pop
<template>
<div class="myBlock">
<div class="tableBlock">
<div class="title">
{{ newObject ? "操作后的数组" : "操作前的数组" }}
</div>
<el-table :data="tableData" :key="num" stripe border>
<el-table-column prop="name" label="姓名" width="200">
</el-table-column>
<el-table-column prop="phone" label="电话" width="200">
</el-table-column>
<el-table-column prop="idCard" label="证件号">
</el-table-column>
</el-table>
</div>
<div class="operate">
<el-button type="success" plain @click="operate">
pop
</el-button>
</div>
<div class="title" v-if="newObject">操作后的返回值</div>
<div class="newObject">
{{ newObject }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
num: 0,
newObject: null,
};
},
watch: {},
mounted() {
for (var i = 1; i < 4; i++) {
this.tableData.push({
name: "员工" + i + "号",
phone: "153****0000",
idCard: "1111**********0000",
});
}
},
methods: {
operate() {
this.newObject = this.tableData.pop();
this.num += 1;
},
},
};
</script>
操作前
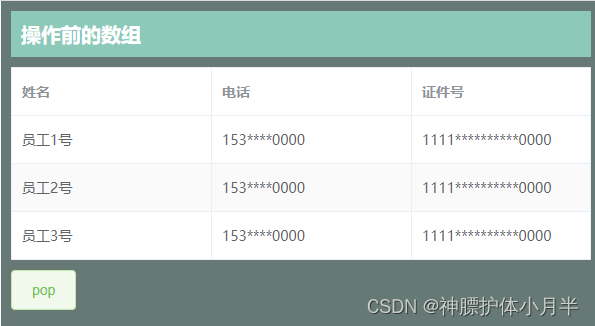
操作后
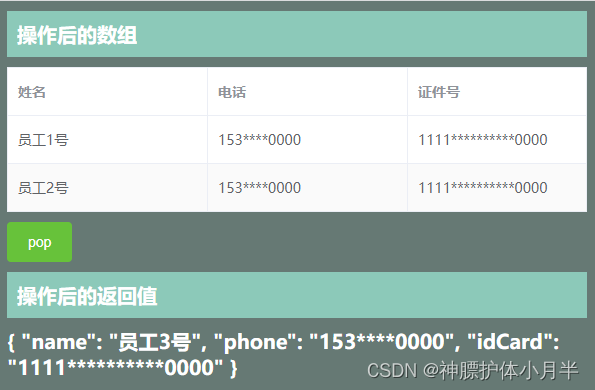
push
- 从尾部添加一项。
- 改变原有数组。
- 返回操作后的数组的长度。
<template>
<div class="myBlock">
<div class="tableBlock">
<div class="title">
{{ newObject ? "操作后的数组" : "操作前的数组" }}
</div>
<el-table :data="tableData" :key="num" stripe border>
<el-table-column prop="name" label="姓名" width="200">
</el-table-column>
<el-table-column prop="phone" label="电话" width="200">
</el-table-column>
<el-table-column prop="idCard" label="证件号">
</el-table-column>
</el-table>
</div>
<div class="operate">
<el-button type="success" plain @click="operate">
push
</el-button>
</div>
<div class="title" v-if="newObject">操作后的返回值</div>
<div class="newObject">
{{ newObject }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
num: 0,
newObject: null,
};
},
watch: {},
mounted() {
for (var i = 1; i < 4; i++) {
this.tableData.push({
name: "员工" + i + "号",
phone: "153****0000",
idCard: "1111**********0000",
});
}
},
methods: {
operate() {
this.newObject = this.tableData.push({
name: "test",
phone: "testPhone",
idCard: "testIdCars",
});
this.num += 1;
},
},
};
</script>
操作前
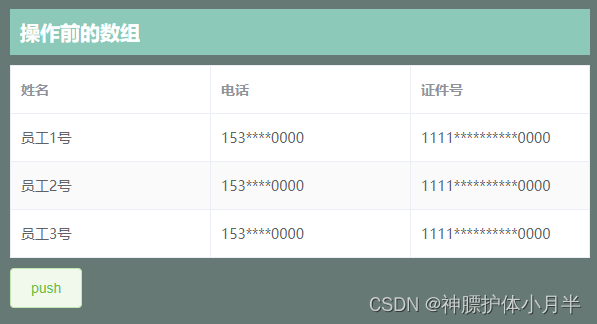
操作后
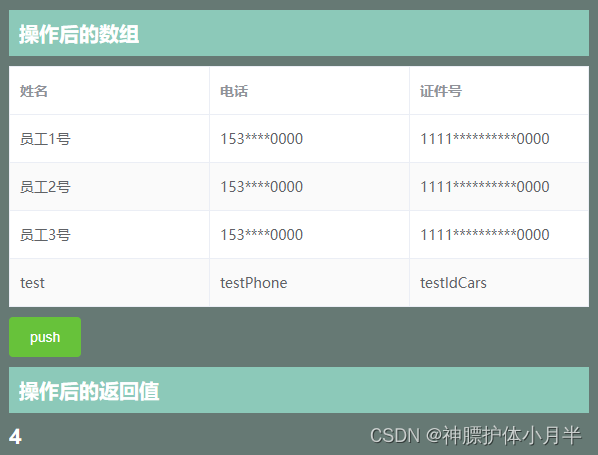
shift
<template>
<div class="myBlock">
<div class="tableBlock">
<div class="title">
{{ newObject ? "操作后的数组" : "操作前的数组" }}
</div>
<el-table :data="tableData" :key="num" stripe border>
<el-table-column prop="name" label="姓名" width="200">
</el-table-column>
<el-table-column prop="phone" label="电话" width="200">
</el-table-column>
<el-table-column prop="idCard" label="证件号">
</el-table-column>
</el-table>
</div>
<div class="operate">
<el-button type="success" plain @click="operate">
shift
</el-button>
</div>
<div class="title" v-if="newObject">操作后的返回值</div>
<div class="newObject">
{{ newObject }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
num: 0,
newObject: null,
};
},
watch: {},
mounted() {
for (var i = 1; i < 4; i++) {
this.tableData.push({
name: "员工" + i + "号",
phone: "153****0000",
idCard: "1111**********0000",
});
}
},
methods: {
operate() {
this.newObject = this.tableData.shift();
this.num += 1;
},
},
};
</script>
操作前
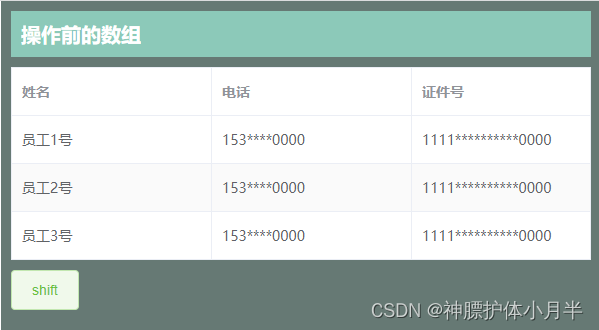
操作后
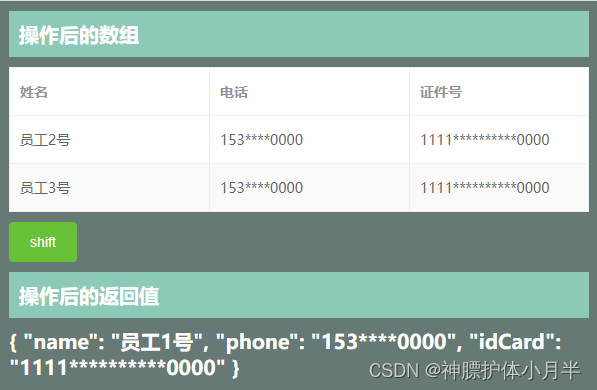
unshift
- 从头部添加一项。
- 改变原有数组。
- 返回添加后的数组的长度。
<template>
<div class="myBlock">
<div class="tableBlock">
<div class="title">
{{ newObject ? "操作后的数组" : "操作前的数组" }}
</div>
<el-table :data="tableData" :key="num" stripe border>
<el-table-column prop="name" label="姓名" width="200">
</el-table-column>
<el-table-column prop="phone" label="电话" width="200">
</el-table-column>
<el-table-column prop="idCard" label="证件号">
</el-table-column>
</el-table>
</div>
<div class="operate">
<el-button type="success" plain @click="operate">
unshift
</el-button>
</div>
<div class="title" v-if="newObject">操作后的返回值</div>
<div class="newObject">
{{ newObject }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [],
num: 0,
newObject: null,
};
},
watch: {},
mounted() {
for (var i = 1; i < 4; i++) {
this.tableData.push({
name: "员工" + i + "号",
phone: "153****0000",
idCard: "1111**********0000",
});
}
},
methods: {
operate() {
this.newObject = this.tableData.unshift({
name: "test",
phone: "testPhone",
idCard: "testIdCars",
});
this.num += 1;
},
},
};
</script>
操作前
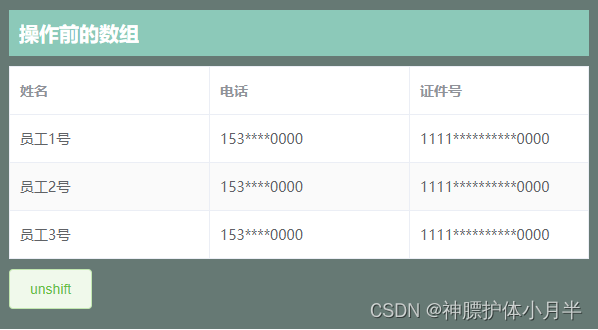
操作后
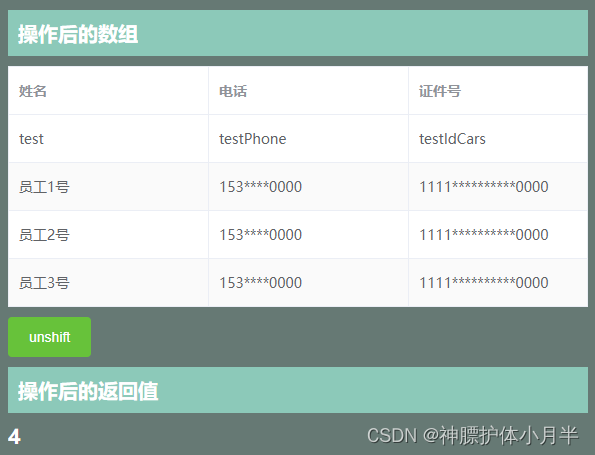