双向列表插入节点的代码
了解问题 (Understand the Problem)
We are given a function called insertNodeAtPosition, which has three parameters, head, data, and position.
我们获得了一个名为insertNodeAtPosition的函数,该函数具有三个参数,head,data和position。
Our head is a SinglyLinkedListNode (class) pointer to the head of the list, our data is an integer value to insert as data in a new node which we will create, and the position is a zero based index position to where we should insert our new node.
我们的头是指向列表头的SinglyLinkedListNode(类)指针,我们的数据是要作为数据插入到我们将要创建的新节点中的整数值,并且该位置是从零开始的索引位置,该位置应插入我们的位置新节点。
head: pointer to the head of the listdata: integer value of our new node (1)position: a zero based index position (2)
In order to insert a Node at a specific position in the singly linked list, we must create a new node with our data parameter, insert it at the position we were given, and return the head node.
为了在单链列表中的特定位置插入一个Node,我们必须使用data参数创建一个新节点,将其插入给定的位置,然后返回头节点。
Example:If our list starts as4 -> 6 -> 2 -> 9and our position = 2 and our data = 3. Our new list will be4 -> 6 -> 3 -> 2 -> 9
We must return a reference to the head node of our finished list.
我们必须返回对完成列表的头节点的引用。
计划 (Plan)
We know that our first step will have to be to create a new node in order to have one to insert. Taking a look at our SinglyLinkedListNode class, we can see that it is expecting data to be passed in.
我们知道,第一步必须是创建一个新节点才能插入节点。 看一下我们的SinglyLinkedListNode类,我们可以看到它正在等待数据传递。
class SinglyLinkedListNode:
def __init__(self, node_data):
self.data = node_data
self.next = None
We can create a new node in our insertNodeAtPosition function definition by setting new to SinglyLinkedListNode(data).
通过将new设置为SinglyLinkedListNode(data),我们可以在insertNodeAtPosition函数定义中创建一个新节点。
new = SinglyLinkedListNode(data)
We know that our position is index value 2, so we’ll need to set a pointer to the head in order to traverse through the list from the very beginning, and also set a counter in order to move our pointer through the list node by node, until we reach our given position.
我们知道我们的位置是索引值2,因此我们需要设置指向头的指针,以便从头开始遍历列表,还需要设置一个计数器,以使指针通过list节点移动节点,直到到达给定位置。
pointer = head
counter = 1
We will need to traverse through the list in order reach our position, starting with the head. Since our pointer is already set to the head, we can use a while loop that will traverse through the list until pointer.next is at our given position. We do this while pointer.next is not None.
我们需要遍历列表,以便从头开始到达位置。 由于我们的指针已经设置在头部,因此我们可以使用while循环遍历列表,直到pointer.next到达给定位置。 我们在pointer.next不是None的情况下执行此操作。
while pointer.next is not None:
We will traverse through the list position by position, with our pointer, until we are at our given position, setting counter to counter + 1.
我们将使用指针逐个遍历列表位置,直到到达给定位置,将counter设置为counter + 1。
counter += 1
pointer = pointer.next
If pointer.next is finally at our given position, where our counter would be equal to our position of 2, we would set new.next to point to pointer.next.
如果pointer.next最终位于给定位置,我们的计数器等于我们的位置2,则将new.next设置为指向pointer.next。
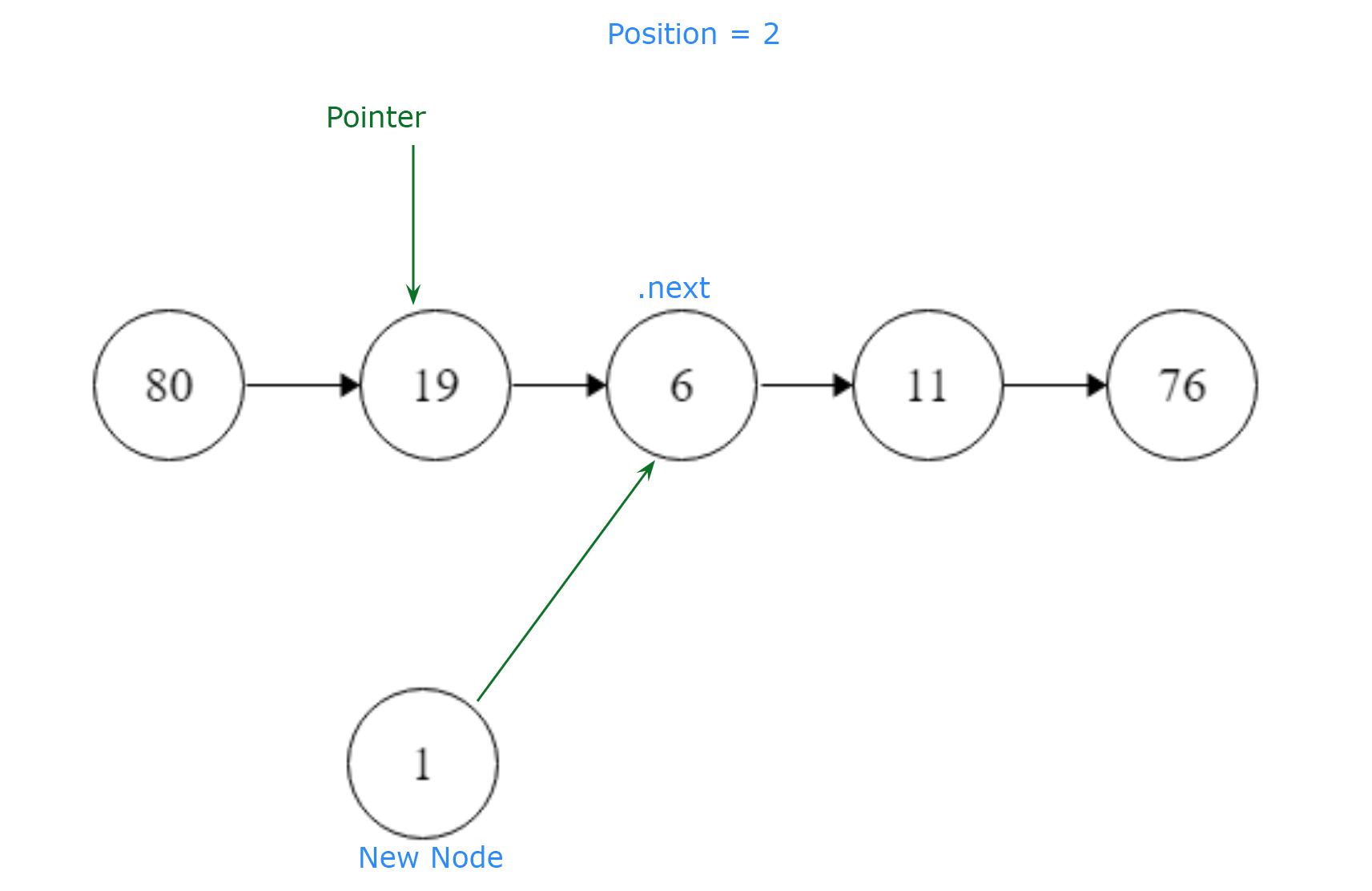
Then we would set our pointer.next to our new node, and break.
然后我们将pointer.next设置到新节点,然后中断。

if counter == position:
# new.next will point to pointer.next
new.next = pointer.next
# and our pointer.next will be equal to our new node
pointer.next = new
break
执行 (Execute)
# For our reference:# SinglyLinkedListNode:
# int data
# SinglyLinkedListNode next
#
#def insertNodeAtPosition(head, data, position): # create a new node
new = SinglyLinkedListNode(data)
# set a pointer to head in case our initial position is not 0
pointer = head
# set our counter so we can traverse through SLL
counter = 1
while pointer.next is not None:
# and if our counter is equal to the position given
if counter == position:
# our new node will point to pointer.next
new.next = pointer.next
# and our pointer.next will be equal to our new node
pointer.next = new
break
# we traverse the list one position at a time until
# counter == position
counter += 1
pointer = pointer.next
# we return the head as requested
return head
奖金 (Bonus)
In the case where our given position is zero, or if we were asked to insert a new node at the beginning of the list, we would set our new node pointer to the head node.
如果给定位置为零,或者要求我们在列表的开头插入新节点,则将新节点指针设置为头节点。
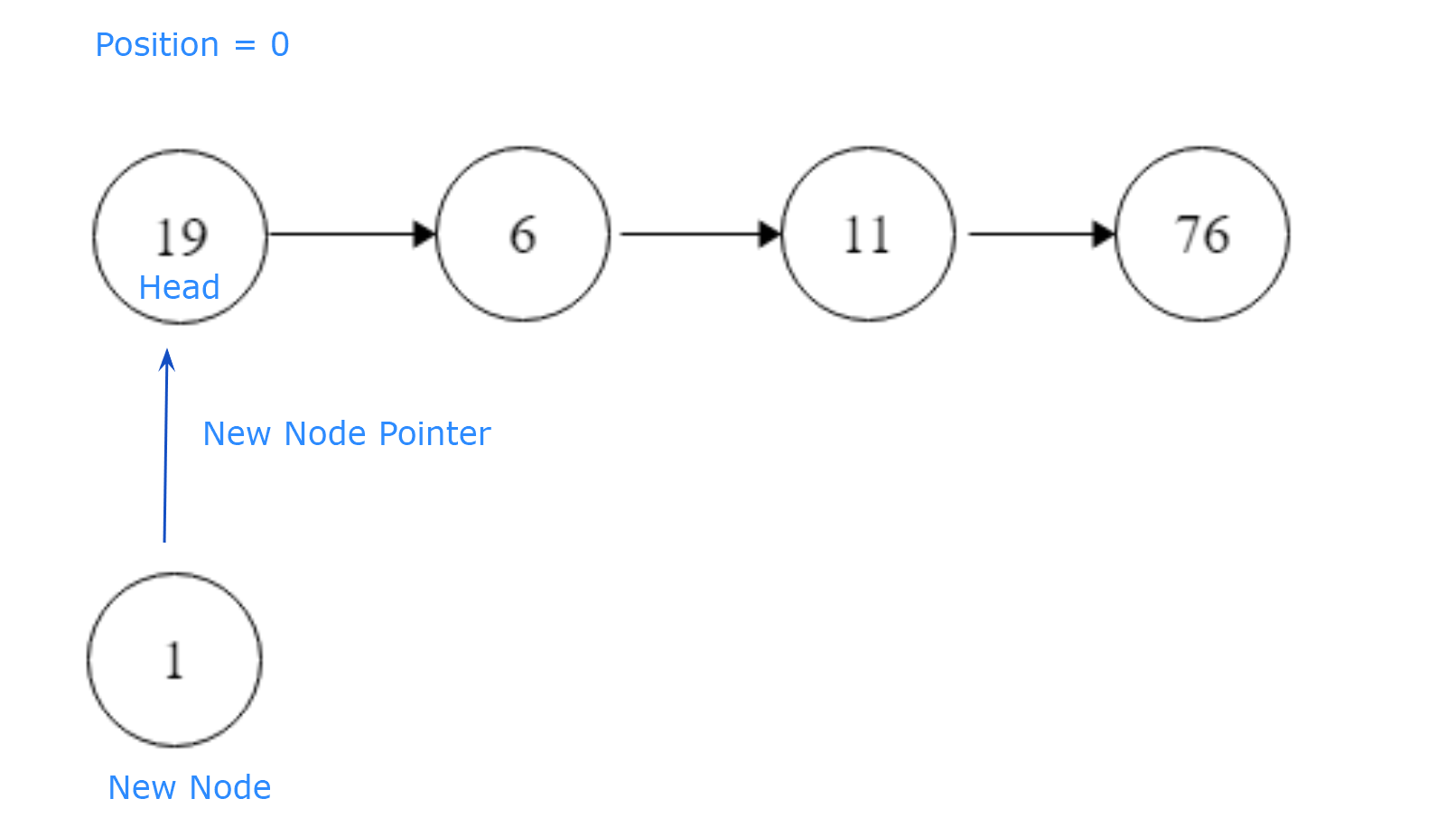
Then we need to set the head to our new node, and return it.
然后,我们需要将头设置为新节点,然后将其返回。
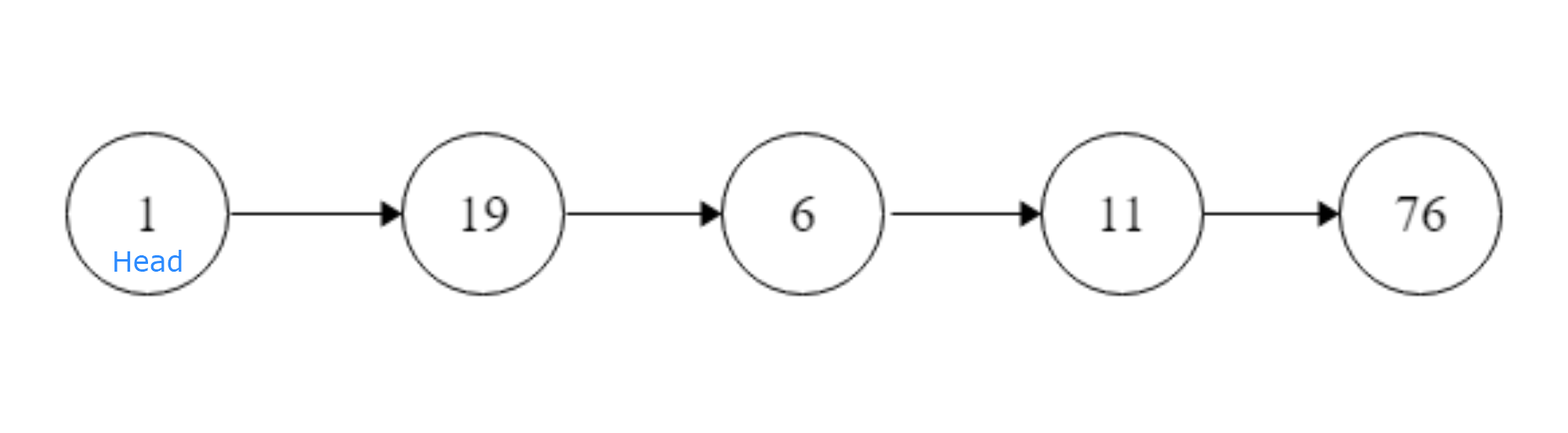
if position == 0:
# our new node will point to the head
new.next = head
# now head will point to the new node
head = new
# we then return head as requested
return head
The execution code with both test cases would be:
这两个测试用例的执行代码为:
# For our reference:# SinglyLinkedListNode:
# int data
# SinglyLinkedListNode next
#
#def insertNodeAtPosition(head, data, position):
# create a new node
new = SinglyLinkedListNode(data)
# set a pointer to head in case our initial position is not 0
pointer = head
# set our counter so we can traverse through SLL
counter = 1
# if our given position is 0
if position == 0:
# our new node will point to the head
new.next = head
# now head will point to the new node
head = new
# we then return head as requested
return head
# while the next node away from the head node is not None
while pointer.next is not None:
# and if our counter is equal to the position given
if counter == position:
# our new node will point to pointer.next
new.next = pointer.next
# and our pointer.next will be equal to our new node
pointer.next = new
break
# we traverse the list one position at a time until counter
# == position
counter += 1
pointer = pointer.next
# we return the head as requested
return head
双向列表插入节点的代码