巴比伦js
灵感来源 (The Inspiration)
The idea seems intuitive. We all have seen the lights leaving beautiful trajectories, and we want to record the transient moment so we can keep it. Picasso famously made some light paintings, which were documented in 1949.
这个想法似乎很直观。 我们所有人都已经看到了灯光留下的优美轨迹,并且我们想要记录瞬态力矩以便保持它。 毕加索著名的一些光绘作品是在1949年记录的。
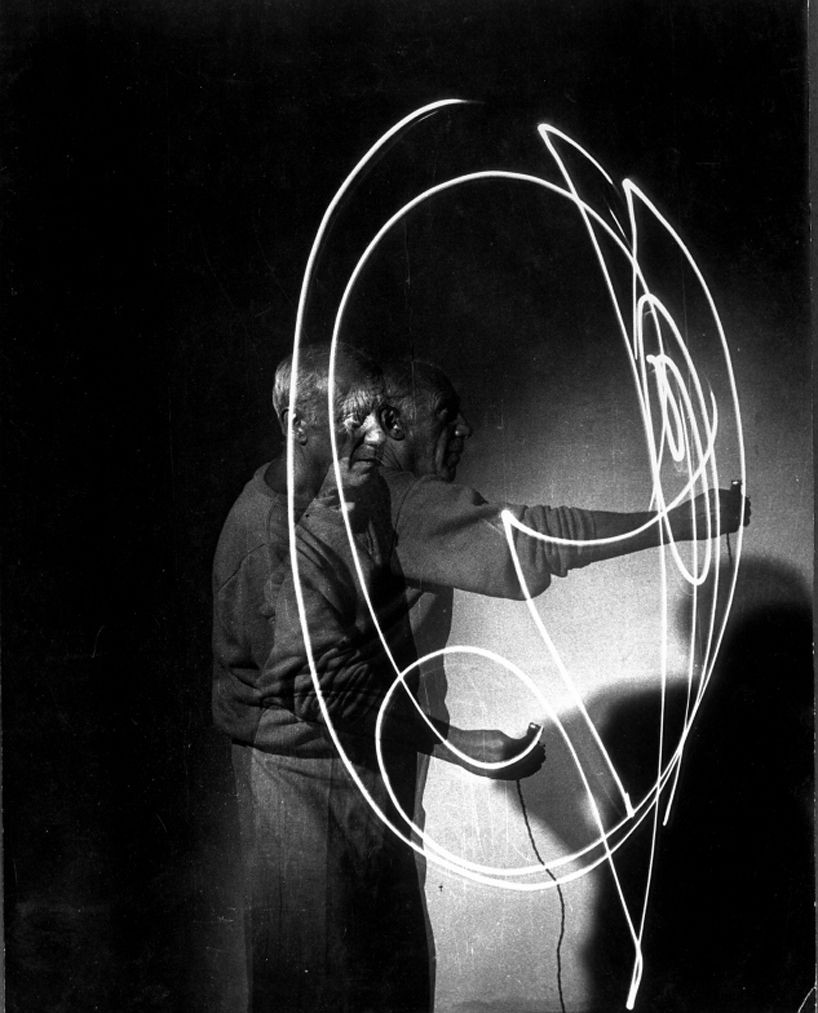
The idea of modeling with a moving point is exactly how a 3D printer functions.
用移动点建模的想法正是3D打印机的功能。
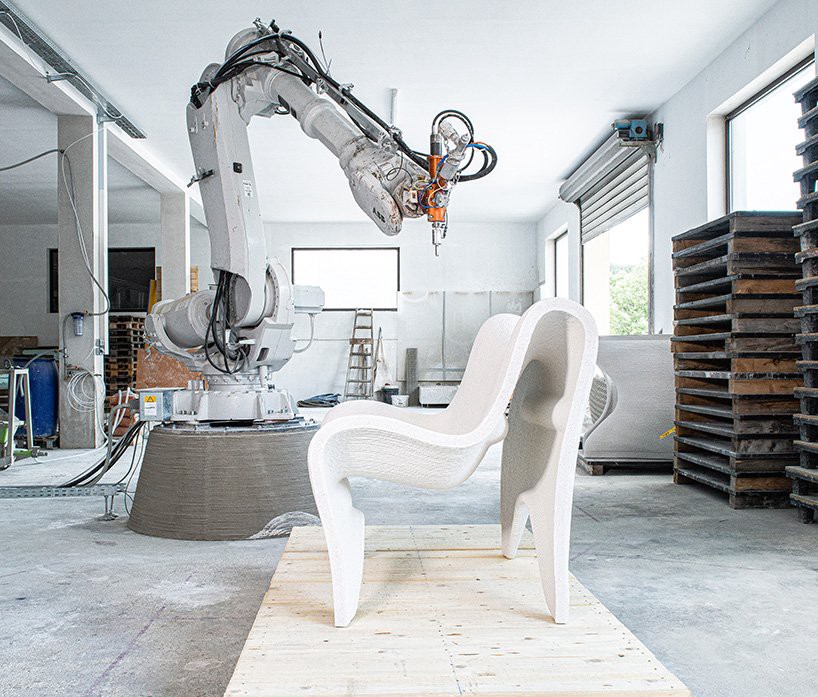