pt,ct符号
Whenever we write code, it’s important to understand how that particular code impacts the speed and performance of our program. “Big O Notation” is a way to classify how quickly the runtime of the algorithm/number of operations or space will increase in relation to the input n
as it grows in size. It looks at the worst case scenario, assuming every possible element is touched upon as your algorithm is written.
每当我们编写代码时,重要的是要了解特定代码如何影响程序的速度和性能。 “ 大O表示法 ”是一种对算法的运行时间/操作数或空间数随输入n
大小增加而增加的速度进行分类的方法。 它考虑了最坏的情况,假设在编写算法时涉及到所有可能的元素。
There are a 7 different classifications in Big O notation, ranging from fantastic, fast and/or space efficient solutions that remain constant despite the input size, to absolutely terrible please-don’t-ever-use-these solutions!
Big O表示法有7种不同的分类,从奇妙的,快速的和/或节省空间的解决方案(尽管输入量很大)保持不变,到绝对糟糕的“ 请勿使用”这些解决方案!
Understanding how different data structures and algorithms fit into this Big O complexity gradient can help you choose which ones to use and how to optimize your code at work or in a tech coding interview.
了解不同的数据结构和算法如何适应这种大O复杂度梯度可以帮助您选择要使用的数据结构和算法,以及如何在工作中或在技术编码采访中优化代码。
O(1)恒定时间 (O(1) Constant Time)
Let’s say that you’re Chef Remy and you’ve been asked to cook your world famous Ratatouille.
假设您是雷米(Remy)厨师,并且被要求煮世界闻名的料理鼠王(Ratatouille)。
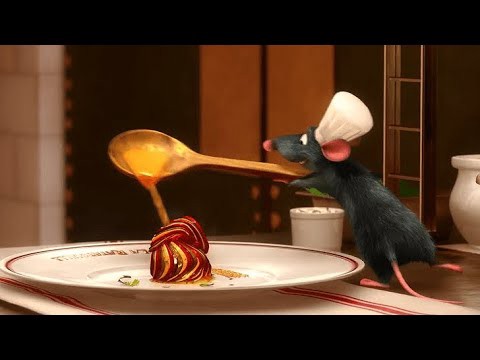
The first thing you’re going to do is gather the necessary ingredients, spices and herbs to prepare this dish. Since the kitchen is basically your second home, you know exactly where to find everything you need:
您要做的第一件事是收集必要的食材,香料和草药以准备这道菜。 由于厨房基本上是您的第二故乡,因此您确切地知道在哪里可以找到所需的一切:
let kitchen = {
vegetables: {
broccoli: {name: "broccoli", ...},
zucchini: {name: "zucchini", ...},
eggplant: {name: "eggplant", ...},
carrot: {name: "carrot", ...},
squash: {name: "squash", ...},
tomato: {name: "tomato", ...},
radish: {name: "radish", ...},
cabbage: {name: "cabbage", ...},
redPepper: {name: "redPepper", ...}
},
seasoning: {
onion: {name: "onion", ...},
garlic: {name: "garlic", ...},
basil: {name: "basil", ...},
oregano: {name: "oregano", ...},
herbesDeProvence: {name: "herbesDeProvence", ...},
sage: {name: "sage", ...},
ginger: {name: "ginger", ...}
}
}
You don’t need everything in the kitchen for this recipe (broccoli and ginger do NOT belong in ratatouille after all! 🙅♀️) so you can grab just the ingredients you need directly like so:
您无需在厨房中准备该食谱的所有东西(西兰花和生姜毕竟不属于料理鼠王!!️),因此您可以像这样直接获取所需的食材:
let ingredients = []ingredients.push(kitchen.vegetables.zucchini.name)
ingredients.push(kitchen.vegetables.eggplant.name)
ingredients.push(kitchen.vegetables.squash.name)
ingredients.push(kitchen.vegetables.tomato.name)
ingredients.push(kitchen.vegetables.redPepper.name)ingredients.push(kitchen.seasoning.onion.name)
ingredients.push(kitchen.seasoning.garlic.name)
ingredients.push(kitchen.seasoning.herbesDeProvence.name)// ingredients = ["zucchini", "eggplant", "squash", "tomato", "redPepper", "onion", "garlic", "herbesDeProvence"]
What would be the Big O notation time complexity of those operations? 🤔
这些操作的Big O表示法时间复杂度是多少? 🤔
Each of these operations (grabbing the ingredient, pushing it into the ingredients
array) will take constant time, or O(1). The number of operations doesn’t grow with the size of the array or the size of the kitchen object. You have direct access to each element to grab exactly what you need, so the time complexity won’t change.
这些操作(抓取成分,将其推入ingredients
数组)中的每一个都将花费固定时间 ,即O(1) 。 操作的数量不会随着数组的大小或厨房对象的大小而增加。 您可以直接访问每个元素以准确获取所需的内容,因此时间复杂度不会改变。
Here’s another example:
这是另一个例子:
function addDigitsUpToNumber(n) {
return n * (n + 1) / 2
}addDigitsUpToNumber(5) // 15, same as 1 + 2 + 3 + 5 = 15
In this function, we are adding up all of the digits from 1 to n
and returning that sum through a quick math trick. This function also has O(1) time complexity because it will take the exact same number of operations regardless of n
being equal to 5, 10, 1,000 or 1,000,000,000.
在此函数中,我们将1到n
所有数字相加,然后通过快速的数学技巧将其返回。 此函数还具有O(1)时间复杂度,因为无论n
等于5、10、1,000或1,000,000,000,它都将执行完全相同的操作数。
O(n)线性时间 (O(n) Linear Time)
To start preparing the recipe for ratatouille, you know that you need to chop all the ingredients first:
要开始准备料理鼠王的食谱,您需要首先将所有成分切碎:
let ingredients = ["zucchini", "eggplant", "squash", "tomato", "redPepper", "onion", "garlic", "herbesDeProvence"]function makeRatatouille(ingredients) {
function chop() {
for (let i = 0; i < ingredients.length; i++) {
console.log(`${ingredients[i]} has been chopped!`)
}
}
}
How long should our makeRatatouille
function take to complete, or better yet, how many operations need to take place? 🤔
我们的makeRatatouille
函数应该花多长时间才能完成,或者更好的是,需要进行多少次操作? 🤔
Since there are 7 elements in our ingredients
array, and the function iterates through each one once (chopping as we go), we could say that 7 operations need to take place (O(7)). If we added additional n
ingredients to our array, this function would take n
operations since it needs to touch upon each one of those elements once, otherwise known as O(n).
由于我们的ingredients
数组中有7个元素,并且该函数在每个元素中迭代一次(切入时切入),因此可以说需要进行7个操作(O(7))。 如果我们向数组中添加其他n
成分,则此函数将执行n
操作,因为它需要一次接触这些元素中的每个元素,否则称为O(n) 。
O(n) time complexity is also known as linear time and generally considered to be an efficient solution. It isn’t as good or as fast as O(1) or O(log n) which we’ll get to later, but usually this time complexity is considered acceptable.
O(n)时间复杂度也称为线性时间 ,通常被认为是一种有效的解决方案。 它不如我们稍后要提到的O(1)或O(log n)那样好或快,但通常认为此时间复杂度是可以接受的。
What would happen if we added another iteration? Those ratatouille ingredients need to roast in the oven after all!
如果再添加一次迭代会怎样? 那些料理鼠王的成分毕竟需要在烤箱中烤!
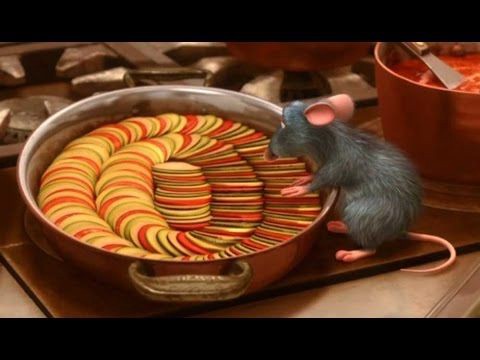
let ingredients = ["zucchini", "eggplant", "squash", "tomato", "red pepper", "onion", "garlic"]function makeRatatouille(ingredients) {
function chop() {
for (let i = 0; i < ingredients.length; i++) {
console.log(`${ingredients[i]} has been chopped!`)
}
} function roast() {
for (let i = 0; i < ingredients.length; i++) {
console.log(`${ingredients[i]} has been roasted!`)
}
}
}
Our makeRatatouille
function now needs to iterate through all of the ingredients twice: first during our chop
function, and then again during our roast
function. We could say this function takes O(2n) time, since it will iterate through the ingredients array exactly twice.
现在,我们的makeRatatouille
函数需要遍历所有成分两次:首先是在我们的chop
函数中,然后是在我们的roast
函数中。 我们可以说此函数需要O(2n)时间,因为它将对配料数组精确地进行两次迭代。
The time complexity of this function still grows directly in proportion to n
, so we would remove any constants (the number 2 in this case) and call this O(n), just like before. Even if this function had additional operations that came out to O(4n + 20) for example, we would still shorten this to say O(n), because in Big O notation, constants and smaller operations aren’t counted.
该函数的时间复杂度仍然直接与n
成正比,因此我们将删除所有常量(在这种情况下为2),并像以前一样将其称为O(n) 。 例如,即使此函数具有O(4n + 20)的其他运算,我们仍将其简称为O(n),因为使用大O表示法时, 不计算常数和较小的运算 。
O(n²)二次时间 (O(n²) Quadratic Time)
As Chef Remy, you hear that famed restaurant critic (and notorious grump) Anton Ego is coming to judge your food for the very first time. Yikes! 😰
作为厨师雷米(Remy),您会听到著名的餐厅评论家(并且是臭名昭著的脾气暴躁)安东·埃格(Anton Ego)第一次来评餐。 kes! 😰
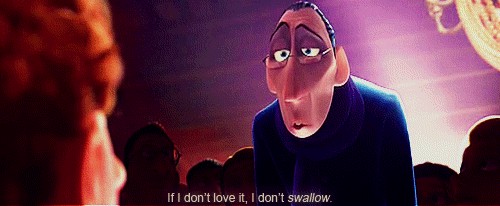
He will accept only the best, so you decide to sort through all of your chopped vegetables to pull aside the best ones for his ratatouille.
他只会接受最好的蔬菜,因此您决定对所有切碎的蔬菜进行分类,以将最好的蔬菜放在一边做料理。
let zucchiniSlices = [0, 1, 2, 3, 4, 5,...]function sortByBestSlices(veggieSlices) {
for (let i = 0; i < veggieSlices.length; i++) {
for (let j = 0; j < veggieSlices.length; j++) {
// some code to compare and sort slices by the best ones
}
}
return veggieSlices.slice(0, 15) // taking the 15 best pieces
}sortByBestSlices(zucchiniSlices)
The time complexity for this function is pretty bad. Here we’re looking at O(n²), also known as quadratic time because we have a nested for
loop situation. In this function we are iterating through each slice in our veggieSlices
array, comparing it to all other slices one by one, as n * n
. If the array had 30 slices, we would have 30 x 30 operations (900), and if we had 100 slices, we would have to do 10,000 operations to sort them this way.
此功能的时间复杂度很差。 在这里,我们查看O(n²) ,也称为二次时间,因为我们有一个嵌套的for
循环情况。 在此函数中,我们遍历veggieSlices
数组中的每个切片,并将其与所有其他切片逐个比较,即n * n
。 如果数组有30个切片,我们将有30 x 30的操作(900),如果我们有100个切片,我们将必须进行10,000个操作以这种方式对它们进行排序。
That sounds like the runtime could grow pretty fast, and Anton Ego is waiting for his meal! Isn’t there a more efficient way to sort, with less impact on the runtime?
听起来运行时可能会非常快,而Anton Ego正在等他吃饭! 是否有一种更有效的排序方式,并且对运行时的影响较小?
Of course there is! 👍
当然有! 👍
There are many algorithms that are much faster than O(n²), such as those that run in O(log n) and O(n log n) time. We’re going to explore those in part 2 of this series.
有许多算法比O(n²)快得多,例如那些以O(log n)和O(n log n)时间运行的算法。 我们将在本系列的第2部分中探讨这些内容。
In the meantime we’ll pour another glass of wine for Anton Ego and hope he stays patient. Stay tuned!
同时,我们将为安东·自我再倒一杯酒,希望他能耐心等待。 敬请关注!
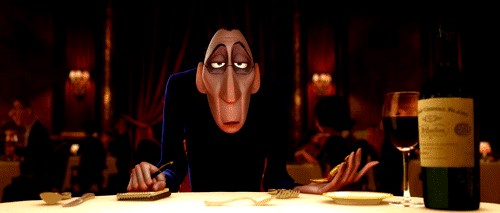
翻译自: https://medium.com/@alison.quaglia/a-beginners-guide-to-big-o-notation-pt-1-19ec031b698b
pt,ct符号