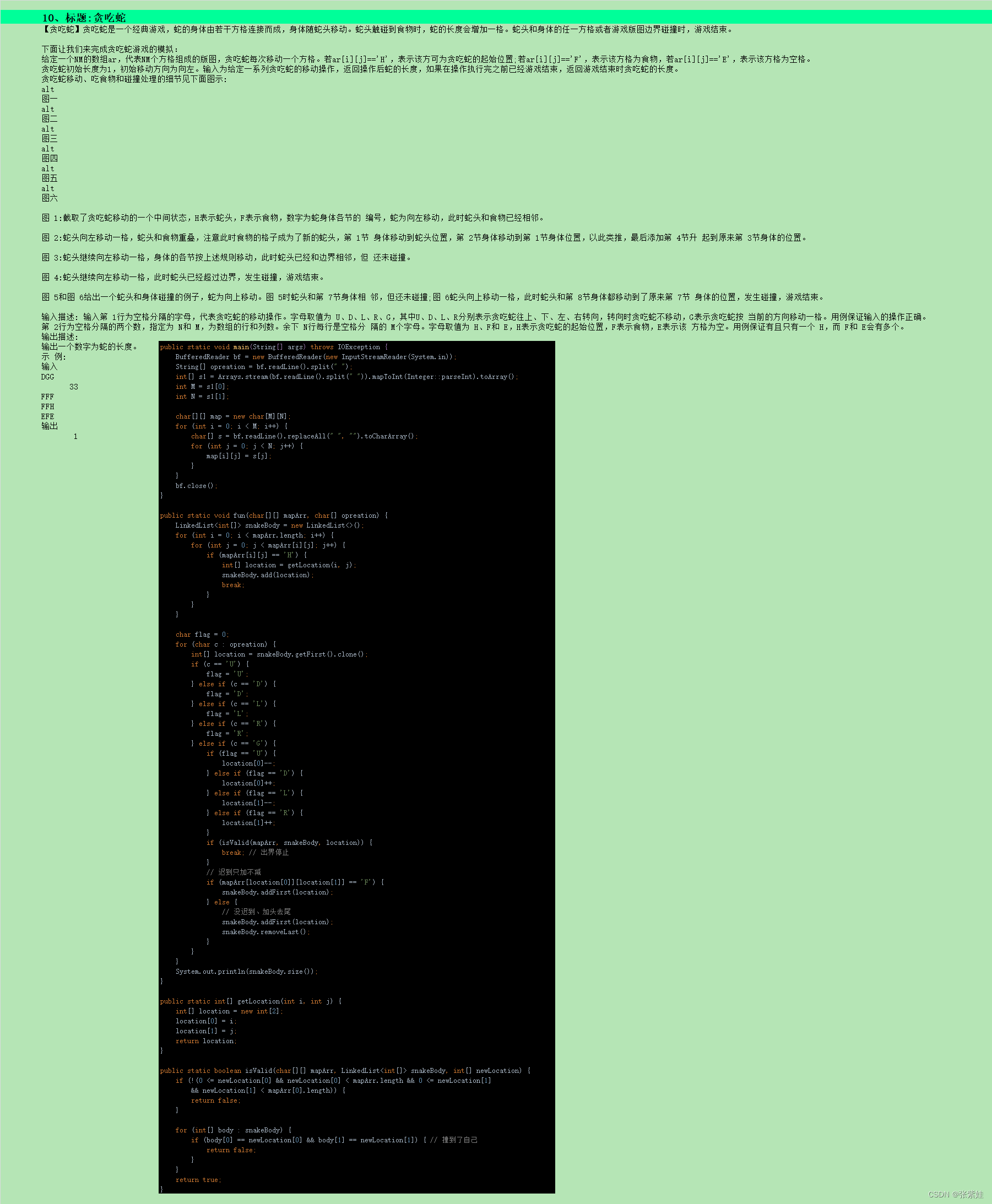
package logic;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.Deque;
import java.util.LinkedList;
import static java.lang.System.in;
public class GluttonousSnake {
public static void main(String[] args) throws IOException {
BufferedReader bf = new BufferedReader(new InputStreamReader(in));
char[] actions = bf.readLine().replaceAll(" ", "").toCharArray();
int[] size = Arrays.stream(bf.readLine().split(" ")).mapToInt(Integer::parseInt).toArray();
int x = size[0];
int y = size[1];
char[][] map = new char[x][y];
for (int i = 0; i < x; i++) {
char[] s = bf.readLine().replaceAll(" ", "").toCharArray();
map[i] = s;
}
bf.close();
Deque<int[]> body = new LinkedList<>();
for (int i = 0; i < x; i++) {
for (int j = 0; j < y; j++) {
if (map[i][j] == 'H') {
body.offerLast(getLocation(i, j));
break;
}
}
}
char tempAction = 0;
for (Character action : actions) {
int[] ints = body.peekFirst().clone();
if ('U' == action) {
tempAction = 'U';
} else if ('D' == action) {
tempAction = 'D';
} else if ('L' == action) {
tempAction = 'L';
} else if ('R' == action) {
tempAction = 'R';
} else if ('G' == action) {
if ('U' == tempAction) {
ints[0]--;
} else if ('D' == tempAction) {
ints[0]++;
} else if ('L' == tempAction) {
ints[1]--;
} else if ('R' == tempAction) {
ints[1]++;
}
if (!isValid(map, ints[0], ints[1], body)) {
break;
}
if (map[ints[0]][ints[1]] == 'F') {
body.addFirst(getLocation(ints[0], ints[1]));
} else {
body.addFirst(getLocation(ints[0], ints[1]));
body.removeLast();
}
}
}
System.out.println(body.size());
}
public static boolean isValid(char[][] map, int i, int j, Deque<int[]> body) {
boolean isValid = true;
if (!(0 <= i && i < map.length && 0 <= j && j < map[i].length)) {
return false;
}
for (int[] ints : body) {
if (ints[0] == i && ints[1] == j) {
return false;
}
}
return isValid;
}
public static int[] getLocation(int i, int j) {
return new int[]{i, j};
}
}