deno使用rust
Last time we introduced about Deno and discussed how it compares to node, like node, Deno is a server side code-execution environment based on web technology.
上一次我们介绍Deno并讨论了它如何与节点(例如节点)进行比较, Deno是基于Web技术的服务器端代码执行环境。
- Node uses JavaScript with commonjs modules and npm/yarn as it’s package manager. Node将JavaScript与commonjs模块以及npm / yarn一起用作包管理器。
- Deno uses Typescript or JavaScript with modern javascript import statements. It does not need a package manager. Deno在现代javascript导入语句中使用Typescript或JavaScript。 它不需要包管理器。
To import a module as usual in deno you reference it by URL:
要像通常一样导入deno中的模块,请通过URL引用它:
import { serve } from "https://deno.land/std/http/server.ts";
You can find many of the modules you may need in the Deno standard library or in the Deno third party modules list but they don’t have everything.
您可以在Deno标准库或Deno第三方模块列表中找到许多您可能需要的模块,但它们没有全部内容。
Sometimes you need to use a module which the maintainers have only made available through the npm ecosystem. Here are some methods from most convenient to least:
有时您需要使用维护者只能通过npm生态系统提供的模块。 以下是从最方便到最不方便的一些方法:
1.如果模块已经使用ES模块的导入/导出语法。 (1. If the module already uses ES modules import/export syntax.)
The libraries you use from deno don’t have to come from the recommended Deno packages they can come from any URL, provided they use the modern import
syntax. Using unpkg is a great way to access these files directly from inside an npm repo.
您从deno中使用的库不必来自推荐的Deno包,它们可以来自任何URL,只要它们使用现代的import
语法即可。 使用unpkg是直接从npm存储库内部访问这些文件的好方法。
import throttle from https://unpkg.com/lodash@4.17.19/throttle.js
2.如果模块本身不使用导入但源代码使用 (2. If the module itself doesn’t use imports but the source code does)
If the module is compiled or in the wrong format though npm you may still have some luck if you take a look at the source code. Many popular libraries have moved away from using commonjs in their source code to the standards compliant es module import
syntax.
如果通过npm编译模块或使用错误格式的模块,那么查看源代码可能仍然有些运气。 许多流行的库已经从在源代码中使用commonjs转移到了符合标准的es模块import
语法。
Some packages have a separate src/
and dist/
directory where the esmodule style code is in src/
which isn’t included in the package available through npm. In that case you can import from the source directly.
某些软件包具有单独的src/
和dist/
目录,其中esmodule样式代码位于src/
中,但npm中未包含在软件包中。 在这种情况下,您可以直接从源导入。
import throttle from "https://raw.githubusercontent.com/lodash/lodash/master/throttle.js";
I got this URL by clicking on the “raw” button on github to get the raw JS file. It’s probably neater to use a github cdn or to see if the file is available through github pages, but this works.
我通过单击github上的“ raw”按钮获取此URL,以获取原始JS文件。 使用github cdn或查看文件是否可以通过github页面使用可能更整洁,但这是可行的。
NB: Some libraries use esmodules with webpack, or a module loader which lets them import from node modules like this:
注意:某些库将esmodules与webpack一起使用,或者使用模块加载器,使它们可以从节点模块中导入,如下所示:
Bad:import { someFunction } from "modulename";import { someOtherFunction } from "modulename/file.js";
The standard for imports is that they need to start with ./
or be a URL to work
导入的标准是,它们需要以./
开头或者是一个可以正常工作的URL
Good:import { someOtherFunction } from "./folder/file.js";
In that situation try the next method:
在这种情况下,请尝试下一种方法:
3.导入commonjs模块 (3. Importing commonjs modules)
Fortunately there is a service called JSPM which will resolve the 3rd party modules and compile the commonjs modules to work as esmodule imports. This tool is for using node modules in the browser without a build step. But we can use it here too.
幸运的是,有一个名为JSPM的服务,它将解析第三者模块并编译commonjs模块以用作esmodule导入。 该工具用于在浏览器中使用节点模块,而无需构建步骤。 但是我们也可以在这里使用它。
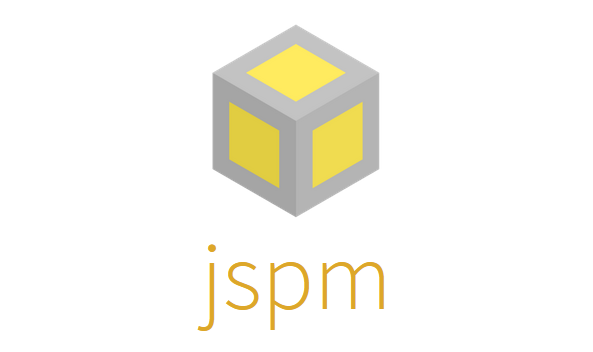
In my most recent project i wanted to do push notifications, which involves generating the credentials for VAPID, there is a deno crypto library which can do encryption but doing the full procedure is difficult and I’d rather use the popular web-push library. I can import it using the JSPM CDN using the URL like below:
在我最近的项目中,我想进行推送通知,其中涉及生成VAPID的凭据,有一个可以加密的deno密码库,但是执行整个过程很困难,我宁愿使用流行的网络推送库。 我可以使用JSPM CDN和如下URL导入它:
import webPush from "https://dev.jspm.io/web-push";
I can now use it like any other module in deno.
我现在可以像deno中的任何其他模块一样使用它。
This almost worked 100% some of the bits which relied on specific node behaviors such as making network requests failed in this situation I had to work around this to use the standardised fetch
API deno uses.
在这种情况下,几乎100%依赖于特定节点行为(例如使网络请求失败)的某些位都起作用,我必须解决此问题以使用标准化的fetch
API表示法使用。
使Typescript类型正常工作 (Getting Typescript types working)
One nice feature of typescipt, which deno uses, is that it provides really good autocomplete for modules. The deno extension for my editor even can autocomplete for third part modules if it knows the type definitions.
deno使用的typecipt的一个不错的功能是,它为模块提供了非常好的自动完成功能。 如果编辑器的deno扩展知道类型定义,它甚至可以自动完成第三方模块。
This isn’t essential to getting the code to work but can provide huge benefits for helping you maintain your code.
这对于使代码正常工作不是必需的,但可以为您维护代码提供巨大的好处。
When I was importing another module called fast-xml-parser when I was looking through the source code I noticed it had a type definitions file which is a file which ends in .d.ts
. These files describe the various interfaces and even work for even for JavaScript .js
files. You can sometimes also find the type definitions files in the @types\somemodule
repo.
当我在查看源代码时导入另一个名为fast-xml-parser的模块时,我注意到它具有一个类型定义文件,该文件以.d.ts
。 这些文件描述了各种接口,甚至适用于JavaScript .js
文件。 有时您也可以在@types\somemodule
存储库中找到类型定义文件。
Using this file typescript can auto complete on things imported from JavaScript files. Even for files imported using JSPM:
使用此文件打字稿可以自动完成从JavaScript文件导入的内容。 即使对于使用JSPM导入的文件:
// Import the fast-xml-parser library
import fastXMLParser from "https://dev.jspm.io/fast-xml-parser";// Import the type definition file from the source code of fast-xml-parser
import * as FastXMLParser from "https://raw.githubusercontent.com/NaturalIntelligence/fast-xml-parser/master/src/parser.d.ts";// Use the parser with the types
const parser = fastXMLParser as typeof FastXMLParser;
I import the type definitions from the definition files as FastXMLParser
(note the uppercase F) this doesn’t contain any working code but is an object which has the same type as the code we want to import.
我从定义文件中将类型定义作为FastXMLParser
(请注意大写的F),它不包含任何工作代码,而是一个与我们要导入的代码具有相同类型的对象。
I import the code from JSPM as fastXMLParser
(lowercase f) which is the working code but has no types.
我从JSPM fastXMLParser
代码导入为fastXMLParser
(小写字母f),这是有效的代码,但没有类型。
Next I combine them together to make parser
which is fastXMLParser
with the type of FastXMLParser
.
接下来,我将它们组合在一起以创建parser
,该parser
是具有fastXMLParser
类型的FastXMLParser
。
Thank you for reading, I hope you give deno a go. The ability to use any module made for the web and even some which were made for node/npm really gives this new server side library ecosystem a good foundation to get started from. 🦕
谢谢您的阅读,希望您能为deno努力 。 使用任何用于Web的模块甚至是用于node / npm的模块的能力确实为这个新的服务器端库生态系统奠定了良好的基础。 🦕
翻译自: https://medium.com/samsung-internet-dev/using-node-modules-in-deno-2885600ed7a9
deno使用rust