docker react
Docker is possibly the most popular solution to have all your services run in a container. Containers allow us to run and develop an application in the same environment, regardless of what machine you’re on.
Docker可能是使所有服务都在容器中运行的最受欢迎的解决方案。 容器使我们能够在相同的环境中运行和开发应用程序,而不管您使用的是哪种计算机。
Containers are a standardized unit of software that allows developers to isolate their app from its environment, solving the “it works on my machine” headache.
容器是软件的标准化单元,它使开发人员可以将其应用程序与环境隔离开来,从而解决“在我的机器上工作”的难题。
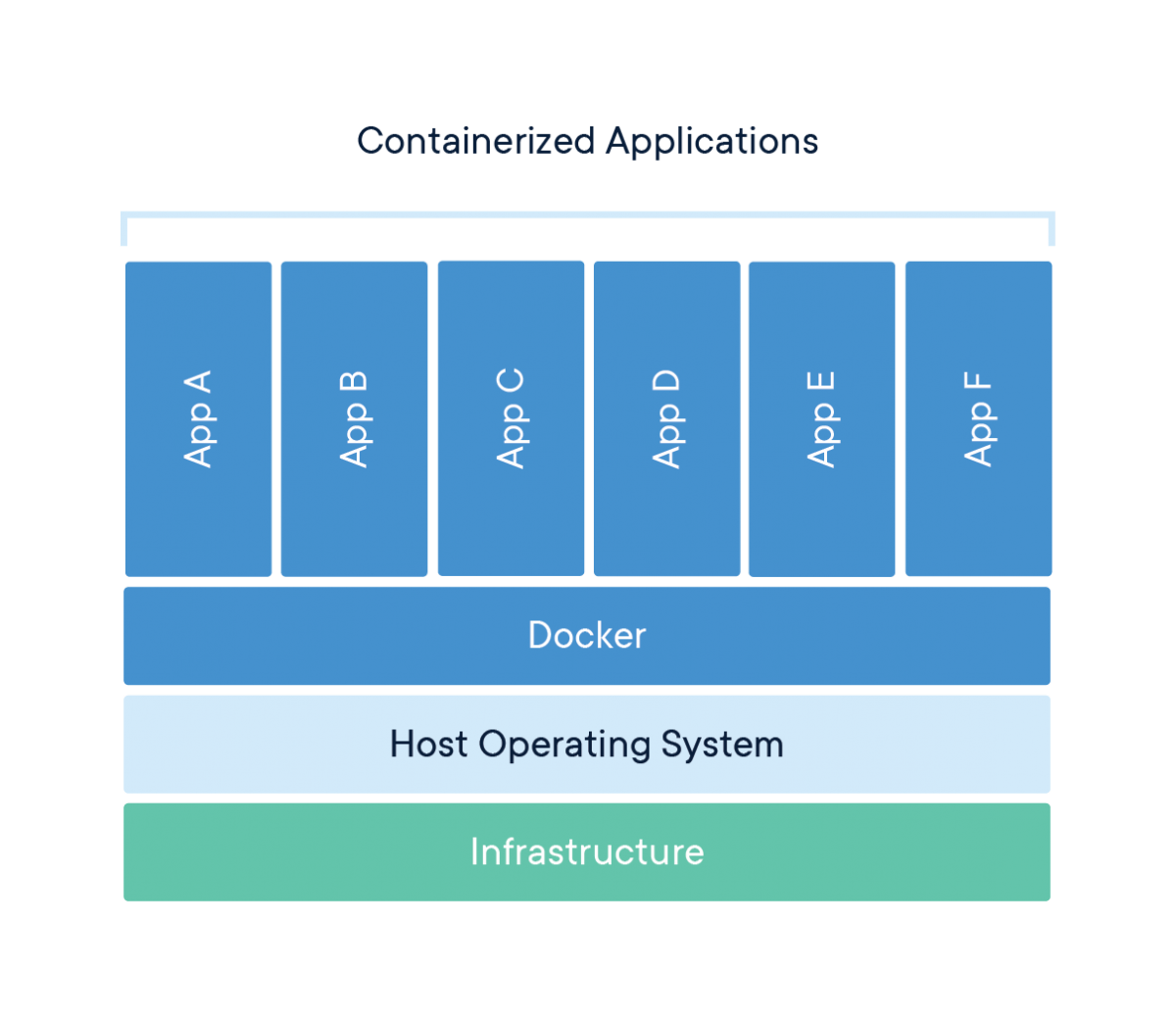
Containers are an abstraction at the app layer that packages code and dependencies together. Multiple containers can run on the same machine and share the OS kernel with other containers.
容器是应用程序层的抽象,将代码和依赖项打包在一起。 多个容器可以在同一台计算机上运行,并与其他容器共享OS内核。
When you deploy an application you have to install all the dependencies (MySQL, Node, Redis) on the host system. With Docker, instead of managing host systems, you just specify a Docker file that lists all the dependencies for the project.
部署应用程序时,必须在主机系统上安装所有依赖项(MySQL,Node,Redis)。 使用Docker,您无需指定主机系统,只需指定一个Docker文件即可列出项目的所有依赖项。
Docker builds an image out of all of those dependencies which we can re-use over multiple computers. Docker users can store images in private or public repositories, and from there can then deploy containers, test images, and share them.
Docker从所有这些依赖关系中构建了一个映像,我们可以在多台计算机上重复使用它们。 Docker用户可以将映像存储在私有或公共存储库中 ,然后可以从那里部署容器,测试映像并共享它们。
Think of Docker applications as tiny containers, which have their own filesystem and dedicated RAM, but a shared kernel with the host operating system.
将Docker应用程序视为微小的容器,它们具有自己的文件系统和专用的RAM,但与主机操作系统共享一个内核。
这是为什么您应该考虑使用Docker的一些原因 (Here Are a Few Reasons Why You Should Consider Using Docker)
- Docker helps to make shipping environments more predictable. Docker帮助使运输环境更加可预测。
- Solves the “works on my machine” problem. 解决“在我的机器上工作”的问题。
- You can share and reuse Docker containers between projects and teams. 您可以在项目和团队之间共享和重用Docker容器。
安装Docker (Installing Docker)
You can download and install Docker on multiple platforms. Refer to the following section and choose the best installation path for you. Head over to this link to download Docker.
您可以在多个平台上下载并安装Docker。 请参考以下部分,并为您选择最佳的安装路径。 转到此链接下载Docker 。
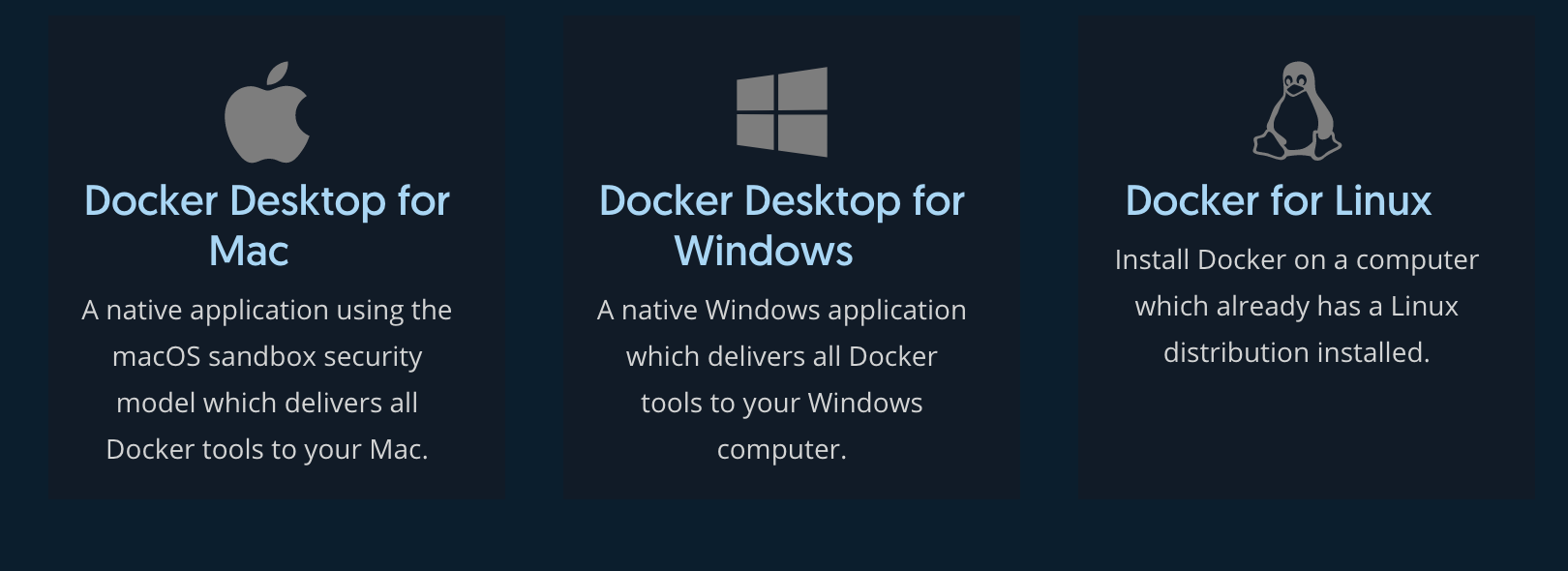
Docker安装确认 (Docker Installation Confirmation)
Once you installed Docker on your machine, open the terminal and type the following. You should see a docker version in the console. However, if you don’t see a version, please reinstall or restart your machine.
在计算机上安装Docker之后,打开终端并输入以下内容。 您应该在控制台中看到一个docker版本。 但是,如果看不到版本,请重新安装或重新启动计算机。
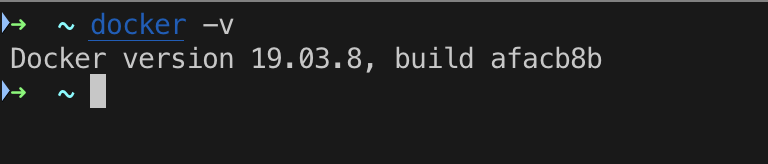
创建一个React项目 (Creating A React Project)
Create React App doesn’t handle backend logic or databases; it just creates a frontend build pipeline, so you can use it with any backend you want. Under the hood, it uses Babel and webpack.
Create React App不处理后端逻辑或数据库; 它只是创建一个前端构建管道,因此您可以将其与所需的任何后端一起使用。 在后台 ,它使用Babel和webpack 。
Note: npx
is not a typo, it’s a package runner tool that comes with npm 5.2+.
注意: npx
不是拼写错误,它是npm 5.2+附带的打包运行程序工具 。
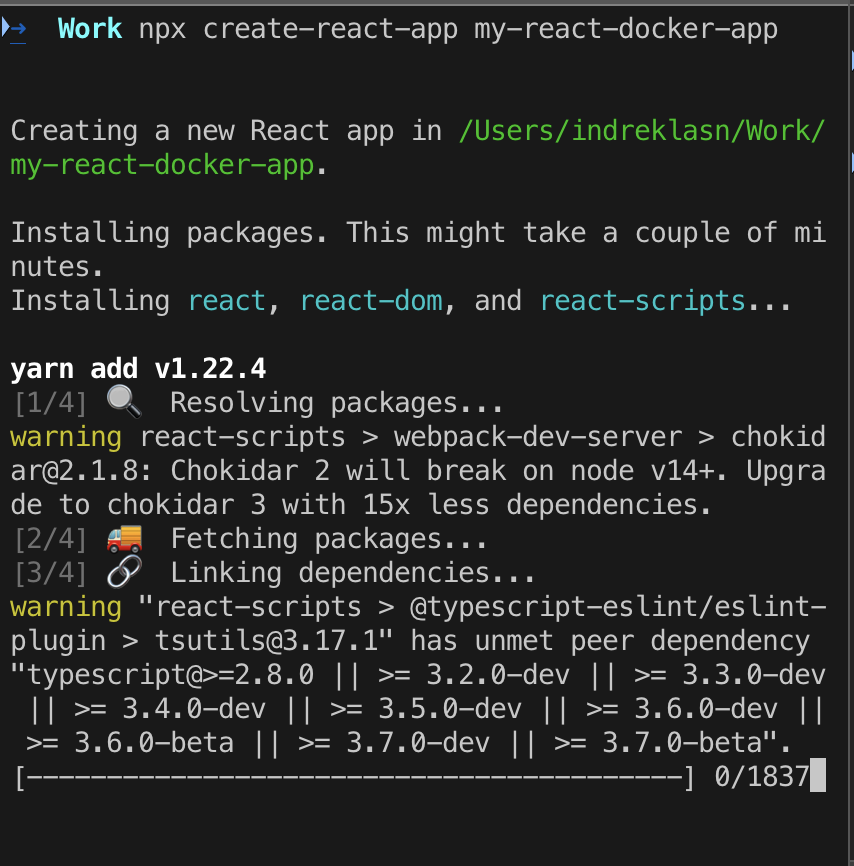
Docker化React应用 (Dockerizing a React App)
Next, open the React project with your favorite text editor or IDE. We’re going to need to create the following three files for Docker:
接下来,使用您喜欢的文本编辑器或IDE打开React项目。 我们将需要为Docker创建以下三个文件:
Dockerfile
: ADockerfile
is a set of instructions used to construct an image. Inside this file, we declare the software we want to use for our project. For instance, for a React project, we’re going to need Node.js. Dockerfile is usually used for production purposes.Dockerfile
:Dockerfile
是用于构造映像的一组指令。 在此文件中,我们声明要在项目中使用的软件。 例如,对于一个React项目,我们将需要Node.js。 Dockerfile通常用于生产目的。Dockerfile.dev
: The same concept as the aboveDockerfile
, the main difference between aDockerfile
andDockerfile.dev
is the former is used for the production environment, the latter is used for the local development environment.Dockerfile.dev
:与上述Dockerfile
相同的概念,Dockerfile
和Dockerfile.dev
之间的主要区别是前者用于生产环境,后者用于本地开发环境。docker-compose.yml
: Compose is a tool for defining and running multi-container Docker applications. With Compose, you use a YAML file to configure your application’s services. Then, with a single command, you create and start all the services from your configuration. To learn more about all the features of Compose, see the list of features.docker-compose.yml
:Compose是用于定义和运行多容器Docker应用程序的工具。 通过Compose,您可以使用YAML文件来配置应用程序的服务。 然后,使用一个命令,就可以从配置中创建并启动所有服务。 要了解有关Compose的所有功能的更多信息,请参阅功能列表 。
编码Dockerfile (Coding the Dockerfiles)
When we start a new Docker project, Docker doesn’t know anything about React, Node, or the project at all. We need to explicitly tell Docker what software we need to run our project. For instance, we know that for a React project we definitely are going to make use of Node.js and NPM or Yarn.
当我们开始一个新的Docker项目时,Docker完全不了解React,Node或项目。 我们需要明确告诉Docker我们需要什么软件来运行我们的项目。 例如,我们知道对于React项目,我们肯定会使用Node.js和NPM或Yarn。
Dockerfile.dev (Dockerfile.dev)
Next, open the Dockerfile.dev
and write the following.
接下来,打开Dockerfile.dev
并编写以下内容。
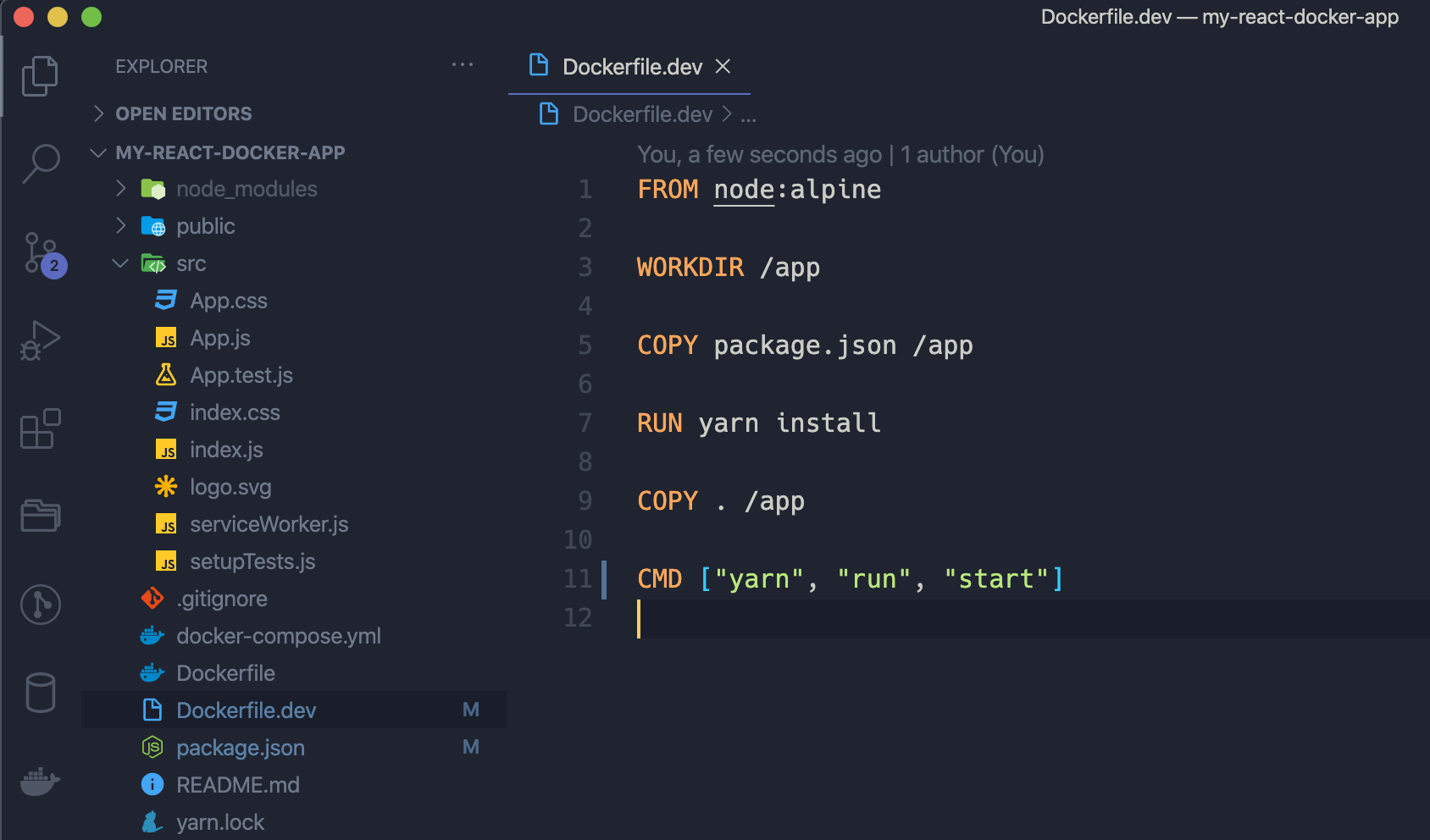
这是正在发生的事情; (Here’s what’s happening;)
We’re telling Docker to grab a copy of Node and specify its Linux distribution as Alpine. Docker uses the Alpine Linux distribution by default. Why Alpine? Alpine Linux is much smaller than most distribution base images (~5MB), and thus leads to much slimmer images in general.
我们要告诉Docker抓取Node的一个副本,并将其Linux发行版指定为Alpine。 Docker默认使用Alpine Linux发行版。 为什么是高山? Alpine Linux比大多数分发基础映像小得多(约5MB),因此通常导致更苗条的映像。
Next, we set our working directory for the Docker container with the
WORKDIR
command. Once we have declared a working directory, anyCMD
,RUN
,ADD
,COPY
command will be executed in the specified working directory.接下来,我们使用
WORKDIR
命令为Docker容器设置工作目录。 声明工作目录后,任何CMD
,RUN
,ADD
,COPY
命令都将在指定的工作目录中执行。Copy the
package.json
from our React project to the Docker container.将
package.json
从我们的React项目复制到Docker容器中。- Install the dependencies and copy the rest of our application to the Docker container. 安装依赖项并将其余应用程序复制到Docker容器。
Lastly, run the development script. For CRA it’s
yarn run start
to start the development server.最后,运行开发脚本。 对于CRA,
yarn run start
要启动开发服务器。
设置docker-compose.yml (Setting Up the docker-compose.yml)
Remember, Compose is a useful tool to chain all the docker commands and services together. Let’s create a service called client
and specify what it should do.
请记住, Compose是将所有docker命令和服务链接在一起的有用工具。 让我们创建一个名为client
的服务,并指定它应该做什么。
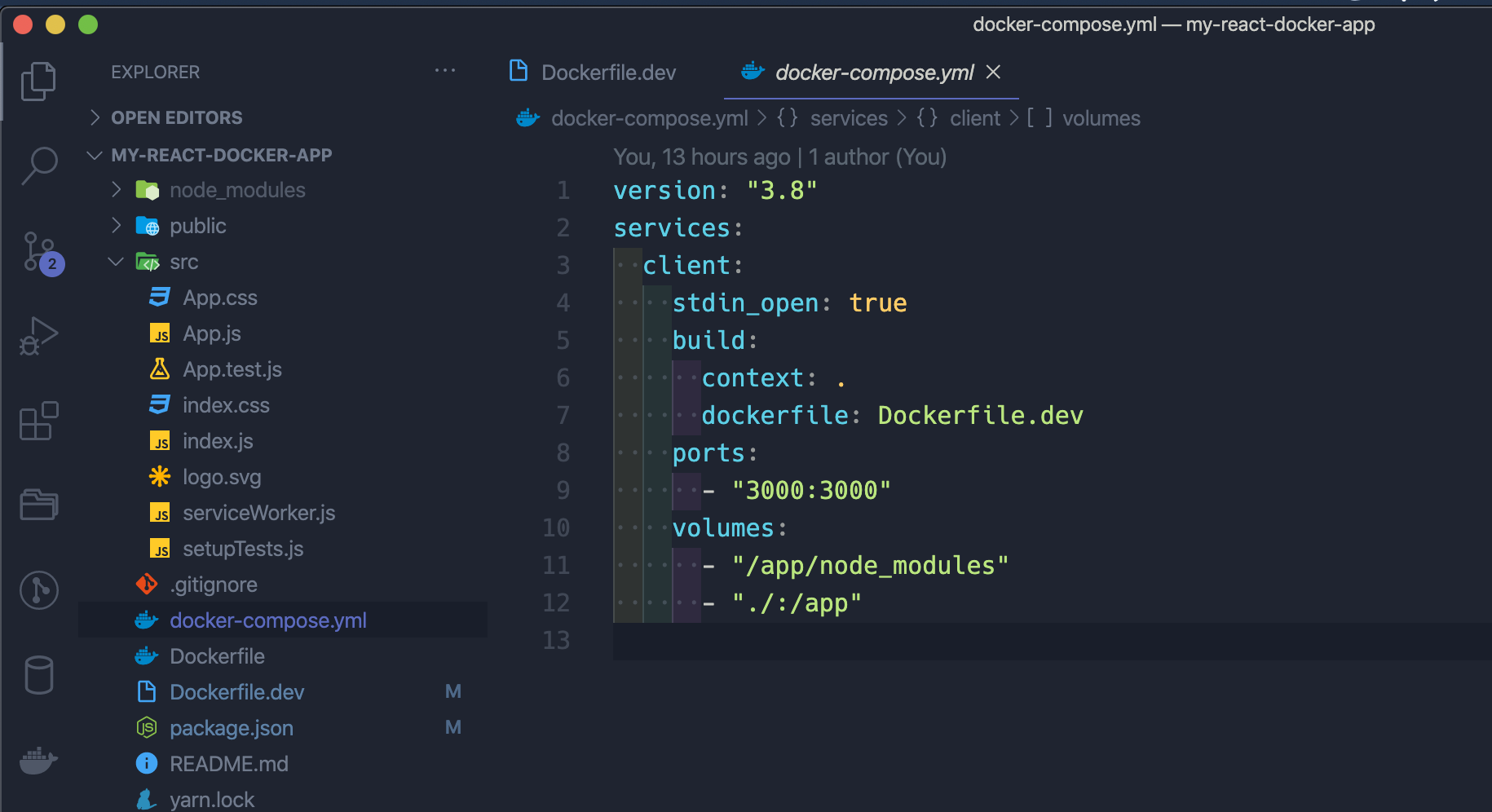
这是docker-compose.yml文件中发生的事情 (Here’s what’s happening inside the docker-compose.yml file)
We specify a version for Compose. Make sure Compose is compatible with Docker, here’s a full list of all versions. At the time of writing this, version
3.8
is the latest release, so we’re going use that.我们为Compose指定一个版本。 确保Compose与Docker兼容, 这是所有版本的完整列表 。 在撰写本文时,版本
3.8
是最新版本,因此我们将使用它。Define the
client
service so we can run it in an isolated environment.定义
client
服务,以便我们可以在隔离的环境中运行它。The
client
service requires a docker file to be specified. For development, we’re going to use theDockerfile.dev
file.client
服务需要指定docker文件。 为了进行开发,我们将使用Dockerfile.dev
文件。Next, we map the port
3000
to Docker. The React application runs on port3000
, so we need to tell Docker which port to expose for our application.接下来,我们将端口
3000
映射到Docker。 React应用程序在端口3000
上运行,因此我们需要告诉Docker为应用程序公开哪个端口。
使用撰写 (Using Compose)
Once we’re done writing the configuration, we can simply run thedocker-compose up
command and Docker builds up everything for us.
完成配置编写后,我们只需运行docker-compose up
命令,Docker便会为我们构建所有内容。
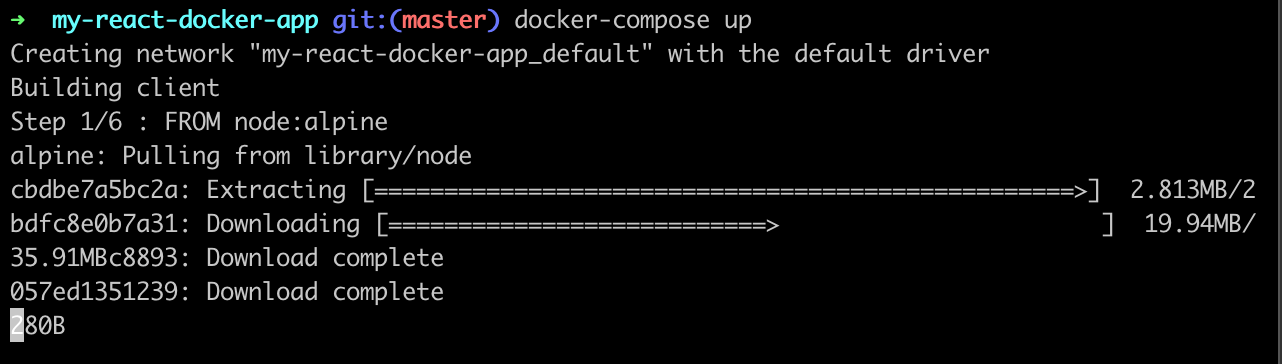
You should see all the commands which we declared in the Dockerfile.dev being executed. Once Compose is done doing its stuff, we can open the React application at http://localhost:3000/
.
您应该看到正在Dockerfile.dev中声明的所有命令正在执行。 完成Compose的工作后,我们可以在http://localhost:3000/
打开React应用程序。
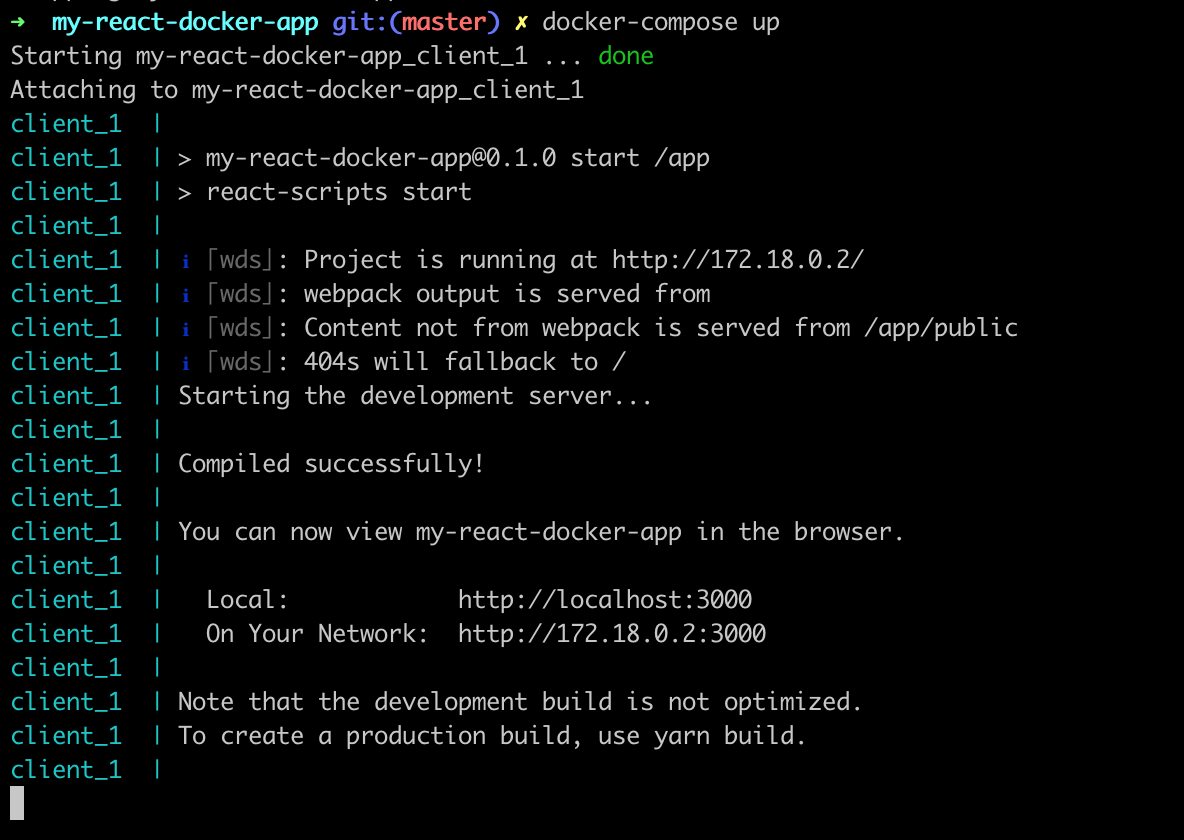
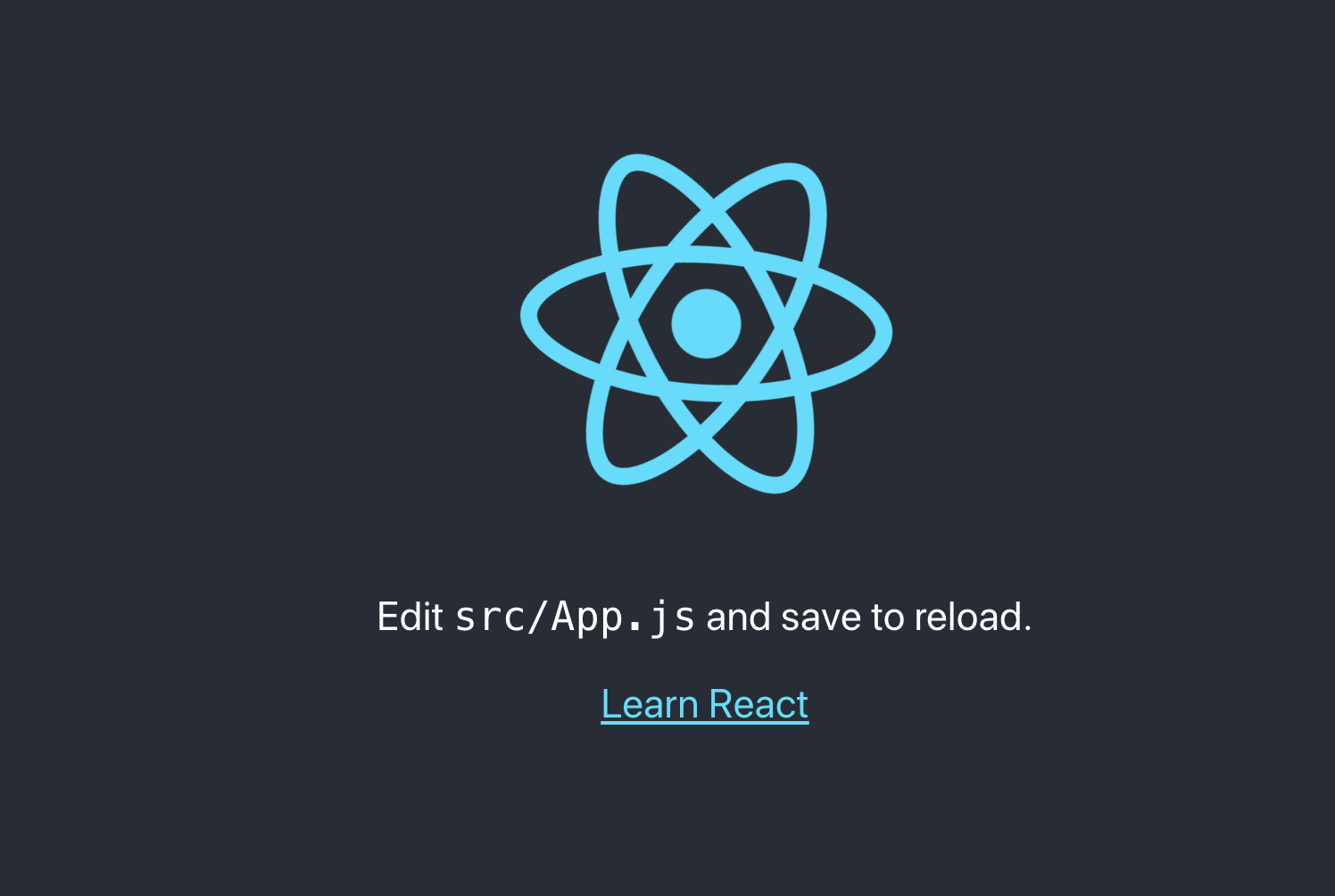
Here we go, now we’re ready to develop our React application. If you did run into any issues, check out the GitHub repository for this article.
到这里开始,现在我们准备开发我们的React应用程序。 如果确实遇到任何问题,请查看本文的GitHub存储库 。
Check out part 2, where we’re going to discuss how to use Docker for production and continuous integration.
请参阅第2部分 ,我们将在这里讨论如何使用Docker进行生产和持续集成。
docker react