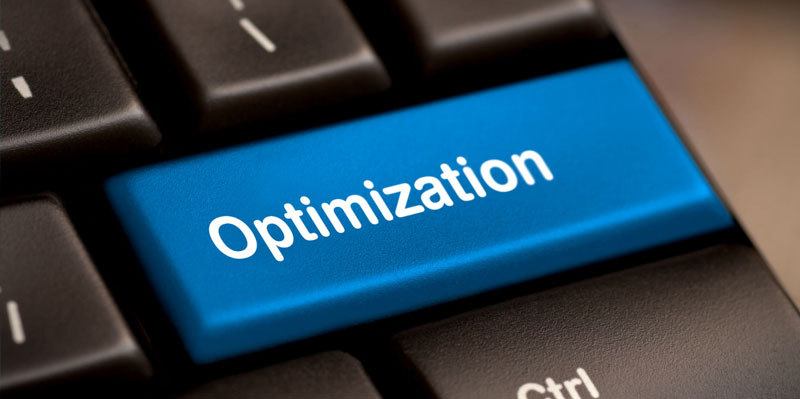
参考资料
https://www.slideshare.net/shunjiumetani/ss-17197023www.slideshare.net https://a3a6340e-a-62cb3a1a-s-sites.googlegroups.com/site/shunjiumetani/file/2dspp_slide.pdf?attachauth=ANoY7crqG_yEvQY0dpoIam8sYgTR3Su3_SPNEO8PYdhCJO4pEvX0zuP_0FvAmoAfGGjXKVtPBtAchSLPOOV30FzD6VBIhvCy_0dZYVKVv1ioxjpxdiZ05LsikVRyeeaFM_jL-A4dzQDBQdMkBKK8-_cIQC6bz_IPRCmCGsltSS2wJcOKYbEB6cJ3IhxG9F1JkoC4Ws7JjnB3cq0ZtKBNs60n2Pq5mYtTx_Z0ZT5eYfo-rmM36aG-s3k%3D&attredirects=0a3a6340e-a-62cb3a1a-s-sites.googlegroups.com- 二维装箱问题
输入
- 宽
,高
大小的长方形材料
- 产品的集合
,各个长方形
的宽为
,高为
约束条件
- 把产品放在容器内。即满足以下约束条件,
- 产品相互重叠。即满足以下约束条件,
二维装箱问题可用整数规划建模求解。这里不详细介绍。
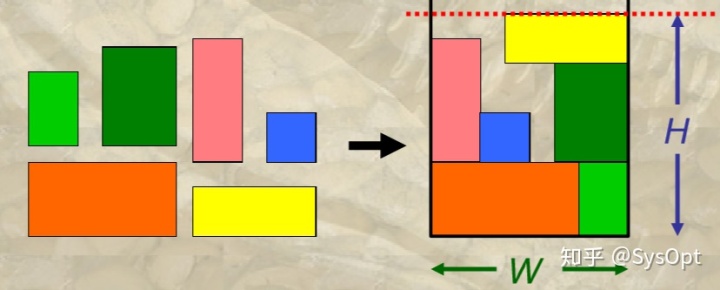
- Next-Fit Algorithm
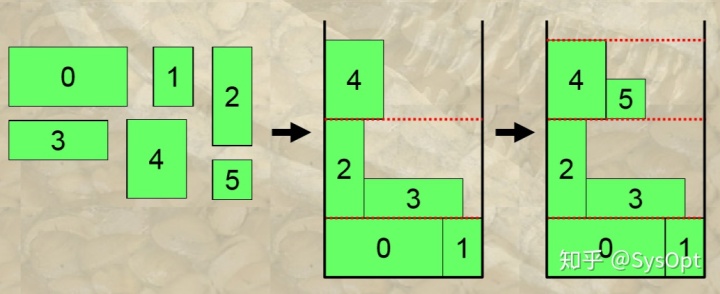
Next-Fit Algorithm 是由长方形的号码顺序进行一个个的放置。如上图中,首先放置0生成红线的level,然后放置1。等到放置不下时,由2产生新的红色level,重复此过程直到所有长方形放置完毕。这个算法的计算时间为
- Code
代码包括
- 数据读取
- 计算时间测量
- Next-Fit Algorithm
- 输出结果
#include <stdlib.h>
#include <stdio.h>
#include <time.h>
#define FALSE 0
#define TRUE 1
#define MAX_N 10000
double strip_width, strip_height;
int n; /* 长方形个数 */
double w[MAX_N], h[MAX_N]; /* w[i]h[i]/
double x[MAX_N], y[MAX_N]; /* 坐标(x[i],y[i])*/
void next_fit();
int main(){
FILE *input_file, *output_file;
double start_time, search_time;
double area, efficiency;
int i;
//读取数据
input_file = fopen("N1.rec", "r");
fscanf(input_file, "w= %lfn", &strip_width);
fscanf(input_file, "n= %dn",&n);
for(i = 0; i < n; i++){
fscanf(input_file, "%lf %lfn",&(w[i]),&(h[i]));
}
fclose(input_file);
printf("w= %fn",strip_width);
printf("n= %dn",n);
for(i = 0; i < n; i++){
printf("%4dt%ft%fn",i,w[i],h[i]);
}
//计算开始
start_time = (double)clock()/CLOCKS_PER_SEC;
next_fit();
//计算结束
search_time = (double)clock()/CLOCKS_PER_SEC - start_time;
strip_height = 0.0;
for(i = 0; i < n; i++){
if(y[i]+h[i] > strip_height){
strip_height = y[i]+h[i];
}
}
//充填率
area = 0.0;
for(i = 0; i < n; i++){
area += w[i] * h[i];
}
efficiency = area / (strip_width * strip_height) * 100.0;
//输出
output_file = fopen("result.dat","w");
fprintf(output_file,"w= %fn",strip_width);
fprintf(output_file,"n= %dn",n);
for(i = 0; i < n; i++){
fprintf(output_file,"%ft%fn",w[i],h[i]);
}
for(i = 0; i < n; i++){
fprintf(output_file,"%ft%fn",x[i],y[i]);
}
fclose(output_file);
//确认
printf("Coordinates of rectangles:n");
for(i = 0; i < n; i++){
printf("%dt%ft%fn",i,x[i],y[i]);
}
printf("nh= %fn",strip_height);
printf("time= %fn",search_time);
return(0);
}
void next_fit(){
double level_width, level_height, max_h;
int i;
level_width = level_height = max_h = 0.0;
//生成level
for(i = 0; i < n; i++){
if(level_width + w[i] > strip_width){
x[i] = 0.0;
y[i] = level_height + max_h;
level_width = w[i];
level_height += max_h;
max_h = h[i];
}else{
x[i] = level_width;
y[i] = level_height;
level_width += w[i];
//更新level
if(h[i] > max_h){
max_h = h[i];
}
}
}
}
数据
w= 40
n= 10
7 6
40 16
5 20
24 24
7 4
4 4
7 8
4 20
5 4
7 6
大家可以以这个程序为基础,设计一些算子,提高算法的性能。另外,这个算法依赖放置的顺序,所以还可以在这个方面下功夫。
如果有什么疑问或者建议,可以给我发邮件。➡ zhaoyou728 atoutlook.com