django创建网站
A sitemap is a XML file on your website that search engines read to intelligently crawl you site. It not only provides information about the pages and files you find important, but it also states when the page was last updated and how often the page is changed.
站点地图是您网站上的XML文件,搜索引擎可以读取该XML文件来对您的网站进行智能爬网。 它不仅提供有关您发现重要的页面和文件的信息,而且还指出页面的最新更新时间和更改频率。
If you are using Django, create you sitemap automatically with the sites and sitemap frameworks.
如果您使用的是Django,请使用网站和站点地图框架自动创建站点地图。
安装网站应用 (Install the sites app)
env > mysite > mysite > settings.py
env> mysite> mysite> settings.py
INSTALLED_APPS = [
'main.apps.MainConfig',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'django.contrib.sites', #add sites to installed apps
]SITE_ID = 1 #define the site id
First, install the sites app. Then define the SITE_ID
in the settings. The sites framework will be used to define the name of the site in the sitemap.
首先,安装站点应用程序。 然后在设置中定义SITE_ID
。 网站框架将用于在站点地图中定义网站的名称。
运行迁移 (Run migrations)
macOS Terminal
macOS终端
(env)User-Macbook:mysite user$ python3 manage.py migrate
Windows Command Prompt
Windows命令提示符
(env)C:\Users\Owner\desktop\env\mysite> py manage.py migrate
Run migrate
in the command prompt to add the sites table to the database.
在命令提示符下运行migrate
将sites表添加到数据库。
创建一个超级用户 (Create a superuser)
macOS Terminal
macOS终端
(env)User-Macbook:mysite user$ python3 manage.py createsuperuser
Username (leave blank to use 'owner'): admin
Email address:
Password: *****
Password (again): *****
Superuser created successfully.(env)User-Macbook:mysite user$ python3 manage.py runserver
Windows Command Prompt
Windows命令提示符
(env) C:\Users\Owner\Desktop\Code\env\mysite>py manage.py createsuperuser
Username (leave blank to use 'owner'): admin
Email address:
Password: *****
Password (again): *****
Superuser created successfully.(env) C:\Users\Owner\Desktop\Code\env\mysite>py manage.py runserver
If you haven’t already, create a superuser so you can access the Django administration built-in your project.
如果还没有,请创建一个超级用户,以便您可以访问项目中内置的Django管理。
登录到Django管理 (Login to the Django administration)
django.contrib.sites
registers a signal handler that creates a default site named example.com. You will need to correct the name and domain of your Django project by logging in to the Django admin at http://127.0.0.1:8000/admin/.
django.contrib.sites
注册了一个信号处理程序,该信号处理程序创建了一个名为example.com的默认站点。 您需要通过登录http://127.0.0.1:8000/admin/上的Django admin来更正Django项目的名称和域。
Find the link Sites and click it.
找到链接站点 ,然后单击它。
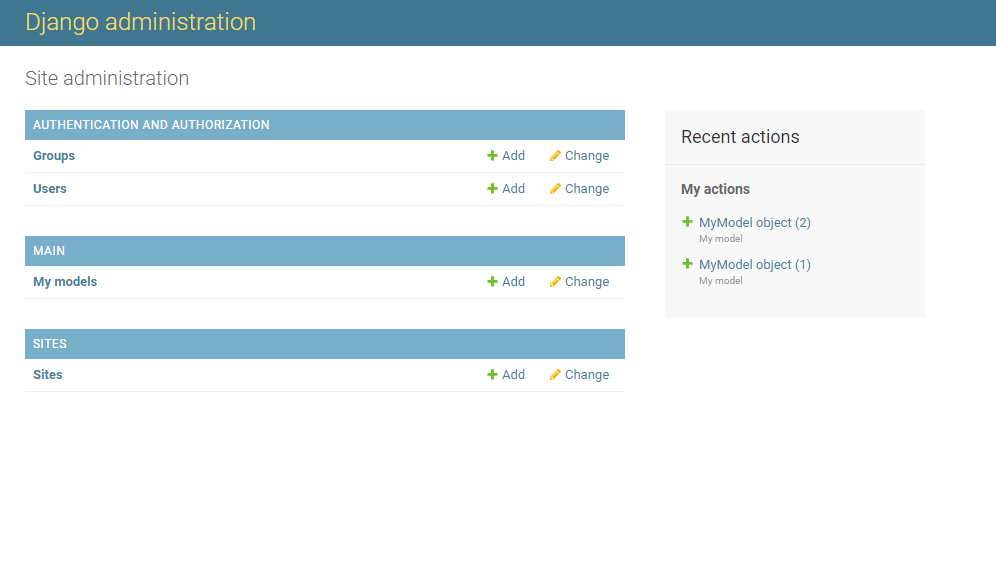
You will then see a site domain name and display name listed, both of which should be changed to the appropriate website name.
然后,您将看到列出的站点域名和显示名称,两者都应更改为适当的网站名称。
For development, use the local host 127.0.0.1:8000. For production, change the names to your actual domain name.
为了进行开发,请使用本地主机127.0.0.1:8000。 对于生产,请将名称更改为您的实际域名。
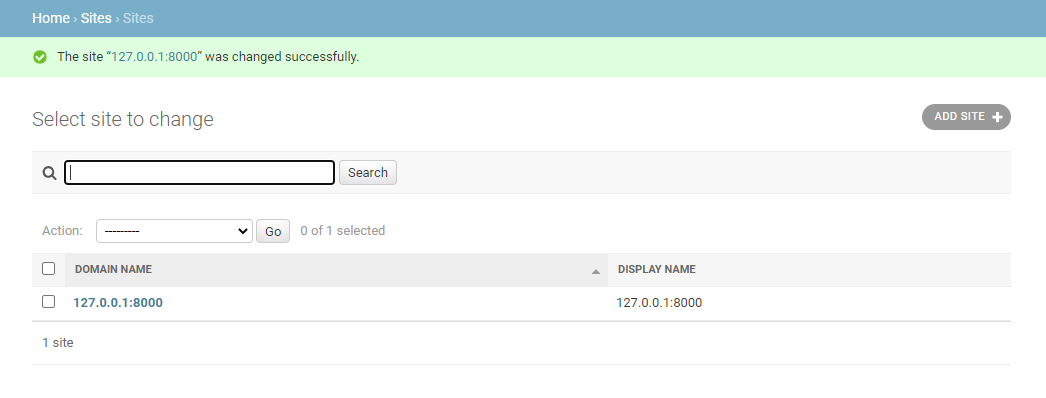
安装站点地图应用 (Install the sitemap app)
env > mysite > mysite > settings.py
env> mysite> mysite> settings.py
INSTALLED_APPS = [
'main.apps.MainConfig',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'django.contrib.sitemaps', #add sitemaps to installed apps
]TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
Now that we have defined the name of the site, we can use the sitemap framework.
现在我们已经定义了站点的名称,我们可以使用站点地图框架。
To install the sitemap app, add 'django.contrib.sitemaps'
to INSTALLED_APPS
. Then scroll down to TEMPLATES
making sure DjangoTemplates
is listed (it should be there by default) and the APP_DIRS
is set to True
.
要安装站点地图应用,请将'django.contrib.sitemaps'
添加到INSTALLED_APPS
。 然后向下滚动到TEMPLATES
以确保列出了DjangoTemplates
(默认情况下应该在其中)并且APP_DIRS
设置为True
。
创建站点地图文件 (Create a sitemap file)
env > mysite > main > (New File) sitemaps.py
env> mysite> main>(新文件)sitemaps.py
from django.contrib.sitemaps import Sitemap
from .models import Article
class ArticleSitemap(Sitemap):
changefreq = "weekly"
priority = 0.8
protocol = 'http' def items(self):
return Article.objects.all() def lastmod(self, obj):
return obj.article_published
def location(self,obj):
return '/blog/%s' % (obj.article_slug)
Creating a sitemap is similar to creating a Django model. First, create a new file called sitemaps.py in your app’s folder. This is the folder with models.py and views.py.
创建站点地图类似于创建Django模型 。 首先,在应用程序的文件夹中创建一个名为sitemaps.py的新文件。 这是包含models.py和views.py的文件夹。
Then import the Sitemap
class from django.contrib.sitemaps
at the top of the file. Also import the model containing all of the articles/pages you want on the sitemap, in this case the model Article
.
然后从文件顶部的django.contrib.sitemaps
导入Sitemap
类。 还要导入包含您想要在站点地图上的所有文章/页面的模型,在本例中为模型Article
。
Next, create a sitemap class named ArticleSitemap
that extends Sitemap
class.
接下来,创建一个名为ArticleSitemap
的站点地图类,以扩展Sitemap
类。
We can then add the attributes changefreq
, priority
, and protocol
.
然后,我们可以添加属性changefreq
, priority
和protocol
。
changefreq
is how frequently the content on the page changes. The value can be:
changefreq
是页面上的内容更改的频率。 该值可以是:
'always'
'always'
'hourly'
'hourly'
'daily'
'daily'
'weekly'
'weekly'
'monthly'
'monthly'
'yearly'
'yearly'
'never'
'never'
priority
is the importance of the pages in relationship to the other pages on your site. The value can be any where from 0.0
to 1.0
.
priority
是页面相对于您网站上其他页面的重要性。 该值可以是0.0
到1.0
任何值。
protocol
is either 'https'
or 'http'
. In this case, we will use http given the local host domain is http://127.0.0.1:8000.
protocol
是'https'
或'http'
。 在这种情况下,鉴于本地主机域为http://127.0.0.1:8000 ,我们将使用http。
The items()
method returns a list of all of the model objects we want listed in the sitemap.
items()
方法返回我们要在站点地图中列出的所有模型对象的列表。
The lastmod()
method returns the last time the model object was modified, meaning you need to return a defined publication date field specified in the model for it to work properly.
lastmod()
方法返回上一次修改模型对象的时间,这意味着您需要返回模型中指定的定义的发布日期字段,以使其正常工作。
Finally, the location()
method returns the URL location of the article. By default, the sitemap framework calls get_absolute_url()
.
最后, location()
方法返回文章的URL位置。 默认情况下,站点地图框架调用get_absolute_url()
。
So if your article URLs is anything other than http://127.0.0.1:8000/name-of-article, you need to add the location() method and return the appropriate slugs listed before the article slug.
因此,如果您的文章URL不是http://127.0.0.1:8000/name-of-article ,则需要添加location()方法并返回在文章段之前列出的适当段。
As per the example above, the URL location is now http://127.0.0.1:8000/blog/name-of-article.
按照上面的示例,URL位置现在为http://127.0.0.1:8000/blog/name-of-article 。
添加站点地图网址 (Add the sitemap URL)
env > mysite > main > urls.py
env> mysite> main> urls.py
from django.urls import path
from . import views
from django.contrib.sitemaps.views import sitemap
from .sitemaps import ArticleSitemapapp_name = "main"sitemaps = {
'blog':ArticleSitemap
}urlpatterns = [
path("", views.homepage, name="homepage"),
path('sitemap.xml', sitemap, {'sitemaps': sitemaps}, name='django.contrib.sitemaps.views.sitemap'),]
The last thing that needs to be done to view your sitemap is to add its URL to the list of app URLs. Import sitemap and ArticleSitemap
from the appropriate files. Then add a sitemap dictionary that maps to the sitemap class ArticleSitemap. Finally, add the URL pattern that points to the sitemap.
查看站点地图的最后一件事是将其URL添加到应用程序URL列表中。 从适当的文件中导入站点地图和ArticleSitemap
。 然后添加一个映射到站点地图类ArticleSitemap的站点地图字典。 最后,添加指向站点地图的URL模式。
查看站点地图 (View the sitemap)
The sitemap is now available at http://127.0.0.1:8000/sitemap.xml/. When you go to this domain, it should list all of the URLs defined in the Article model along with the attributes added in sitemaps.py.
现在可以从http://127.0.0.1:8000/sitemap.xml/获得站点地图。 转到该域时,它应该列出在Article模型中定义的所有URL以及sitemaps.py中添加的属性。
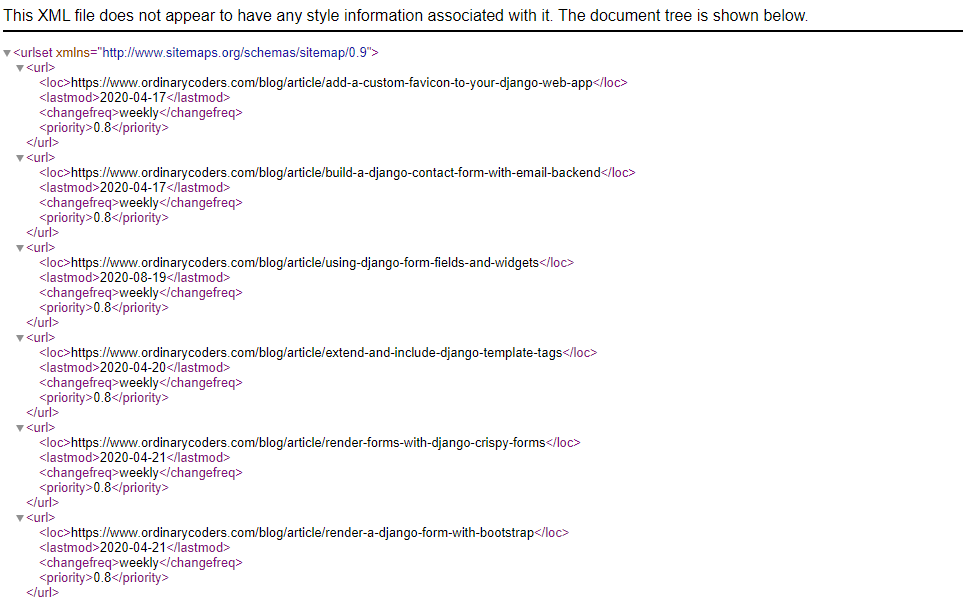
Originally published at https://www.ordinarycoders.com.
最初发布在 https://www.ordinarycoders.com上 。
翻译自: https://levelup.gitconnected.com/how-to-create-a-sitemap-in-django-bdb1caead3a8
django创建网站