dart编程语言
Dart is primarily known as the programming language for Flutter, Google’s UI toolkit for building natively compiled mobile, web, and desktop apps from a single codebase. It’s optimized for building user-interfaces and developed by Google. It’s used to build mobile, desktop, server, and web applications. Dart can compile to native code and JavaScript.
Dart主要被称为Flutter的编程语言,Flutter是Google的UI工具包,用于从单个代码库构建本机编译的移动,Web和桌面应用程序。 由Google开发并针对构建用户界面进行了优化。 它用于构建移动,桌面,服务器和Web应用程序。 Dart可以编译为本机代码和JavaScript。
If you want to follow along with this article, which I highly recommend, you can do so without installing anything on DartPad.dev.
如果您要遵循我强烈建议的这篇文章,则无需在DartPad.dev上安装任何内容即可 。
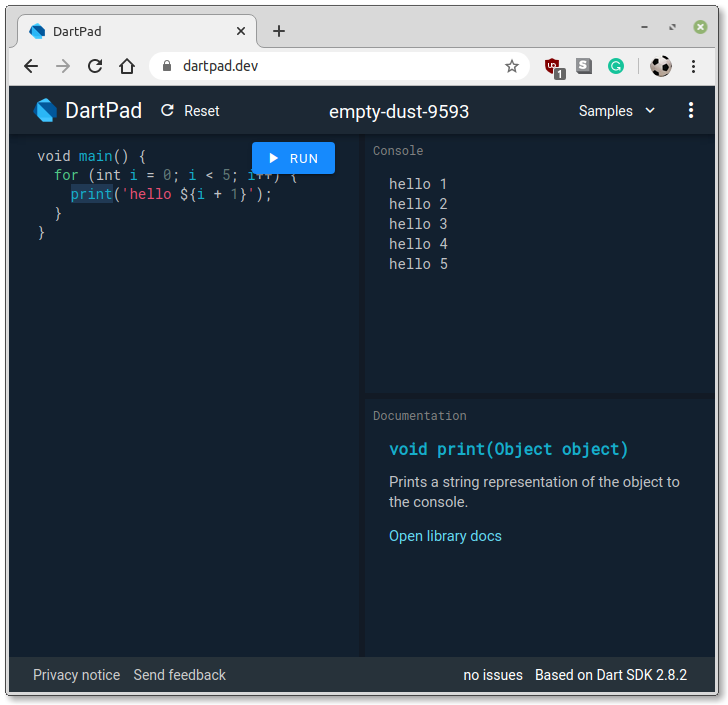
你好,世界 (Hello World)
Let’s start with the obligatory “Hello world”:
让我们从强制性的“ Hello world”开始:
void main() {
print('Hello World!');
}
A few conclusions that can already be drawn:
可以得出一些结论:
Dart needs a
main()
function as a starting pointDart需要
main()
函数作为起点- Dart doesn’t require a class (Like C, unlike Java) Dart不需要类(就像C,与Java不同)
- Dart uses two-character indentation by convention Dart按照约定使用两个字符的缩进
Two character indentation makes the code more compact. This is really helpful when you start creating a Flutter application since Flutter apps are based on a tree of nested widgets, which can get out of soon.
两个字符的缩进使代码更紧凑。 当您开始创建Flutter应用程序时,这非常有帮助,因为Flutter应用程序基于嵌套的小部件树,这些小部件可能很快就会消失。
Dart代码紧凑 (Dart code is compact)
class Person {
String name;
int age;Person(this.name, this.age);
int lieAboutMyAge() {
print("My name is $name, my age is ${age - 10}");
}
}void main() {final me = Person("Erik", 38);
var girlfriend = Person("Shannon", 30);
me.lieAboutMyAge();
}
Dart code has many modern features to reduce code size. As you can see from the above example:
Dart代码具有许多现代功能,可以减少代码大小。 从上面的示例可以看出:
- You can simply refer to instance variables in a bodiless constructor to automatically assign values to them 您只需在无体构造器中引用实例变量即可自动为其分配值
Strings have template-like features, allowing you to directly use variables or even expressions like
${age - 10}
字符串具有类似模板的功能,使您可以直接使用变量甚至
${age - 10}
类的表达式When creating objects, you don’t need to use the
new
keyword, but if you want, you can创建对象时,不需要使用
new
关键字,但是如果需要,可以Dart supports both top-level functions like
main()
and class methods.Dart同时支持诸如
main()
和类方法之类的顶级功能。
类型推断 (Type Inference)
Dart is strongly typed. But did you notice that Dart uses type inference? We didn’t need to specify the type (Person
) of the variables me
and girlfriend
. Instead, Dart inferred the type for us at compile time. This is in line with other modern languages like Kotlin.
Dart是强类型的。 但是您是否注意到Dart使用类型推断? 我们不需要指定me
和girlfriend
变量的类型( Person
)。 相反,Dart在编译时为我们推断了类型。 这与其他现代语言(例如Kotlin)一致。
总决赛 (Final and Const)
If you are sure a variable won’t change, you can declare it final
. If you’re not sure — perhaps you’ll find another girlfriend next week — you use var
.
如果您确定变量不会更改,则可以将其声明为final
。 如果不确定(也许下周会找到另一个女朋友),请使用var
。
There’s also a const
modifier, which is a bit more difficult to grasp and has effect on the value.
还有一个const
修饰符,该修饰符较难掌握,并且会影响该值。
A final variable can be assigned after compile time, like storing the response of an HTTP request, while a const must be known and defined before compilation.
可以在编译后分配最终变量,例如存储HTTP请求的响应,而在编译之前必须知道并定义const。
If a collection or object is a const, it’s complete state is immutable at compile-time, hence it must be created from data that is available at compile time. In contrast, if a variable is final, you can’t reassign a new object to it, but you can still change the collection or object that it points to.
如果集合或对象是const,则它的完整状态在编译时是不变的,因此必须从编译时可用的数据中创建它。 相反,如果变量是final,则不能将新对象重新分配给它,但仍可以更改其指向的集合或对象。
对象 (Objects)
Everything that can be assigned to a variable, is an object. Even numbers, functions, and null
are objects. All objects are based on a class, and all objects inherit from a base object with the unsurprising name Object.
可以分配给变量的所有对象都是对象。 偶数,函数和null
都是对象。 所有对象都基于一类,并从与不足为奇名称的基本对象继承的所有对象的对象 。
I like languages that do this because it allows numbers to have convenience methods like isNegative
and isOdd
, resulting in pleasant code that almost reads like a good novel:
我喜欢这样做的语言,因为它允许数字具有方便的方法,例如isNegative
和isOdd
,从而产生令人愉悦的代码,读起来就像一本好小说:
if (age.isNegative) {
print("The age can not be negative, you fool!")
}
Speaking about numbers, Dart make life easier by only offering two types of numbers: integers and doubles (both 64 bits and signed).
说到数字,Dart仅提供两种类型的数字,使生活更轻松:整数和双精度数(64位和带符号)。
私人和公共 (Private and public)
All identifiers are public by default. Dart doesn’t have keywords for public, private, or protected.
默认情况下,所有标识符都是公共的。 Dart没有公共,私有或受保护的关键字。
A library in Dart is everything that is in a single file. So library privacy means that the identifier is visible only inside the file that the identifier is defined in. To mark a Dart identifier private to its library, start its name with an underscore.
Dart中的库是单个文件中的所有内容。 因此,库保密性意味着该标识符仅在定义该标识符的文件内部可见。要标记Dart标识符为其库私有,请使用下划线开头其名称。
表达式和陈述 (Expressions and statements)
Dart has both expressions that result in a value and statements like if… else
that don’t. This doesn’t differ from most other languages. Some of the usual suspects that are available in Dart too:
Dart既有产生值的表达式,又有if… else
语句if… else
就没有。 这与大多数其他语言没有什么不同。 Dart也提供一些常见的嫌疑人:
- while loops and for loops while循环和for循环
- if…else statements 如果…其他陈述
- The switch statement 切换语句
The construct
condition ? [expression if true] : [expression if false]
构造
condition ? [expression if true] : [expression if false]
condition ? [expression if true] : [expression if false]
- Exceptions 例外情况
列表,集合和地图 (Lists, Sets, and Maps)
Dart supports these data types natively. Let’s look at some examples to get a feel for these data types, starting with lists:
Dart本机支持这些数据类型。 让我们看一些示例,从列表开始了解这些数据类型:
var myList = [1, 2, 3];
assert(myList.length == 3);
assert(myList[1] == 2);
var constantList = const [1, 2, 3];
constantList[1] = 1; // This causes an error!
Sets are unordered collections of unique items:
集合是唯一项的无序集合:
var mySet = {'john', 'eric', 'martha'};
var elements = <String>{};
elements.add('fluorine');
Something that will bite you sometime: if you forget the type annotation on a set definition like the above one for elements
, a map will be created. This is simply because maps also use accolades, and maps appeared first in the Dart language.
某个时候会刺痛您的事情:如果您忘记了像上面的elements
定义这样的集合定义上的类型注释,则会创建一个映射。 这仅仅是因为地图也使用赞誉,并且地图首先以Dart语言出现。
A map associates keys and values that can be any type. The following map is inferred to the type Map<String, String>
:
映射关联可以为任何类型的键和值。 以下映射被推断为Map<String, String>
:
var gifts = {
'first': 'partridge',
'second': 'turtledoves',
'fifth': 'golden rings'
};gifts['fourth'] = 'calling birds';
Dart套件管理员 (Dart Package Manager)
The website https://pub.dev/ contains publicly available packages that can be installed easily with Dart’s package manager, pub. The website, as of writing this, contains more than twelve thousand packages, some for Dart and many specifically for Flutter.
网站https://pub.dev/包含公共可用的软件包,可以使用Dart的软件包管理器pub轻松安装。 在撰写本文时,该网站包含一万二千个软件包,其中一些用于Dart,许多专门用于Flutter。
You can also use put to install locally downloaded packages or packages hosted on GitHub.
您还可以使用put安装本地下载的软件包或托管在GitHub上的软件包。
结论 (Conclusion)
Dart is a modern, easy to learn language. If you want to dive in deeper, I recommend one or more of the following sites:
Dart是一种现代且易于学习的语言。 如果您想更深入地潜水,建议您选择以下一个或多个地点:
翻译自: https://medium.com/tech-explained/the-dart-programming-language-in-five-minutes-12990caccca1
dart编程语言