列表合并 python
清单: (List:)
Python knows a number of compound data types, used to group together other values. The most versatile is the list, which can be written as a list of comma-separated values (items) between square brackets. Lists might contain items of different types, but usually the items all have the same type.
Python知道许多复合数据类型,用于将其他值组合在一起。 最通用的是list ,可以将其写成方括号之间用逗号分隔的值(项目)的列表。 列表可能包含不同类型的项目,但通常所有项目都具有相同的类型。
合并列表的不同方法: (Different ways to merge list:)

append
附加
- The append method will add an item to the end of the list. append方法会将一个项目添加到列表的末尾。
- The length of the list will be increased by 1. 列表的长度将增加1。
- It will update the original list itself. 它将更新原始列表本身。
Return type is
None
.返回类型为
None
。
l1=[1,2,3]
l2=[4,5,6]
l1.append(l2)
print (l1)#Output:[1, 2, 3, [4, 5, 6]]
2. extend
2.扩展
- The extend method will extend the list by appending all the items from the iterable. expand方法将通过附加来自iterable的所有项来扩展列表。
- The length of the list will be increased depends on the length of the iterable. 列表的长度将取决于迭代对象的长度。
- It will update the original list itself. 它将更新原始列表本身。
Return type is
None.
返回类型为
None.
l1=[1,2,3]
l2=[4,5,6]
l1.extend(l2)
print (l1)#Output:[1, 2, 3, 4, 5, 6]
3. Concatenation
3.串联
- The list also supports concatenation operations. 该列表还支持串联操作。
We can add two or more lists using
+
operator.我们可以使用
+
运算符添加两个或多个列表。- It won’t update the original list. 它不会更新原始列表。
Return type is a new
list object
.返回类型是一个新的
list object
。
Example 1:Concatenating two lists
示例1:连接两个列表
l1=[1,2,3]
l2=[4,5,6]
l3=l1+l2
print (l3)#Output:[1, 2, 3, 4, 5, 6]
Example 2:Concatenating two or more lists
示例2:连接两个或更多列表
l1=[1,2,3]
l2=[4,5,6]
l3=[7,8]
l4=[9,10]
l5=l1+l2+l3+l4
print (l5)#Output:[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
4. UnpackingAn asterisk *
denotes iterable unpacking. Its operand must be iterable. The iterable is expanded into a sequence of items, which are included in the new tuple, list, or set, at the site of the unpacking.list1=[*list2,*list3]
First, it will unpack the contents and then create a list from its contents.
4.开箱星号*
表示可重复开箱 。 它的操作数必须是可迭代的。 可迭代的对象被扩展为一系列项目,这些项目包括在拆包现场的新元组,列表或集合中。 list1=[*list2,*list3]
首先,它将解压缩内容,然后根据其内容创建一个列表。
l1=[1,2,3]
l2=[4,5,6]
l3=[*l1,*l2]
print (l3)#Output:[1, 2, 3, 4, 5, 6]
5. itertools.chainMakes an iterator that returns an element from the first iterable until its exhausted, then proceeds to the next iterable. It will treat consecutive sequences as a single sequence.itertools.chain(*iterables)
The list is also iterable, so we can use itertools.chain() to merge two dictionaries. The return type will be itertools.chain object. We can convert to a list using the list() constructor.
5. itertools.chain使一个迭代器从第一个可迭代的元素返回直到元素耗尽为止,然后进行到下一个可迭代的元素。 它将连续序列视为单个序列。 itertools.chain(*iterables)
该列表也是可迭代的,因此我们可以使用itertools.chain()合并两个字典。 返回类型将是itertools.chain对象。 我们可以使用list ()构造函数转换为列表。
import itertools
l1=itertools.chain([1,2,3],[4,5,6])#Returns an iterator objectprint (l1)#Output:<itertools.chain object at 0x029FE4D8>
#converting iterator object to list objectprint(list(l1))#Output:[1, 2, 3, 4, 5, 6]
6. List comprehension.A list comprehension consists of brackets[]
containing an expression followed by a for
clause, then zero or more for
or if
clauses. The result will be a new list resulting from evaluating the expression in the context of the for
and if
clauses that follow it.[expression for item in iterable if conditional]
6.列表理解。 列表推导由方括号[]
组成,方括号[]
包含一个表达式,后跟一个for
子句,然后是零个或多个for
或if
子句。 结果将是一个新列表,该列表是通过在表达式后面的for
和if
子句的上下文中评估表达式而得出的。 [expression for item in iterable if conditional]
Example 1.Joining two lists using list comprehension
例1.使用列表推导连接两个列表
l1=[1,2,3]
l2=[4,5,6]
l3=[x for n in (l1,l2) for x in n]
print (l3)#Output:[1, 2, 3, 4, 5, 6]
7. for loop
7. for循环
- First for loop to go through the lists. (for n in (l1,l2) 首先是要遍历列表的for循环。 (对于(l1,l2)中的n
- Second for loop to go through the elements in the list. (for x in n) 第二个for循环遍历列表中的元素。 (对于x in n)
Then it will append each element to the empty list created before
l3
然后它将每个元素附加到
l3
之前创建的空列表中
l1=[1,2,3]
l2=[4,5,6]
l3=[]
for n in (l1,l2):
for x in n:
l3.append(x)
print (l3)
#Output:[1, 2, 3, 4, 5, 6]
How to join all strings in a list:str.join(iterable)
如何加入列表中的所有字符串: str. join ( iterable )
str. join ( iterable )
Return a string which is the concatenation of the strings in iterable. A
TypeError
will be raised if there are any non-string values in iterable, includingbytes
objects. The separator between elements is the string providing this method.返回一个字符串,该字符串是iterable中字符串的串联。 如果iterable中有任何非字符串值(包括
bytes
对象),则会引发TypeError
。 元素之间的分隔符是提供此方法的字符串。
Example 1: Joining all strings in the list. The separator is given as space.
示例1:连接列表中的所有字符串。 分隔符以空格形式给出。
l1=["Welcome", "to", "python", "programming", "language"]
l2=" ".join(l1)
print (l2)#Output:Welcome to python programming language
Example 2: Joining all strings in the list. The separator is given as -
示例2:连接列表中的所有字符串。 分隔符为-
l1=["1","2","3"]
l2="-".join(l1)
print (l2)#Output:1-2-3
How to remove duplicate elements while merging two lists.Python sets don’t contain duplicate elements.To remove duplicate elements from lists we can convert the list to set using set() and then convert back to list using list() constructor.
合并两个列表时如何删除重复的元素。 Python集不包含重复元素。要从列表中删除重复元素,我们可以使用set()将列表转换为set,然后使用list()构造函数转换回list。
l1=[1,2,3,4,5]
l2=[1,3,5,7,9]
l3=list(set(l1+l2))
print (l3)#Output:[1, 2, 3, 4, 5, 7, 9]
The fastest way to merge lists in python.
在python中合并列表的最快方法。
time.time() → float
time. time () → float
Return the time in seconds since the epoch as a floating-point number. The epoch is the point where the time starts and is platform dependent. The specific date of the epoch and the handling of leap seconds is platform dependent.
以纪元为单位返回自纪元以来的时间(以秒为单位)。 纪元是时间开始的时间点,并且取决于平台。 时期的具体日期和and秒的处理方式取决于平台。
Calculating the time taken by the append method
计算append方法花费的时间
import time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start=time.time()
l1.append(l2)
print (time.time()-start)#Output:0.0019817352294921875
Calculating the time taken to merge lists using the extend method.
使用扩展方法计算合并列表所需的时间 。
import time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start1=time.time()
l1.extend(l2)
print (time.time()-start1)#Output:0.00701141357421875
Calculating the time taken to join lists using concatenation operator.
使用串联运算符计算加入列表所需的时间。
import time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start2=time.time()
l1+l2
print (time.time()-start2)#Output:0.014112710952758789
Calculating the time taken to merge the lists using unpacking method
使用拆包方法计算合并列表所需的时间
import time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start3=time.time()
[*l1,*l2]
print (time.time()-start3)#Output:0.020873069763183594
Calculate the time taken to merge lists using itertools.chain()
使用itertools.chain()计算合并列表所需的时间
import itertoolsimport time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start4=time.time()
l3=itertools.chain(l1,l2)
list(l3)
print (time.time()-start4)#Output:0.045874595642089844
Calculate the time taken to merge lists using list comprehension.
计算使用列表理解合并列表所花费的时间。
import time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start5=time.time()
l3=[x for n in (l1,l2) for x in n]
print (time.time()-start5)#Output:0.06680965423583984
Calculate the time taken to merge lists using for loop.
使用for循环计算合并列表所需的时间。
import time
l1=list(range(1,1000000))
l2=list(range(1000000,2000000))
start5=time.time()
l3=[]for n in (l1,l2):
for x in n:
l3.append(x)
print (time.time()-start5)#Output:0.23975229263305664
Let’s compare the time taken by all methods to merge the lists.
让我们比较一下所有方法合并列表所花费的时间。
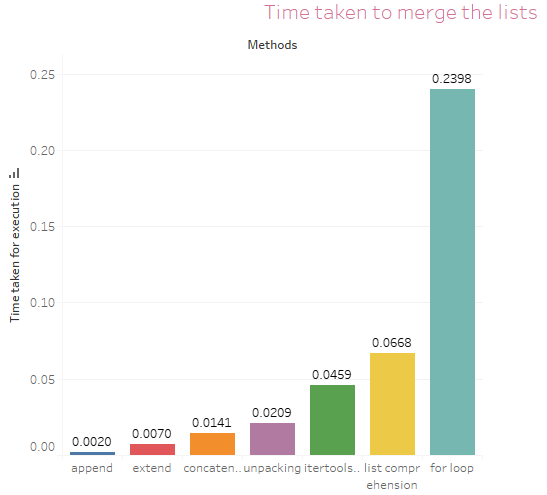
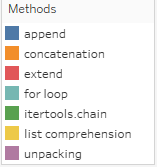
结论: (Conclusion:)
append method will add the list as one element to another list. The length of the list will be increased by one only after appending one list.
append方法会将列表作为一个元素添加到另一列表。 仅在追加一个列表后,列表的长度才增加一。
The extend method will extend the list by appending all the items from iterable(another list). The length of the list will be increased depends on the length of the iterable.
扩展方法将通过附加来自iterable(另一个列表)的所有项来扩展列表。 列表的长度将取决于迭代对象的长度。
Both the append and extend method will modify the original list.
append和extend方法都将修改原始列表 。
Concatenation, unpacking, list comprehension returns a new list object. It won’t modify the original list
串联,拆包,列表理解将返回一个新的列表对象。 它不会修改原始列表
itertools.chain()-Return type will be itertools.chain object. We can convert to a list using the list() constructor.
itertools.chain( )-返回类型将是itertools.chain对象 。 我们可以使用list ()构造函数转换为列表。
翻译自: https://medium.com/analytics-vidhya/merging-lists-in-python-4a386e4b2f21
列表合并 python