Let try to understand different types of variable available in JavaScript. We will start from the old school variable declaration to the latest ES6 (ES 2015) variable which is new and has more flexibility over `var`.
让我们尝试了解JavaScript中可用的不同类型的变量。 我们将从旧式变量声明开始,到最新的ES6(ES 2015)变量,它是新变量,并且比`var`具有更大的灵活性。
So this is all level but I will try from a basic so if any new to the world of programming or JavaScript can take lots from here.
所以这是所有级别的内容,但是我将从一个基本的角度尝试,以便使编程或JavaScript领域的任何新手都可以从这里受益。
那么什么是变量? (So what is variable?)
So if you google it what is variable you will find a lot of definition on the variable.
因此,如果您用谷歌搜索什么是变量,您会在变量上找到很多定义。
Let try to understand in layman language. In the field of programming, Variables are the names you give to computer memory locations which are used to store values in a computer program. It is similar to our University library where the books are store in different sections based on their categories. In Programming, we used to store data in computer memory and these memories are also categories with different uses. So some memory is available for storing & running program. we will not go it deep just stick on basic definitions.
让我们尝试用外行语言理解。 在编程领域,变量是您赋予计算机内存位置的名称,用于在计算机程序中存储值。 它类似于我们的大学图书馆,在该图书馆中,根据类别将书籍存储在不同的部分。 在编程中,我们曾经将数据存储在计算机内存中,这些内存也是具有不同用途的类别。 因此,有些内存可用于存储和运行程序。 我们不会深入探讨,只是坚持基本定义。
So different programming language has a different way of defining variable & work upon them. In JavaScript, we have 3 types of variables used to store the expression & use them.
因此,不同的编程语言具有不同的定义变量和对其进行处理的方式。 在JavaScript中,我们有3种类型的变量用于存储表达式并使用它们。
JS in Dynamically Typed Programming Language (A Variable Doesn’t Enforce the type). The variable in JS does not have any attached.
动态类型编程语言中的JS(变量不会强制类型)。 JS中的变量没有任何附加。
In C language if we have to define variable we have stated their type.
在C语言中,如果必须定义变量,则说明了它们的类型。
int a, b; // Here int used to state a variable is of type interger.
float f; // Here float used to state a variable if of type float or decimal
JS is both dynamically typed and weakly typed.
JS既是动态类型的,也是弱类型的。
在JavaScript中定义变量 (Defining a Variable in JavaScript)
As above I said we don’t have to state type in JS. So we can define a variable using any of the following keywords const
, let
, & var.
如上所述,我们不必在JS中声明类型。 因此,我们可以使用以下任意关键字const
, let
和var.
定义变量var.
const PI = 3.14;
let user = "itz me"
var isActive = true;
In the above, we declared a variable using all available options and I don’t have to say what type of data is that. So we can declare a variable using any of these but there is a difference in them and you will find what to use and when to use after this.
在上面,我们使用所有可用选项声明了一个变量,而我不必说那是什么类型的数据。 因此,我们可以使用这些变量中的任何一个来声明变量,但是它们之间存在差异,之后您将发现使用什么以及何时使用。
var- (var -)
Before the release of ES6 specification, we had only one way to initialize a variable in JavaScript using a var
keyword. To define a variable using var we had to use keyword var
and after that variable name.
在ES6规范发布之前,我们只有一种方法可以使用var
关键字在JavaScript中初始化变量。 要使用var定义变量,我们必须在该变量名之后使用关键字var
。
var user; // Initialize a variable using var and name it user
Here we initialize and variable and didn’t give any value by default all variable without value has a default value of undefined.
在这里,我们初始化和变量,并且默认情况下不提供任何值。 所有没有值的变量的默认值均为undefined。

variable defined using var
is functional scope means that if we defined a variable inside a function it will available to that function.
使用var
定义的变量是函数作用域,这意味着如果我们在函数内部定义了变量,则该变量对该函数可用。
Variable created using var
outside a function are global scoped (A scope in any programming is a region of the program where a defined variable can have its existence and beyond that variable, it cannot be accessed). Mean that globally created variable using can be used and update anywhere in the program.
使用var
在函数外部创建的变量是全局范围的(任何编程中的范围是程序的一个区域,在该区域中,已定义的变量可以存在并且超出该变量,则无法访问该变量)。 意味着可以使用全局创建的变量using并在程序中的任何位置更新。
var
in function
var
函数
var user = "global";
function scope() {
var user = "function scope"
console.log(user); // "function scope"
}
scope();
console.log(user); // "global"
Here we defined a variable named user
in the global scope with the value of global and another variable with the same name in the function scope if we see console message when we call scope()
function in there a new scope created which is also known as the functional scope and there we assign a value “function scope” in when it reaches to line 5 it prints function scope
on a REPL or console and get out of functional context and run then line 9 here we can see that the value of a user is still global
in the global context. So variable created using var
is functional scope.
在这里,我们在全局作用域中定义了一个名为user
的变量,其值为global,在函数作用域中定义了另一个具有相同名称的变量,如果我们在创建新作用域的范围内调用scope()
函数时看到控制台消息, 功能范围,然后我们在第5行分配一个值“功能范围”,在REPL或控制台上打印function scope
,退出功能上下文并运行第9行,在这里我们可以看到用户的值在全球范围内仍然是global
性的。 因此,使用var
创建的变量是功能范围。
Another difference is that using var
if you created a variable with the same name in the global or function context it will get overwritten by the last value of that variable
另一个区别是,如果使用var
如果您在全局或函数上下文中创建了一个具有相同名称的变量,它将被该变量的最后一个值覆盖

we can see it get updated with the new value. Nowadays people try to avoid using var and use ES6 latest way for defining a variable with more flexibility and other features.
我们可以看到它已使用新值更新。 如今,人们尝试避免使用var并使用ES6最新方法来定义具有更多灵活性和其他功能的变量。
As above we see a kind of disadvantage of using var
suppose you are collaborating with other developers and both of you defined using same name then it will break your program. So avoid using var.
如上,我们看到了使用var
的一种缺点,假设您正在与其他开发人员合作,并且你们两个都使用相同的名称进行定义,那么它将破坏您的程序。 因此,请避免使用var.
让- (let -)
let
& const
is a new addition on ES6 to defining variable which has a more secure way of defining a variable.
let
& const
是ES6上对变量的新添加,它具有更安全的变量定义方式。
To define a variable using let
we use let
keyword
使用let
定义变量,我们使用let
关键字
let user = "itz me";
let
and const
both are block-scoped. we saw var
is functional scoped and variable defined using var
inside a function is in function context but let
& const
are block-scoped (a block in JavaScript is anything in between { }).
let
和 const
都是块作用域的 。 我们看到var
在函数范围内,并且在函数内部使用var
定义的变量在函数上下文中,但let
& const
被块作用域化(JavaScript中的块位于{}之间) 。
Let see with Example
让我们看一下例子
let user = "global";
if (true) {
let user = "inside if";
console.log(user); // "inside it"
}
function letScope() {
let user = "inside function";
console.log(user) // "inside function"
}
letScope()
console.log(user) // "global"
Above we created a variable using let
in a global context if block and function block. As this program run it comes to first to if block and run it and here we defined a user using let with a value inside if
that gets printed on console then it reached to line 8 and here function gets called and moved to lined 8 here we redefined a variable using let with a value inside function
that gets printed on the console you can see as the function is also block-scope because the block is anything is inside curly braces {}
. In last our JS run line 15 and print global
to console. As see our globally defined variable using let
not get updated by if block and function block.
上面我们在if块和function块的全局上下文中使用let
创建了一个变量。 在运行该程序时,首先要进行if阻止并运行它,在这里我们使用一个let定义了一个用户, inside if
该值在控制台上打印出来,则该值inside if
第8行,在这里函数被调用并移至第8行,使用let使用inside function
值重新定义了一个变量,该变量被打印在控制台上,您可以看到,因为该函数也是块作用域,因为该块是在花括号{}
。 最后,我们的JS运行第15行,并global
打印到控制台。 如所见,我们的全局定义变量使用let
不能被if块和功能块更新。
Similarly const
is also block-scoped
.
同样, const
也是block-scoped
。
Let see what happens if we redefined a variable in a global context or block context.
让我们看看如果在全局上下文或块上下文中重新定义变量会发生什么。
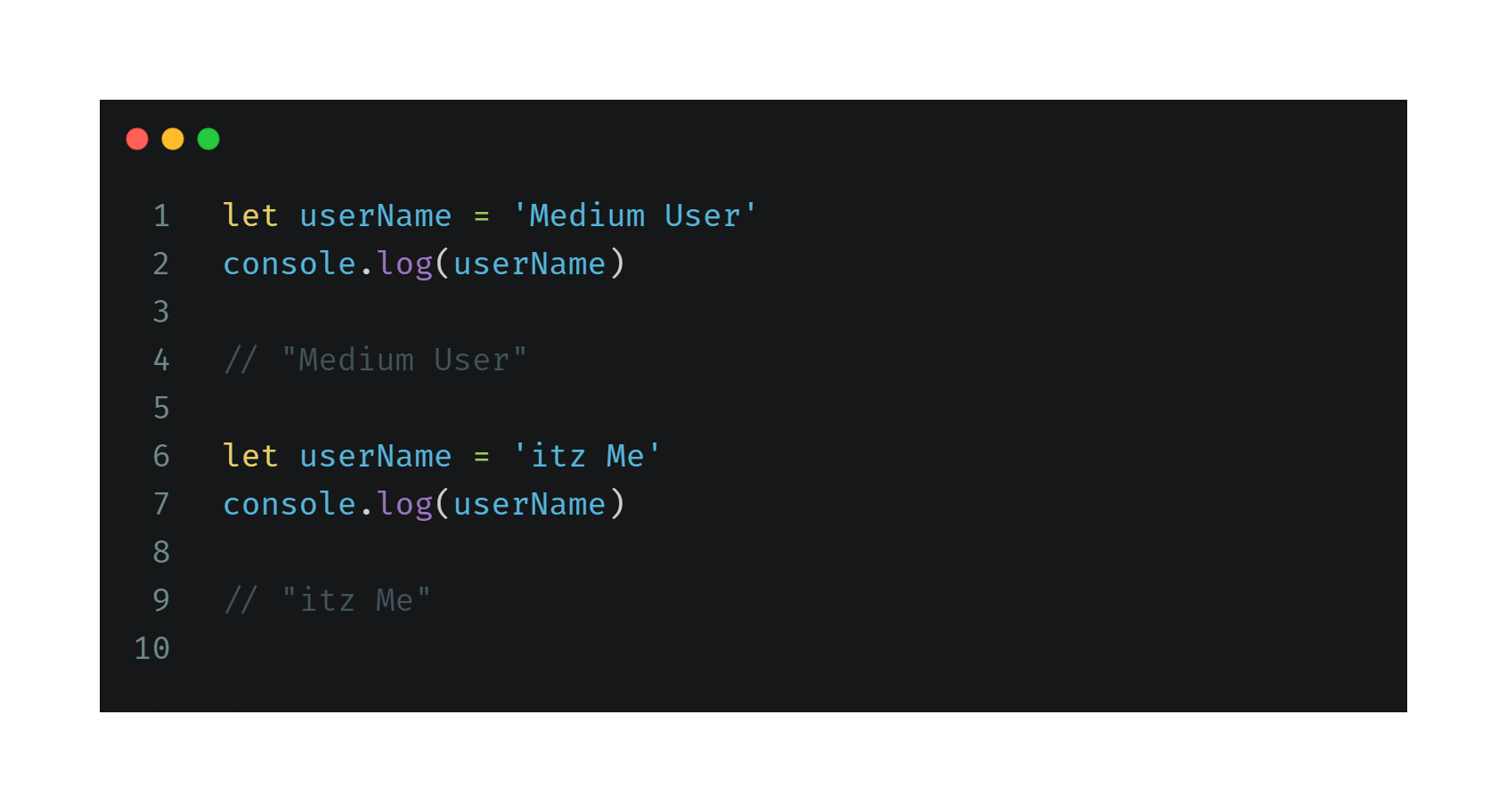
If we try to run this we can see it will throw an error ( if you try to copy and pasting on the browser that may not throw and error try creating a js file)
如果我们尝试运行此脚本,我们可以看到它将引发错误(如果您尝试在浏览器中复制并粘贴可能不会引发错误的消息,请尝试创建js文件)
Uncaught SyntaxError: Identifier 'userName' has already been declared

You had seen power of let
& will not create an issue in collaborating. if we had issue of redefining with the same name we will get an error and also save debugging time.
您已经看到let
&的强大功能不会在协作中产生问题。 如果我们遇到了使用相同名称进行重新定义的问题,则会收到错误消息并节省调试时间。
const- (const -)
The only difference in let
and const
is that variable created using const
can’t be reassigned with the new value. Here const
means constant.
let
和const
的唯一区别是使用const
创建的变量无法用新值重新分配。 const
是常量。
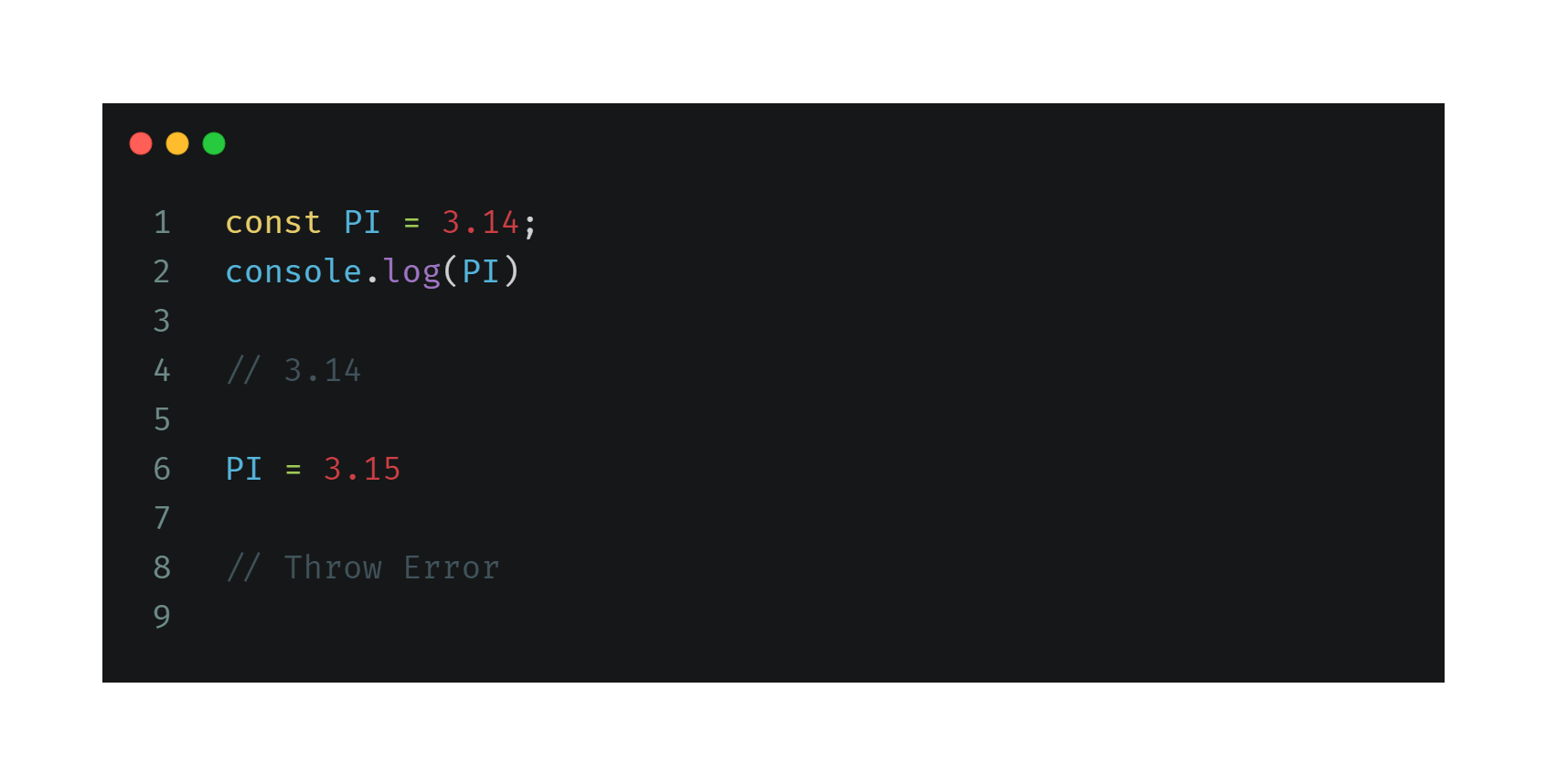
Everything is similarly to let
.
一切都与let
类似。
There is more with variable and JavaScript Hoisting I will cover it next blog.
还有更多关于变量和JavaScript提升的内容,我将在下一个博客中介绍。
Follow Me Medium — Kushal
跟我来— Kushal
Follow Me on Twitter — ikushaldave
在Twitter上关注我— ikushaldave
翻译自: https://medium.com/@ikushaldave/const-vs-let-vs-var-in-javascript-4abf0277ff07