hmac api
Nowadays with the huge popularity of apps, more and more of us are using apps that handle sensitive private financial data, like mobile banking and trading digital assets.
如今,随着应用程序的广泛普及,我们中越来越多的人正在使用处理敏感私人财务数据的应用程序,例如移动银行和交易数字资产。
When it comes to security every system can be broken, us as app developers can only make it harder for hackers to break through and cause havoc. We can only secure apps by adding multiple layers of security to protect user data.
在安全性方面,每个系统都可能被破坏,我们作为应用程序开发人员只能使黑客更难以突破并造成破坏。 我们只能通过添加多层安全保护用户数据来保护应用安全。
With this article I hope to show you how to add another layer of protection to the communication between your app and your backend api.
我希望通过本文,向您展示如何为应用程序与后端api之间的通信增加另一层保护。
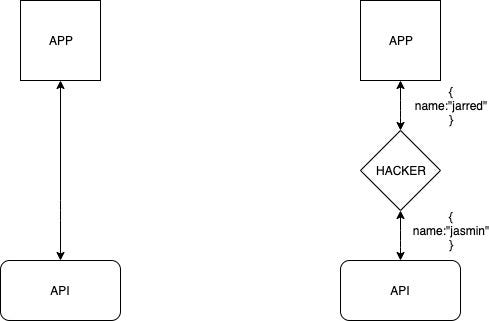
The diagram above illustrates the situation we will try to protect our app from, where a hacker intercepts the requests from our app and modifies the contents to be whatever they want.
上图说明了我们将试图保护我们的应用程序免受黑客攻击的情况,黑客拦截了来自我们应用程序的请求并将其内容修改为他们想要的内容。
Of course one of the ways we can protect the app from a man-in-the-middle attack is to use secure HTTPS and certificate pinning, here we will just be adding another layer to make it harder for the attacker to compromise our app.
当然,可以保护应用程序免受中间人攻击的一种方法是使用安全的HTTPS和证书固定,这里我们将添加另一层,以使攻击者更难以破坏我们的应用程序。
如何保护我们免受这种攻击? (How can we be protected from this attack?)
What we want to achieve is being able to ensure that whatever the api is receiving is what the app actually sent, so how can we do this?
我们想要实现的是能够确保api接收到的内容实际上是应用程序实际发送的内容,那么我们该怎么做呢?
Request body signing
请求正文签名
We can follow an approach called HMAC (Hash based message authentication code) in order to secure our request body.
我们可以采用一种称为HMAC (基于哈希的消息身份验证代码)的方法来保护我们的请求主体。
Using the HMAC we can create a hash (SHA-256) of the request body and send the hash along with the request where our api can verify the authenticity and integrity of our request body. Our api would then compute the HMAC of the received request body with the secret key and compare it with what was sent in the request, if the two hashes match we can say that the request body has not be altered in flight.
使用HMAC,我们可以创建请求主体的哈希(SHA-256),并将哈希与请求一起发送,我们的api可以验证请求主体的真实性和完整性 。 然后,我们的api将使用密钥计算接收到的请求主体的HMAC ,并将其与请求中发送的密钥进行比较,如果两个哈希值匹配,我们可以说请求主体在飞行中没有被更改。
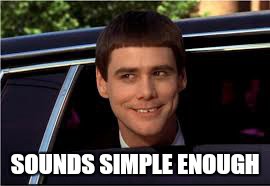
not so fast, the problem with computing an HMAC is that we need a key to be able to perform the hashing. but where can we store this key in the app?
没那么快,计算HMAC的问题在于我们需要能够执行散列的密钥。 但是我们可以将该密钥存储在应用程序中的什么位置?
- Source code 源代码
- A .txt file .txt文件
- A binary file 二进制文件
If we just hard code the key in our source code, a hacker could decompile our .apk and read our source code and extract our key and use it to correctly sign all kinds of request body.
如果我们只是在源代码中对密钥进行硬编码,则黑客可能会反编译.apk并读取我们的源代码,然后提取我们的密钥并使用它来正确签名各种请求正文。
Same goes for the other two options…
其他两个选项也一样...
A way to store the key more securely and make it harder for hackers to retrieve it would be to use native code and the Android NDK. As native code written in C++ would be a lot harder to disassemble and read our secret key.
一种更安全地存储密钥并使黑客更难检索密钥的方法是使用本机代码和Android NDK。 由于用C ++编写的本机代码将很难反汇编和读取我们的密钥。
This is not a fool proof solution to save the secret from disassembly, it is possible but this just adds another layer for a hacker to bypass.
这不是一个万无一失的解决方案,它可以防止机密被拆卸,这是有可能的,但这只是为黑客绕开了另一层。
https://developer.android.com/studio/projects/add-native-code
https://developer.android.com/studio/projects/add-native-code
让我们看一些代码... (lets see some code…)
First we need to setup an android studio project that has native C++ support
首先,我们需要设置一个具有本地C ++支持的android studio项目
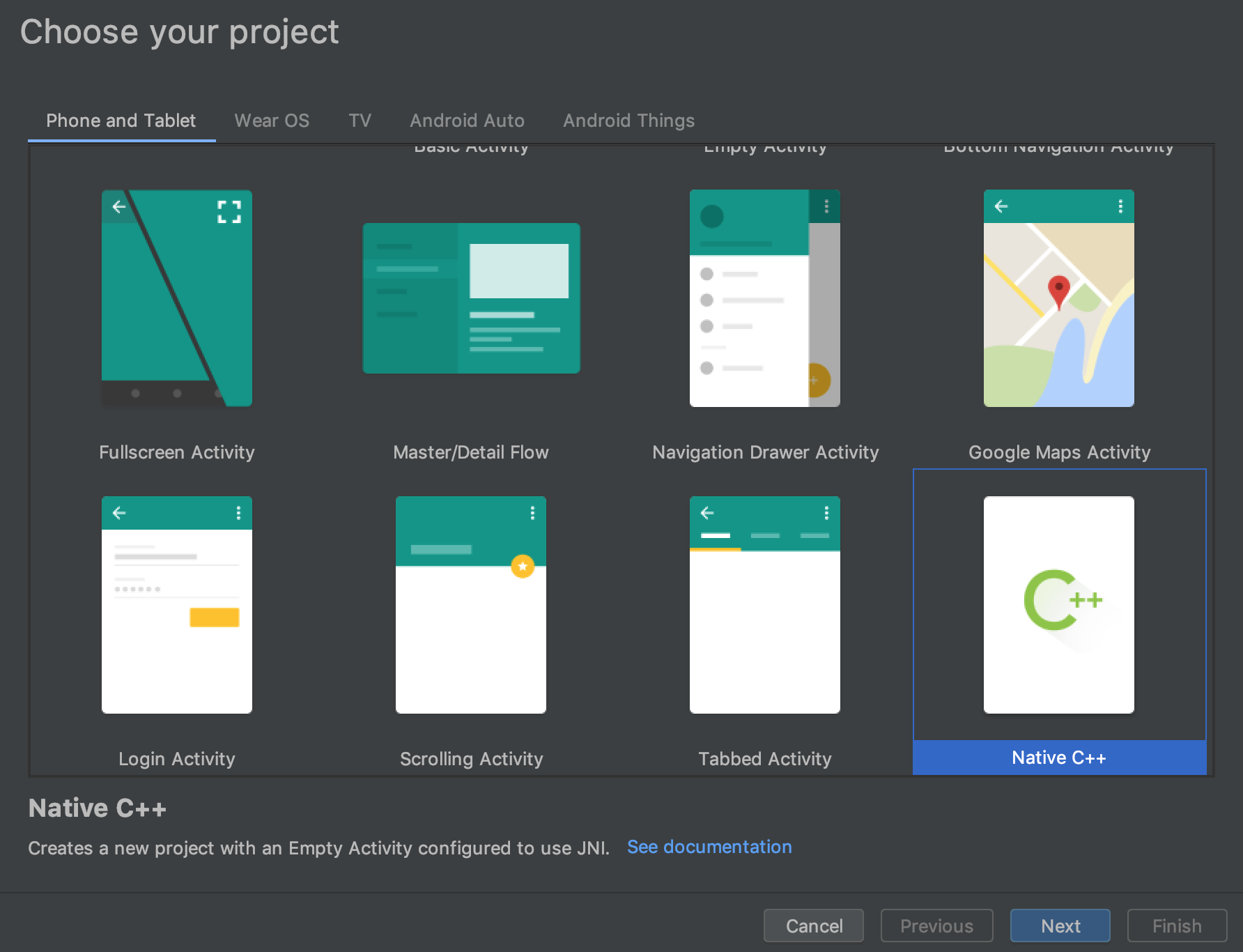
Android studio will automagically create the necessary files; additions to your build.grade, CMAKE file and a C++ file.
Android Studio将自动创建必要的文件; 除了build.grade,CMAKE文件和C ++文件。
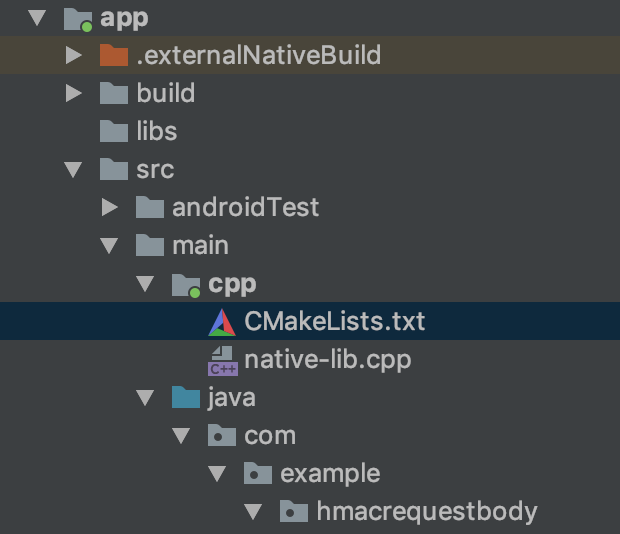
The native-lib.cpp is where we will store our secret key, it’s a C++ file.
native-lib.cpp是我们将存储密钥的位置,它是一个C ++文件。
The file is really simple all we have is a method that returns a string which would be our secret key.
该文件非常简单,我们所拥有的只是一个返回字符串的方法,该字符串将成为我们的密钥。
The method name Java_com_example_hmacrequestbody_HttpClient_clientSecret must include the full package name of where this method will be called from. We can split this one up as follows:
方法名称Java_com_example_hmacrequestbody_HttpClient_clientSecret必须包含从中调用该方法的完整程序包名称。 我们可以将其拆分如下:
java_com_example_hmacrequestbody
java_com_example_hmacrequestbody
The directory path
目录路径
HttpClient
HttpClient
The class in which the method is called
调用方法的类
clientSecret
客户秘密
The method name
方法名称
HMAC和正文签名 (HMAC and body signing)
Now that we have our secret key stored in our app, we need to somehow:
现在我们已经将密钥存储在我们的应用程序中,我们需要以某种方式:
- retrieve it 找回它
- sign our request body 在我们的请求正文上签名
- append signature to request header 将签名附加到请求标头
We do that by first loading our native library, in the example it’s the first thing done when the class is instantiated in the init block. Then we have our function that returns the secret key as a String as an external fun.
我们首先加载本机库来完成此操作,在该示例中,这是在init块中实例化该类时要做的第一件事。 然后,我们有一个函数,该函数以外部乐趣形式将密钥作为String返回。
Now that we have our secret key we need to use it in our cryptographic hashing function, for the purpose of this example we will be using HmacSHA256. It’s important to note here, that the string data that we are computing the cryptographic hash of, is the appended strings of;
现在我们有了秘密密钥,我们需要在加密哈希函数中使用它,就本例而言,我们将使用HmacSHA256 。 这里需要注意的重要一点是,我们正在计算其密码哈希的字符串数据是附加的字符串;
- JSON body of the request serialized 序列化的请求的JSON主体
- The request URL 请求网址
This ensures that the hash would be unique in two dimensions of endpoint and body data.
这确保哈希在端点数据和主体数据的两个维度上是唯一的。
And finally the the hash is encoded to a String using BASE64
最后,使用BASE64将哈希值编码为字符串
放在一起 (Putting it all together)
To use the function we just call it like any other function, and put it all together here.
要使用该函数,我们只需像调用其他任何函数一样将其调用,然后将其放在一起即可。
Here we are using the standard OkHTTP library for making our request, as you can see the hmac goes into the header of the request.
在这里,我们使用标准的OkHTTP库发出请求,因为您可以看到hmac进入了请求的标头。
That would be it for the App side of this example, we would need to adapt our server side code to handle the additional header called “authorisation” and along with the clients secret key then verify that that the hmac sent corresponds to the body data in the request, maybe I’ll write another medium post demonstrating this side.
就本例的App端而言,就是这样,我们需要调整服务器端代码以处理称为“授权”的附加标头,并与客户端秘密密钥一起,然后验证发送的hmac是否对应于其中的主体数据。该请求,也许我会写另一篇文章说明这一方面。
If you’ve made it this far, I’d like to thank you for taking the time to read my article and hope you gained some useful knowledge. It was a fun exercise and it’s always great to contribute some knowledge to the Android community.
如果您已经做到了这一点,我要感谢您抽出宝贵的时间阅读我的文章,并希望您获得了一些有用的知识。 这是一个有趣的练习,向Android社区贡献一些知识总是很棒的。
✌️✌️
✌️✌️
翻译自: https://medium.com/@jarredmartin/secure-app-api-communication-using-an-hmac-316314b63445
hmac api