fizzbuzz
Write a program that prints the numbers from 1 to 100.But for multiples of three print “Fizz” instead of the number and for the multiples of five print “Buzz”.For numbers which are multiples of both three and five print “FizzBuzz”.
编写一个程序,打印从1到100的数字。但是对于三倍的打印“ Fizz”(而不是数字)和五倍的打印“ Buzz”。对于三和五的倍数打印“ FizzBuzz” 。
I will post my own code snippets/solution towards the end, but for now, I want you to try and tackle these steps on your own as I explain.
我将在最后发布自己的代码段/解决方案,但是现在,我希望您像我解释的那样尝试自行解决这些步骤。
从哪里开始? (Where to even start?)
Let’s forget about languages for a second. What is required of us to solve this problem?
让我们暂时忘掉语言。 我们需要什么来解决这个问题?
To begin with, we simply need to print the numbers 1 to 100. Don’t worry about the next part of the problem. Just get the numbers 1 through 100 printed(in JavaScript you can just console.log your code).
首先 ,我们只需要打印数字1到100。不必担心问题的下一部分。 只需打印出1到100的数字即可( 在JavaScript中,您可以console.log代码 )。
Hint: In order to log every number 1 to 100, you will need a loop, console logging each number.
提示 :为了记录每个1到100的数字,您将需要一个循环,控制台记录每个数字。
After you have gotten the numbers 1 to 100 logged to your console, you are ready for the next step.
在将数字1到100记录到控制台后,就可以进行下一步了。
Next, we are asked to print “Fizz” for multiples of 3. What does this even mean? “A multiple of 3” is a number that is divisible by 3 with no remainder left over.
ñ 分机 ,我们被要求打印出“嘶嘶声”为3,这是什么,即使平均倍数? “ 3的倍数”是可以被3整除且无余数的数字。
So we need to check whether or not each number is divisible by 3. If the number is divisible by 3, you need to log “Fizz” instead of the actual number. You are already looping through 1–100, now we need to check for conditionals. Let’s use an if statement! One of the simplest ways to check for conditions in JavaScript.
因此,我们需要检查每个数字是否可被3整除。如果数字可被3整除,则需要登录“ Fizz”而不是实际数字。 您已经遍历了1–100,现在我们需要检查条件。 让我们使用一个if 语句 ! 检查JavaScript中条件的最简单方法之一。
If the number is divisible by 3, print “Fizz”, else continue logging all other numbers. Once you have this implemented, let’s move on.
如果 数字可以被3整除,请打印“ Fizz”, 否则继续记录所有其他数字。 一旦实现了这一点,让我们继续。
Next, we are asked to print “Buzz” for multiples of 5. If you were able to complete the previous step, this step should be a breeze.
ñ 分机 ,我们被要求打印“巴斯”为5的倍数。如果你能够完成上一步骤,这一步应该是轻而易举的事。
If the number is divisible by 5, print “Buzz”, else continue logging all other numbers.
如果 数字可被5整除,则打印“ Buzz”, 否则继续记录所有其他数字。
Finally, we are asked to print “FizzBuzz” for all numbers that are multiples of both 3 and 5. You’ve made it this far, how can you check if a number is divisible by multiple numbers?
˚Finally,我们被要求打印“FizzBuzz”对于那些既3和5的倍数找到了这一步,你怎么能检查是否一个数是由多个数字整除的所有数字?
Yes, an if statement, combined with the logical and operator.
是的,一个if语句 ,结合了逻辑和运算符。
If the number is divisible by both 3 and 5, print “FizzBuzz”, else continue logging all other numbers.
如果 数字可以被3和5整除,则打印“ FizzBuzz”, 否则继续记录所有其他数字。
By this point, you have solved the coding challenge. You are printing “Fizz” for all multiples of 3, you are printing “Buzz” for all multiples of 5, and lastly, you are printing “FizzBuzz” for all multiples of both 3 and 5.
至此,您已经解决了编码难题。 您要为3的所有倍数打印“ Fizz”,要为5的所有倍数打印“ Buzz”,最后,要为3和5的所有倍数打印“ FizzBuzz”。
Well done! Now let’s look at my own personal solution to this problem.
做得好! 现在,让我们看看我自己对这个问题的解决方案。
Remember: My code is written in JavaScript. Is it the most optimized solution? No. However, it’s readable and easy to understand. Other developers are going to be coming behind you weeks, months, maybe years down the line. Make your code readable!
记住:我的代码是用JavaScript编写的。 这是最优化的解决方案吗? 不会。但是,它易于阅读且易于理解。 其他开发人员将落后您数周,数月甚至数年的时间。 使您的代码可读!
将1到100记录到控制台 (Logging 1 through 100 to your console)
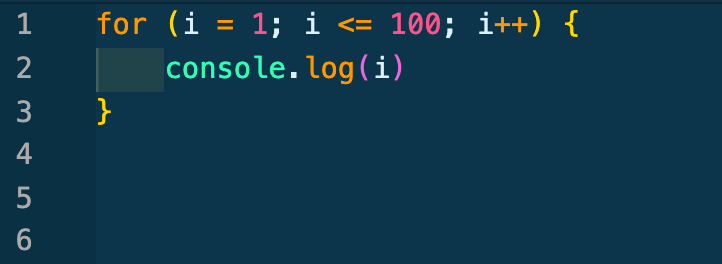
Here we are using JavaScript’s for loop, looping through the numbers 1 to 100, console logging each number. Notice, we are assigning a value to (i), and for each iteration of the loop as long as (i) is less than or equal to 100, we add 1 to (i). The conditions inside of the for loop curly braces {} run for every iteration of the loop, this is how we are able to log our numbers and words.
在这里,我们使用JavaScript的for loop ,在数字1到100之间循环,控制台记录每个数字。 注意,我们正在为( i )分配一个值,并且 对于循环的每次迭代,只要( i )小于或等于100,我们就将( i )加1。 for循环花括号{}内的条件在循环的每次迭代中都运行,这是我们能够记录数字和单词的方式。
打印“嘶嘶声”,而不是实际数字(3的倍数) (Print “Fizz” instead of the actual number for multiples of 3)
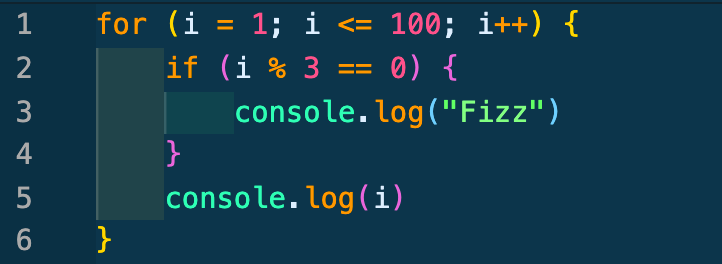
Getting closer! Inside of my if statement, I’m checking to see if (i) is divisible by 3 evenly. If so, I am logging “Fizz” to the console. If not, then (i) is logged to the console
越来越近! 在我的if语句中 ,我正在检查( i )是否被3整除。 如果是这样,我将“ Fizz”登录到控制台。 如果不是,则将( i )记录到控制台
I am performing the division using the remainder(%) operator. The remainder operator allows me to divide two numbers, checking to see if the remainder is equal to 0. If (i) divided by 3 = 0 (meaning the number is divisible by 3) we are console logging “Fizz” instead of the actual number, just like our problem statement is asking of us.
我正在使用余数 (%)运算符执行除法。 余数运算符使我可以将两个数相除,检查余数是否等于0。 ( 我 ) 除以3 = 0(意味着该数字可以被3整除),我们在控制台上记录“ Fizz”而不是实际数字,就像我们的问题陈述要求我们一样。
将“嗡嗡声”打印为5的倍数,而不是实际数字 (Print ”Buzz” for multiples of 5, instead of the actual number)
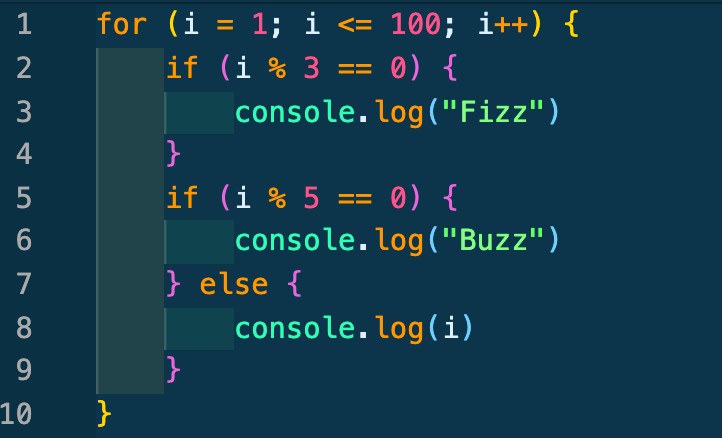
By now you should be catching on. If (i) is divisible by 3, log “Fizz”. If (i) is divisible by 5, log “Buzz”. Else, log all other numbers
到现在为止,您应该开始流行。 如果( i ) 被3整除,登录“ Fizz” 。 如果( i )被5整除,则记录“嗡嗡声”。 否则,记录所有其他数字
打印“ FizzBuzz” 3和5的倍数 (Print “FizzBuzz” for multiples of both 3 and 5)
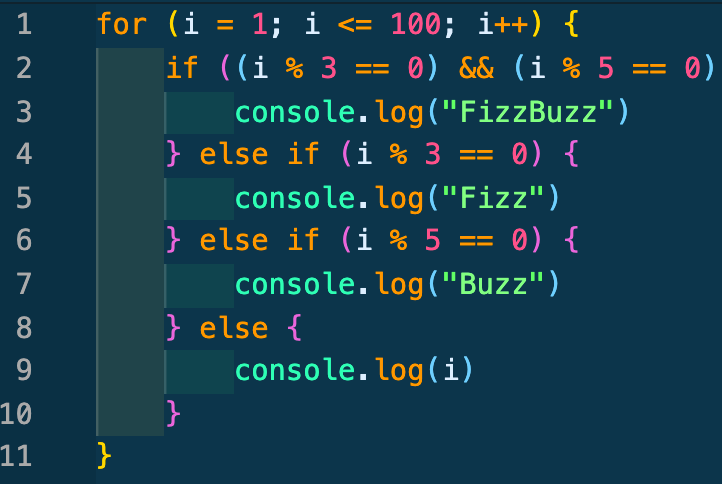
Now, you will notice a slight difference in my syntax from the previous images. Notice that now our very first conditional we are checking to see if the number (i) is divisible by both 3 and 5 evenly. If so, print “FizzBuzz”, else continue checking other conditionals.
现在,您会发现我的语法与以前的图像略有不同。 请注意,现在我们的第一个条件是检查数字 ( i )是否能被3和5均整。 如果是这样,请打印“ FizzBuzz”,否则继续检查其他条件。
And that’s it! Play around with your code, if you think you can optimize it, make a better solution, etc. Go for it! Share it! This solution is readable, and it does exactly what is needed.
就是这样! 如果您认为自己可以优化代码,提供更好的解决方案等,请试用一下代码。 分享它! 该解决方案可读性强,并且完全满足需要。
收盘时 (In Closing)
I write articles tech-related, non-tech-related, and a few things in between. I'm currently on this journey of life, just as you are. Let’s go make the most of it!
我写的文章与技术有关,与技术无关,介于两者之间。 和您一样,我目前正在人生的旅途中。 让我们充分利用它!
翻译自: https://medium.com/swlh/how-to-solve-fizzbuzz-in-javascript-e928150297a0
fizzbuzz