ruby 访问者模式
Even if you’ve never heard of object-orientation, I’m sure you have heard of the term ‘abstraction’. Today, I want to share some insights on what these terms mean in the world of programming and how we use them as tools to make our code both beautifully simplistic and effective.
即使您从未听说过面向对象,我相信您也听说过“抽象”一词。 今天,我想就这些术语在编程世界中的含义以及我们如何将它们用作工具来使我们的代码既简单又有效的观点发表一些见解。
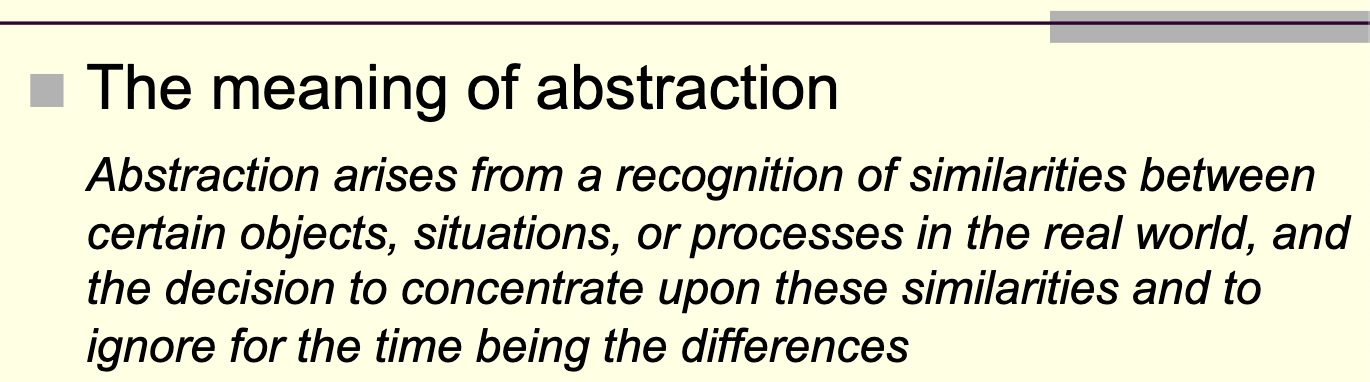
Given the definition of abstraction, you can probably think of an example of this in everyday life. This is something that represents the idea of an ‘object’.
给定抽象的定义,您可能会想到日常生活中的一个例子。 这代表了“对象”的概念。
Think about when you clicked this link on your smartphone… is that a real button? No, it’s an abstraction of a “real” button. Isn’t a button just an abstraction for action as well? When you click on a link, you’re not really physically touching or opening anything, it’s an abstraction!
想想当您单击智能手机上的此链接时,这是一个真正的按钮吗? 不,这是“真实”按钮的抽象。 按钮不仅是动作的抽象吗? 当您单击链接时,您实际上并没有真正触摸或打开任何东西,这是一种抽象!
If you’ve ever looked at sheet music, the notes on a page are not the actual notes. They are symbols that indicate the specific sound and the length of the sound that they are referring to.
如果您曾经看过乐谱,则页面上的笔记不是实际的笔记。 它们是表示特定声音及其所指声音长度的符号。
It definitely takes some time to fully understand this concept. Once you do, I promise you’ll see it everywhere.
完全理解此概念肯定需要一些时间。 完成后,我保证您会在任何地方看到它。
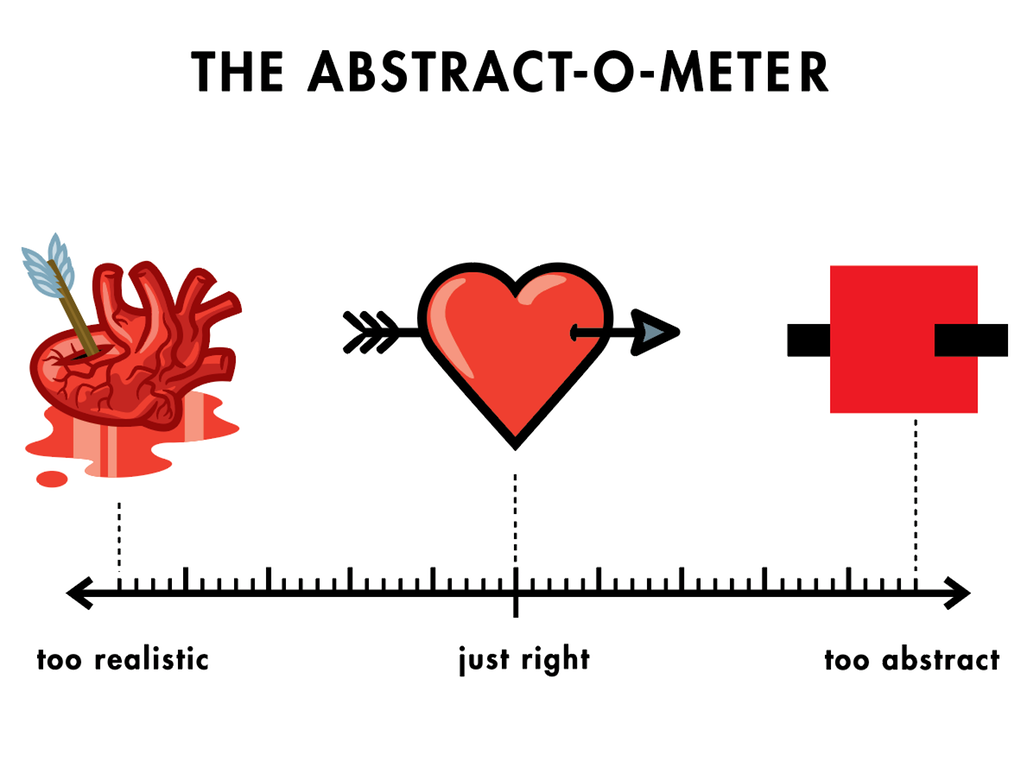
什么是物体? (What are Objects?)
You may be wondering how this ties into object-orientation. An object in programming can be defined as something with specific characteristics and functions that we use to get things done.
您可能想知道这与对象定向的关系。 可以将编程中的对象定义为具有特定特征和功能的事物,我们可以使用它们来完成任务。
In Ruby, everything is an object. For example, a word(s) wrapped in quotations is a string. Strings have built-in functions like .capitalize or .length. Integers are objects as well. Integers also have built-in functions like .to_s, which turns an integer into a string. You can ask for a list of methods for a specific object by typing .methods.
在Ruby中,一切都是对象。 例如,用引号引起来的单词是一个字符串。 字符串具有内置功能,例如.capitalize或.length。 整数也是对象。 整数还具有诸如.to_s之类的内置函数,该函数将整数转换为字符串。 您可以通过键入.methods来请求特定对象的方法列表。
string.methods
If a value has been assigned to a variable, we may not remember what type of object it is. We can find out by typing the name of the variable followed by .class. This is important so that we don’t try to perform a string method like .capitalize on an integer — giving us a TypeError.
如果已将值分配给变量,我们可能不记得它是什么类型的对象。 我们可以通过键入变量名称后跟.class来找出。 这很重要,因此我们不要尝试对整数执行.capitalize之类的字符串方法-为我们提供TypeError。
x = 90
x.class
=> string
As the gods/goddesses of our program, we can use the concept of abstraction to create our own objects. Of course, this comes with some responsibility. It’s important to maintain logical naming conventions and attributes. Otherwise, other people that collaborate with us on a project will be very confused when you name your ‘dog’ class ‘being’ and give it an attribute of ‘value’. This is not useful and can even confuse the creator if they want to update this code in the future.
作为程序的神/女神,我们可以使用抽象的概念来创建我们自己的对象。 当然,这带有一些责任。 维护逻辑命名约定和属性很重要。 否则,当您将“狗”类命名为“ being”并为其赋予属性“ value”时,与我们进行项目合作的其他人会非常困惑。 如果创建者将来想更新此代码,这将没有用,甚至可能使创建者感到困惑。
Let’s use a physical example. How about popsicles!
让我们使用一个物理示例。 冰棒怎么样!
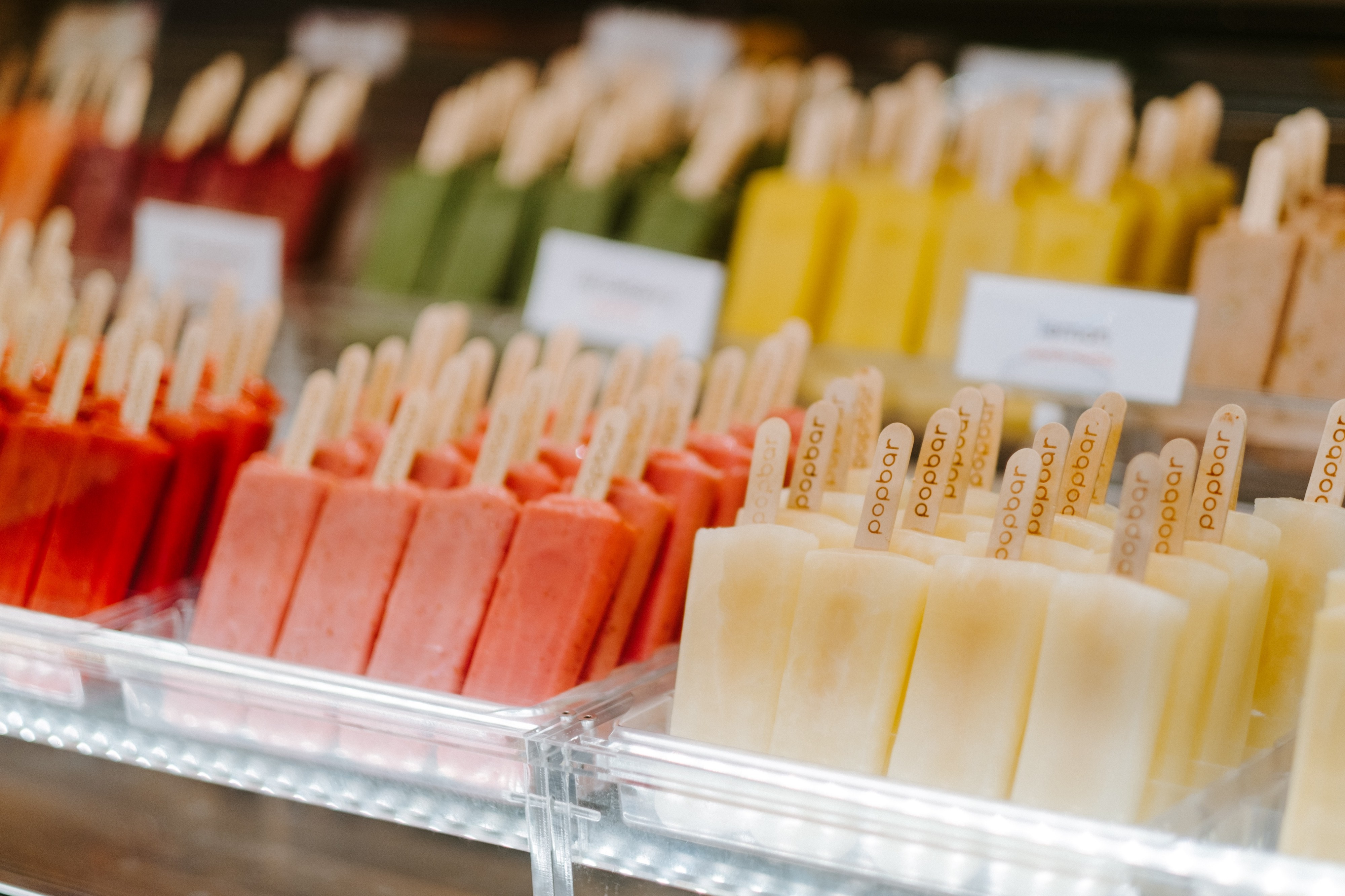
When you think of this tasty summer treat, bright colors and delicious flavors naturally come to mind. These are certainly the most important characteristics to assign when creating a ‘class’ of popsicles. It could be translated to code like this:
当您想到这种美味的夏季美食时,自然就会想到鲜艳的色彩和美味的风味。 这些无疑是创建“冰棍”类时要分配的最重要的特征。 可以将其翻译成如下代码:
class Popsicleattr_accessor :color, :flavordef initialize(color, flavor)
@color = color
@flavor = flavor
end#some more code with functionsend
In this example, each popsicle should have its own unique color and flavor. We can assign these ‘attributes’ to describe one specific popsicle or ‘instance’.
在此示例中,每个冰棍应该具有自己独特的颜色和风味。 我们可以分配这些“属性”来描述一个特定的冰棍或“实例”。
The initialize method is setting up what needs to happen each time we create a new popsicle instance and it is activated when we use the class method .new. Every time we create a new popsicle, we need to pass it an argument of flavor and color. (Because that’s what makes it a popsicle!)
初始化方法是设置每次我们创建新的冰棒实例时需要发生的事情,当我们使用类方法.new时,它将被激活。 每次创建新的冰棍时,我们都需要传递一个有关口味和颜色的参数。 (因为这就是使它成为冰棒的原因!)
If you’re wondering what @color = color does, it is stating that color is going to be a variable for each individual popsicle that we instantiate using the initialize method. That variable is going to equal the ‘color’ that we pass in as an argument when creating a new popsicle. It might look something like this:
如果您想知道@color = color是做什么的,那说明对于我们使用initialize方法实例化的每个单独的冰棒,color都将是一个变量。 该变量将等于我们在创建新冰棒时作为参数传递的“颜色”。 它可能看起来像这样:
lemon_popsicle = Popsicle.new(yellow, lemon)
This is an instance of a lemon popsicle. We can create as many as we want. Since all the different popsicles are living in the same class, we can create class methods to perform an action on all the popsicles by referring to the class as ‘self’ in a class method. (We won’t go into what ‘self’ means today, but it’s another great topic that I would like to cover in a future post.)
这是柠檬冰棒的一个实例。 我们可以创建任意数量的东西。 由于所有不同的冰棒都在同一个类中,因此我们可以创建类方法,以通过在类方法中将类称为“自身”来对所有冰棒执行操作。 (我们今天不会讨论“自我”的含义,但这是我想在以后的帖子中介绍的另一个重要话题。)
class Popsicleattr_accessor :color, :flavordef self.melt
puts "All the popsicles are melting!"
endend
OR we can create an instance method to function only on one specific popsicle.
或者,我们可以创建一个实例方法以仅在一个特定的冰棒上起作用。
class Popsicleattr_accessor :color, :flavordef melt
puts "This popsicle is melting!"
endend
You see, objects give us a lot of freedom. We can create an object, assign it attributes that mimic real-world characteristics, and execute the functions we need. This allows us to interact with our code in a more ‘realistic’ way that makes sense for our human needs. The sky is the limit.
你看,物体给了我们很多自由。 我们可以创建一个对象,为其分配模拟现实世界特征的属性,并执行所需的功能。 这使我们能够以更“现实”的方式与我们的代码进行交互,从而满足人类的需求。 天空才是极限。
Happy Coding!
编码愉快!
翻译自: https://medium.com/swlh/how-rubyists-use-abstraction-5ff417939940
ruby 访问者模式