In continuation of my previous post ,we will keep on deep diving into basic fundamentals of PyTorch. In this post we will discuss about ways to transform data in PyTorch.
延续我以前的 发布后 ,我们将继续深入研究PyTorch的基本原理。 在这篇文章中,我们将讨论在PyTorch中转换数据的方法。
需要数据扩充 (Need for Data Augmentation)
Data augmentation is an approach that aids in increasing the variety of data for training models thus increasing the breadth of available information.The augmented data thus represents a more comprehensive set of possible data points. It improves the performance and ability of the model to generalize and hence addresses overfitting.
数据扩充是一种有助于增加训练模型数据种类的方法,从而增加了可用信息的广度。 它提高了模型的性能和泛化能力,从而解决了过拟合问题。
如何进行转换? (How to perform transformations?)
torchvision module of PyTorch provides transforms to accord common image transformations. These transformations can be chained together using Compose.
PyTorch的torchvision模块提供转换以符合常见的图像转换。 可以使用Compose将这些转换链接在一起。
transforms.Compose- Compose helps to bind multiple transforms together so we can use more than one transformation.
transforms.Compose -Compose有助于将多个转换绑定在一起,因此我们可以使用多个转换。

Multiple transformations have been chained using transforms.Compose and then it has been passed as an argument while loading dataset.
已使用transforms.Compose链接了多个转换,然后在加载数据集时将其作为参数传递。
transforms.ToTensor — Applies a scaling operation of changing range from 0–255 to 0–1. It converts a PIL Image or numpy ndarray to a tensor (C x H x W) in the range of 0–1.
transforms.ToTensor —应用缩放操作,将范围从0–255更改为0–1。 它将PIL图像或numpy ndarray转换为范围为0-1的张量(C xH x W)。
transforms.Normalize- This operation normalizes a tensor image with provided mean and standard deviation. For an image with 3 channels (RGB), 3 values for mean and 3 values for standard deviation are given as parameters(in form of tuple) corresponding to each channel.
transforms.Normalize-此操作使用提供的均值和标准差对张量图像进行归一化。 对于具有3个通道(RGB)的图像,将给出3个平均值和3个标准差值作为与每个通道相对应的参数(以元组的形式)。
Example :- transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5)) where first tuple is for mean and second for standard deviation.
示例: -transforms.Normalize((0.5,0.5,0.5),(0.5,0.5,0.5))其中,第一个元组表示平均值,第二个元组表示标准偏差。
transforms.Pad — It pads given image on all sides with the given padding value. If a single integer value is provided for padding parameter then that value is used to pad all borders. If tuple of length 2 is provided then this acts as padding on left/right and top/bottom respectively. For constant padding mode default value for fill 0 is used.
transforms.Pad —它使用给定的填充值在所有面上填充给定的图像。 如果为填充参数提供单个整数值,则该值将用于填充所有边界。 如果提供长度为2的元组,则这分别充当左侧/右侧和顶部/底部的填充。 对于恒定填充模式 ,使用填充 0的默认值。
Example :- torchvision.transforms.Pad(10)
示例: -torchvision.transforms.Pad(10)
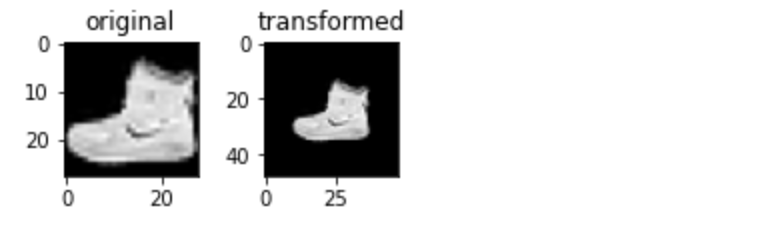
You can notice the output size of the transformed image.
您会注意到转换后的图像的输出大小。
Using image from FMNIST dataset, used in my previous post. Image size for this dataset is 28*28 .
使用 我以前的 文章中 使用的 FMNIST数据集中的 图像 。 该数据集的图像大小为28 * 28。
transforms.RandomHorizontalFlip — Flipping operation helps in changing the orientation of the image. RandomHorizontalFlip changes the orientation horizontally similarly we can use RandomVerticalFlip for changing vertical orientation. It flips the image randomly with a given probability (p).
transforms.RandomHorizontalFlip —翻转操作有助于更改图像的方向。 类似地,我们可以使用RandomVerticalFlip来改变垂直方向。 它以给定的概率(p)随机翻转图像。
Example :- torchvision.transforms.RandomHorizontalFlip(p=1)
示例: -torchvision.transforms.RandomHorizontalFlip(p = 1)
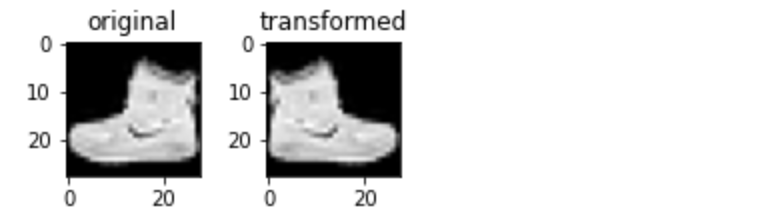
transforms.CenterCrop- We can do cropping of an image using this transformation. CenterCrop crops the given image at the center as per the size parameter. Crop can be square or rectangle in shape depending on the size parameter dimensions. Similarly, we have RandomCrop that crops the given image at a random location.
transforms.CenterCrop-我们可以使用此变换来裁剪图像。 CenterCrop根据大小参数在中心裁剪给定图像。 作物的形状可以是正方形或矩形,具体取决于大小参数的尺寸。 同样,我们有RandomCrop可以在任意位置裁剪给定图像。
Example :- torchvision.transforms.CenterCrop((18, 18))
示例: -torchvision.transforms.CenterCrop((18,18))
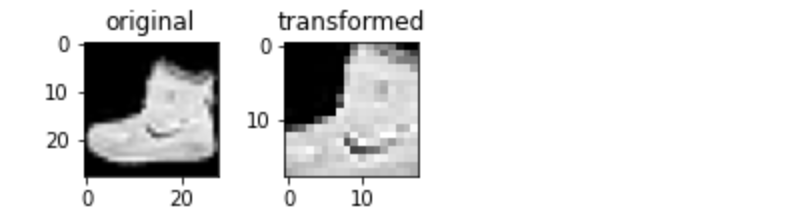
transforms.Resize —To resize image this transformation can be used. It is also very useful incase of images with large dimensions to reduce it to a particular size (parameter for desired output size) . By resizing, the resolution can be lowered and thus will help in training the network faster.
transforms.Resize —要调整图像的大小,可以使用此变换。 在具有大尺寸的图像的情况下将其减小到特定尺寸 (用于期望的输出尺寸的参数)也是非常有用的。 通过调整大小,可以降低分辨率,从而有助于更快地训练网络。
Example :- torchvision.transforms.Resize((300, 300))
范例: -torchvision.transforms.Resize((300,300))
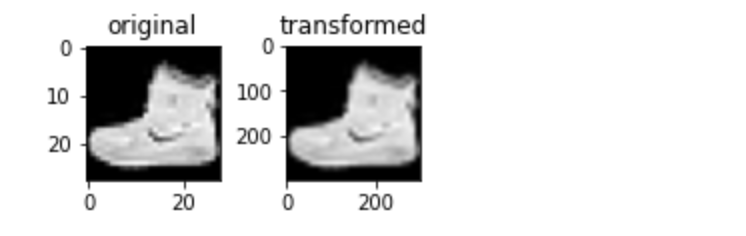
transforms.RandomRotation- To rotate an image by certain degrees (parameter). If degrees is an integer rather than (min, max) then the range is interpreted as (-degrees, +degrees).
transforms.RandomRotation-将图像旋转一定程度 (参数)。 如果度数是整数而不是(min,max),则该范围将解释为(-度数+ +度数)。
Example :- torchvision.transforms.RandomRotation(degrees=(180))
范例: -torchvision.transforms.RandomRotation(degrees =(180))
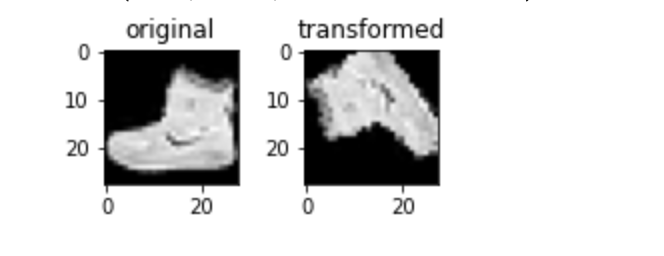
transforms.ColorJitter- It helps to change the brightness, contrast and saturation of an image.
transforms.ColorJitter-它有助于 更改图像的亮度,对比度和饱和度。
Apart from these above mentioned transformations, you can refer to full list here.
除了上述转换之外,您还可以在此处参考完整列表。
最后的想法 (Final Thoughts)
It is not always necessary to use multiple augmentations all at once, it is more of data dependant process.We need to be careful while using these transformations . For example, with crop operation if the crops are too small, we might be at risk of cutting out important parts of the image and making the model train on the wrong thing. For instance, if a dog is playing near a tree and the crop takes out the dog and just leaves part of the tree to be classified as dog that may create issues.
不一定总是一次使用多个扩充,更多的是依赖于数据的过程。在使用这些转换时,我们需要小心。 例如,如果作物太小,则使用作物操作时,我们可能会面临切掉图像重要部分并使模型针对错误的事物进行训练的风险。 例如,如果一只狗在树附近玩耍,而庄稼将狗拔出,只留下部分树被分类为狗,这可能会造成问题。
Apart from torchvision.transforms we can explore albumentations library too for deep learning image augmentation.
除了torchvision.transforms我们可以探索albumentations库过深学习图像增强。
翻译自: https://medium.com/analytics-vidhya/transforming-data-in-pytorch-741fab9e008c