In this tutorial, I will be building a live BTC exchange rate ticker by fetching the currency exchange rate data from Cryptonator.com, which provides free cryptocurrencies exchange rate APIs.
在本教程中,我将通过从Cryptonator.com获取货币汇率数据来构建实时的BTC汇率报价器,该数据提供了免费的加密货币汇率API。
Although they provide currency exchange rates for various cryptocurrencies, but here I will be dealing only with Bitcoin.
尽管它们提供了各种加密货币的货币汇率,但是在这里我将只处理比特币。
You can see the ticker in action here.
您可以在 此处 查看股票行情 。
入门… (Getting started…)
设置节点服务器 (Setup node server)
Create a folder named btcticker
. Go to the folder path in the terminal or command prompt and give the command npm init
, just respond to the prompts and it will create package.json
file inside the folder.
创建一个名为btcticker
的文件夹。 转到终端或命令提示符中的文件夹路径,并给命令npm init
,只需响应提示符,它将在文件夹内创建package.json
文件。
Open package.json file in the IDE of your choice and update the values of the below keys:main: “server.js”start: “node server”
在您选择的IDE中打开package.json文件,并更新以下键的值: main: “server.js”start: “node server”
After this install the required node modules by giving the commandnpm i express request socket.io
之后,通过给出命令npm i express request socket.io
安装所需的节点模块。
Now create two files server.js
and fetchbtc.js
. We will also create one folder named public
, which will hold all client-side code by which we will display our currency ticker on the client’s browser.
现在创建两个文件server.js
和fetchbtc.js
。 我们还将创建一个名为public
文件夹,该文件夹将保存所有客户端代码,通过它们我们将在货币代码显示在客户端的浏览器中。
项目结构 (Project Structure)

Now let’s discuss the server-side code written in the above files.
现在让我们讨论以上文件中编写的服务器端代码。
server.js (server.js)

In the code above we are creating a very basic node server by importing required node modules along with express and socket.io.
在上面的代码中,我们通过导入所需的节点模块以及express和socket.io创建了一个非常基础的节点服务器。
On line number 20 we are emitting a socket.io event ‘subscribed-btc-prices’
at the interval of every 5 seconds, which will be subscribed by the client.
在第20行,我们每隔5秒发送一次socket.io事件'subscribed-btc-prices'
,该事件将由客户端进行订阅。
fetchbtc.js (fetchbtc.js)

The fetchAPI()
method in the above code returns a promise, which is returned by the request
node module after fetching the provided API path. The promise either returns a resolve object with the API response body or rejects it with an error message.
上面代码中的fetchAPI()
方法返回一个fetchAPI()
该fetchAPI()
在获取提供的API路径后由request
节点模块返回。 许诺要么返回带有API响应主体的resolve对象,要么通过错误消息拒绝它。
The pushUpdates()
method chains the fetchAPI()
method which calls four different APIs. The response of the API is pushed into btcArr[]
array and returned as a promise.
pushUpdates()
方法链接fetchAPI()
方法,该方法调用四个不同的API。 API的响应被推入btcArr[]
数组并作为btcArr[]
返回。
We get the following response returned by the server at the interval of every 5 seconds.
我们每隔5秒就会收到服务器返回的以下响应。

客户端代码 (Client-side code)
Once the server-side code is done, let’s explore the client-side code.
服务器端代码完成后,让我们探索客户端代码。
index.html
index.html

In the above code I have included two javascript libraries socket.io.js
and sparkline.js
along with the app.js
. We will display the currency data and ticker inside the table with the id, btcticker
and the currency Ticker Tape inside the div with the id, tickertape
.
在上面的代码中,我包括了两个JavaScript库socket.io.js
和sparkline.js
以及app.js
我们将与ID,显示表内的货币数据和股票btcticker
和货币纸带的DIV中与ID, tickertape
。
app.js
app.js
We will begin by creating the instance of socket.ioconst socket = io();
And then we will subscribe to the below socket event emitted by the server.
我们将从创建socket.io实例开始。const const socket = io();
然后,我们将订阅服务器发出的以下套接字事件。
socket.on('subscribed-btc-prices', tickerdata => {
refreshTicker(tickerdata);
});

The refreshTicker()
function is called after every 5 seconds with the argument, tickerdata
.Here we loop over the tickerdata
array and then find the table row whose first column contains ‘data-currency’
attribute. If the column’s data attribute matches with the item.target
value, we simply remove that row.Once the row is removed we add the new row with the latest data received by the server.
每隔5秒就会使用参数tickerdata
调用refreshTicker()
函数。在这里,我们遍历tickerdata
数组,然后查找表行,其第一列包含'data-currency'
属性。 如果该列的data属性与item.target
值匹配,则只需删除该行。删除该行后,我们将添加服务器接收到的最新数据的新行。
The above logic can also handle the condition, when the server returns the currency data in random order.
当服务器以随机顺序返回货币数据时,上述逻辑还可以处理这种情况。

The printCurrency()
function uses a simple switch statements to display the currency symbol.
printCurrency()
函数使用简单的switch语句来显示货币符号。
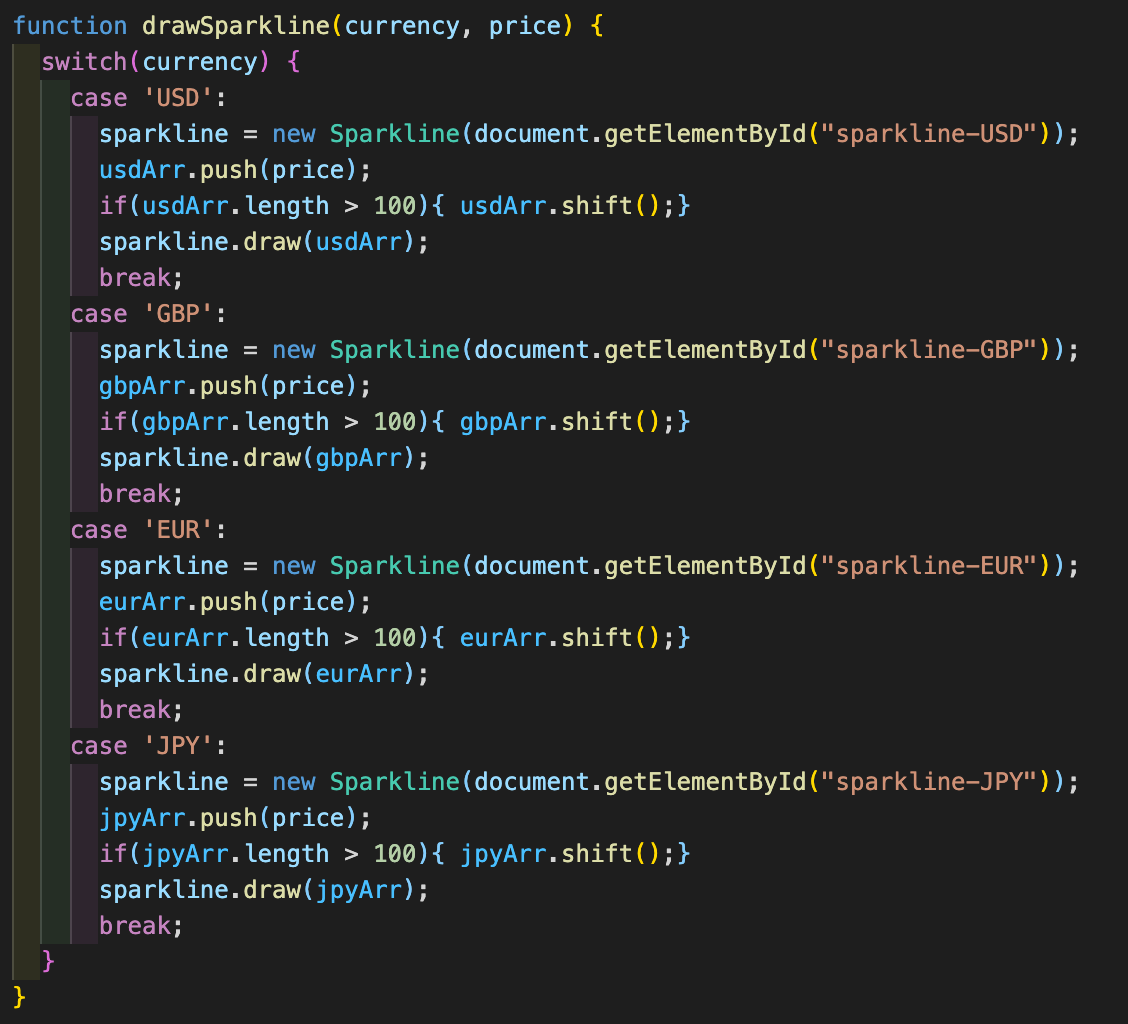
The drawSparkline()
function accepts currency and price as two arguments and uses switch statements to draw a sparkline graph for the passed currency by pushing the latest price into the currency specific array.
drawSparkline()
函数接受货币和价格作为两个参数,并使用switch语句通过将最新价格推入特定于货币的数组中来为传递的货币绘制drawSparkline()
图。

The tapeTicker()
function creates the currency Tape Ticker based on the passed tickerdata
.This function doesn’t gets called until the currency array contains two or more items.
tapeTicker()
函数根据传递的tickerdata
数据创建货币Tape Ticker。直到货币数组包含两个或多个项目,该函数才会被调用。

The getDirection()
function accepts currency as argument and uses switch statements to show the up (↑), down (↓) or no change (↔) arrows.
getDirection()
函数接受货币作为参数,并使用switch语句显示向上(↑),向下(↓)或无变化(↔)箭头。

The formatDate()
function formats the date using javascript inbuilt Intl.DateTimeFormat()
method.
formatDate()
函数使用javascript内置的Intl.DateTimeFormat()
方法来格式化日期。
是时候查看我们的代码了 (Time to see our code in action)
Give the command npm start
in the terminal, this will spin up the node server. Then access http://localhost:1978 in your favorite browser.You will see something like the image below in your browser’s screen.
在终端中输入命令npm start
,这将启动节点服务器。 然后在您喜欢的浏览器中访问http:// localhost:1978 。您将在浏览器的屏幕中看到如下图所示的内容。

You will notice that the above ticker keeps getting updated after every 5 seconds.
您会注意到,上述行情自动收录器每5秒更新一次。
结论 (Conclusion)
In the above tutorial, I have explained one of the possible ways to create a simple currency exchange rate ticker. The same logic can be used to build a stock ticker where users can search and subscribe to the multiple stocks of their choice.
在上面的教程中,我解释了创建简单货币汇率行情的一种可能方法。 可以使用相同的逻辑来构建股票报价器,用户可以在其中搜索和订阅他们选择的多种股票。
You can find the complete source code of the above project, hosted on my Github profile here.
你可以找到以上项目的完整源代码,托管在Github上我的个人资料在这里 。
I hope you might have enjoyed reading my article, do let me know your thoughts.
我希望您可能喜欢阅读我的文章,并让我知道您的想法。
翻译自: https://medium.com/@Akhil_Srivastv/building-a-currency-ticker-with-nodejs-and-socket-io-3718280f1803