Uploading user photos and videos to server is a common practice for customising app user’s profile or creating a post in social media. For example, Facebook, Twitter and WhatsApp, etc. Apple provides a simple-to-use tool UIImagePickerController
to allow developer to implement this feature within app in an easy way.
üploading用户的照片和视频服务器是用于自定义应用程序用户的配置文件或创建社交媒体后一种常见的做法。 例如,Facebook,Twitter和WhatsApp等。Apple提供了易于使用的工具UIImagePickerController
以允许开发人员以简单的方式在应用程序内实现此功能。
Developer can define the media type (photo or video) and the source (user’s album or camera) of file. User can also crop the selected image and cut the selected video before sending to app.
开发人员可以定义文件的媒体类型(照片或视频)和源(用户的相册或相机)。 用户还可以裁剪所选图像并剪切所选视频,然后再发送到应用程序。
In this article, it will cover the setup and main properties of each media selection method. Hope this can help you learn more about media selection in iOS! Let’s start!
在本文中,它将介绍每种媒体选择方法的设置和主要属性。 希望这可以帮助您了解有关iOS中媒体选择的更多信息! 开始吧!
从相册中选择照片和视频 (Select Photo and Video from Album)
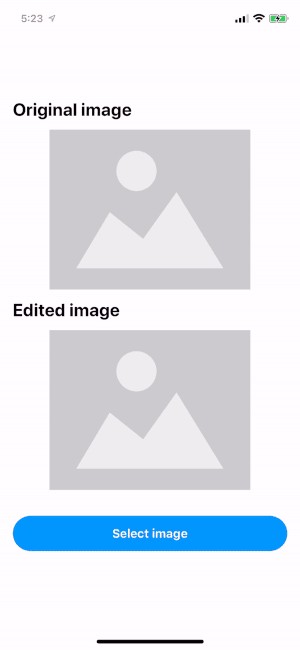
import UIKit
import MobileCoreServices
class FooDemoImagePickerViewController: UIViewController {
@IBAction func selectImageAction(_ sender: UIButton) {
let pickerController = UIImagePickerController()
/*
* Part 1: Select the origin of media source
Either one of the belows:
1. .photoLibrary -> Go to album selection page
2. .savedPhotosAlbum -> Go to Moments directly
*/
pickerController.sourceType = .savedPhotosAlbum
// Must import `MobileCoreServices`
// Part 2: Allow user to select both image and video as source
pickerController.mediaTypes = [kUTTypeImage as String, kUTTypeMovie as String]
// Part 3: User can optionally crop only a certain part of the image or video with iOS default tools
pickerController.allowsEditing = true
// Part 4: For callback of user selection / cancellation
pickerController.delegate = self
// Part 5: Show UIImagePickerViewController to user
present(pickerController, animated: true, completion: nil)
}
extension FooDemoImagePickerViewController: UIImagePickerControllerDelegate, UINavigationControllerDelegate {
// Discuss later
}
第1部分-sourceType (Part 1— sourceType)
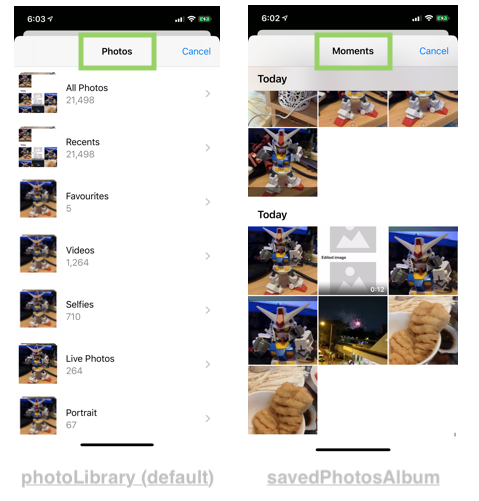
sourceType
represents the origin of the files. It can be either:
sourceType
表示文件的来源。 可以是:
photoLibrary
(default) shows album selection pagephotoLibrary
(默认)显示相册选择页面savedPhotosAlbum
shows the Moments page directlysavedPhotosAlbum
直接显示“时刻”页面
第2部分-mediaTypes (Part 2— mediaTypes)

mediaTypes
defines the types of file shown at the UIImagePickerViewController
and can be one of the below:
mediaTypes
定义在UIImagePickerViewController
显示的文件类型,可以是以下类型之一:
kUTTypeImage
represents image sourcekUTTypeImage
表示图像源kUTTypeMovie
represents video sourcekUTTypeMovie
表示视频源
By default, only
kUTTypeImage
is included in themediaTypes
. If you want to select video files, you must set it manually.Reference: MediaTypes official website默认情况下,
kUTTypeImage
仅包含mediaTypes
。 如果要选择视频文件,则必须手动设置。参考: MediaTypes官方网站
第3部分-allowEditing (Part 3 — allowEditing)
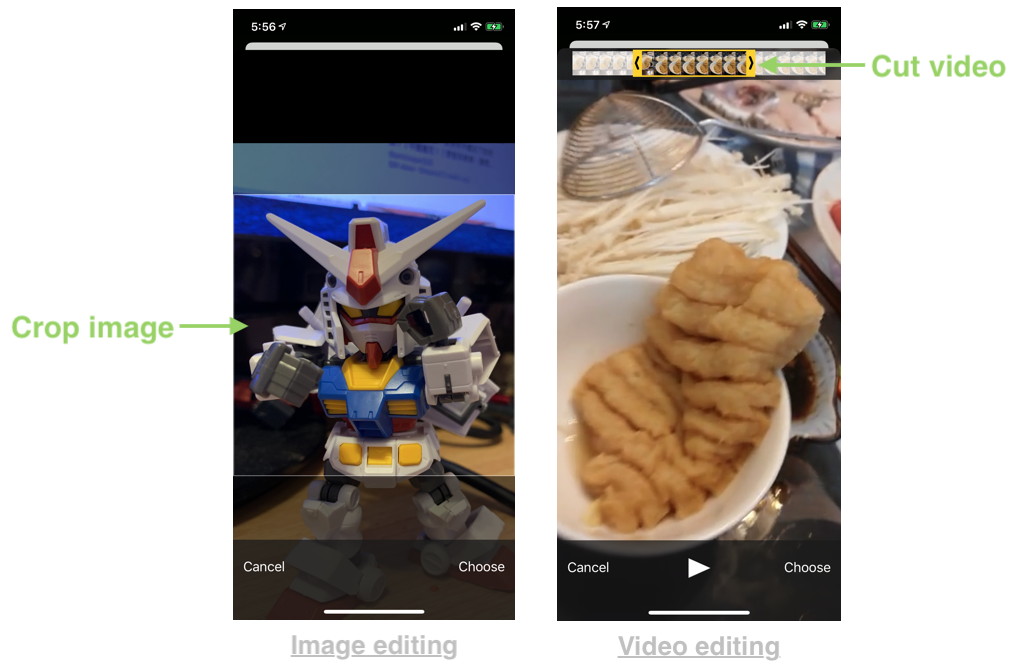
allowEditing
means user can edit the photo and video after selecting them. Image can be cropped and video can be cut.
allowEditing
表示用户可以在选择照片和视频后对其进行编辑。 可以裁剪图像和剪切视频。
第4部分-设置代表 (Part 4 — Setting delegate)

The delegate
property in UIImagePickerController
is in a special type. It is both UIImagePickerControllerDelegate
and UINavigationControllerDelegate
. The details will be discussed later session.
UIImagePickerController
的delegate
属性是一种特殊类型。 它是UIImagePickerControllerDelegate
和UINavigationControllerDelegate
。 细节将在以后的会议中讨论。
第5部分—当前的UIImagePickerViewController (Part 5 — Present UIImagePickerViewController)
The final part is just simply present the UIImagePickerViewController
as a normal UIViewController
.
最后一部分只是将UIImagePickerViewController
呈现为普通的UIViewController
。
拍摄照片和视频 (Capture photo and video)
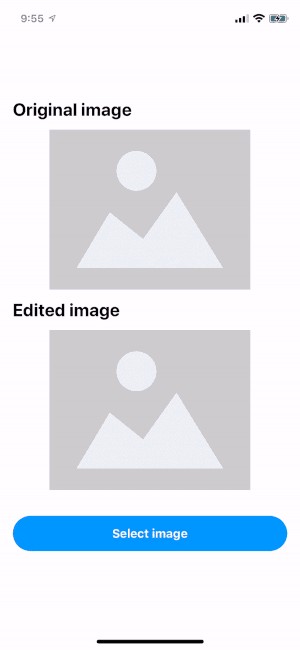
import UIKit
import MobileCoreServices
class FooDemoImagePickerViewController: UIViewController {
@IBAction func selectImageAction(_ sender: UIButton) {
let pickerController = UIImagePickerController()
// Part 1: File origin
pickerController.sourceType = .camera
// Must import `MobileCoreServices`
// Part 2: Define if photo or/and video is going to be captured by camera
pickerController.mediaTypes = [kUTTypeMovie as String, kUTTypeImage as String]
// Part 3: camera settings
pickerController.cameraCaptureMode = .photo // Default media type .photo vs .video
pickerController.cameraDevice = .rear // rear Vs front
pickerController.cameraFlashMode = .on // on, off Vs auto
// Part 4: User can optionally crop only a certain part of the image or video with iOS default tools
pickerController.allowsEditing = true
// Part 5: For callback of user selection / cancellation
pickerController.delegate = self
// Part 6: Present the UIImagePickerViewController
present(pickerController, animated: true, completion: nil)
}
}
第1部分:摄像头和麦克风的权限 (Part 1: Camera & Microphone Permissions)


Camera and microphone permissions must be granted before taking photos or recording a video. Developer must declared the NSCameraUsageDescription
and NSMicrophoneUsageDescription
at the info.plist
and system will then handle the permission request for us.
在拍照或录制视频之前,必须先授予相机和麦克风权限。 开发人员必须在info.plist
声明NSCameraUsageDescription
和NSMicrophoneUsageDescription
,然后系统将为我们处理许可请求。
第2部分-mediaTypes (Part 2 — mediaTypes)
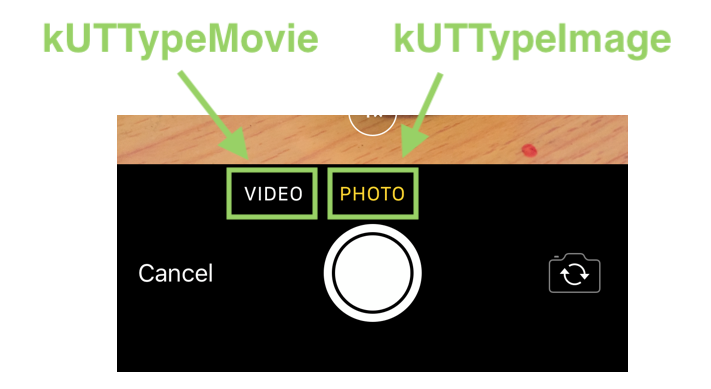
mediaTypes
defines the types of file captured by camera.
mediaTypes
定义相机捕获的文件类型。
By default, only
kUTTypeImage
is included in themediaTypes
. If you want to capture video files, you must set it manually.Reference: MediaTypes official website默认情况下,
kUTTypeImage
仅包含mediaTypes
。 如果要捕获视频文件,则必须手动设置。参考: MediaTypes官方网站
第3部分:相机设置 (Part 3: Camera settings)
There are 3 common camera parameters:
共有3个常见的相机参数:

cameraCaptureMode
: Either.photo
(default) or.video
. It defines whether photo or video is the initial media type when camera is launched.cameraCaptureMode
:.photo
(默认)或.video
。 它定义启动相机时照片或视频是初始媒体类型。cameraDevice
: Either.rear
(default) or.front
cameraDevice
:.rear
(默认)或.front
cameraFlashMode
: Can be.auto
(default),.on
or.off
.cameraFlashMode
:可以.auto
(默认),.on
或.off
。
第4部分—其他: allowEditing ,设置委托和呈现UIImagePickerViewController (Part 4 — Other: allowEditing, setting delegate & presenting UIImagePickerViewController)
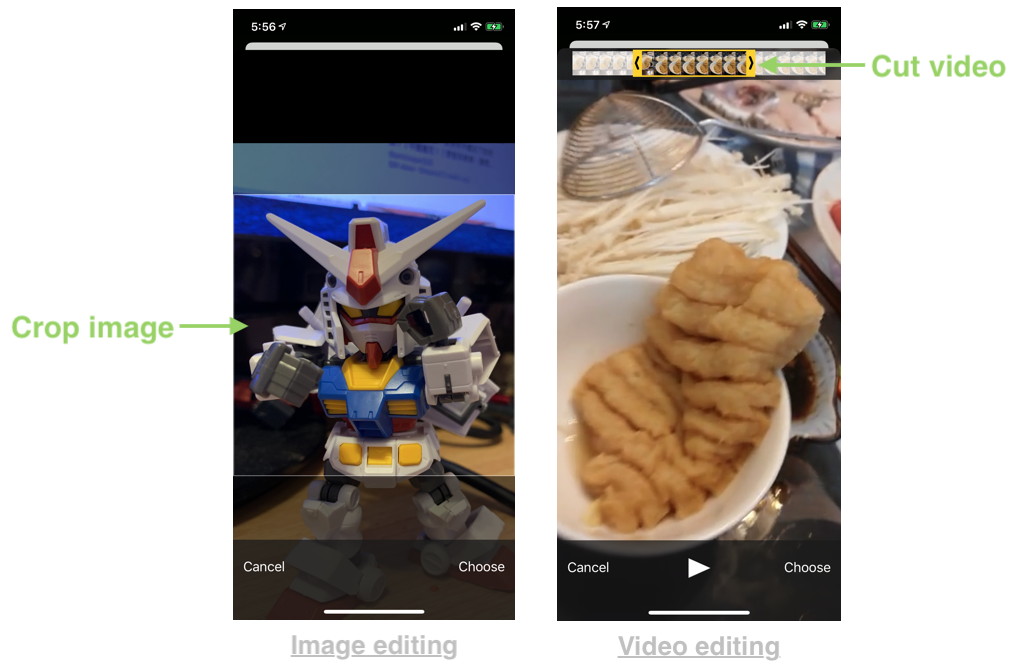
Other properties are the same as the one for selecting media from user’s album. Please refer to the previous session for more information.
其他属性与从用户相册中选择媒体的属性相同。 请参考上一届会议以获取更多信息。
UIImagePickerControllerDelegate
(UIImagePickerControllerDelegate
)
UIImagePickerControllerDelegate
is used to handle the user’s actions, including confirmation and cancelation of media selection. There are only two callbacks of UIImagePickerControllerDelegate
:
UIImagePickerControllerDelegate
用于处理用户的动作,包括确认和取消媒体选择。 UIImagePickerControllerDelegate
只有两个回调:
1. imagePickerController(_:didFinishPickingMediaWithInfo :) (1. imagePickerController(_:didFinishPickingMediaWithInfo:))
import MobileCoreServices
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [UIImagePickerController.InfoKey : Any]) {
// Check for the media type
let mediaType = info[UIImagePickerController.InfoKey.mediaType] as! CFString
switch mediaType {
case kUTTypeImage:
// Handle image selection result
print("Selected media is image")
let editedImage = info[UIImagePickerController.InfoKey.editedImage] as! UIImage
fooEditedImageView.image = editedImage
let originalImage = info[UIImagePickerController.InfoKey.originalImage] as! UIImage
fooOriginalImageView.image = originalImage
case kUTTypeMovie:
// Handle video selection result
print("Selected media is video")
let videoUrl = info[UIImagePickerController.InfoKey.mediaURL] as! URL
default:
print("Mismatched type: \(mediaType)")
}
picker.dismiss(animated: true, completion: nil)
}
This is the callback when user has just selected or captured a media (after optionally editing the file).
当用户刚刚选择或捕获媒体时(可选编辑文件之后),这是回调。
The info
contains all the data of the selected file and mediaType
can be used to distinguish whether image or video file is chosen. Different selected files return different information.
该info
包含所选文件的所有数据,并且mediaType
可用于区分是选择图像文件还是视频文件。 选择的不同文件返回不同的信息。
Original image and edited image can be retrieved back by the key originalImage
and editedImage
. Edited video URL can be retrieved by mediaURL
.
原始图像和编辑后的图像可以通过键originalImage
和editedImage
检索回来。 可以通过mediaURL
检索编辑的视频URL。
2. imagePickerControllerDidCancel(_ :) (2. imagePickerControllerDidCancel(_:))
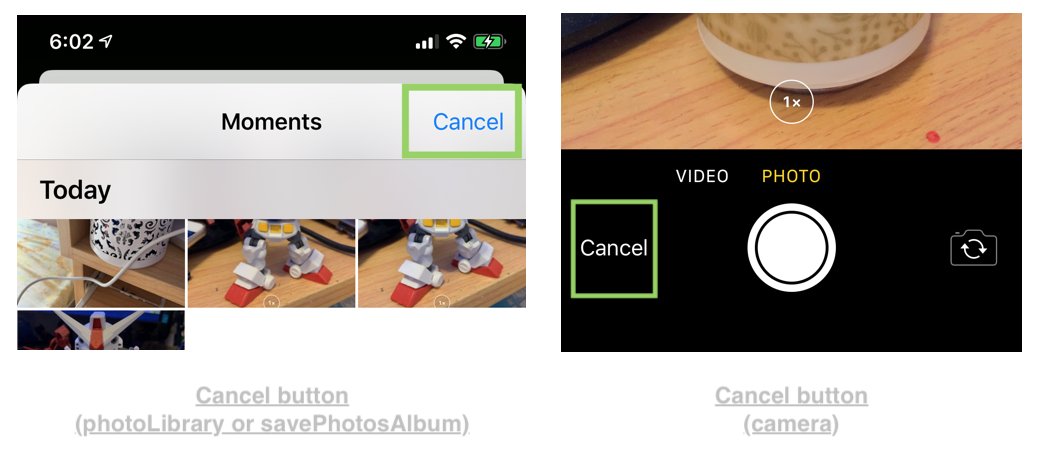
func imagePickerControllerDidCancel(_ picker: UIImagePickerController) {
// Dismiss the picker since it is not dismissed automatically
picker.dismiss(animated: true, completion: nil)
}
This callback is triggered when the cancel button is pressed in either the media selection mode or capture mode.
在媒体选择模式或捕获模式下按“取消”按钮时,将触发此回调。
In both callbacks of
UIImagePickerControllerDelegate
, it is developer’s responsibility to dismiss theUIImagePickerController
when user confirms or cancel the selection.在
UIImagePickerControllerDelegate
两个回调中,开发人员有责任在用户确认或取消选择时关闭UIImagePickerController
。
局限性 (Limitation)

- Only 1 file can be selected each time 每次只能选择1个文件
- Duration of captured video cannot be longer than 10 mins by default. 默认情况下,捕获的视频的时长不能超过10分钟。
- No grid line can be shown 无法显示网格线
- Not all photo types can be supported by UIImagePickerViewController. For example, panorama, live photos, time-lapse and slow motion UIImagePickerViewController并非支持所有照片类型。 例如,全景,实时照片,延时和慢动作
摘要 (Summary)
UIImagePickerViewController
provides an easy to use API for app user to select either photo or video from device. Media files can be selected or captured from Moments ,album or camera.UIImagePickerViewController
提供了易于使用的API,供应用程序用户从设备中选择照片或视频。 可以从Moments,相册或相机中选择或捕获媒体文件。Camera and microphone permissions are required to recording video and capturing photos by camera. Developer must declared the
NSCameraUsageDescription
andNSMicrophoneUsageDescription
at theinfo.plist
需要摄像机和麦克风权限才能通过摄像机录制视频和捕获照片。 开发人员必须在
info.plist
声明NSCameraUsageDescription
和NSMicrophoneUsageDescription
Camera can be configured with capture mode, camera device and flash mode. However, there is no grid line and extra photo mode in
UIImagePickerViewController
.可以为相机配置拍摄模式,相机设备和闪光模式。 但是,
UIImagePickerViewController
没有网格线和多余的照片模式。Developer needs to dismiss
UIImagePickerViewController
once user has confirmed or canceled the media selection process.用户确认或取消媒体选择过程后,开发人员需要关闭
UIImagePickerViewController
。
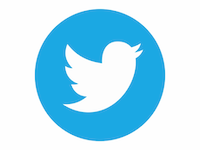
You are welcome to follow me at Twitter@myrick_chow for more information and articles. Thank you for reading this article. Have a nice day! 😄
欢迎您通过Twitter @ myrick_chow关注我,以获取更多信息和文章。 感谢您阅读本文。 祝你今天愉快! 😄
翻译自: https://itnext.io/swift-uiimagepickercontroller-e37ece745740