)
console.log(res.path)
}
})
只允许从相机扫码
wx.scanCode({
onlyFromCamera:true,
success:(res) => {
console.log(res);
}
})