c++ combine使用
In this short post, I will show you how to create a generic Networking API that you can reuse in your own implementations using generics and Combine. We won’t go in-depth of explaining elements of the Combine framework, instead, I will show you an example on how to use generics to boost your code reusability.
在这篇简短的文章中,我将向您展示如何创建通用的网络API,您可以使用通用和组合在自己的实现中重用它们。 我们将不深入解释Combine框架的元素,而是向您展示一个有关如何使用泛型来提高代码可重用性的示例。
You will see here:
您将在这里看到:
- Mix of generics and Combine Framework to create a reusable networking layer. 泛型和Combine Framework的混合,以创建可重用的网络层。
- Display elements from a network response in a SwiftUI list. 在SwiftUI列表中显示网络响应中的元素。
Don’t want to keep reading give me the code!. 😡
不想继续读给我的代码! 。 😡
Let’s divide this into 3 simple steps…
让我们将其分为3个简单的步骤…
Step 1: Build the generic networking layer.
步骤1:构建通用网络层。
Imagine you have to build an API that handles a request, handles errors, decodes the response, and also retries the network call if it fails 😳.
假设您必须构建一个API,该API可以处理请求,处理错误,解码响应以及在失败时重试网络调用。
Well using Combine it will look as simple as this…
好吧,使用Combine看起来就像这样简单……
What do we have…
我们有什么...
1 .- A protocol that has an URLSession
and a function that returns a Publisher
.
1 .-一种协议,具有URLSession
和返回Publisher
的函数。
2 .- Extending the protocol so we can have a default implementation.
2 .-扩展协议,以便我们可以使用默认实现。
3 .- Here is how we improve our networking layer, if you don’t see it already I highly recommend checking this talk to see what is new in networking for iOS. We will use the dataTaskPublisher
from the Combine
framework instead of using a simple dataTask.
3 .-这是我们如何改善网络层的方法,如果您还没有看到的话,我强烈建议您检查一下本讲座,以了解iOS的网络方面的新功能。 我们将使用Combine
框架中的dataTaskPublisher
,而不是使用简单的dataTask .
That’s it, now you have a generic API that can Decode any kind of object! 🙃
就这样,现在您有了可以解码任何类型对象的通用API! 🙃
As you can see the code is more readable and concise, now let me show you how to use it in your own client…
如您所见,该代码更具可读性和简洁性,现在让我向您展示如何在自己的客户端中使用它…
Step 2: Adopting the Protocol in your own client.
步骤2:在您自己的客户端中采用协议。
What do we have…
我们有什么...
1 .- The URLSession
to satisfy the CombineApi
protocol.
1 .-的URLSession
满足CombineApi
协议。
2 .- A couple of convenient initializers to facilitate instantiation.
2 .-几个方便的初始化程序,以方便实例化。
3.- MovieFeed
is a model that contains everything we need to perform a URLRequest
, the code of it is irrelevant for this post because you can model it as you wish, but here is an idea.
MovieFeed
是一个模型,其中包含执行URLRequest
所需的一切,它的代码与本文无关, 因为您可以根据需要对其进行建模,但这是一个主意 。
4.- Here we call the execute
method passing as parameters a request, the meta-type of the object that we want to decode, and an integer to define the number of retries in case it fails.
4.-在这里,我们称为execute
方法,将请求作为参数传递,将要解码的对象的元类型作为参数传递,并定义一个整数,以定义失败时的重试次数。
With all of this, you can create a model that conforms to the ObservableObject
protocol to wrap your client networking logic, like this…
有了这些,您就可以创建一个符合ObservableObject
协议的模型来包装您的客户端网络逻辑,就像这样……
Step 3: Defining an ObservableObject.
步骤3:定义ObservableObject。
1 .- A class that conforms to ObservableObject
, it will be able to notify updates before the object has changed.
1 .-符合ObservableObject
类,它将能够在对象更改之前通知更新。
2 .- An AnyCancellable
Subscriber to cancel the subscription on deallocation.
2 .- AnyCancellable
订阅服务器,以取消分配时的订阅。
3 .- A Publisher
that streams the changes in the collection.
3 .- Publisher
,用于流式传输集合中的更改。
4 .- An instance of your client. 😉
4 .-您的客户端的实例。 😉
5.- Combine uses the .sink
closure that attaches a subscriber with closures that gets executed on completion and when there is a new value available, by assigning the value to the movies
publisher, it will publish the changes 🚀.
5.-结合使用. sink
. sink
关闭,将订户附加到完成时执行的关闭,当有新值可用时,通过将值分配给movies
发布者,它将发布更改🚀。
The cool thing here is that you can also reuse this code in an UIKit application but just for fun let’s see how it will do it using SwiftUI…
这里很酷的事情是,您也可以在UIKit应用程序中重用此代码,但仅出于娱乐目的,让我们看看如何使用SwiftUI来实现此目的……
- Since iOS 14 you can build apps using just Swift UI, the
@main
keyword and theApp
protocol defines the starting point of your app.-从iOS 14开始,您可以仅使用Swift UI构建应用程序,
@main
关键字和App
协议定义了应用程序的起点。Since iOS 14 you can use
@StateObject
as well as@ObservedObject
, both works in the same way but the first one has improvements in performance, theMoviesProvider
is the@ObservableObject
we just created.从iOS 14开始,您可以使用
@ StateObject
和@ ObservedObject
,两者的工作方式相同,但是第一个在性能上有所改进,MoviesProvider
是我们刚刚创建的@ObservableObject
。Since iOS 14 you also need to have a struct that conforms to the
@Scene
protocol to “wrap” your application.从iOS 14开始,您还需要具有符合
@ Scene
协议的结构来“包装”您的应用程序。- A navigation view, if you want to perform any navigation tasks. 导航视图(如果要执行任何导航任务)。
Big shout out to generics, if you didn’t notice already, they are everywhere! As you can see the
List
view is a container that takes an array of any kind of elements, a keypath (This id comes from theIdentifiable
protocol and every movie view model has one check it here.).向仿制药大声疾呼,如果您还没有注意到,它们无处不在! 如您所见,“
List
视图是一个容器,该容器采用任何类型的元素组成的数组,一个键路径( ID来自可Identifiable
协议,每个电影视图模型都 在此处进行检查 。 )。List
has a closure that provides an element from the array and it returns any kind of view that conforms toView
protocol, if you are thinking inUIKit
this is the equivalent of a cell. (repo for the movie row implementation here.)List
有一个提供数组元素的闭包,它返回符合View
协议的任何类型的视图,如果您在UIKit
中认为这等效于一个单元格。 ( 此处 为电影行实现的回购 。 )
Here is the app! in the next post I will show you how to load images in the items of your list using combine and how to create a custom Image
view to cache them.
这是应用程序! 在下一篇文章中,我将向您展示如何使用Combine将图像加载到列表项中,以及如何创建自定义Image
视图以对其进行缓存。
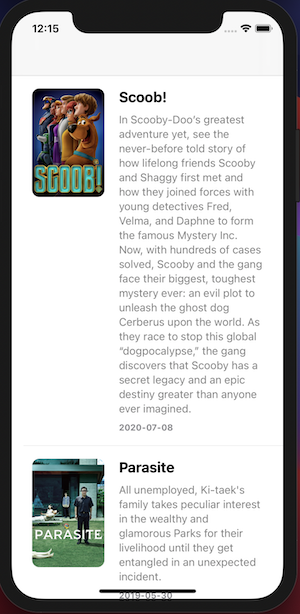
Here is the full repo. 🤖
这是完整的仓库 。 🤖
c++ combine使用