python 示例
I’m listening to Eminem as I’m writing this and I’m pretty sure he didn’t know much about Data Types in Python
; or even Data Science for that matter. Then again, I’m pretty sure he wouldn’t be that interested in it as he’s doing pretty well for himself.
在写这篇文章时,我正在听Eminem,我可以肯定他对Python
数据类型了解不多; 甚至是数据科学。 再说一次,我很确定他不会对此感兴趣,因为他为自己做的很好。
Python
is a very dynamic language and the flexibility on offer has resulted in it becoming one of the most popular languages: you can literally build anything in Python
.
Python
是一种非常动态的语言,所提供的灵活性使它成为最受欢迎的语言之一:您可以使用Python
真正地构建任何东西。
Given that, the types of data you use in Python
are the very foundations that you’ll build your research on. Concepts like immutability
seem complicated at first but will eventually become centre to a lot of the decisions you make.
鉴于此,您将在Python
上使用的数据类型是您进行研究的基础。 immutability
概念immutability
看起来似乎很复杂,但最终将成为您做出的许多决定的中心。
As such, knowing the different data types will help your research projects as you’ll need to be able to comfortably control, handle and augment data.
因此,了解不同的数据类型将对您的研究项目有所帮助,因为您需要能够轻松地控制,处理和扩充数据。
In what follows, I go over a variety of topics and briefly demonstrate their various forms of function.
在接下来的内容中,我将讨论多个主题,并简要演示它们的各种形式的功能。
数值类型 (Numeric Types)
A Numeric
data type can be of different varieties. It can be Integer
, Float
or Complex
Numbers.
Numeric
数据类型可以具有不同的种类。 它可以是Integer
, Float
或Complex
。
Integers
are a whole number which can be positive or negative. Integers
can be Decimal
(base 10), Octal
(base 8) or Hexadecimal
(base 16). For example, 5 is Decimal
, 0o546 is Octal
and 0X5AE is Hexadecimal
. An Octal
number must start with 0o and a Hexadecimal
must start with 0X, otherwise, Python
will throw error.
Integers
是可以为正或为负的整数。 Integers
可以是Decimal
(以10为底), Octal
(以8为底)或Hexadecimal
(以16为底)。 例如,5是Decimal
,0o546是Octal
,0X5AE是Hexadecimal
。 Octal
数必须以0o开头,而Hexadecimal
必须以0X开头,否则, Python
会引发错误。
Floats
are real numbers with a decimal point. For scientific notations, sometimes there can be a character e or E, followed by a positive or negative integer, may be appended. For example, 7.5 is a Float
. If you perform any mathematical operation with an integer and a float, the result will be a float.
Floats
是带小数点的实数。 对于科学记数法,有时可能会附加字符e或E,后跟正或负整数。 例如,7.5是Float
。 如果使用整数和浮点数执行任何数学运算,则结果将是浮点数。
Complex
Numbers are specified as (real part) + (imaginary part). For example, a complex
number is 3+4j. Remember, it does not matter how you write it (you can write as 4j+3), but Python
will always take the real part first and then the imaginary
part (3+4j).
Complex
数字被指定为(实部)+(虚部)。 例如, complex
是3 + 4j。 请记住,如何编写(可以写为4j + 3)并不重要,但是Python
始终会先占实部,然后是imaginary
部(3 + 4j)。
Let us see some examples of Numeric
data types in python
.
让我们来看一些python
的Numeric
数据类型的例子。
# Float Data Type
d = 2.5
print("Float Data :", d)e = a/d
print("Integer and Float Mathematical Operations result in Float Data: ", e)# Complex Number Data Type
f = 3 + 4j
print("Complex Number : ", f)g = 5j + 6
print("Real Part first, then Complex part next: ", g)
The Output of the above program will be as follows:
以上程序的输出如下:

序列类型 (Sequence Types)
A sequence
is an ordered collection
of similar or different types of elements. In many programming languages, array
is considered as the sequence
data type, but Python
does not use array. It uses more versatile data types like String
, List
and Tuple
.
sequence
是相似或不同类型元素的有序collection
。 在许多编程语言中, array
被视为sequence
数据类型,但是Python
不使用数组。 它使用更通用的数据类型,例如String
, List
和Tuple
。
字符串类型 (String Types)
Python
does not support character
data types. In Python, a character is a String of length 1. A String
is a collection of one or more characters inside quotes. The quotes can be single (‘’), double (“”) or triple (‘’’’’’). Accessing any element of a String
can be done using Indexing. Indexing starts from 0 in Python
. Let us look at an example of how to access elements of a String
using Indexing.
Python
不支持character
数据类型。 在Python中,字符是长度为1的String
。 String
是引号内一个或多个字符的集合。 引号可以是单(”),双(“”)或三(“''”)。 可以使用索引来访问String
任何元素。 在Python
索引从0开始。 让我们看一个如何使用索引访问String
元素的示例。
str = ‘Hello World’
print(str[0]) # Output: "H"
print(str[3]) # Output: "l"
print(len(str)) # Output: 11
In the above code chunk, we can see that using the index position allows us to access the element of a String
. In the String
“Hello World”, The 1st element is “H” which is at index position 0. Similarly, at index position 3, the element is “l”.
在上面的代码块中,我们可以看到使用索引位置可以访问String
的元素。 在String
“ Hello World”中,第一个元素是“ H”,它在索引位置0。类似地,在索引位置3,元素是“ l”。
One important aspect of String
is that they are immutable
. For an immutable object, it fixes its value once it is assigned. Let us look at the following example.
String
一个重要方面是它们是immutable
。 对于不可变的对象,一旦分配,它就固定其值。 让我们看下面的例子。
a = “Hello”
print(id(a)) # Output : 4352473136
likewise:
同样地:
a = “World”
print(id(a)) # Output : 4352473328
further:
进一步:
print(a[0]) # Output: W
further:
进一步:
a[0] = “x”
## Output: TypeError: 'str' object does not support item assignment
In this example, when we assign a = “Hello” and then a = “World”, it does not mean “Hello” is replaced by “World”. It means that another section of the memory
is now assigned a value of “World” i.e., there are now two different memory blocks
containing “Hello” and “World” and the variable a is assigned now to the memory block
containing “World” that previously was pointing to the memory block
containing “Hello”. This is called immutability
and when we are trying to change the value of the String
, it is giving error.
在此示例中,当我们先分配一个=“ Hello” ,然后分配一个=“ World”时 ,并不意味着“ Hello”被“ World”代替。 这意味着现在为memory
另一部分分配了“世界”值,即,现在有两个不同的memory blocks
包含“你好”和“世界”,并且变量a现在已分配给包含“世界”的memory block
,以前指向的是包含“ Hello”的memory block
。 这称为immutability
,当我们尝试更改String
的值时,它给出了错误。
清单类型 (List Types)
A List
is an ordered set of elements enclosed in square brackets. A list
can have multiple types of data types. A List
is mutable
. Objects
can be added or removed from a list
. When you print a list
, it retains the order in which the elements were inserted into the list
. Elements of a list
can be accessed using Indexing
. Let us look a few examples of list
and indexing on a list
.
List
是括在方括号中的元素的有序集合。 list
可以具有多种类型的数据类型。 List
是mutable
。 可以在list
添加或删除Objects
。 当您打印list
,它会保留将元素插入list
的顺序。 list
元素可以使用Indexing
访问。 让我们看一下list
和在list
上建立索引的一些示例。
# Empty List
list1 = list()
print("Empty List: ", list) # Sequence in List
list2 = list(range(5))
print("Sequence in a List: ", list2)# Variables in sequence
a = "COEUS"
list3 = list(a)
print("Variable in a sequence in a list: ", list3)# Accessing list, indexing
list4 = ['COEUS', 'Machine Learning', 'Python']
print("The List is ", list4)
print("First Element of List4 is ", list4[0])
print("Last Element of List4 is ", list4[-1])
The output of the above code snippet is as follows:
上面的代码片段的输出如下:

元组类型 (Tuple Types)
A tuple
is like a list
, but it is immutable
. As a tuple
is immutable
, it cannot be changed. But a subset of tuple can be used to create a new tuple. All the index operations in a tuple is same as that of list. A tuple can be created without any parenthesis also. It is called as “Tuple Packing”. Let us take a look at a code snippet of tuple.
tuple
就像一个list
,但它是immutable
。 由于tuple
是immutable
,因此无法更改。 但是,可以使用元组的子集来创建新的元组。 元组中的所有索引操作都与列表相同。 也可以在没有任何括号的情况下创建一个元组。 它被称为“元组包装”。 让我们看一下元组的代码片段。
# Declaring a tuple
tup1 = ("Python", "Machine Learning", 15, 35)# Index in a Tuple
tup2 = ("Python", "Java", "C#", "SQL")
print("Index operation in tuple ", tup2.index("C#"))
print("R" in tup2)
print("SQL" in tup2)
print(tup2[1:3])
print(tup2[-1])
Where the output of the above snippet is,
以上代码段的输出是

布尔型 (Boolean Type)
Python
supports Boolean
Data Types. There are two values for Boolean
data types — True
and False
. Python is case sensitive, so True
is not same as ‘true’. Hence, using true and false in Python will throw an error:
Python
支持Boolean
数据类型。 Boolean
数据类型有两个值True
和False
。 Python区分大小写,因此True
与'true'不同。 因此,在Python中使用true和false将引发错误:
print(type(True)) # Output : <class ‘bool’>
print(type(False)) # Output : <class ‘bool’>
print(type(true)) # Output : NameError: name 'true' is not defined
print(type(false)) # Output : NameError: name 'false' is not defined
In the screenshot above, we can see that the type “bool” is being returned for “True
” and “False
”. But when we are putting something else, it is throwing error.
在上面的屏幕截图中,我们可以看到为“ True
”和“ False
”返回的类型为“布尔”。 但是当我们放其他东西时,它会抛出错误。
设定类型 (Set Type)
Set
is an unordered collection of unique elements i.e., no duplicate values exist in a set
. The elements in a set
is heterogeneous i.e., different types of elements can be there in a set
. Set’s
are also mutable. Let us look at some examples of set in the following screenshot.
Set
是唯一元素的无序集合,即set
不存在重复值。 在该元件set
是异质即,不同类型的元素可在那里set
。 Set's
也是可变的 。 让我们在以下屏幕快照中查看set的一些示例。
set1 = {1,2,3,4,5}#Defining a set using the set() function
a = set("123456")
print(a)
# Printing two sets
print("Set1 :", set1)
set2 = {4,5,6,7,8}
print("Set 2:", set2)# Union of two sets
print("union of set1 and set2 results in:")
print(set1|set2)# Intersection of two sets
print("Intersection of set1 and set2 results in:")
print(set1&set2)# Difference of two sets
print("Difference of set1 and set2 results in: ")
print(set1 - set2)
print("Difference of set2 and set1 results in: ")
print(set2 - set1)
The output of the above snippet is,
以上代码段的输出是,

字典类型 (Dictionary Type)
A Dictionary
is an unordered collection of arbitrary objects. Dictionaries
have a key-value pair, rather than positional offset. This is where dictionary
is different from List
, Tuple
or Set
which work on the offset positions. Dictionary
is represented as a series of “key
:value
” pairs, separated by commas and enclosed in curly braces {}. Dictionary
items can be fetched using the “key
”. All the keys in a dictionary
are in sorted order. The following code snippet screenshot gives some examples of a dictionary
in Python
.
Dictionary
是任意对象的无序集合。 Dictionaries
具有键值对,而不是位置偏移。 这是dictionary
不同于List
, Tuple
或Set
,后者在偏移位置上工作。 Dictionary
用一系列“ key
: value
”对表示,用逗号分隔并用花括号{}括起来。 可以使用“ key
”来获取Dictionary
项。 dictionary
中的所有键均按排序顺序。 下面的代码片段屏幕截图给出了一些Python
dictionary
示例。
# Creating an empty Dictionary
d1 = {}
print("Example of Empty Dictionary: ", d1)# Creating a dictionary with a key: value pair
d2 = {1: "Python", 2: "Machine Learning", 3: "AI", 4: "R"}
print("Example of a dictionary :", d2)# Functions in a dictionary
print("Fetching a value using a key: ", d2[2])
print("Fetching all the keys: ", d2.keys())
print("Length of d2L ", len(d2))
print(1 in d2)
print(5 in d2)
print("Values in d2: ", d2.values())
print("Items in d2: ", d2.items())
Where the output is,
输出在哪里
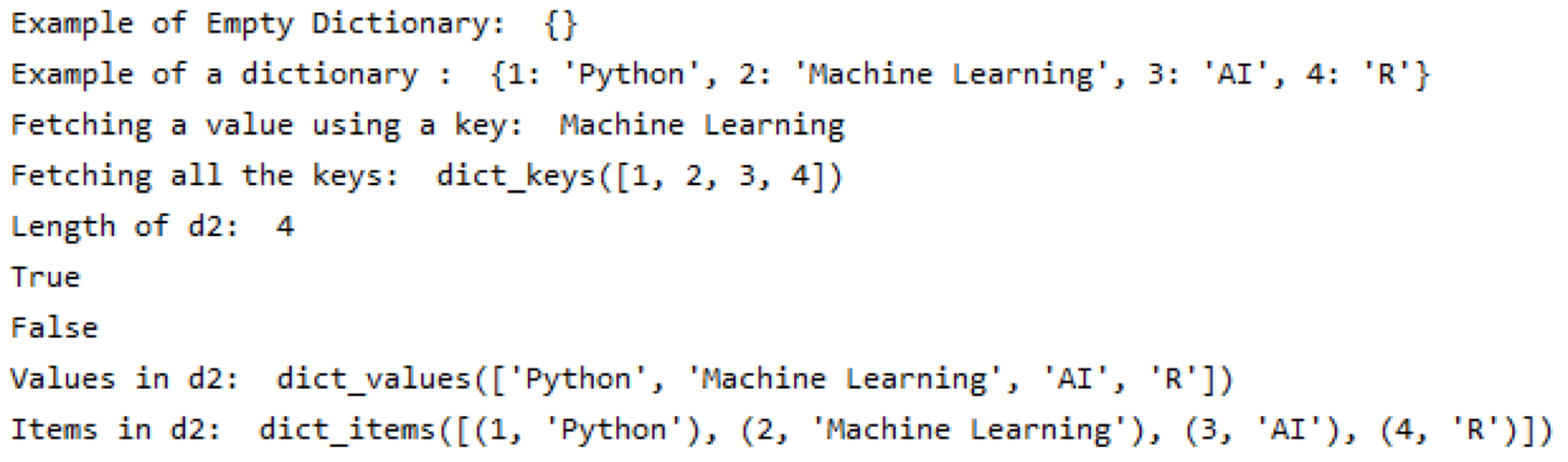
The above article is a short introduction into data types in Python, along with a few examples to help the reader with any implementation issues they may have.
上面的文章简要介绍了Python中的数据类型,并提供了一些示例来帮助读者解决他们可能遇到的任何实现问题。
Data Science has changed in the few years that it’s been around and as the python language itself develops, it’s important that any practitioners in the space understand these deep fundamentals so that if anything changes on the Python language side or the data they’ve received looks weird and isn’t playing ball, then they can at least quickly diagnose the issue and crack on.
数据科学在已经存在的几年中发生了变化,并且随着python语言本身的发展,重要的是,该领域的任何从业人员都必须了解这些深层的基础知识,以便在Python语言方面或他们收到的数据发生任何变化时很奇怪并且没有打球,那么他们至少可以Swift诊断出问题并继续前进。
Thanks for reading, and please let me know if you have any questions!
感谢您的阅读,如果您有任何疑问,请告诉我!
python 示例