# 8.1 定义函数
def greet_user():
"""显示简单的问候语"""
print("Hello!")
greet_user()
## 8.1.1 向函数传递信息
def greet_user(username): #形参
"""显示简单的问候语"""
print(f"Hello,{username.title()}!")
greet_user("zxr") #实参
## 8.1.2 形参和实参
# 8.2 传递实参
位置实参+关键字实参
## 8.2.1 位置实参
任务:打印
I have a xxx
My xxxx's name is xxx
def describe_pet(animal_type,pet_name):
"""显示宠物地信息"""
print(f"\nI have a {animal_type}" )
print(f"My {animal_type}'s name is {pet_name}")
describe_pet('haster','wd')
基于实参的顺序在函数调用时把每个实参关联到函数定义中的形参
## 1,多次调用函数
def describe_pet(animal_type,pet_name):
"""显示宠物地信息"""
print(f"\nI have a {animal_type}" )
print(f"My {animal_type}'s name is {pet_name.title()}")
describe_pet('haster','wd')
describe_pet('sgsa','dd')
## 2,位置实参的顺序很重要
## 8.2.2 关键字实参
在实参中将名称和值关联起来
def describe_pet(animal_type,pet_name):
"""显示宠物地信息"""
print(f"\nI have a {animal_type}" )
print(f"My {animal_type}'s name is {pet_name.title()}")
describe_pet(animal_type = 'haster',pet_name = 'wd')
## 8.2.2 默认值
def describe_pet(pet_name,animal_type = "dog"):
"""显示宠物地信息"""
print(f"\nI have a {animal_type}" )
print(f"My {animal_type}'s name is {pet_name.title()}")
describe_pet(pet_name='whillie') #默认为形参的值
## 8.2.4 等效的函数调用
def describe_pet(pet_name,animal_type = "dog"):
"""显示宠物地信息"""
print(f"\nI have a {animal_type}" )
print(f"My {animal_type}'s name is {pet_name.title()}")
describe_pet(pet_name='whillie') #默认为形参的值 #关键字实参
describe_pet('whillie')
describe_pet('dke','wfjwo')
describe_pet(pet_name='dj',animal_type='dbj')
describe_pet(animal_type='dug',pet_name='dnj') #关键字,可不用顺序
## 8.2.5 避免实参错误
def describe_pet(pet_name,animal_type = "dog"):
"""显示宠物地信息"""
print(f"\nI have a {animal_type}" )
print(f"My {animal_type}'s name is {pet_name.title()}")
describe_pet()
# 8.3 返回值
return
## 8.3.1 返回简单值
def get_formatted_name(first_name,last_name):
"""返回整洁的名字"""
full_name = f"{first_name} {last_name}"
return full_name.title()
musician = get_formatted_name('Zhang',"Xiaorong")
print(musician)
## 8.3.2 让实参变为可选的
def get_formatted_name(first_name,middle_name,last_name):
"""返回整洁的名字"""
full_name = f"{first_name} {middle_name} {last_name}"
return full_name.title()
musician = get_formatted_name('Zhang',"sdaj","Xiaorong")
print(musician)
def get_formatted_name(first_name,last_name,middle_name=""): #额外设,放末尾
"""返回整洁的名字"""
#检查是否提供中间名
if middle_name: #if语句进行可选择性
full_name = f"{first_name} {middle_name} {last_name}"
else:
full_name = f"{first_name} {last_name}"
return full_name.title()
musician = get_formatted_name('Zhang',"sdaj","Xiaorong")
print(musician)
musician = get_formatted_name('Zhang',"sdaj")
print(musician)
## 8.3.3 返回字典
{'first':'jimi','last':'hendrix'}
def build_persion(first_name,last_name):
"""返回一个字典,其中包含有关一个人的信息"""
person = { 'first':first_name ,'last':last_name}
return person
muician = build_persion('z','xr')
print(muician)
def build_persion(first_name,last_name,age=None): #None是占位值.条件测试中,None相当于False
"""返回一个字典,其中包含有关一个人的信息"""
person = { 'first':first_name ,'last':last_name}
if age:
person['age'] = age #如果age由值,这个键值对添加到字典中
return person
muician = build_persion('z','xr',age=27)
print(muician)
## 8.3.4 结合使用函数和while循环
def get_formatted_name(first_name,last_name):
'''返回整洁的姓名'''
full_name = f'{first_name}{last_name}'
return full_name.title()
while True:
print("\nPlease tell me your name:")
print("enter 'q' at any time to quit")
f_name = input("First name:")
if f_name == 'q':
break
l_name = input("Last name:")
if l_name == 'q':
break
formatted_name = get_formatted_name(f_name,l_name)
print(f"\nHello,{formatted_name}")
# 8.4 传递列表
def greet_users(names):
'''向列表中的每位发出简单的问候'''
for name in names:
msg = f"Hello,{name.title()}!"
print(msg)
username = ['ecq','qce','c3']
greet_users(username)
## 8.4.1 在函数中修改列表
def print_model(unprinted_designs,completed_models):
"""打印资料"""
while unprinted_designs:
current_design = unprinted_designs.pop()
print(f"Printing model:{current_design}")
completed_models.append(current_design)
def show_completed_models(completed_models):
"""显示所有打印好的模型"""
print("\nThe following models have been printed:")
for completed_model in completed_models:
print(completed_model)
unprinted_designs = ['d3','2fc','2v4','34v']
completed_models = []
print_model(unprinted_designs,completed_models)
show_completed_models(completed_models)
## 8.4.2 禁止函数修改列表
#将列表的副本传递给函数 原来的列表值不变,不为空
function_name(list_name[:])
def print_model(unprinted_designs[:],completed_models): #切片方式
"""打印资料"""
while unprinted_designs:
current_design = unprinted_designs.pop()
print(f"Printing model:{current_design}")
completed_models.append(current_design)
def show_completed_models(completed_models):
"""显示所有打印好的模型"""
print("\nThe following models have been printed:")
for completed_model in completed_models:
print(completed_model)
unprinted_designs = ['d3','2fc','2v4','34v']
completed_models = []
print_model(unprinted_designs[:],completed_models)
show_completed_models(completed_models)
# 8.5 传递任意数量的实参
# def make_pizza(toppings):
# '''打印顾客点的所有配料'''
# print(toppings)
# make_pizza('ew')
# make_pizza('qwd','3f1','4f32')
# *创建一个topping的空元组 --- *toppings。可接受多个实参
def make_pizza(*toppings):
'''打印顾客点的所有配料'''
print(toppings)
make_pizza('ew')
make_pizza('qwd','3f1','4f32')
## 8.5.1 结合使用位置实参和任意数量实参
def make_pizza(size,*toppings): #先匹配关键字实参,再收集。*toppings写后面
'''打印顾客点的所有配料'''
print(f"\nMaking a {size}-inch pizza with the following toppings:")
print(toppings)
make_pizza(13,'ew')
make_pizza(12,'qwd','3f1','4f32')
## 8.5.2 使用任意数量的关键字实参
def build_profile(first,last,**user_info): #两个**,创建1一个空字典
"""创建一个字典,里面包含我们知道的有关用户的一切"""
user_info['first_name'] = first
user_info['last_name'] = last
return user_info
user_profile = build_profile('w','2e',location = 'we',field = 'w')
print(user_profile)
# 8.6将函数存储在模块中
#函数 def make_pizza: 保存名:pizza
## 8.6.1 导入整个模块
#导入模块
import pizza
pizza.make_pizza(...)
## 8.6.2 导入特定的函数
#导入模块下的特定函数
from pizza import make_pizza
make_pizza(...)
## 8.6.3 使用as给函数指定别名
#设置别名
from pizza import make_pizza as mp
mp(...)
## 8.6.4 使用as给模块指定别名
#模块指定别名
import pizza as p
p.make_pizza(...)
## 8.6.5 导入模块中的所有函数
#导入模块下的所有函数
from pizza import *
make_pizza(...)
python基础:七,函数
于 2023-07-16 11:27:24 首次发布
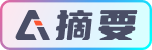