javascript编写
重点(Top highlight)
JavaScript is a very flexible programming language, and you can implement your requirements in many different ways. But following a few principles and tips can make your code more readable and efficient.
JavaScript是一种非常灵活的编程语言,您可以通过多种不同方式来实现您的要求。 但是遵循一些原则和技巧可以使您的代码更具可读性和效率。
1.以强类型语言的风格编写代码 (1. Write code in the style of a strongly typed language)
JavaScript is a weakly typed programming language in which a variable can have different types of values syntactically. But to improve compilation performance and make your code easier for other programmers to read, it is recommended that you write your code in a strongly typed style.
JavaScript是一种弱类型的编程语言,其中变量可以在语法上具有不同类型的值。 但是,为了提高编译性能并使代码更易于其他程序员阅读,建议您以强类型风格编写代码。
定义变量时应指定数据类型 (You should specify the data type when you define a variable)
Bad code:
错误代码:
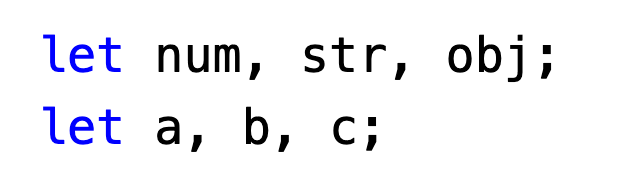
The variables in the above code lack type information, making it difficult for other programmers to understand the code or for the JavaScript interpreter to optimize.
上面代码中的变量缺少类型信息,这使得其他程序员难以理解代码或JavaScript解释器难以优化。
Good code:
好代码:

不要随意更改变量的类型 (Do not arbitrarily change the type of a variable)
Bad code:
错误代码:
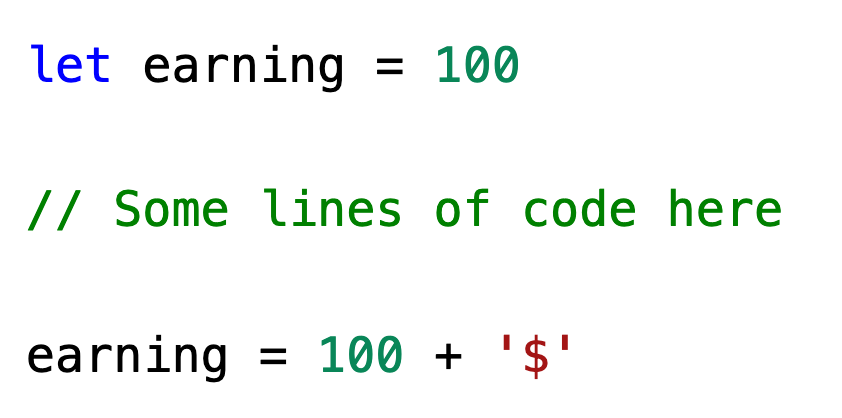
earning
is an integer at the beginning and then becomes a string. If someone else needs to read or modify this code, he or she is bound to have doubts about the code and even write buggy code.
earning
开头是整数,然后变成字符串。 如果其他人需要阅读或修改此代码,则他或她必然会对代码有疑问,甚至会编写错误的代码。
At the same time, JavaScript code in engines like V8 is converted to bytecode and then executed, and the data type in the bytecode is determined. If we change the data type of a variable in JavaScript code, the compiler has to do some extra processing, which can slow down the performance of the program.
同时,将V8等引擎中JavaScript代码转换为字节码,然后执行,并确定字节码中的数据类型。 如果我们更改JavaScript代码中变量的数据类型,则编译器必须进行一些额外的处理,这可能会降低程序的性能。
Good code:
好代码:
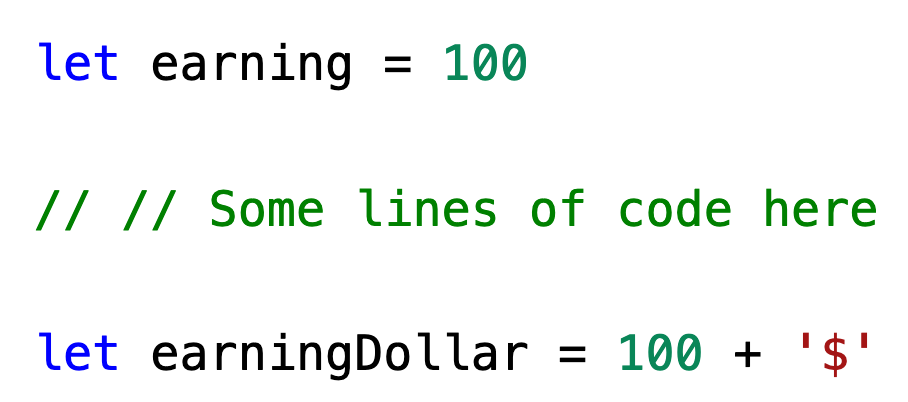
When you need to convert the type of a value, use a new variable.
当您需要转换值的类型时,请使用新变量。
函数的返回类型应固定 (The return type of a function should be fixed)
Bad code:
错误代码:
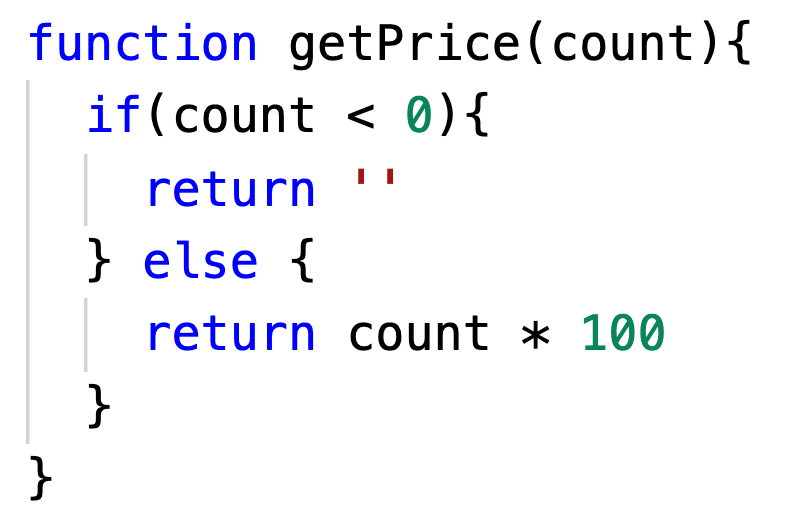
This function may return an integer or a string. Although this is in line with JavaScript syntax, it makes it difficult for the person calling the function to do the arithmetic directly with the result of getPrice()
.
该函数可以返回整数或字符串。 尽管这符合JavaScript语法,但使调用该函数的人很难直接使用getPrice()
的结果进行算术运算。
Good code:
好代码:
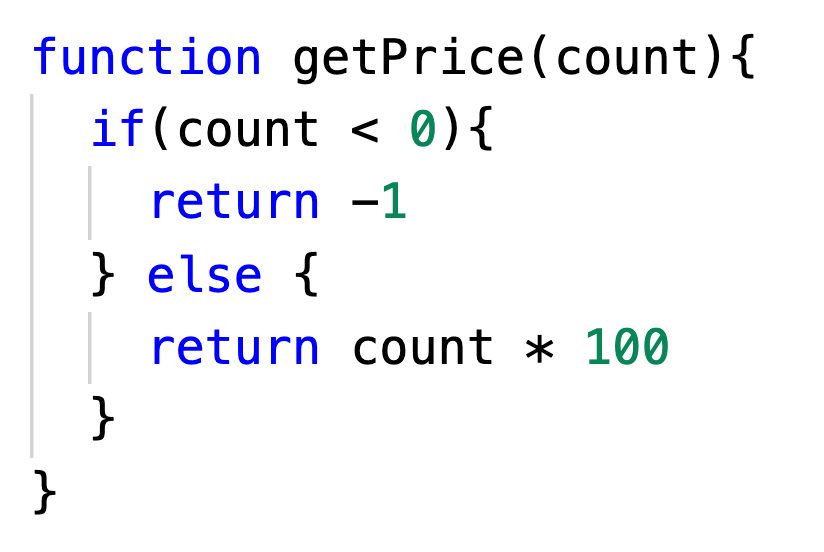
We can agree in a function comment that a return of -1
means the argument is invalid. This allows the caller to handle the result of the function in a uniform way.
我们可以在函数注释中达成一致,即返回-1
表示参数无效。 这允许调用者以统一的方式处理函数的结果。
2.减少不必要的范围查找 (2. Reduce unnecessary scope lookups)
JavaScript supports nested scopes and scope chain, which allows us to write efficient code. But using these syntaxes incorrectly messes up the code.
JavaScript支持嵌套作用域和作用域链,这使我们能够编写高效的代码。 但是使用这些语法会错误地弄乱代码。
如果没有必要,不要将代码暴露给全局范围 (Don’t expose your code to global scope if it’s not necessary)
Bad code:
错误代码:
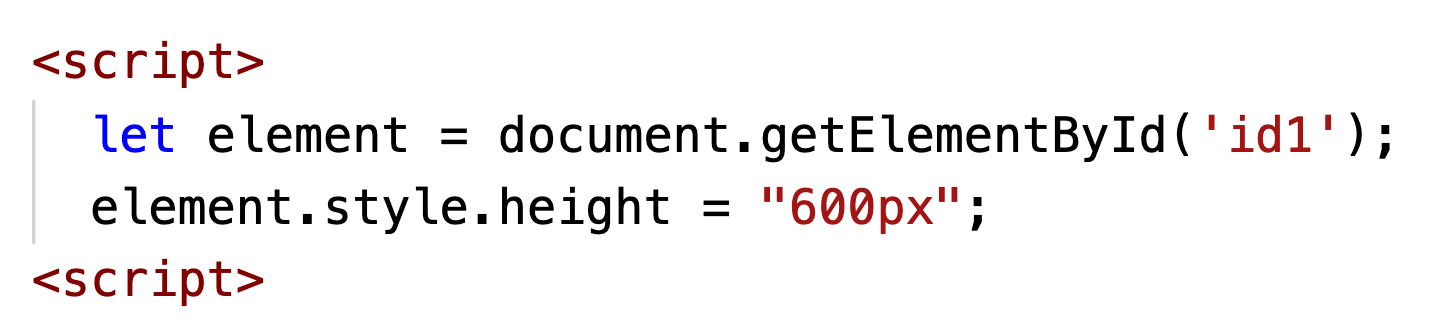
First of all, all variables in the script tag are in the global scope, and the code in different script tags may be developed by different programmers, which may cause naming conflicts.
首先,script标签中的所有变量都在全局范围内,并且不同脚本标签中的代码可能由不同的程序员开发,这可能会导致命名冲突。
Second, the second line of code above, when using the element
variable, look for the element
variable in the global scope, reducing the performance of the program.
其次,上面的第二行代码在使用element
变量时,在全局范围内查找element
变量,从而降低了程序的性能。
Good code:
好代码:
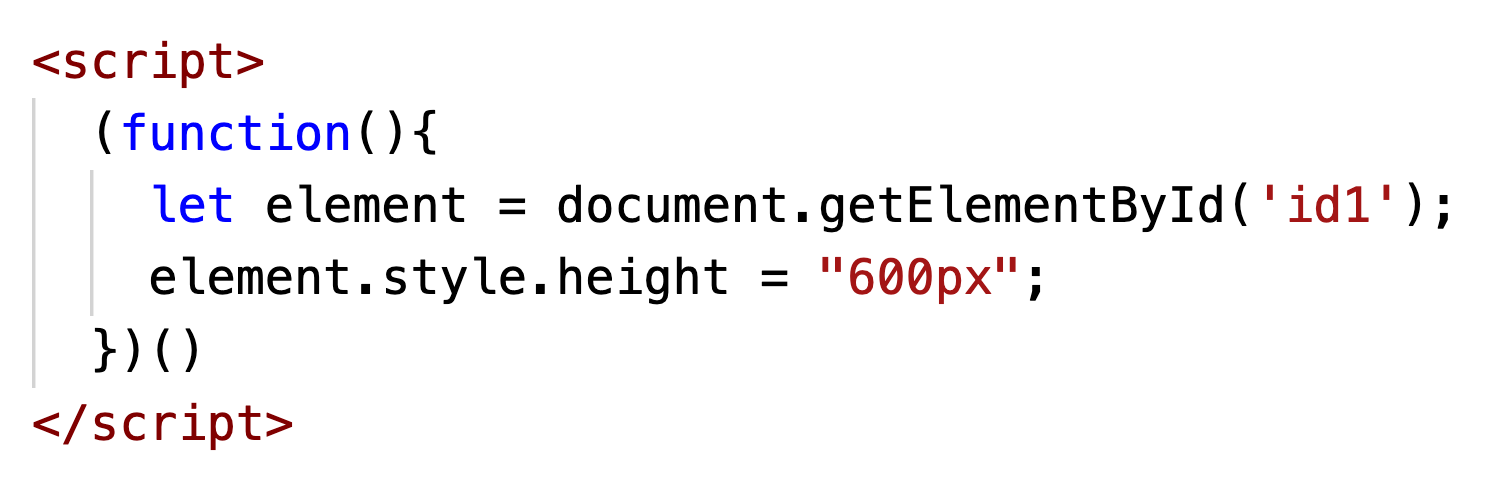
In this way, we use closures to hide the element
variable. This does not contaminate the global scope, and it is faster to find variables in the local scope.
这样,我们使用闭包来隐藏element
变量。 这不会污染全局范围,并且在局部范围内查找变量更快。
Of course, if you are sure that you will use the element
variable elsewhere, you should still expose it in the global scope.
当然,如果确定要在其他地方使用element
变量,则仍应在全局范围内公开它。
不要滥用闭包 (Don’t abuse closures)
JavaScript looks for variables by scope chain. If a variable cannot be found in the current scope, the JavaScript engine looks in the parent scope of the current scope, then up to the global scope level by level. So the deeper the closure is nested, the longer the variable lookup takes.
JavaScript按作用域链查找变量。 如果在当前作用域中找不到变量,则JavaScript引擎将在当前作用域的父作用域中查找,然后逐级查找全局作用域。 因此,闭包嵌套的深度越深,变量查找所花费的时间就越长。
Bad code:
错误代码:
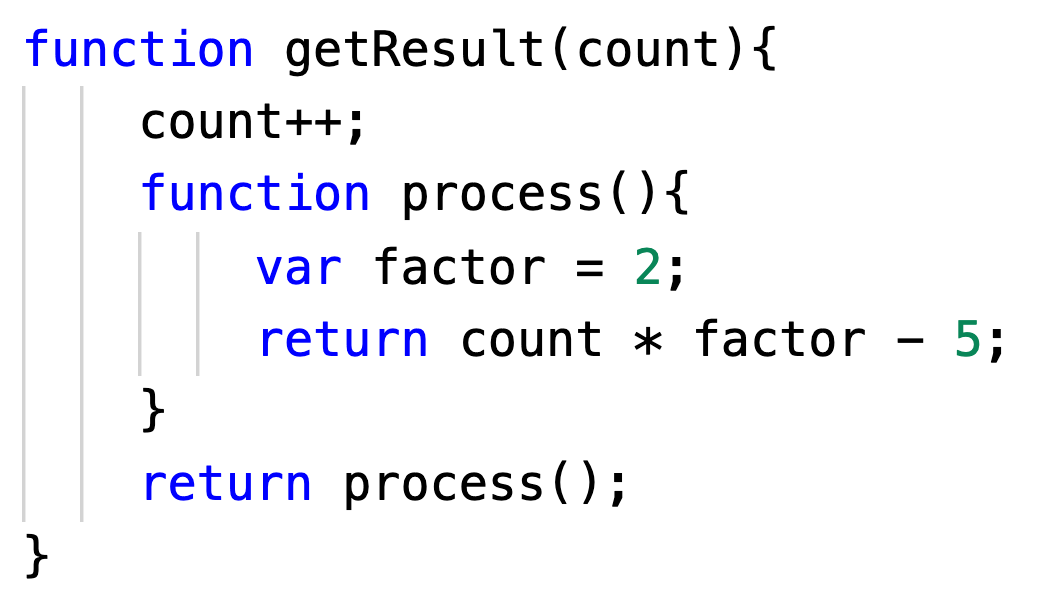
The up-level scoped variable count
is used inside the process
function, which makes it more time-consuming for the JavaScript engine to find the count
variable when the process
function is called.
上级范围变量count
用于process
函数内部,这使JavaScript引擎在调用process
函数时查找count
变量更加耗时。
At the same time, if the scope is nested multiple levels and there are dozens of lines of code between process
and count
, it is easy to confuse the count variable when reading the process function.
同时,如果范围嵌套了多个级别,并且process
和count
之间有数十行代码,则在读取流程函数时很容易混淆count变量。
A better approach is to pass count
as a parameter to process
.
更好的方法是将count
作为参数传递给process
。
Good code:
好代码:
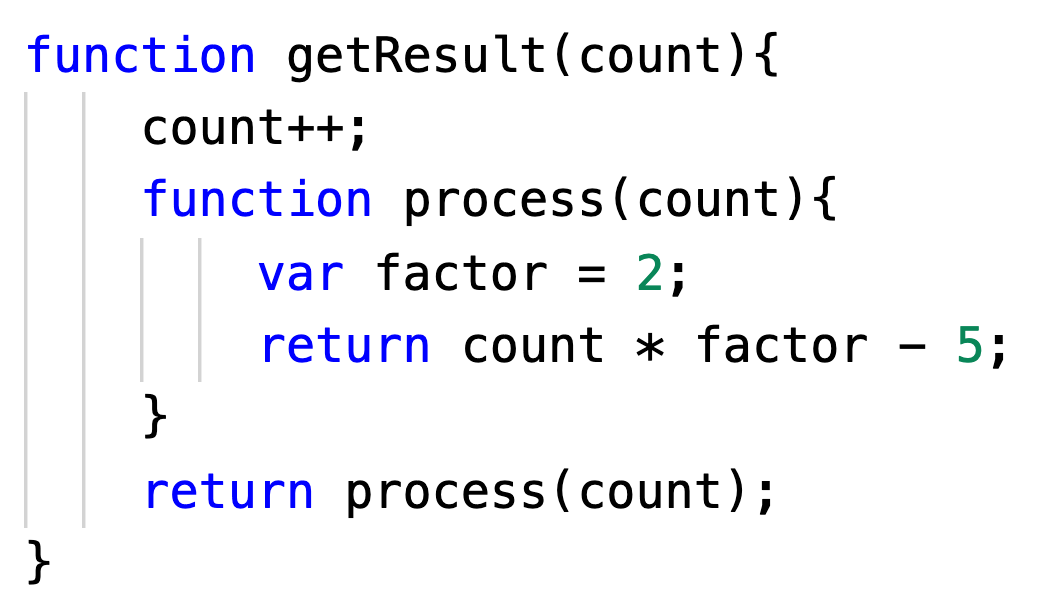
3.使用ES6功能简化代码 (3. Use ES6 features to simplify the code)
ES6 has been around for a number of years and is now very compatible. We should actively embrace ES6 to make the code more concise and elegant.
ES6已经存在很多年了,现在已经非常兼容。 我们应该积极拥抱ES6,以使代码更加简洁和优雅。
使用箭头函数代替普通函数作为回调函数 (Use arrow functions instead of normal functions as the callback function)
If you don’t need to consider about this
binding, it’s a good idea to use arrow functions instead of normal functions as callbacks.
如果您不需要考虑this
绑定,则最好使用箭头函数而不是普通函数作为回调。
Bad code:
错误代码:
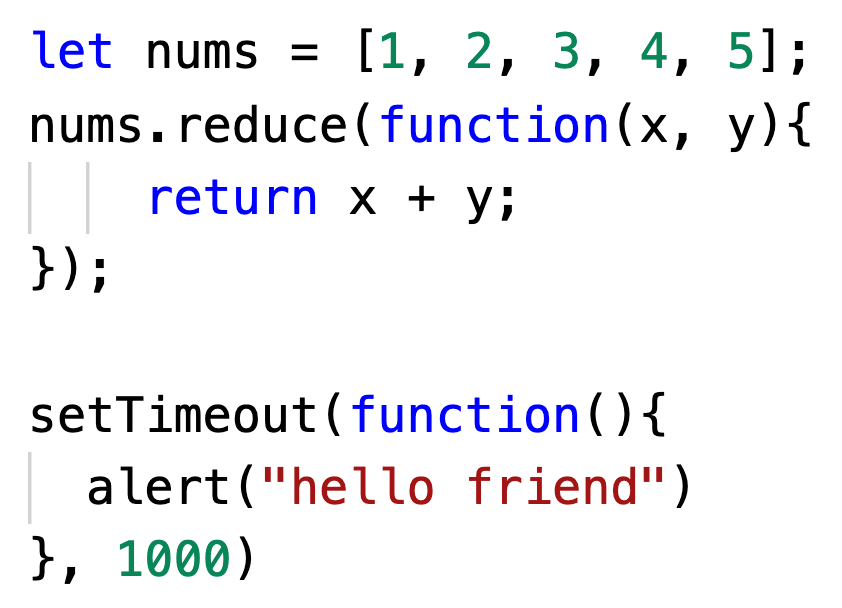
Good code:
好代码:
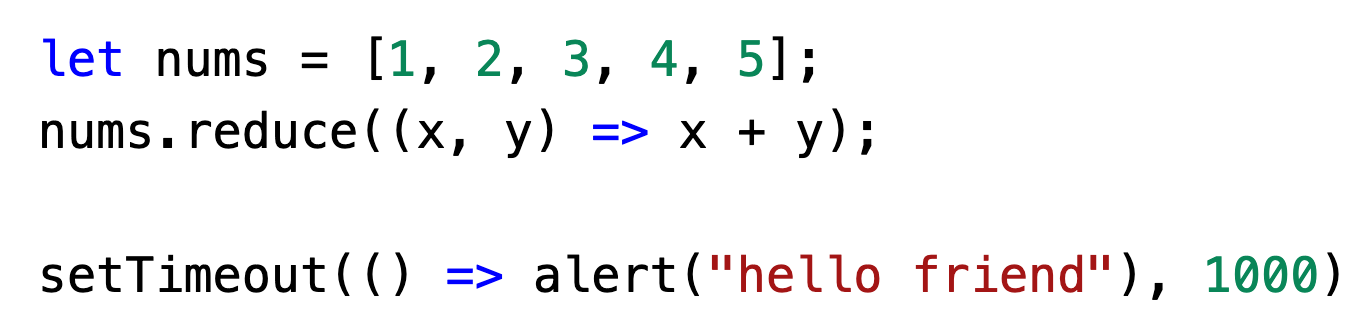
使用类别 (Use class)
Using the traditional prototype syntax will separate the constructor code from the prototype method code, making it impossible to organize the code effectively.
使用传统的原型语法会将构造函数代码与原型方法代码分开,从而无法有效地组织代码。
Bad code:
错误代码:
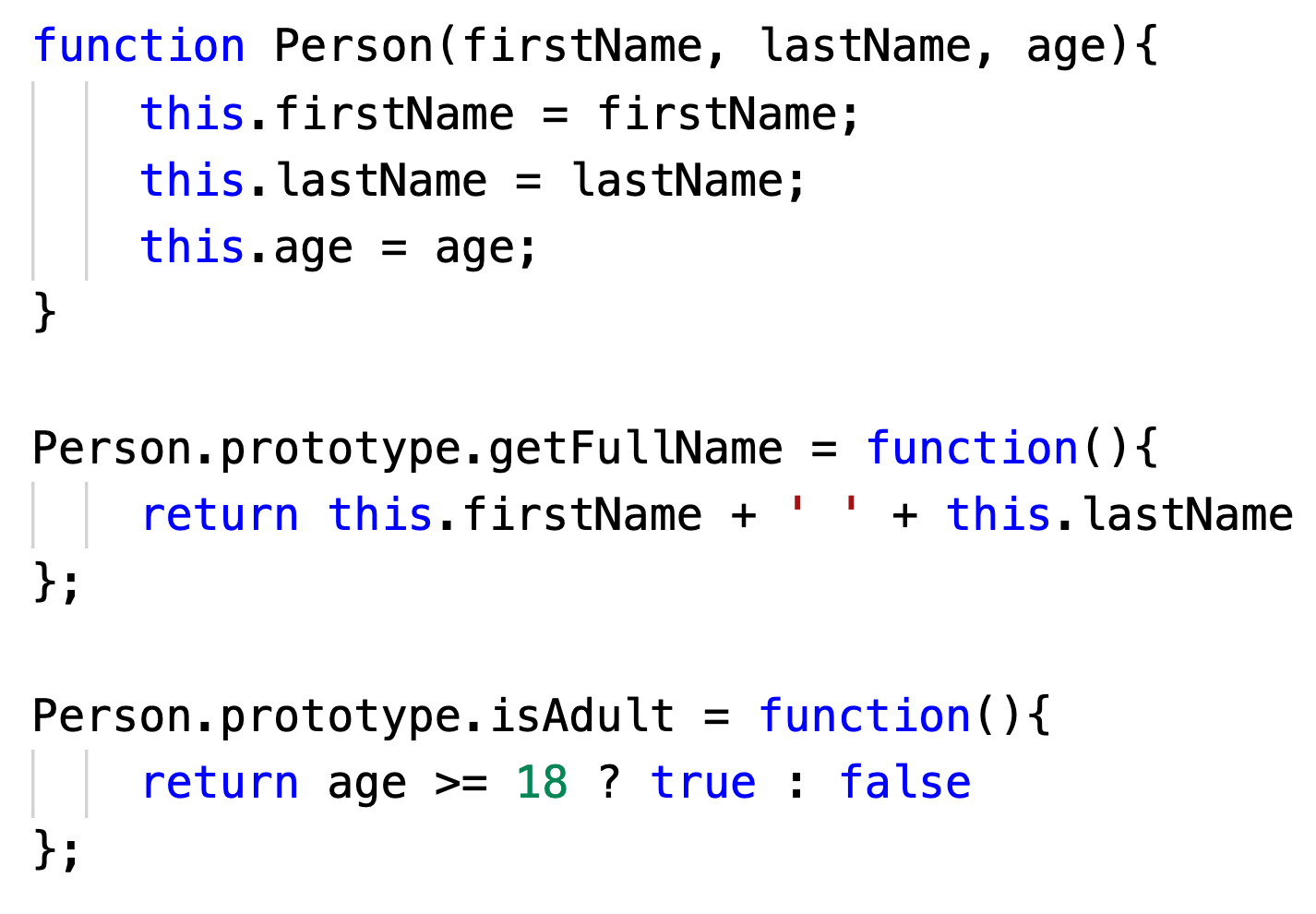
Using class makes the code much simpler and easier to understand, while using class also makes it easy to implement inherited, static member functions.
使用类使代码更加简单易懂,而使用类也使实现继承的静态成员函数变得容易。
Good code:
好代码:
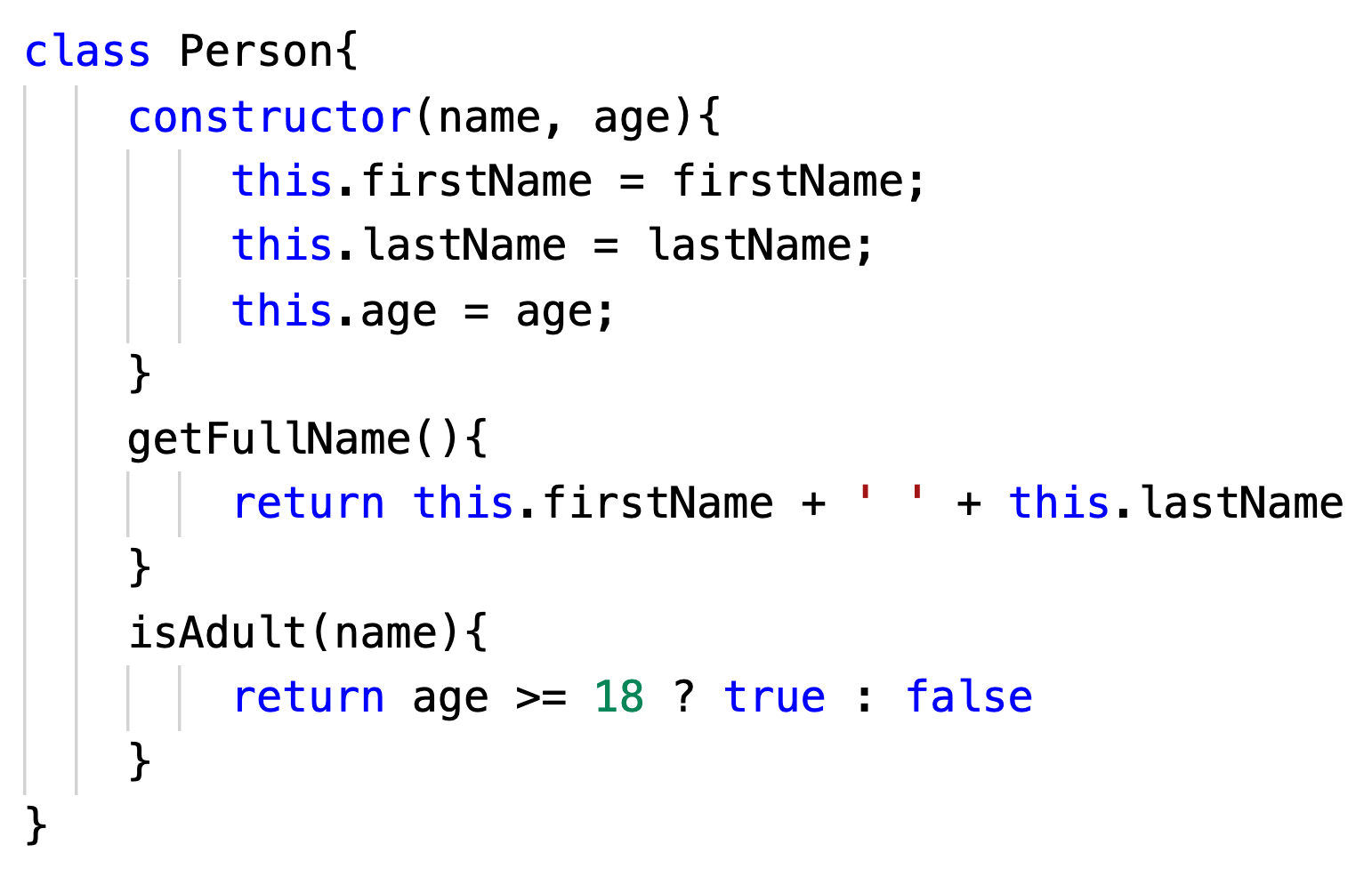
使用模板字符串 (Use template strings)
Template strings are enclosed by the backtick (` `
) character instead of double or single quotes.
模板字符串用反引号( ` `
)括起来,而不用双引号或单引号引起来。
Bad code:
错误代码:
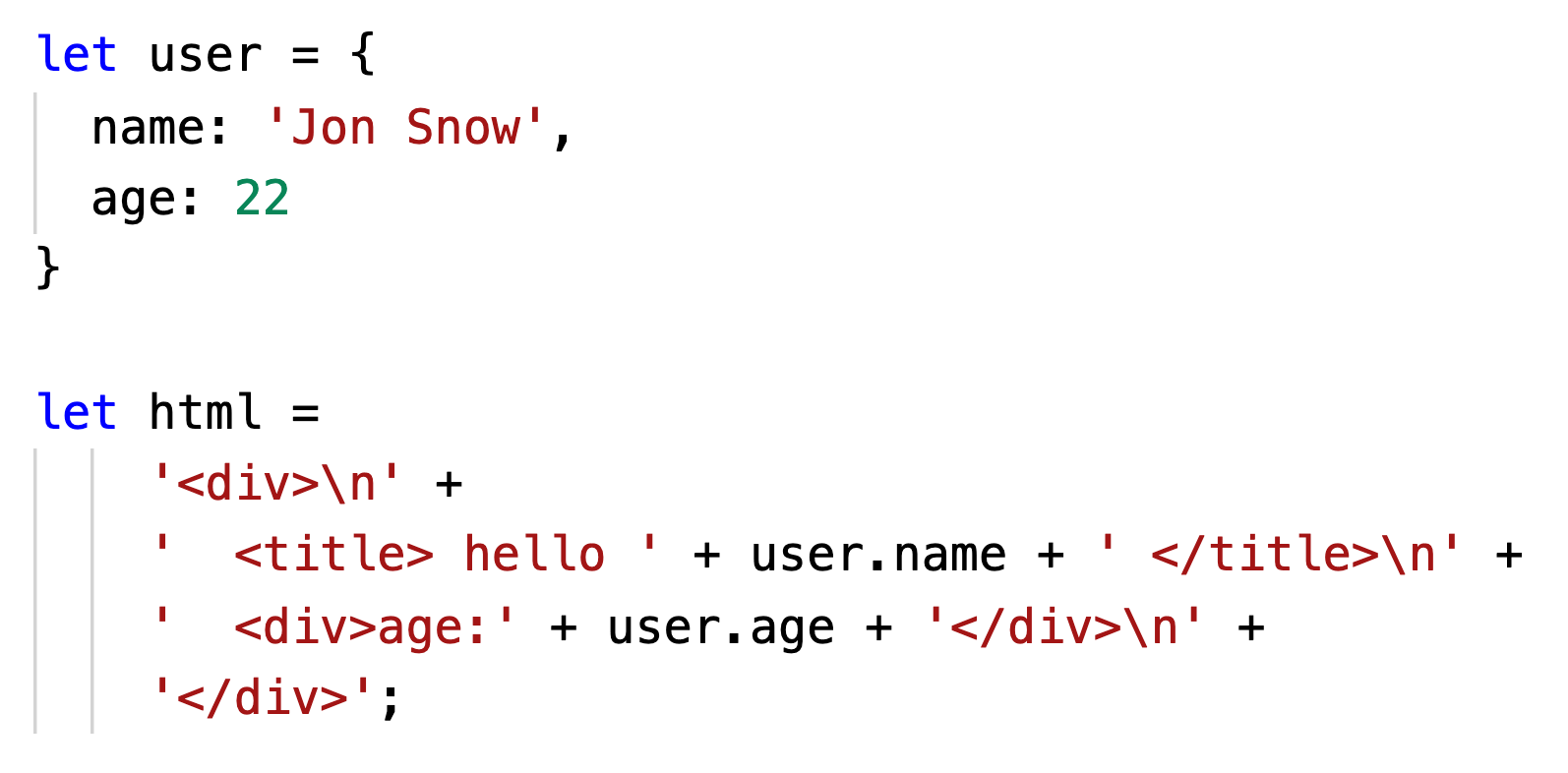
In template strings, we can use any characters instead of escape characters like \n
.
在模板字符串中,我们可以使用任何字符代替\n
等转义字符。
At the same time, we can directly use the expression ${}
to insert variables, instead of splitting strings and using +
to concatenation.
同时,我们可以直接使用表达式${}
插入变量,而不是拆分字符串并使用+
进行串联。
Good code:
好代码:
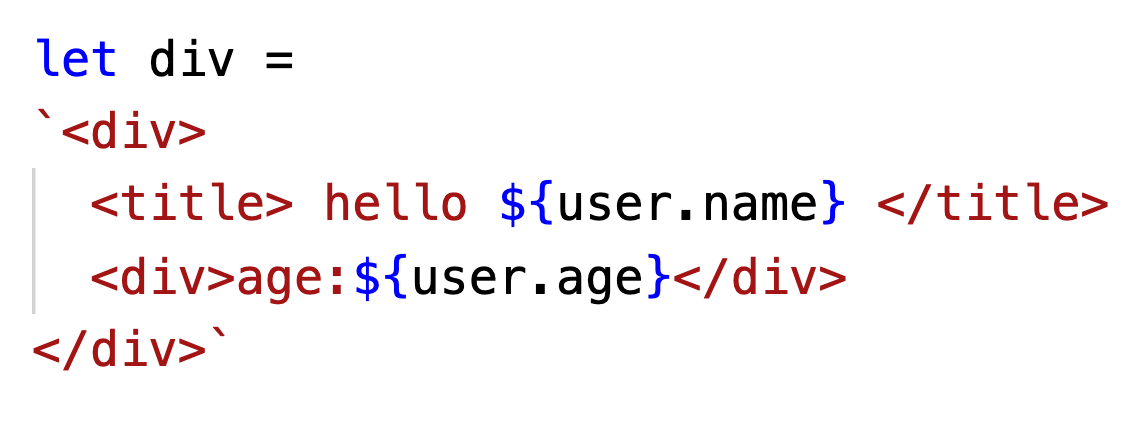
This is obviously easier to read.
这显然更容易阅读。
使用默认参数 (Use default parameters)
In ES5, if we wanted to give a default value to the function’s arguments, we might write something like this:
在ES5中,如果我们想给函数的参数赋一个默认值,我们可以这样写:
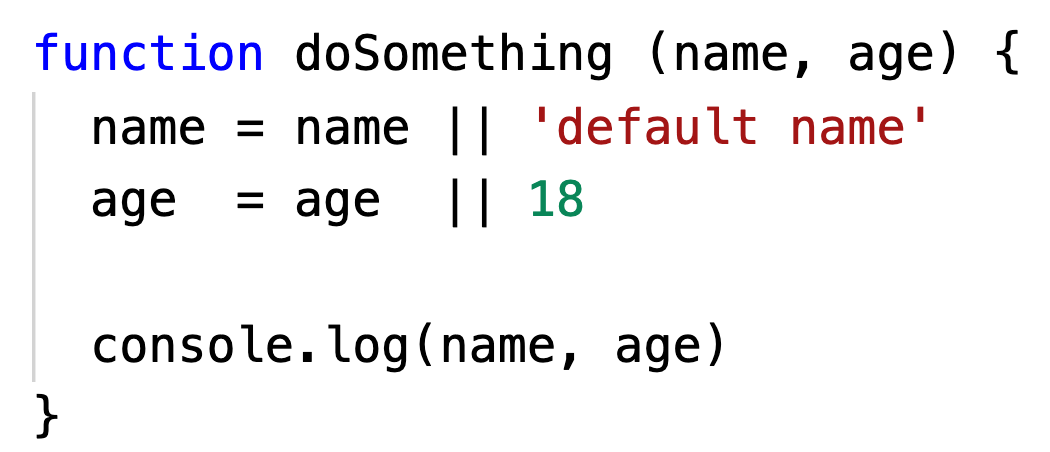
In ES6, we can write in a simpler, more readable way:
在ES6中,我们可以用更简单,更易读的方式编写:

使用块范围变量 (Use block scope variables)
If we want the console to print out 0, 1, 2, …10 at 100 milliseconds intervals, in turn, some people might write code like this:
如果我们想让控制台以100毫秒的间隔打印出0、1、2,…10,那么有些人可能会编写如下代码:
for(var index = 0; index <= 10; index++){
setTimeout(() => console.log(index), 100)
}
Unfortunately, the above code does not fulfill the requirements. Because the variables declared by var
are in the global scope, the value of the index
has been changed to 11 when the setTimeout callback function executes.
不幸的是,以上代码无法满足要求。 由于var
声明的变量在全局范围内,因此在执行setTimeout回调函数时, index
的值已更改为11。
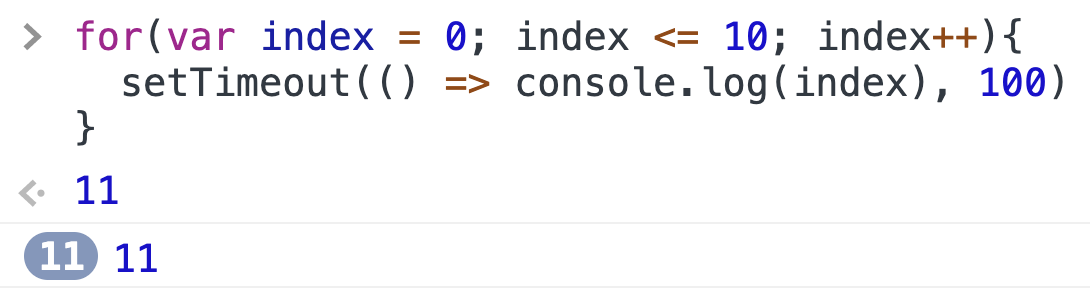
One solution in ES5 is to use closures:
ES5中的一种解决方案是使用闭包:
for(var index = 0; index <= 10; index++){
(function(archivedIndex){
setTimeout(() => console.log(archivedIndex), 100)
})(index)
}
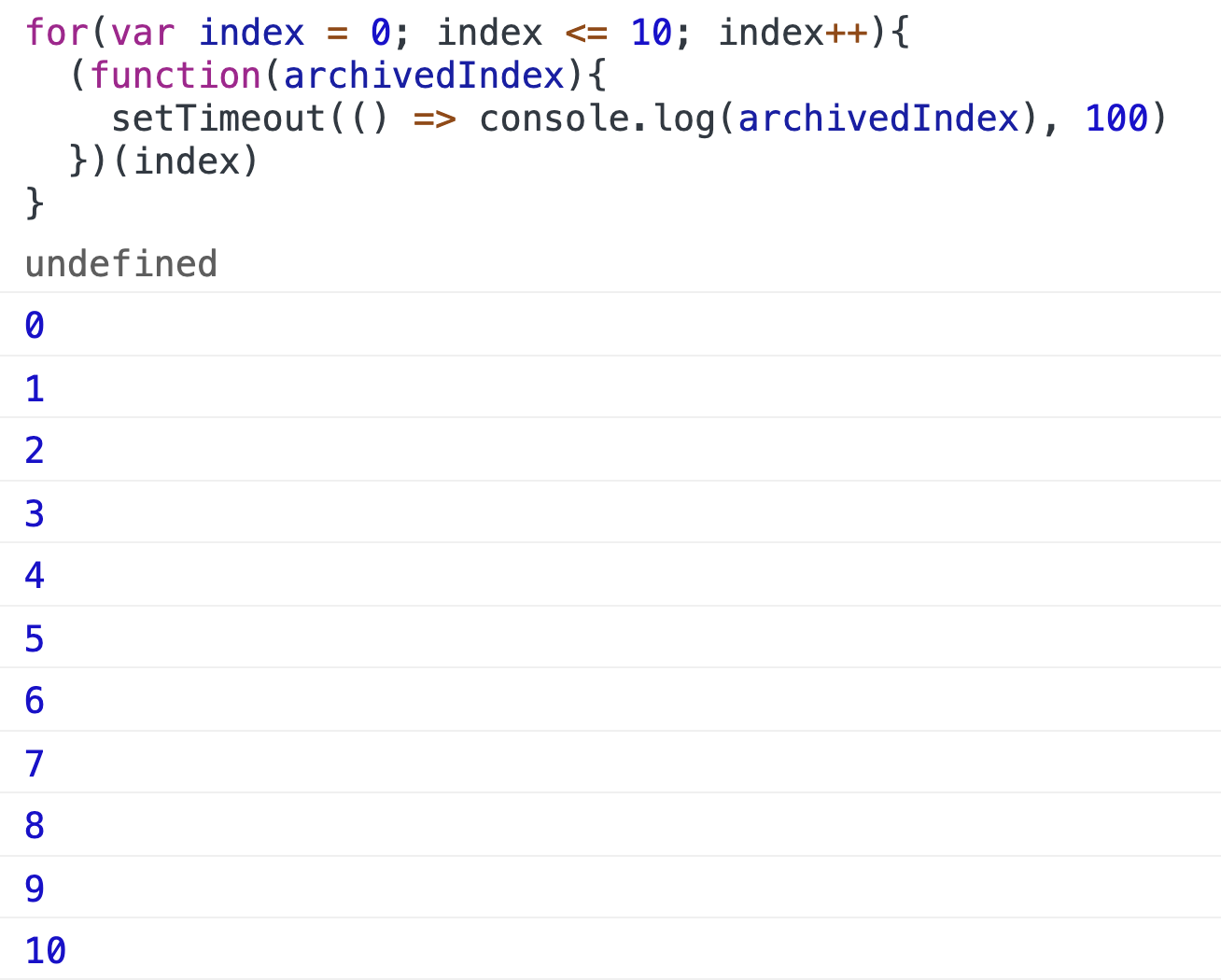
Here we save the index
value through the closure, so that every time the setTimeout is executed, the correct index
value will be found.
在这里,我们通过闭包保存index
值,以便每次执行setTimeout时,都会找到正确的index
值。
But the above writing is very troublesome and difficult to understand. A better way is to use let
to declare a block-scoped variable.
但是上面的写作很麻烦而且很难理解。 更好的方法是使用let
声明一个块作用域变量。
for(let index = 0; index <= 10; index++){
setTimeout(() => console.log(index), 100)
}
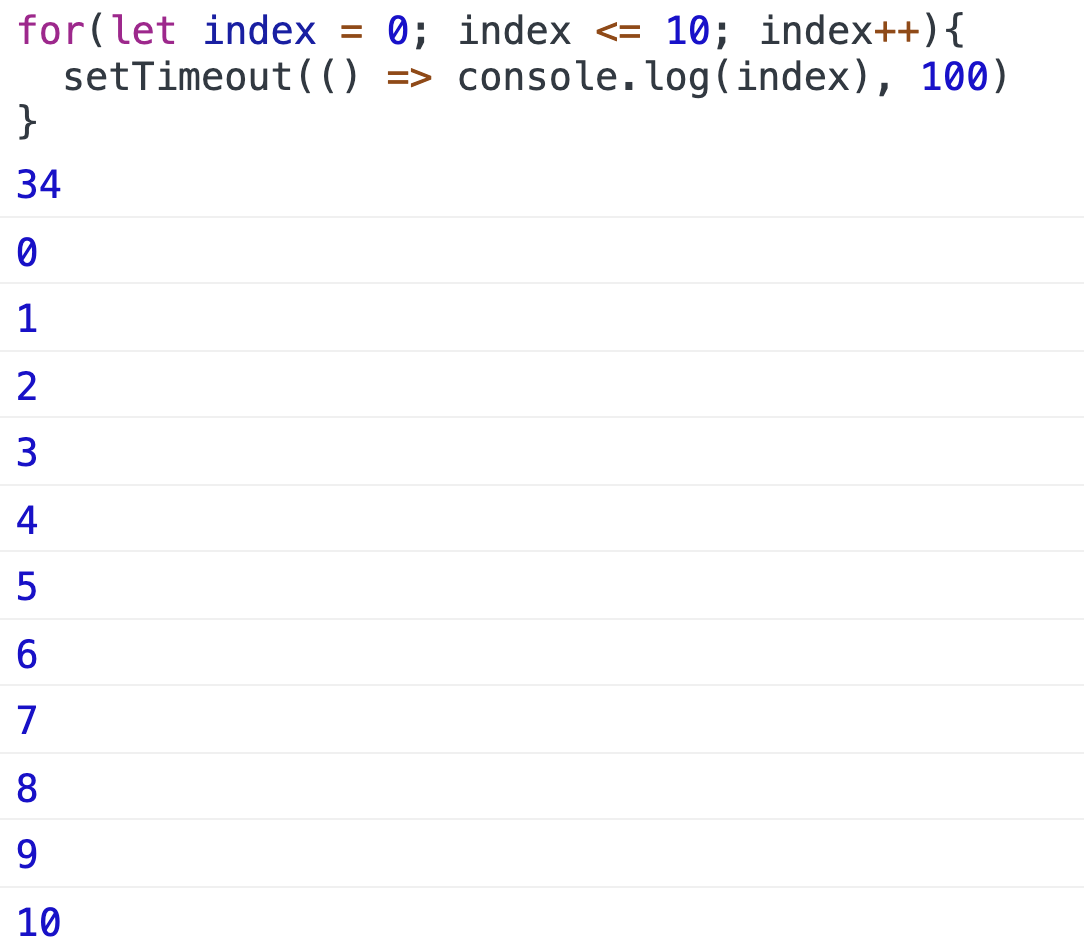
You only need to change three characters to complete the requirements, which are very simple, convenient, and readable.
您只需更改三个字符即可完成要求,它们非常简单,方便且可读。
4.语法风格 (4. Grammar style)
用三元运算符替换简单的if-else(Replace the simple if-else with the ternary operator)
In general, the syntax of the ternary operator is as follows:
通常,三元运算符的语法如下:
condition ? expression_1 : expression_2;
The condition
is an expression that evaluates to a Boolean value, either true
or false
. If the condition is true
, the ternary operator returns expression_1
, otherwise, it returns the expression_2
.
condition
是一个表达式,其结果为布尔值true
或false
。 如果条件为true
,则三元运算符返回expression_1
,否则返回expression_2
。
Bad Code:
错误代码:
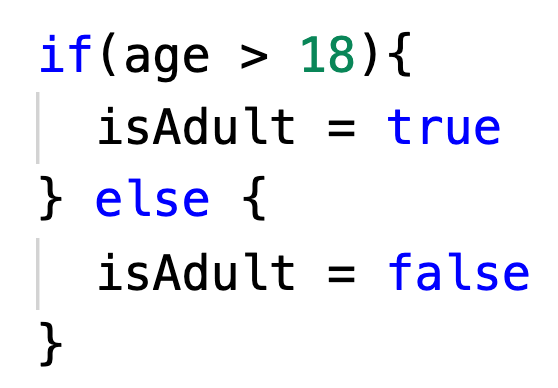
Good code:
好代码:

避免== (Avoid ==)
==
has many special mechanisms, too much use of ==
will make our code more difficult to understand. We can convert data types explicitly to make the code easier to understand.
==
有很多特殊的机制,太多的使用==
会使我们的代码更难以理解。 我们可以显式转换数据类型,以使代码更易于理解。
Bad code:
错误代码:
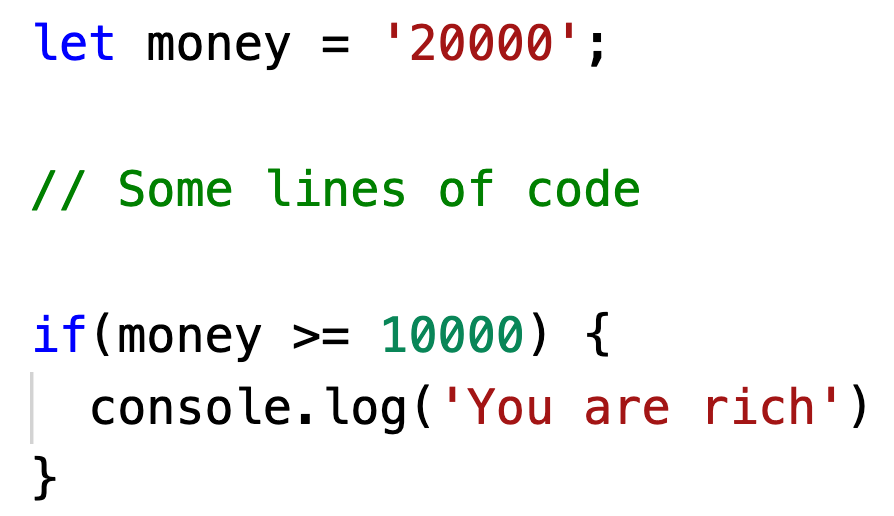
Good code:
好代码:
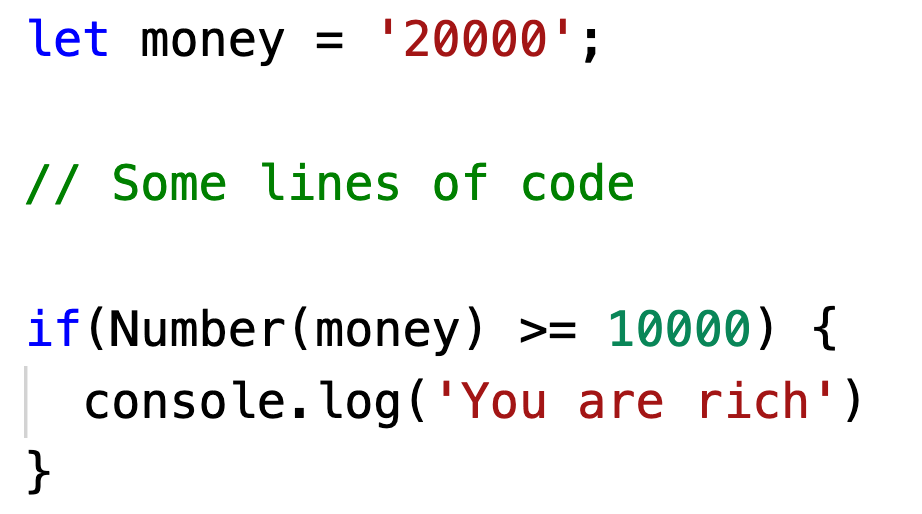
javascript编写