singleNumber-函数
class Solution(object):
def singleNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
nums.sort()
for i in range(len(nums)):
if len(nums) == 1:
return nums[0]
if nums[0] == nums[1]:
del nums[0]
del nums[0]
else:
return nums[0]
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80636509
版权声明:本文为博主原创文章,转载请附上博文链接!
- python 循环:for I in range ( ):
range ( start, stop, step) // 包括start,不包括stop
- del用法
del 删除变量,list中保存的是变量
containsDuplicate
class Solution(object):
def plusOne(self, digits):
"""
:type digits: List[int]
:rtype: List[int]
"""
num = 1
for i in range(len(digits)):
num+=int(digits[i])*(10**(len(digits)-i-1))
return [int(i) for i in list(str(num))]
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80636514
版权声明:本文为博主原创文章,转载请附上博文链接!
- 运算符
** // 指数
- python 循环语句
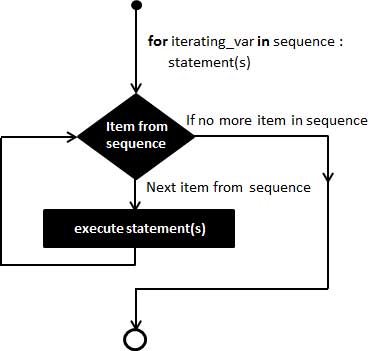
return[int(i)for i inlist(str(num))]
moveZeroes
class Solution(object):
def moveZeroes(self, nums):
"""
:type nums: List[int]
:rtype: void Do not return anything, modify nums in-place instead.
"""
j=0
for i in xrange(len(nums)):
if nums[j] == 0:
nums.append(nums.pop(j))
else:
j+=1
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80636518
版权声明:本文为博主原创文章,转载请附上博文链接!
- xrange
xrange生成一个生成器而非list,range直接生成一个list,在循环中xrange效率要高
Python中 range 和xrange的详细区别blog.csdn.net- append() //在列表末尾添加新的对象。
- pop() 函数用于移除列表中的一个元素(默认最后一个元素),并且返回该元素的值
twoSum
class Solution(object):
def twoSum(self, nums, target):
"""
:type nums: List[int]
:type target: int
:rtype: List[int]
"""
for i in xrange(len(nums)):
for j in xrange(i+1,len(nums)):
if nums[i]+nums[j] == target:
return [i,j]
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80636521
版权声明:本文为博主原创文章,转载请附上博文链接!
- 迭代
isValidSudoku
class Solution(object):
def isValidSudoku(self, board):
"""
:type board: List[List[str]]
:rtype: bool
"""
for i in xrange(9):
hang = board[i]
lie = []
for j in range(9):
lie.append(board[j][i])
for m in hang:
if hang.count(m)>1 and m!='.': //行中某元素出现次数>1
return False
for n in lie:
if lie.count(n)>1 and n!='.': //列中 某元素出现次数>1
return False
for j in xrange(9):
if i%3==0 and j%3==0:
small = [] //small 为一个宫
for p in range(i,i+3):
for q in range(j,j+3):
if board[p][q] != '.':
small.append(board[p][q]) //board中的元素存到宫中
if len(small) != len(list(set(small))): //set() 删除重复数据,如果len()不等说明有重复
print i,j,p,q
return False
return True
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80636525
版权声明:本文为博主原创文章,转载请附上博文链接!
- 二元
for j in range(9):
lie.append(board[j][i])
- 横向纵向检测有没有重复
if hang.count(m)>1 and m!='.':// m 出现次数>1
return False
- 3*3检测有没有重复
if len(small) != len(list(set(small))): //set() 删除重复数据,如果len()不等说明有重复
set(). //set()函数创建一个无序不重复元素集,可进行关系测试,删除重复数据,还可以计算交集、差集、并集等。
Python set() 函数 | 菜鸟教程www.runoob.comrotate
class Solution(object):
def rotate(self, matrix):
"""
:type matrix: List[List[int]]
:rtype: void Do not return anything, modify matrix in-place instead.
"""
n = len(matrix)
for i in range(n/2):
for j in range(i,n-i-1):
tmp = matrix[i][j] //存储[0][0]位置的值
print tmp
matrix[i][j]=matrix[n-j-1][i] //从外层向内层遍历
matrix[j][n-i-1],tmp = tmp,matrix[j][n-i-1] //?
matrix[n-i-1][n-j-1],tmp = tmp,matrix[n-i-1][n-j-1] //?
matrix[n-j-1][i]=tmp
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80636531
版权声明:本文为博主原创文章,转载请附上博文链接!
- 外层(由角到边)
matrix[j][n-i-1],tmp = tmp,matrix[j][n-i-1] //?
matrix[n-i-1][n-j-1],tmp = tmp,matrix[n-i-1][n-j-1] //?
->更改?
matrix[n - 1 - j][i] = matrix[n - 1 - i][n - 1 - j];
matrix[n - 1 - i][n - 1 - j] = matrix[j][n - 1 - i];
firstUniqChar
class Solution(object):
def firstUniqChar(self, s):
"""
:type s: str
:rtype: int
"""
for i in range(len(s)):
if s[i] not in s[i+1:] and s[i] not in s[:i]:
return i
return -1
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80655674
版权声明:本文为博主原创文章,转载请附上博文链接!
- list s[I:]
if s[i] not in s[i+1:] and s[i] not in s[:i]: //s[i+1]后和s[i]前都没有和s[i]一样的值
?isAnagram
class Solution(object):
def isAnagram(self, s, t):
"""
:type s: str
:type t: str
:rtype: bool
"""
if len(s) != len(t):
return False
while s:
tmp = s[0]
s=s.replace(tmp,'')
t=t.replace(tmp,'')
if len(s)!=len(t):
return False
return True
---------------------
作者:我去热饭
来源:CSDN
原文:https://blog.csdn.net/qq_22795513/article/details/80655684
版权声明:本文为博主原创文章,转载请附上博文链接!
- replace(old, new)
while s:
tmp = s[0]
s=s.replace(tmp,'') //将s中所有等于tmp的值为空
t=t.replace(tmp,'') //t中所有等于tmp的值为空
if len(s)!=len(t):
return False
感觉这个答案有点问题,如果是不同的字母出现的次数不同,但是总字母数是相同的,结果虽然return true,但是两个并不是字母异位词呀,是不是可以限制replace 的max为1,这样一个一个replace?