JavaScript is an elegant and eloquent in-demand programming language, yet it has some parts which are initially somewhat difficult to grasp and may cause a feeling of awkwardness. One such weird part is the difference between three variable types: var, let, const. In this article, we’ll try to understand why these differences exist in the first place and how to make the most use of them, by describing the situations where they can be most appropriate.
JavaScript是一种优雅而雄辩的按需编程语言,但是它具有一些最初很难理解的部分,可能会导致笨拙的感觉。 这样一个奇怪的部分是三种变量类型之间的区别: var,let,const。 在本文中,我们将通过描述最合适的情况来尝试理解为什么首先存在这些差异以及如何最大程度地利用它们。
First of all, let’s understand what a variable represents. Imagine you go to a bank and put into safe whatever you want, money, valuables, gold, etc. This is exactly what variables do, you can store in them any information you want (except actual money or gold), like arrays, objects, functions, number, boolean, string, undefined. Later, you can access all these items by invoking variables by their names that they got when were first declared. Consider the following:
首先,让我们了解一个变量代表什么。 想象一下,您去了一家银行,将想要的任何东西,金钱,贵重物品,黄金等放入保险箱。这正是变量的作用,您可以在其中存储任何想要的信息(实际货币或黄金除外),例如数组,对象,函数,数字,布尔值,字符串,未定义 。 以后,您可以通过使用变量在首次声明时获得的名称来调用变量,从而访问所有这些项目。 考虑以下:
var name; // undefined (variable declaration, undefined upon it)
var name; //未定义 (变量声明, 未定义 )
name = “John” // “John” (assignment of an existing variable)
name =“ John” //“ John” (现有变量的分配)
or we can combine these two lines into one (why bother?)
或者我们可以将这两行合并为一(为什么要打扰?)
var name = “John” // “John” (variable declaration and assignment)
var name =“ John” //“ John” (变量声明和赋值)
Several rules to note here are that the variables cannot be named by certain words, which are known as reserved words in JavaScript, they also cannot start with a number (var 1name), cannot contain spaces (var name and last name). They should begin with lower case letters, dollar sign ($) or underscore (_), and be camel-cased in case of concatenating more than one word (var firstNameAndLastName) for better readability.
这里要注意的几个规则是,变量不能用某些单词命名,这些单词在JavaScript中称为保留单词 ,它们也不能以数字开头( var 1name ),不能包含空格( var name和last name )。 它们应以小写字母,美元符号($)或下划线(_)开头,并且在连接多个单词( var firstNameAndLastName )的情况下使用驼峰式大小写,以提高可读性。
var, let & const
var,let&const
Imagine that we intend to declare and assign multiple variables in a single breath. JavaScript can alleviate this process by eliminating unnecessary repetitions. Instead of
想象一下,我们打算一次声明并分配多个变量。 JavaScript可以通过消除不必要的重复来减轻此过程。 代替
var a = 1
var a = 1
var b = 2
var b = 2
var c = 3
var c = 3
we can write
我们可以写
var a = 1, b = 2, c = 3
var a = 1,b = 2,c = 3
thus avoiding repetition of var word. var-s values can be changed, for instance, if we assigned a variable to the “Michael” string, it can afterward be changed into another value, like “Jackson”:
从而避免重复var字。 可以更改var -s的值,例如,如果我们将变量分配给“ Michael”字符串,则此后可以将其更改为另一个值,例如“ Jackson”:
var person = “Michael”;
var person =“ Michael”;
person = “Jackson”;
人=“杰克逊”;
let person = “Michael”;
让个人=“迈克尔”;
person = “Jackson”;
人=“杰克逊”;
BUT
但
const person = “Michael”;
const person =“迈克尔”;
person = “Jackson”; // Uncaught TypeError: Assignment to constant variable.
人= “杰克逊”; //未捕获的TypeError:分配给常数变量。
So both var and let can be declared+assigned first and then re-assigned, unlike const, as the name suggests, it is constant, and can’t be re-assigned. But here the differences don’t end!
因此,与const不同,顾名思义, var和let都可以先声明+ assigned,然后再重新赋值,就像const一样,它是const , 不能重新赋值 。 但是在这里差异不会结束!
var has been there for many years before implementing other variables. You can easily encounter it in any pre-ES2015 code. Also, var-s have scope issues, that’s why in the ES2016 era it’s advisable to use let and const instead. To go more detailed into this issue, we must first declare, that JavaScript has two types of scopes, function-scope, and block-scope.
var在实现其他变量之前已经存在了很多年。 您可以在任何ES2015之前的代码中轻松遇到它。 另外, var -s存在范围问题,这就是为什么在ES2016时代,建议改用let和const的原因 。 为了更详细地讨论这个问题,我们必须首先声明JavaScript具有两种类型的作用域: function-scope和block-scope 。
Function-scope:
功能范围:
Here we can see that var name is only visible in the function-scope when it is console logged within the function, JavaScript recognizes var name and prints out its value (string “Michael”). However, when we try to call it outside of the function (the second time when it’s console logged), it throws an error, complaining about the name not being defined with ReferenceError. The situation can get more complicated when exemplifying the block-scope
在这里,我们可以看到,只有在控制台中将var name记录在函数中时,该变量名才在功能范围内可见,JavaScript识别var name并输出其值(字符串“ Michael”)。 但是,当我们尝试在函数外部调用它时(第二次登录控制台时),它将引发错误,并抱怨该名称未使用ReferenceError定义。 以块状镜为例,情况可能变得更加复杂
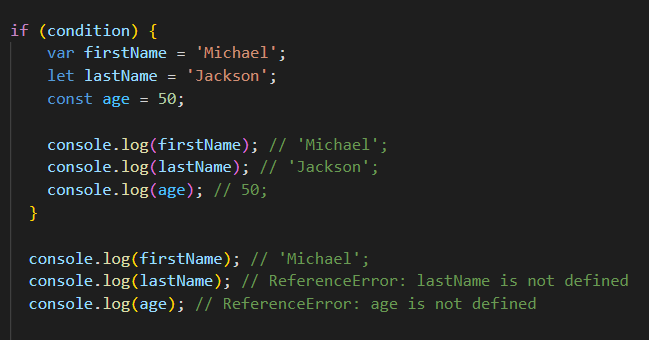
Within the block scope (the space between curly braces, {…}), all our variables, whether they are var, let, or const, are easily invoked. Things get complicated when trying to invoke them outside of the scope. Ideally, they shouldn’t be visible outside of the scope, while they should only live within the scope! This rule is correct for let and const, as they throw a ReferenceError when tried to be console logged outside of the scope, but var firstName can be easily accessed even outside of the scope. And this is a big issue, while we want to keep variables private for the scope they live in, but var breaks this rule, which is actually a bug. Therefore, let and const variables were created to the rescue and elimination of this issue, they are more specific and tell us, in case of ReferenceError, that they cannot be addressed outside of the scope if they live within it!
在块范围内(大括号{…}之间的空格),我们所有的变量,无论它们是var,let还是const ,都可以轻松调用。 当试图在范围之外调用它们时,事情变得复杂。 理想情况下,它们不应在范围外可见,而应仅存在于范围内! 此规则对le t和const是正确的,因为它们试图在范围外进行控制台记录时会抛出ReferenceError ,但是即使在范围外也可以轻松访问var firstName 。 这是一个大问题,尽管我们想让变量在它们所处的作用域内保持私有,但是var打破了该规则,这实际上是一个错误。 因此,创建了let和const变量来挽救和消除此问题,它们更加具体,并告诉我们(在ReferenceError的情况下)如果存在于范围内,则无法在范围之外对其进行寻址!
çonclusion (Conclusion)
All right, so we finally understand the difference between these three types of variables. What is the practical usage? Which variables to be used and where?
好的,所以我们最终了解了这三种类型的变量之间的区别。 实际用途是什么? 使用哪些变量,在哪里?
As we underlined, var is an old version with a block-scope bug coming with it, so it is good to know about it but it’s the best practice never to use it! Ok, what about let and const? As was indicated above, const should be used as a constant, so it is advisable to benefit from it pretty much most of the time, but when the value of a variable is intended to be altered, for instance in loops, in counters, it is required to use let so that it changes its value after each iteration.
正如我们所强调的那样, var是一个旧版本,它附带了一个带有范围监视功能的bug,因此很高兴知道它,但最好不要使用它! 好吧, let和const呢? 如上所述, const应该用作常量,因此建议大部分时间都可以从中受益,但是当要更改变量的值时(例如,在循环中,在计数器中),建议使用const。需要使用let,以便在每次迭代后更改其值。